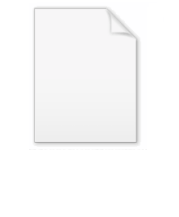
Return statement
Encyclopedia
In computer programming
, a return statement causes execution to leave the current subroutine
and resume at the point in the code immediately after where the subroutine was called, known as its return address. The return address is saved, usually on the process's
call stack
, as part of the operation of making the subroutine call. Return statements in many languages allow a function to specify a return value to be passed back to the code
that called the function.
/C++
,
) is a statement
that tells a function to return execution of the program to the calling function, and report the value of
, the return statement can be used without a value, in which case the program just breaks out of the current function and returns to the calling one.
In Pascal
there is no return statement. A subroutine automatically returns when execution reaches its last executable statement. Values may be returned by assigning to an identifier that has the same name as the subroutine, a function in Pascal terminology. This way the function identifier is used for recursive calls and as result holder. In other languages a user defined result variable is used instead of the function identifier.
Certain programming languages, such as Perl
and Ruby
, allow the programmer to omit an explicit return statement, specifying instead that the last evaluated expression is the return value of the subroutine. In Python
, the value
In Windows PowerShell
all evaluated expressions which are not captured (e.g. assigned to a variable, cast
to void
or piped
to $null
) are returned from the subroutine as elements in an array, or as a single object in the case that only one object has not been captured.
In Perl, a return value or values of a subroutine can depend on the context in which it was called. The most fundamental distinction is a scalar
context where the calling code expects one value, a list context where the calling code expects a list of values and a void
context where the calling code doesn't expect any return value at all. A subroutine can check the context using the
, and various reference
types contexts. Also, a context-sensitive object
can be returned using a contextual return sequence, with lazy evaluation
of scalar values.
Values returned by the program when it terminates are often captured by batch programs
.
statement. For instance, that in later development, a return statement could be overlooked by a developer, and an action which should be performed at the end of a subroutine (e.g.: a trace
statement) might not be performed in all cases. Conversely, it can be argued that using the return statement is worthwhile when the alternative is more convoluted code, harming readability.
Some early implementations of languages such as the original Pascal and C restricted the types that can be returned by a function( e.g., not supporting record or struct types) to simplify their compiler
s.
Computer programming
Computer programming is the process of designing, writing, testing, debugging, and maintaining the source code of computer programs. This source code is written in one or more programming languages. The purpose of programming is to create a program that performs specific operations or exhibits a...
, a return statement causes execution to leave the current subroutine
Subroutine
In computer science, a subroutine is a portion of code within a larger program that performs a specific task and is relatively independent of the remaining code....
and resume at the point in the code immediately after where the subroutine was called, known as its return address. The return address is saved, usually on the process's
Process (computing)
In computing, a process is an instance of a computer program that is being executed. It contains the program code and its current activity. Depending on the operating system , a process may be made up of multiple threads of execution that execute instructions concurrently.A computer program is a...
call stack
Call stack
In computer science, a call stack is a stack data structure that stores information about the active subroutines of a computer program. This kind of stack is also known as an execution stack, control stack, run-time stack, or machine stack, and is often shortened to just "the stack"...
, as part of the operation of making the subroutine call. Return statements in many languages allow a function to specify a return value to be passed back to the code
Source code
In computer science, source code is text written using the format and syntax of the programming language that it is being written in. Such a language is specially designed to facilitate the work of computer programmers, who specify the actions to be performed by a computer mostly by writing source...
that called the function.
Overview
In CC (programming language)
C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system....
/C++
C++
C++ is a statically typed, free-form, multi-paradigm, compiled, general-purpose programming language. It is regarded as an intermediate-level language, as it comprises a combination of both high-level and low-level language features. It was developed by Bjarne Stroustrup starting in 1979 at Bell...
,
return exp;
(where exp
is an expressionExpression (programming)
An expression in a programming language is a combination of explicit values, constants, variables, operators, and functions that are interpreted according to the particular rules of precedence and of association for a particular programming language, which computes and then produces another value...
) is a statement
Statement (programming)
In computer programming a statement can be thought of as the smallest standalone element of an imperative programming language. A program written in such a language is formed by a sequence of one or more statements. A statement will have internal components .Many languages In computer programming...
that tells a function to return execution of the program to the calling function, and report the value of
exp
. If a function has the return type voidVoid type
The void type, in several programming languages derived from C and Algol68, is the type for the result of a function that returns normally, but does not provide a result value to its caller. Usually such functions are called for their side effects, such as performing some task or writing to their...
, the return statement can be used without a value, in which case the program just breaks out of the current function and returns to the calling one.
In Pascal
Pascal (programming language)
Pascal is an influential imperative and procedural programming language, designed in 1968/9 and published in 1970 by Niklaus Wirth as a small and efficient language intended to encourage good programming practices using structured programming and data structuring.A derivative known as Object Pascal...
there is no return statement. A subroutine automatically returns when execution reaches its last executable statement. Values may be returned by assigning to an identifier that has the same name as the subroutine, a function in Pascal terminology. This way the function identifier is used for recursive calls and as result holder. In other languages a user defined result variable is used instead of the function identifier.
Certain programming languages, such as Perl
Perl
Perl is a high-level, general-purpose, interpreted, dynamic programming language. Perl was originally developed by Larry Wall in 1987 as a general-purpose Unix scripting language to make report processing easier. Since then, it has undergone many changes and revisions and become widely popular...
and Ruby
Ruby (programming language)
Ruby is a dynamic, reflective, general-purpose object-oriented programming language that combines syntax inspired by Perl with Smalltalk-like features. Ruby originated in Japan during the mid-1990s and was first developed and designed by Yukihiro "Matz" Matsumoto...
, allow the programmer to omit an explicit return statement, specifying instead that the last evaluated expression is the return value of the subroutine. In Python
Python (programming language)
Python is a general-purpose, high-level programming language whose design philosophy emphasizes code readability. Python claims to "[combine] remarkable power with very clear syntax", and its standard library is large and comprehensive...
, the value
None
is returned when the return statement is omitted.In Windows PowerShell
Windows PowerShell
Windows PowerShell is Microsoft's task automation framework, consisting of a command-line shell and associated scripting language built on top of, and integrated with the .NET Framework...
all evaluated expressions which are not captured (e.g. assigned to a variable, cast
Type conversion
In computer science, type conversion, typecasting, and coercion are different ways of, implicitly or explicitly, changing an entity of one data type into another. This is done to take advantage of certain features of type hierarchies or type representations...
to void
Void type
The void type, in several programming languages derived from C and Algol68, is the type for the result of a function that returns normally, but does not provide a result value to its caller. Usually such functions are called for their side effects, such as performing some task or writing to their...
or piped
Pipeline (Unix)
In Unix-like computer operating systems , a pipeline is the original software pipeline: a set of processes chained by their standard streams, so that the output of each process feeds directly as input to the next one. Each connection is implemented by an anonymous pipe...
to $null
/dev/null
In Unix-like operating systems, /dev/null or the null device is a special file that discards all data written to it and provides no data to any process that reads from it ....
) are returned from the subroutine as elements in an array, or as a single object in the case that only one object has not been captured.
In Perl, a return value or values of a subroutine can depend on the context in which it was called. The most fundamental distinction is a scalar
Scalar (computing)
In computing, a scalar variable or field is one that can hold only one value at a time; as opposed to composite variables like array, list, hash, record, etc. In some contexts, a scalar value may be understood to be numeric. A scalar data type is the type of a scalar variable...
context where the calling code expects one value, a list context where the calling code expects a list of values and a void
Void type
The void type, in several programming languages derived from C and Algol68, is the type for the result of a function that returns normally, but does not provide a result value to its caller. Usually such functions are called for their side effects, such as performing some task or writing to their...
context where the calling code doesn't expect any return value at all. A subroutine can check the context using the
wantarray
function. A special syntax of return without arguments is used to return an undefined value in scalar context and an empty list in list context. The scalar context can be further divided into Boolean, number, stringString (computer science)
In formal languages, which are used in mathematical logic and theoretical computer science, a string is a finite sequence of symbols that are chosen from a set or alphabet....
, and various reference
Reference (computer science)
In computer science, a reference is a value that enables a program to indirectly access a particular data item, such as a variable or a record, in the computer's memory or in some other storage device. The reference is said to refer to the data item, and accessing those data is called...
types contexts. Also, a context-sensitive object
Object (computer science)
In computer science, an object is any entity that can be manipulated by the commands of a programming language, such as a value, variable, function, or data structure...
can be returned using a contextual return sequence, with lazy evaluation
Lazy evaluation
In programming language theory, lazy evaluation or call-by-need is an evaluation strategy which delays the evaluation of an expression until the value of this is actually required and which also avoids repeated evaluations...
of scalar values.
Values returned by the program when it terminates are often captured by batch programs
Batch file
In DOS, OS/2, and Microsoft Windows, batch file is the name given to a type of script file, a text file containing a series of commands to be executed by the command interpreter....
.
Syntax
Return statements come in many shapes. The following syntaxes are most common:Language | Return Statement | If value omitted, Return |
---|---|---|
Ada Ada (programming language) Ada is a structured, statically typed, imperative, wide-spectrum, and object-oriented high-level computer programming language, extended from Pascal and other languages... , C C (programming language) C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system.... , C++ C++ C++ is a statically typed, free-form, multi-paradigm, compiled, general-purpose programming language. It is regarded as an intermediate-level language, as it comprises a combination of both high-level and low-level language features. It was developed by Bjarne Stroustrup starting in 1979 at Bell... , Java Java (programming language) Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities... , PHP PHP PHP is a general-purpose server-side scripting language originally designed for web development to produce dynamic web pages. For this purpose, PHP code is embedded into the HTML source document and interpreted by a web server with a PHP processor module, which generates the web page document... , C#, JavaScript JavaScript JavaScript is a prototype-based scripting language that is dynamic, weakly typed and has first-class functions. It is a multi-paradigm language, supporting object-oriented, imperative, and functional programming styles.... |
|
in C and C++, undefined if function is value-returning |
BASIC BASIC BASIC is a family of general-purpose, high-level programming languages whose design philosophy emphasizes ease of use - the name is an acronym from Beginner's All-purpose Symbolic Instruction Code.... |
|
|
Lisp | |
last statement value |
Perl Perl Perl is a high-level, general-purpose, interpreted, dynamic programming language. Perl was originally developed by Larry Wall in 1987 as a general-purpose Unix scripting language to make report processing easier. Since then, it has undergone many changes and revisions and become widely popular... , Ruby Ruby (programming language) Ruby is a dynamic, reflective, general-purpose object-oriented programming language that combines syntax inspired by Perl with Smalltalk-like features. Ruby originated in Japan during the mid-1990s and was first developed and designed by Yukihiro "Matz" Matsumoto... |
return $value; return; or a contextual return sequence |
last statement value |
Python Python (programming language) Python is a general-purpose, high-level programming language whose design philosophy emphasizes code readability. Python claims to "[combine] remarkable power with very clear syntax", and its standard library is large and comprehensive... |
|
None |
Smalltalk Smalltalk Smalltalk is an object-oriented, dynamically typed, reflective programming language. Smalltalk was created as the language to underpin the "new world" of computing exemplified by "human–computer symbiosis." It was designed and created in part for educational use, more so for constructionist... |
|
|
Visual Basic .NET Visual Basic .NET Visual Basic .NET , is an object-oriented computer programming language that can be viewed as an evolution of the classic Visual Basic , which is implemented on the .NET Framework... |
|
|
Windows PowerShell Windows PowerShell Windows PowerShell is Microsoft's task automation framework, consisting of a command-line shell and associated scripting language built on top of, and integrated with the .NET Framework... |
|
object |
x86 assembly | |
contents of eax register (by convention) |
Java Java Java is an island of Indonesia. With a population of 135 million , it is the world's most populous island, and one of the most densely populated regions in the world. It is home to 60% of Indonesia's population. The Indonesian capital city, Jakarta, is in west Java... |
|
Returns Object value. |
Criticism
It has been argued that one should eschew the use of the explicit return statement except at the textual end of a subroutine, considering that, when it is used to "return early", it may suffer from the same sort of readability problems that are claimed to exist for the GOTOGoto
goto is a statement found in many computer programming languages. It is a combination of the English words go and to. It performs a one-way transfer of control to another line of code; in contrast a function call normally returns control...
statement. For instance, that in later development, a return statement could be overlooked by a developer, and an action which should be performed at the end of a subroutine (e.g.: a trace
Tracing (software)
In software engineering, tracing is a specialized use of logging to record information about a program's execution. This information is typically used by programmers for debugging purposes, and additionally, depending on the type and detail of information contained in a trace log, by experienced...
statement) might not be performed in all cases. Conversely, it can be argued that using the return statement is worthwhile when the alternative is more convoluted code, harming readability.
Some early implementations of languages such as the original Pascal and C restricted the types that can be returned by a function( e.g., not supporting record or struct types) to simplify their compiler
Compiler
A compiler is a computer program that transforms source code written in a programming language into another computer language...
s.