.gif)
Process (computing)
Encyclopedia
In computing, a process is an instance
of a computer program
that is being executed. It contains the program code and its current activity. Depending on the operating system
(OS), a process may be made up of multiple threads of execution
that execute instructions concurrently
.
A computer program is a passive collection of instructions; a process is the actual execution of those instructions. Several processes may be associated with the same program; for example, opening up several instances of the same program often means more than one process is being executed.
Multitasking
is a method to allow multiple processes to share processors (CPU
s) and other system resources. Each CPU executes a single task at a time. However, multitasking allows each processor to switch
between tasks that are being executed without having to wait for each task to finish. Depending on the operating system implementation, switches could be performed when tasks perform input/output
operations, when a task indicates that it can be switched, or on hardware interrupts.
A common form of multitasking is time-sharing
. Time-sharing is a method to allow fast response for interactive user applications. In time-sharing systems, context switch
es are performed rapidly. This makes it seem like multiple processes are being executed simultaneously on the same processor. The execution of multiple processes seemingly simultaneously is called concurrency
.
For security and reliability reasons most modern operating system
s prevent direct communication
between independent processes, providing strictly mediated and controlled inter-process communication functionality.
The operating system holds most of this information about active processes in data structures called process control block
s
Any subset of resource, but typically at least the processor state, may be associated with each of the process' threads
in operating systems that support threads or 'daughter' processes.
The operating system keeps its processes separated and allocates the resources they need, so that they are less likely to interfere with each other and cause system failures (e.g., deadlock
or thrashing). The operating system may also provide mechanisms for inter-process communication
to enable processes to interact in safe and predictable ways.
* operating system
may just switch between processes to give the appearance of many processes executing
concurrently
or simultaneously, though in fact only one process can be executing at any one time on a single-core CPU
(unless using multi-threading or other similar technology).
It is usual to associate a single process with a main program, and 'daughter' ('child') processes with any spin-off, parallel processes, which behave like asynchronous subroutines. A process is said to own resources, of which an image of its program (in memory) is one such resource. (Note, however, that in multiprocessing systems, many processes may run off of, or share, the same reentrant program at the same location in memory— but each process is said to own its own image of the program.)
Processes are often called tasks in embedded
operating systems. The sense of 'process' (or task) is 'something that takes up time', as opposed to 'memory', which is 'something that takes up space'. (Historically, the terms 'task' and 'process' were used interchangeably, but the term 'task' seems to be dropping from the computer lexicon.)
The above description applies to both processes managed by an operating system, and processes as defined by process calculi.
If a process requests something for which it must wait, it will be blocked. When the process is in the Blocked State
, it is eligible for swapping to disk, but this is transparent in a virtual memory
system, where blocks of memory values may be really on disk and not in main memory at any time. Note that even unused portions of active processes/tasks (executing programs) are eligible for swapping to disk. All parts of an executing program and its data do not have to be in physical memory for the associated process to be active.
______________________________
*Tasks and processes refer essentially to the same entity. And, although they have somewhat different terminological histories, they have come to be used as synonyms. Today, the term process is generally preferred over task, except when referring to 'multitasking', since the alternative term, 'multiprocessing', is too easy to confuse with multiprocessor (which is a computer with two or more CPUs).
An operating system kernel that allows multi-tasking needs processes to have certain states
. Names for these states are not standardised, but they have similar functionality.
Processes frequently need to communicate, for instance in a shell pipeline, the output of the first process need to pass to the second one, and so on to the other process. It is preferred in a well-structured way not using interrupts.
It is even possible for the two processes to be running on different machines. The operating system (OS) may differ from one process to the other, therefore some mediator(s) (called protocols) are needed.
, to Executive control software. Computers got "faster" and computer time
was still neither "cheap" nor fully used. It made multiprogramming
possible and necessary.
Multiprogramming means that several programs run "at the same time" (concurrently
). At first they ran on a single processor (i.e., uniprocessor
) and shared scarce resources. Multiprogramming is also basic form of multiprocessing
, a much broader term.
Programs consist of sequence of instruction for processor. A single processor can run only one instruction at a time. Therefore it is impossible to run more programs at the same time. A program might need some resource
(input ...) which has a "big" delay. A program might also start some slow operation (output to printer ...). This all leads to processor being "idle" (unused). To use processor at all time the execution of such program was halted. At that point, a second (or nth) program was started or restarted. User perceived that programs run "at the same time" (hence the term, concurrent).
Shortly thereafter, the notion of a 'program' was expanded to the notion of an 'executing program and its context'. The concept of a process was born.
This became necessary with the invention of re-entrant code.
Thread
s came somewhat later. However, with the advent of time-sharing
; computer networks; multiple-CPU, shared memory
computers; etc., the old "multiprogramming" gave way to true multitasking
, multiprocessing and, later, multithreading
.
Object (computer science)
In computer science, an object is any entity that can be manipulated by the commands of a programming language, such as a value, variable, function, or data structure...
of a computer program
Computer program
A computer program is a sequence of instructions written to perform a specified task with a computer. A computer requires programs to function, typically executing the program's instructions in a central processor. The program has an executable form that the computer can use directly to execute...
that is being executed. It contains the program code and its current activity. Depending on the operating system
Operating system
An operating system is a set of programs that manage computer hardware resources and provide common services for application software. The operating system is the most important type of system software in a computer system...
(OS), a process may be made up of multiple threads of execution
Thread (computer science)
In computer science, a thread of execution is the smallest unit of processing that can be scheduled by an operating system. The implementation of threads and processes differs from one operating system to another, but in most cases, a thread is contained inside a process...
that execute instructions concurrently
Concurrency (computer science)
In computer science, concurrency is a property of systems in which several computations are executing simultaneously, and potentially interacting with each other...
.
A computer program is a passive collection of instructions; a process is the actual execution of those instructions. Several processes may be associated with the same program; for example, opening up several instances of the same program often means more than one process is being executed.
Multitasking
Computer multitasking
In computing, multitasking is a method where multiple tasks, also known as processes, share common processing resources such as a CPU. In the case of a computer with a single CPU, only one task is said to be running at any point in time, meaning that the CPU is actively executing instructions for...
is a method to allow multiple processes to share processors (CPU
Central processing unit
The central processing unit is the portion of a computer system that carries out the instructions of a computer program, to perform the basic arithmetical, logical, and input/output operations of the system. The CPU plays a role somewhat analogous to the brain in the computer. The term has been in...
s) and other system resources. Each CPU executes a single task at a time. However, multitasking allows each processor to switch
Context switch
A context switch is the computing process of storing and restoring the state of a CPU so that execution can be resumed from the same point at a later time. This enables multiple processes to share a single CPU. The context switch is an essential feature of a multitasking operating system...
between tasks that are being executed without having to wait for each task to finish. Depending on the operating system implementation, switches could be performed when tasks perform input/output
Input/output
In computing, input/output, or I/O, refers to the communication between an information processing system , and the outside world, possibly a human, or another information processing system. Inputs are the signals or data received by the system, and outputs are the signals or data sent from it...
operations, when a task indicates that it can be switched, or on hardware interrupts.
A common form of multitasking is time-sharing
Time-sharing
Time-sharing is the sharing of a computing resource among many users by means of multiprogramming and multi-tasking. Its introduction in the 1960s, and emergence as the prominent model of computing in the 1970s, represents a major technological shift in the history of computing.By allowing a large...
. Time-sharing is a method to allow fast response for interactive user applications. In time-sharing systems, context switch
Context switch
A context switch is the computing process of storing and restoring the state of a CPU so that execution can be resumed from the same point at a later time. This enables multiple processes to share a single CPU. The context switch is an essential feature of a multitasking operating system...
es are performed rapidly. This makes it seem like multiple processes are being executed simultaneously on the same processor. The execution of multiple processes seemingly simultaneously is called concurrency
Concurrency (computer science)
In computer science, concurrency is a property of systems in which several computations are executing simultaneously, and potentially interacting with each other...
.
For security and reliability reasons most modern operating system
Operating system
An operating system is a set of programs that manage computer hardware resources and provide common services for application software. The operating system is the most important type of system software in a computer system...
s prevent direct communication
Inter-process communication
In computing, Inter-process communication is a set of methods for the exchange of data among multiple threads in one or more processes. Processes may be running on one or more computers connected by a network. IPC methods are divided into methods for message passing, synchronization, shared...
between independent processes, providing strictly mediated and controlled inter-process communication functionality.
Representation
In general, a computer system process consists of (or is said to 'own') the following resources:- An image of the executable machine codeMachine codeMachine code or machine language is a system of impartible instructions executed directly by a computer's central processing unit. Each instruction performs a very specific task, typically either an operation on a unit of data Machine code or machine language is a system of impartible instructions...
associated with a program. - Memory (typically some region of virtual memoryVirtual memoryIn computing, virtual memory is a memory management technique developed for multitasking kernels. This technique virtualizes a computer architecture's various forms of computer data storage , allowing a program to be designed as though there is only one kind of memory, "virtual" memory, which...
); which includes the executable code, process-specific data (input and output), a call stackCall stackIn computer science, a call stack is a stack data structure that stores information about the active subroutines of a computer program. This kind of stack is also known as an execution stack, control stack, run-time stack, or machine stack, and is often shortened to just "the stack"...
(to keep track of active subroutines and/or other events), and a heap to hold intermediate computation data generated during run time. - Operating system descriptors of resources that are allocated to the process, such as file descriptorFile descriptorIn computer programming, a file descriptor is an abstract indicator for accessing a file. The term is generally used in POSIX operating systems...
s (UnixUnixUnix is a multitasking, multi-user computer operating system originally developed in 1969 by a group of AT&T employees at Bell Labs, including Ken Thompson, Dennis Ritchie, Brian Kernighan, Douglas McIlroy, and Joe Ossanna...
terminology) or handlesHandle (computing)In computer programming, a handle is a particular kind of smart pointer. Handles are used when application software references blocks of memory or objects managed by another system, such as a database or an operating system....
(WindowsMicrosoft WindowsMicrosoft Windows is a series of operating systems produced by Microsoft.Microsoft introduced an operating environment named Windows on November 20, 1985 as an add-on to MS-DOS in response to the growing interest in graphical user interfaces . Microsoft Windows came to dominate the world's personal...
), and data sources and sinks. - SecurityComputer securityComputer security is a branch of computer technology known as information security as applied to computers and networks. The objective of computer security includes protection of information and property from theft, corruption, or natural disaster, while allowing the information and property to...
attributes, such as the process owner and the process' set of permissions (allowable operations). - ProcessorCentral processing unitThe central processing unit is the portion of a computer system that carries out the instructions of a computer program, to perform the basic arithmetical, logical, and input/output operations of the system. The CPU plays a role somewhat analogous to the brain in the computer. The term has been in...
state (context), such as the content of registersProcessor registerIn computer architecture, a processor register is a small amount of storage available as part of a CPU or other digital processor. Such registers are addressed by mechanisms other than main memory and can be accessed more quickly...
, physical memory addressing, etc. The state is typically stored in computer registers when the process is executing, and in memory otherwise.
The operating system holds most of this information about active processes in data structures called process control block
Process control block
Process Control Block is a data structure in the operating system kernel containing the information needed to manage a particular process...
s
Any subset of resource, but typically at least the processor state, may be associated with each of the process' threads
Thread (computer science)
In computer science, a thread of execution is the smallest unit of processing that can be scheduled by an operating system. The implementation of threads and processes differs from one operating system to another, but in most cases, a thread is contained inside a process...
in operating systems that support threads or 'daughter' processes.
The operating system keeps its processes separated and allocates the resources they need, so that they are less likely to interfere with each other and cause system failures (e.g., deadlock
Deadlock
A deadlock is a situation where in two or more competing actions are each waiting for the other to finish, and thus neither ever does. It is often seen in a paradox like the "chicken or the egg"...
or thrashing). The operating system may also provide mechanisms for inter-process communication
Inter-process communication
In computing, Inter-process communication is a set of methods for the exchange of data among multiple threads in one or more processes. Processes may be running on one or more computers connected by a network. IPC methods are divided into methods for message passing, synchronization, shared...
to enable processes to interact in safe and predictable ways.
Process management in multi-tasking operating systems
A multitaskingComputer multitasking
In computing, multitasking is a method where multiple tasks, also known as processes, share common processing resources such as a CPU. In the case of a computer with a single CPU, only one task is said to be running at any point in time, meaning that the CPU is actively executing instructions for...
* operating system
Operating system
An operating system is a set of programs that manage computer hardware resources and provide common services for application software. The operating system is the most important type of system software in a computer system...
may just switch between processes to give the appearance of many processes executing
Execution (computers)
Execution in computer and software engineering is the process by which a computer or a virtual machine carries out the instructions of a computer program. The instructions in the program trigger sequences of simple actions on the executing machine...
concurrently
Concurrency (computer science)
In computer science, concurrency is a property of systems in which several computations are executing simultaneously, and potentially interacting with each other...
or simultaneously, though in fact only one process can be executing at any one time on a single-core CPU
Central processing unit
The central processing unit is the portion of a computer system that carries out the instructions of a computer program, to perform the basic arithmetical, logical, and input/output operations of the system. The CPU plays a role somewhat analogous to the brain in the computer. The term has been in...
(unless using multi-threading or other similar technology).
It is usual to associate a single process with a main program, and 'daughter' ('child') processes with any spin-off, parallel processes, which behave like asynchronous subroutines. A process is said to own resources, of which an image of its program (in memory) is one such resource. (Note, however, that in multiprocessing systems, many processes may run off of, or share, the same reentrant program at the same location in memory— but each process is said to own its own image of the program.)
Processes are often called tasks in embedded
Embedded system
An embedded system is a computer system designed for specific control functions within a larger system. often with real-time computing constraints. It is embedded as part of a complete device often including hardware and mechanical parts. By contrast, a general-purpose computer, such as a personal...
operating systems. The sense of 'process' (or task) is 'something that takes up time', as opposed to 'memory', which is 'something that takes up space'. (Historically, the terms 'task' and 'process' were used interchangeably, but the term 'task' seems to be dropping from the computer lexicon.)
The above description applies to both processes managed by an operating system, and processes as defined by process calculi.
If a process requests something for which it must wait, it will be blocked. When the process is in the Blocked State
Process states
In a multitasking computer system, processes may occupy a variety of states. These distinct states may not actually be recognized as such by the operating system kernel, however they are a useful abstraction for the understanding of processes....
, it is eligible for swapping to disk, but this is transparent in a virtual memory
Virtual memory
In computing, virtual memory is a memory management technique developed for multitasking kernels. This technique virtualizes a computer architecture's various forms of computer data storage , allowing a program to be designed as though there is only one kind of memory, "virtual" memory, which...
system, where blocks of memory values may be really on disk and not in main memory at any time. Note that even unused portions of active processes/tasks (executing programs) are eligible for swapping to disk. All parts of an executing program and its data do not have to be in physical memory for the associated process to be active.
______________________________
*Tasks and processes refer essentially to the same entity. And, although they have somewhat different terminological histories, they have come to be used as synonyms. Today, the term process is generally preferred over task, except when referring to 'multitasking', since the alternative term, 'multiprocessing', is too easy to confuse with multiprocessor (which is a computer with two or more CPUs).
Process states
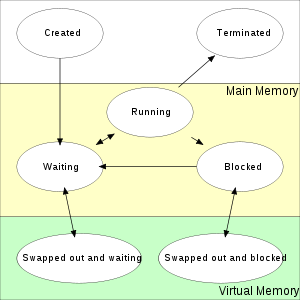
Process states
In a multitasking computer system, processes may occupy a variety of states. These distinct states may not actually be recognized as such by the operating system kernel, however they are a useful abstraction for the understanding of processes....
. Names for these states are not standardised, but they have similar functionality.
- First, the process is "created" - it is loaded from a secondary storage device (hard diskHard diskA hard disk drive is a non-volatile, random access digital magnetic data storage device. It features rotating rigid platters on a motor-driven spindle within a protective enclosure. Data is magnetically read from and written to the platter by read/write heads that float on a film of air above the...
or CD-ROMCD-ROMA CD-ROM is a pre-pressed compact disc that contains data accessible to, but not writable by, a computer for data storage and music playback. The 1985 “Yellow Book” standard developed by Sony and Philips adapted the format to hold any form of binary data....
...) into main memory. After that the process schedulerScheduling (computing)In computer science, a scheduling is the method by which threads, processes or data flows are given access to system resources . This is usually done to load balance a system effectively or achieve a target quality of service...
assigns it the state "waiting".
- While the process is "waiting" it waits for the schedulerScheduling (computing)In computer science, a scheduling is the method by which threads, processes or data flows are given access to system resources . This is usually done to load balance a system effectively or achieve a target quality of service...
to do a so-called context switchContext switchA context switch is the computing process of storing and restoring the state of a CPU so that execution can be resumed from the same point at a later time. This enables multiple processes to share a single CPU. The context switch is an essential feature of a multitasking operating system...
and load the process into the processor. The process state then becomes "running", and the processor executes the process instructions.
- If a process needs to wait for a resource (wait for user input or file to open ...), it is assigned the "blocked" state. The process state is changed back to "waiting" when the process no longer needs to wait.
- Once the process finishes execution, or is terminated by the operating system, it is no longer needed. The process is removed instantly or is moved to the "terminated" state. When removed, it just waits to be removed from main memory.
Inter-process communication
When processes communicate with each other it is called "Inter-process communication" (IPC).Processes frequently need to communicate, for instance in a shell pipeline, the output of the first process need to pass to the second one, and so on to the other process. It is preferred in a well-structured way not using interrupts.
It is even possible for the two processes to be running on different machines. The operating system (OS) may differ from one process to the other, therefore some mediator(s) (called protocols) are needed.
History
By the early 60s computer control software had evolved from Monitor control software, e.g., IBSYSIBSYS
IBSYS was the tape based operating system that IBM supplied with its IBM 7090 and IBM 7094 computers. A similar operating system , also called IBSYS, was provided with IBM 7040 and IBM 7044 computers...
, to Executive control software. Computers got "faster" and computer time
Time-sharing
Time-sharing is the sharing of a computing resource among many users by means of multiprogramming and multi-tasking. Its introduction in the 1960s, and emergence as the prominent model of computing in the 1970s, represents a major technological shift in the history of computing.By allowing a large...
was still neither "cheap" nor fully used. It made multiprogramming
Multiprogramming
Computer multiprogramming is the allocation of a computer system and its resources to more than one concurrent application, job or user ....
possible and necessary.
Multiprogramming means that several programs run "at the same time" (concurrently
Concurrency (computer science)
In computer science, concurrency is a property of systems in which several computations are executing simultaneously, and potentially interacting with each other...
). At first they ran on a single processor (i.e., uniprocessor
Uniprocessor
A uniprocessor system is a computer system with a single central processing unit. As more and more computers employ multiprocessing architectures, such as SMP and MPP, the term is used to refer to systems that still have only one CPU. Most desktop computers are now shipped with multiprocessing...
) and shared scarce resources. Multiprogramming is also basic form of multiprocessing
Multiprocessing
Multiprocessing is the use of two or more central processing units within a single computer system. The term also refers to the ability of a system to support more than one processor and/or the ability to allocate tasks between them...
, a much broader term.
Programs consist of sequence of instruction for processor. A single processor can run only one instruction at a time. Therefore it is impossible to run more programs at the same time. A program might need some resource
Resource (computer science)
A resource, or system resource, is any physical or virtual component of limited availability within a computer system. Every device connected to a computer system is a resource. Every internal system component is a resource...
(input ...) which has a "big" delay. A program might also start some slow operation (output to printer ...). This all leads to processor being "idle" (unused). To use processor at all time the execution of such program was halted. At that point, a second (or nth) program was started or restarted. User perceived that programs run "at the same time" (hence the term, concurrent).
Shortly thereafter, the notion of a 'program' was expanded to the notion of an 'executing program and its context'. The concept of a process was born.
This became necessary with the invention of re-entrant code.
Thread
Thread (computer science)
In computer science, a thread of execution is the smallest unit of processing that can be scheduled by an operating system. The implementation of threads and processes differs from one operating system to another, but in most cases, a thread is contained inside a process...
s came somewhat later. However, with the advent of time-sharing
Time-sharing
Time-sharing is the sharing of a computing resource among many users by means of multiprogramming and multi-tasking. Its introduction in the 1960s, and emergence as the prominent model of computing in the 1970s, represents a major technological shift in the history of computing.By allowing a large...
; computer networks; multiple-CPU, shared memory
Shared memory
In computing, shared memory is memory that may be simultaneously accessed by multiple programs with an intent to provide communication among them or avoid redundant copies. Depending on context, programs may run on a single processor or on multiple separate processors...
computers; etc., the old "multiprogramming" gave way to true multitasking
Computer multitasking
In computing, multitasking is a method where multiple tasks, also known as processes, share common processing resources such as a CPU. In the case of a computer with a single CPU, only one task is said to be running at any point in time, meaning that the CPU is actively executing instructions for...
, multiprocessing and, later, multithreading
Thread (computer science)
In computer science, a thread of execution is the smallest unit of processing that can be scheduled by an operating system. The implementation of threads and processes differs from one operating system to another, but in most cases, a thread is contained inside a process...
.
See also
- Process algebra
- Process calculusProcess calculusIn computer science, the process calculi are a diverse family of related approaches for formally modelling concurrent systems. Process calculi provide a tool for the high-level description of interactions, communications, and synchronizations between a collection of independent agents or processes...
- Child processChild processA child process in computing is a process created by another process .A child process inherits most of its attributes, such as open files, from its parent. In UNIX, a child process is in fact created as a copy of the parent...
- ExitExit (operating system)On many computer operating systems, a computer process terminates its execution by making an exit system call. More generally, an exit in a multithreading environment means that a thread of execution has stopped running. The operating system reclaims resources that were used by the process...
- ForkFork (operating system)In computing, when a process forks, it creates a copy of itself. More generally, a fork in a multithreading environment means that a thread of execution is duplicated, creating a child thread from the parent thread....
- Orphan processOrphan processAn orphan process is a computer process whose parent process has finished or terminated, though itself remains running.In a Unix-like operating system any orphaned process will be immediately adopted by the special init system process. This operation is called re-parenting and occurs automatically...
- Parent processParent processIn computing, a parent process is a process that has created one or more child processes.- Unix :In the operating system Unix, every process except is created when another process executes the fork system call. The process that invoked fork is the parent process and the newly-created process is...
- Process groupProcess groupIn POSIX-conformant operating systems, a process group denotes a collection of one or more processes. Process groups are used to control the distribution of signals. A signal directed to a process group is delivered individually to all of the processes that are members of the group.Process groups...
- Process statesProcess statesIn a multitasking computer system, processes may occupy a variety of states. These distinct states may not actually be recognized as such by the operating system kernel, however they are a useful abstraction for the understanding of processes....
- TaskTask (computers)A task is an execution path through address space. In other words, a set of program instructions that are loaded in memory. The address registers have been loaded with the initial address of the program. At the next clock cycle, the CPU will start execution, in accord with the program. The sense is...
- ThreadThread (computer science)In computer science, a thread of execution is the smallest unit of processing that can be scheduled by an operating system. The implementation of threads and processes differs from one operating system to another, but in most cases, a thread is contained inside a process...
- WaitWait (operating system)In modern computer operating systems, a process may wait on another process to complete its execution. In most systems, a parent process can create an independently executing child process. The parent process may then issue a wait system call, which suspends the execution of the parent process...
- Zombie processZombie processOn Unix and Unix-like computer operating systems, a zombie process or defunct process is a process that has completed execution but still has an entry in the process table. This entry is still needed to allow the process that started the process to read its exit status. The term zombie process...
- Process management (computing)Process management (computing)Process management is an integral part of any modern day operating system . The OS must allocate resources to processes, enable processes to share and exchange information, protect the resources of each process from other processes and enable synchronisation among processes...
- Light-weight processLight-weight processIn computer operating systems, a light-weight process is a means of achieving multitasking. In the traditional meaning of the term, as used in Unix System V and Solaris, an LWP runs in user space on top of a single kernel thread and shares its address space and system resources with other LWPs...