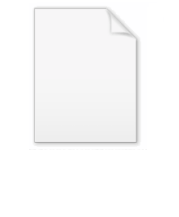
Statement (programming)
Encyclopedia
In computer programming
a statement can be thought of as the smallest standalone element of an imperative
programming language
. A program written in such a language is formed by a sequence of one or more statements. A statement will have internal components (e.g., expressions).
Many languages (e.g. C
) make a distinction between statements and definitions, with a statement only containing executable code and a definition declaring an identifier
. A distinction can also be made between simple and compound statements; the latter may contain statements as components.
like if, while or repeat. Often statement keywords
are reserved such that they cannot be used as names of variables
or functions. Imperative languages typically use special syntax for each statement, which looks quite different to function calls. Common methods to describe the syntax of statements are Backus–Naur Form
and syntax diagrams.
calls by their handling of parameters
. Usually an actual subroutine parameter is evaluated once before the subroutine is called. This contrasts to many statement parameters which can be evaluated several times (e.g. the condition of a while loop
) or not at all (e.g. the loop body of a while loop). Technically such statement parameters are closures
which are executed when needed (see also lazy evaluation
). When closure parameters are available for subroutines a statement like behaviour can be implemented by subroutines also (see Lisp). For languages without closure parameters the semantic description of a loop or conditional is usually beyond the capabilities of the language. Therefore standard documents often refer to semantic descriptions in natural language.
s in that statements do not return results and are executed solely for their side effects, while expressions always return a result and often do not have side effects at all. Among imperative programming languages, Algol 68
is one of the few in which a statement can return a result. In languages which mix imperative and functional
styles, such as the Lisp family, the distinction between expressions and statements is not made: even expressions executed in sequential contexts solely for their side effects and whose return values are not used are considered 'expressions'. In purely functional
programming, there are no statements; everything is an expression.
Computer programming
Computer programming is the process of designing, writing, testing, debugging, and maintaining the source code of computer programs. This source code is written in one or more programming languages. The purpose of programming is to create a program that performs specific operations or exhibits a...
a statement can be thought of as the smallest standalone element of an imperative
Imperative programming
In computer science, imperative programming is a programming paradigm that describes computation in terms of statements that change a program state...
programming language
Programming language
A programming language is an artificial language designed to communicate instructions to a machine, particularly a computer. Programming languages can be used to create programs that control the behavior of a machine and/or to express algorithms precisely....
. A program written in such a language is formed by a sequence of one or more statements. A statement will have internal components (e.g., expressions).
Many languages (e.g. C
C (programming language)
C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system....
) make a distinction between statements and definitions, with a statement only containing executable code and a definition declaring an identifier
Identifier
An identifier is a name that identifies either a unique object or a unique class of objects, where the "object" or class may be an idea, physical [countable] object , or physical [noncountable] substance...
. A distinction can also be made between simple and compound statements; the latter may contain statements as components.
Kinds of statements
The following are the major generic kinds of statements with examples in typical imperative languages:Simple statements
- assignmentAssignment (computer science)In computer programming, an assignment statement sets or re-sets the value stored in the storage location denoted by a variable name. In most imperative computer programming languages, assignment statements are one of the basic statements...
:A:= A + 1
- callSubroutineIn computer science, a subroutine is a portion of code within a larger program that performs a specific task and is relatively independent of the remaining code....
:CLEARSCREEN
- returnReturn statementIn computer programming, a return statement causes execution to leave the current subroutine and resume at the point in the code immediately after where the subroutine was called, known as its return address. The return address is saved, usually on the process's call stack, as part of the operation...
:return 5;
- gotoGotogoto is a statement found in many computer programming languages. It is a combination of the English words go and to. It performs a one-way transfer of control to another line of code; in contrast a function call normally returns control...
:goto 1
- assertionAssertion (computing)In computer programming, an assertion is a predicate placed in a program to indicate that the developer thinks that the predicate is always true at that place.For example, the following code contains two assertions:...
:assert(ptr != NULL);
Compound statements
- block:
begin integer NUMBER; WRITE('Number? '); READLN(NUMBER); A:= A*NUMBER end
- if-statementConditional statementIn computer science, conditional statements, conditional expressions and conditional constructs are features of a programming language which perform different computations or actions depending on whether a programmer-specified boolean condition evaluates to true or false...
:if A > 3 then WRITELN(A) else WRITELN("NOT YET"); end
- switch-statementSwitch statementIn computer programming, a switch, case, select or inspect statement is a type of selection control mechanism that exists in most imperative programming languages such as Pascal, Ada, C/C++, C#, Java, and so on. It is also included in several other types of languages...
:switch (c) { case 'a': alert; break; case 'q': quit; break; }
- while-loopWhile loopIn most computer programming languages, a while loop is a control flow statement that allows code to be executed repeatedly based on a given boolean condition. The while loop can be thought of as a repeating if statement....
:while NOT EOF DO begin READLN end
- do-loopDo while loopIn most computer programming languages, a do while loop, sometimes just called a do loop, is a control flow statement that allows code to be executed repeatedly based on a given Boolean condition. Note though that unlike most languages, Fortran's do loop is actually analogous to the for loop.The...
:do { computation(&i); } while (i < 10);
- for-loopFor loopIn computer science a for loop is a programming language statement which allows code to be repeatedly executed. A for loop is classified as an iteration statement....
:for A:=1 to 10 do WRITELN(A) end
Syntax
The appearance of statements shapes the look of programs. Programming languages are characterized by the flavor of statements they use (e.g.: The curly brace language family). Many statements are introduced by identifiersIdentifier
An identifier is a name that identifies either a unique object or a unique class of objects, where the "object" or class may be an idea, physical [countable] object , or physical [noncountable] substance...
like if, while or repeat. Often statement keywords
Keyword (computer programming)
In computer programming, a keyword is a word or identifier that has a particular meaning to the programming language. The meaning of keywords — and, indeed, the meaning of the notion of keyword — differs widely from language to language....
are reserved such that they cannot be used as names of variables
Variable (programming)
In computer programming, a variable is a symbolic name given to some known or unknown quantity or information, for the purpose of allowing the name to be used independently of the information it represents...
or functions. Imperative languages typically use special syntax for each statement, which looks quite different to function calls. Common methods to describe the syntax of statements are Backus–Naur Form
Backus–Naur form
In computer science, BNF is a notation technique for context-free grammars, often used to describe the syntax of languages used in computing, such as computer programming languages, document formats, instruction sets and communication protocols.It is applied wherever exact descriptions of...
and syntax diagrams.
Semantics
Semantically many statements differ from subroutineSubroutine
In computer science, a subroutine is a portion of code within a larger program that performs a specific task and is relatively independent of the remaining code....
calls by their handling of parameters
Parameter (computer science)
In computer programming, a parameter is a special kind of variable, used in a subroutine to refer to one of the pieces of data provided as input to the subroutine. These pieces of data are called arguments...
. Usually an actual subroutine parameter is evaluated once before the subroutine is called. This contrasts to many statement parameters which can be evaluated several times (e.g. the condition of a while loop
While loop
In most computer programming languages, a while loop is a control flow statement that allows code to be executed repeatedly based on a given boolean condition. The while loop can be thought of as a repeating if statement....
) or not at all (e.g. the loop body of a while loop). Technically such statement parameters are closures
Closure (computer science)
In computer science, a closure is a function together with a referencing environment for the non-local variables of that function. A closure allows a function to access variables outside its typical scope. Such a function is said to be "closed over" its free variables...
which are executed when needed (see also lazy evaluation
Lazy evaluation
In programming language theory, lazy evaluation or call-by-need is an evaluation strategy which delays the evaluation of an expression until the value of this is actually required and which also avoids repeated evaluations...
). When closure parameters are available for subroutines a statement like behaviour can be implemented by subroutines also (see Lisp). For languages without closure parameters the semantic description of a loop or conditional is usually beyond the capabilities of the language. Therefore standard documents often refer to semantic descriptions in natural language.
Expressions
In most languages, statements contrast with expressionExpression (programming)
An expression in a programming language is a combination of explicit values, constants, variables, operators, and functions that are interpreted according to the particular rules of precedence and of association for a particular programming language, which computes and then produces another value...
s in that statements do not return results and are executed solely for their side effects, while expressions always return a result and often do not have side effects at all. Among imperative programming languages, Algol 68
ALGOL 68
ALGOL 68 isan imperative computerprogramming language that was conceived as a successor to theALGOL 60 programming language, designed with the goal of a...
is one of the few in which a statement can return a result. In languages which mix imperative and functional
Functional programming
In computer science, functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids state and mutable data. It emphasizes the application of functions, in contrast to the imperative programming style, which emphasizes changes in state...
styles, such as the Lisp family, the distinction between expressions and statements is not made: even expressions executed in sequential contexts solely for their side effects and whose return values are not used are considered 'expressions'. In purely functional
Purely functional
Purely functional is a term in computing used to describe algorithms, data structures or programming languages that exclude destructive modifications...
programming, there are no statements; everything is an expression.
Extensibility
Most languages have a fixed set of statements defined by the language, but there have been experiments with extensible languages which allow the programmer to define new statements.See also
- Control flowControl flowIn computer science, control flow refers to the order in which the individual statements, instructions, or function calls of an imperative or a declarative program are executed or evaluated....
- ExpressionExpression (programming)An expression in a programming language is a combination of explicit values, constants, variables, operators, and functions that are interpreted according to the particular rules of precedence and of association for a particular programming language, which computes and then produces another value...
(contrast) - Comparison of Programming Languages - Statements
- Extensible languages