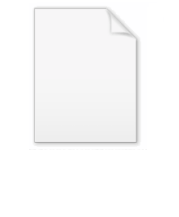
Pseudocode
Encyclopedia
In computer science
and numerical computation, pseudocode is a compact and informal high-level description of the operating principle of a computer program
or other algorithm
. It uses the structural conventions of a programming language
, but is intended for human reading rather than machine reading. Pseudocode typically omits details that are not essential for human understanding of the algorithm, such as variable declarations, system-specific code and some subroutines. The programming language is augmented with natural language
descriptions details, where convenient, or with compact mathematical notation. The purpose of using pseudocode is that it is easier for persons to understand than conventional programming language code, and that it is an efficient and environment-independent description of the key principles of an algorithm. It is commonly used in textbooks and scientific publications that are documenting various algorithms, and also in planning of computer program development, for sketching out the structure of the program before the actual coding takes place.
No standard for pseudocode syntax exists, as a program in pseudocode is not an executable program. Pseudocode resembles, but should not be confused with, skeleton programs including dummy code, which can be compiled
without errors. Flowchart
s and UML
charts can be thought of as a graphical alternative to pseudocode, but are more spacious on paper.
and numerical computation often use pseudocode in description of algorithms, so that all programmers can understand them, even if they do not all know the same programming languages. In textbooks, there is usually an accompanying introduction explaining the particular conventions in use. The level of detail of the pseudo-code may in some cases approach that of formalized general-purpose languages.
A programmer
who needs to implement a specific algorithm, especially an unfamiliar one, will often start with a pseudocode description, and then "translate" that description into the target programming language and modify it to interact correctly with the rest of the program. Programmers may also start a project by sketching out the code in pseudocode on paper before writing it in its actual language, as a top-down structuring approach.
rules of any particular language; there is no systematic standard form, although any particular writer will generally borrow style and syntax for example control structures from some conventional programming language. Popular syntax sources include Pascal, BASIC
, C
, C++
, Java
, Lisp
, and ALGOL
. Variable declarations are typically omitted. Function calls and blocks of code, for example code contained within a loop, is often replaced by a one-line natural language sentence.
Depending on the writer, pseudocode may therefore vary widely in style, from a near-exact imitation of a real programming language at one extreme, to a description approaching formatted prose at the other.
This is an example of pseudocode (for the mathematical game bizz buzz):
For i = 1 to 100
set print_number to true
if i mod 3 = 0
print "Bizz" and set print_number to false
if i mod 5 = 0
print "Buzz" and set print_number to false
if print_number, print i
print a newline
, typically from set
and matrix
theory, mixed with the control structures of a conventional programming language, and perhaps also natural language
descriptions. This is a compact and often informal notation that can be understood by a wide range of mathematically trained people, and is frequently used as a way to describe mathematical algorithm
s. For example, the sum operator (capital-sigma notation) or the product operator (capital-pi notation) may represent a for loop and perhaps a selection structure in one expression:

Normally non-ASCII
typesetting
is used for the mathematical equations, for example by means of TeX
or MathML
markup, or proprietary formula editor
s.
These are examples of articles that contain mathematical style pseudo code:
Mathematical style pseudocode is sometimes referred to as pidgin code, for example pidgin ALGOL
(the origin of the concept), pidgin Fortran
, pidgin BASIC
, pidgin Pascal
, pidgin C
, and pidgin Ada
.
, Lingo, AppleScript
, SQL
, Inform
and to some extent Python
. In these languages, parentheses and other special characters are replaced by prepositions, resulting in quite talkative code. These languages are typically dynamically typed, meaning that variable declarations and other boilerplate code can be omitted. Such languages may make it easier for a person without knowledge about the language to understand the code and perhaps also to learn the language. However, the similarity to natural language is usually more cosmetic than genuine. The syntax rules may be just as strict and formal as in conventional programming, and do not necessarily make development of the programs easier.
Several formal specification language
s include set theory notation using special characters. Examples are:
Some array programming languages include vectorized expressions and matrix operations as non-ASCII formulas, mixed with conventional control structures. Examples are:
Since the usual aim of pseudocode is to present a simple form of some algorithm, you could use a language syntax closer to the problem domain. This would make the expression of ideas in the pseudocode simpler to convey in those domains.
Computer science
Computer science or computing science is the study of the theoretical foundations of information and computation and of practical techniques for their implementation and application in computer systems...
and numerical computation, pseudocode is a compact and informal high-level description of the operating principle of a computer program
Computer program
A computer program is a sequence of instructions written to perform a specified task with a computer. A computer requires programs to function, typically executing the program's instructions in a central processor. The program has an executable form that the computer can use directly to execute...
or other algorithm
Algorithm
In mathematics and computer science, an algorithm is an effective method expressed as a finite list of well-defined instructions for calculating a function. Algorithms are used for calculation, data processing, and automated reasoning...
. It uses the structural conventions of a programming language
Programming language
A programming language is an artificial language designed to communicate instructions to a machine, particularly a computer. Programming languages can be used to create programs that control the behavior of a machine and/or to express algorithms precisely....
, but is intended for human reading rather than machine reading. Pseudocode typically omits details that are not essential for human understanding of the algorithm, such as variable declarations, system-specific code and some subroutines. The programming language is augmented with natural language
Natural language
In the philosophy of language, a natural language is any language which arises in an unpremeditated fashion as the result of the innate facility for language possessed by the human intellect. A natural language is typically used for communication, and may be spoken, signed, or written...
descriptions details, where convenient, or with compact mathematical notation. The purpose of using pseudocode is that it is easier for persons to understand than conventional programming language code, and that it is an efficient and environment-independent description of the key principles of an algorithm. It is commonly used in textbooks and scientific publications that are documenting various algorithms, and also in planning of computer program development, for sketching out the structure of the program before the actual coding takes place.
No standard for pseudocode syntax exists, as a program in pseudocode is not an executable program. Pseudocode resembles, but should not be confused with, skeleton programs including dummy code, which can be compiled
Compiler
A compiler is a computer program that transforms source code written in a programming language into another computer language...
without errors. Flowchart
Flowchart
A flowchart is a type of diagram that represents an algorithm or process, showing the steps as boxes of various kinds, and their order by connecting these with arrows. This diagrammatic representation can give a step-by-step solution to a given problem. Process operations are represented in these...
s and UML
Unified Modeling Language
Unified Modeling Language is a standardized general-purpose modeling language in the field of object-oriented software engineering. The standard is managed, and was created, by the Object Management Group...
charts can be thought of as a graphical alternative to pseudocode, but are more spacious on paper.
Application
Textbooks and scientific publications related to computer scienceComputer science
Computer science or computing science is the study of the theoretical foundations of information and computation and of practical techniques for their implementation and application in computer systems...
and numerical computation often use pseudocode in description of algorithms, so that all programmers can understand them, even if they do not all know the same programming languages. In textbooks, there is usually an accompanying introduction explaining the particular conventions in use. The level of detail of the pseudo-code may in some cases approach that of formalized general-purpose languages.
A programmer
Programmer
A programmer, computer programmer or coder is someone who writes computer software. The term computer programmer can refer to a specialist in one area of computer programming or to a generalist who writes code for many kinds of software. One who practices or professes a formal approach to...
who needs to implement a specific algorithm, especially an unfamiliar one, will often start with a pseudocode description, and then "translate" that description into the target programming language and modify it to interact correctly with the rest of the program. Programmers may also start a project by sketching out the code in pseudocode on paper before writing it in its actual language, as a top-down structuring approach.
Syntax
As the name suggests, pseudocode generally does not actually obey the syntaxSyntax
In linguistics, syntax is the study of the principles and rules for constructing phrases and sentences in natural languages....
rules of any particular language; there is no systematic standard form, although any particular writer will generally borrow style and syntax for example control structures from some conventional programming language. Popular syntax sources include Pascal, BASIC
BASIC
BASIC is a family of general-purpose, high-level programming languages whose design philosophy emphasizes ease of use - the name is an acronym from Beginner's All-purpose Symbolic Instruction Code....
, C
C (programming language)
C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system....
, C++
C++
C++ is a statically typed, free-form, multi-paradigm, compiled, general-purpose programming language. It is regarded as an intermediate-level language, as it comprises a combination of both high-level and low-level language features. It was developed by Bjarne Stroustrup starting in 1979 at Bell...
, Java
Java (programming language)
Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities...
, Lisp
Lisp programming language
Lisp is a family of computer programming languages with a long history and a distinctive, fully parenthesized syntax. Originally specified in 1958, Lisp is the second-oldest high-level programming language in widespread use today; only Fortran is older...
, and ALGOL
ALGOL
ALGOL is a family of imperative computer programming languages originally developed in the mid 1950s which greatly influenced many other languages and became the de facto way algorithms were described in textbooks and academic works for almost the next 30 years...
. Variable declarations are typically omitted. Function calls and blocks of code, for example code contained within a loop, is often replaced by a one-line natural language sentence.
Depending on the writer, pseudocode may therefore vary widely in style, from a near-exact imitation of a real programming language at one extreme, to a description approaching formatted prose at the other.
This is an example of pseudocode (for the mathematical game bizz buzz):
For i = 1 to 100
set print_number to true
if i mod 3 = 0
print "Bizz" and set print_number to false
if i mod 5 = 0
print "Buzz" and set print_number to false
if print_number, print i
print a newline
Mathematical style pseudocode
In numerical computation, pseudocode often consists of mathematical notationMathematical notation
Mathematical notation is a system of symbolic representations of mathematical objects and ideas. Mathematical notations are used in mathematics, the physical sciences, engineering, and economics...
, typically from set
Set theory
Set theory is the branch of mathematics that studies sets, which are collections of objects. Although any type of object can be collected into a set, set theory is applied most often to objects that are relevant to mathematics...
and matrix
Matrix (mathematics)
In mathematics, a matrix is a rectangular array of numbers, symbols, or expressions. The individual items in a matrix are called its elements or entries. An example of a matrix with six elements isMatrices of the same size can be added or subtracted element by element...
theory, mixed with the control structures of a conventional programming language, and perhaps also natural language
Natural language
In the philosophy of language, a natural language is any language which arises in an unpremeditated fashion as the result of the innate facility for language possessed by the human intellect. A natural language is typically used for communication, and may be spoken, signed, or written...
descriptions. This is a compact and often informal notation that can be understood by a wide range of mathematically trained people, and is frequently used as a way to describe mathematical algorithm
Algorithm
In mathematics and computer science, an algorithm is an effective method expressed as a finite list of well-defined instructions for calculating a function. Algorithms are used for calculation, data processing, and automated reasoning...
s. For example, the sum operator (capital-sigma notation) or the product operator (capital-pi notation) may represent a for loop and perhaps a selection structure in one expression:
Return

Normally non-ASCII
ASCII
The American Standard Code for Information Interchange is a character-encoding scheme based on the ordering of the English alphabet. ASCII codes represent text in computers, communications equipment, and other devices that use text...
typesetting
Typesetting
Typesetting is the composition of text by means of types.Typesetting requires the prior process of designing a font and storing it in some manner...
is used for the mathematical equations, for example by means of TeX
TeX
TeX is a typesetting system designed and mostly written by Donald Knuth and released in 1978. Within the typesetting system, its name is formatted as ....
or MathML
MathML
Mathematical Markup Language is an application of XML for describing mathematical notations and capturing both its structure and content. It aims at integrating mathematical formulae into World Wide Web pages and other documents...
markup, or proprietary formula editor
Formula editor
A formula editor is a name for a computer program that is used to typeset mathematical works or formulae.Formula editors typically serve two purposes:...
s.
These are examples of articles that contain mathematical style pseudo code:
|
|
Mathematical style pseudocode is sometimes referred to as pidgin code, for example pidgin ALGOL
ALGOL
ALGOL is a family of imperative computer programming languages originally developed in the mid 1950s which greatly influenced many other languages and became the de facto way algorithms were described in textbooks and academic works for almost the next 30 years...
(the origin of the concept), pidgin Fortran
Fortran
Fortran is a general-purpose, procedural, imperative programming language that is especially suited to numeric computation and scientific computing...
, pidgin BASIC
BASIC
BASIC is a family of general-purpose, high-level programming languages whose design philosophy emphasizes ease of use - the name is an acronym from Beginner's All-purpose Symbolic Instruction Code....
, pidgin Pascal
Pascal (programming language)
Pascal is an influential imperative and procedural programming language, designed in 1968/9 and published in 1970 by Niklaus Wirth as a small and efficient language intended to encourage good programming practices using structured programming and data structuring.A derivative known as Object Pascal...
, pidgin C
C (programming language)
C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system....
, and pidgin Ada
Ada (programming language)
Ada is a structured, statically typed, imperative, wide-spectrum, and object-oriented high-level computer programming language, extended from Pascal and other languages...
.
Natural language grammar in programming languages
Various attempts to bring elements of natural language grammar into computer programming have produced programming languages such as HyperTalkHyperTalk
HyperTalk is a high-level, procedural programming language created in 1987 by Dan Winkler and used in conjunction with Apple Computer's HyperCard hypermedia program by Bill Atkinson. The main target audience of HyperTalk was beginning programmers, hence HyperTalk programmers were usually called...
, Lingo, AppleScript
AppleScript
AppleScript is a scripting language created by Apple Inc. and built into Macintosh operating systems since System 7. The term "AppleScript" may refer to the scripting system itself, or to particular scripts that are written in the AppleScript language....
, SQL
SQL
SQL is a programming language designed for managing data in relational database management systems ....
, Inform
Inform
Over the following decade, version 6 became reasonably stable and a popular language for writing interactive fiction. In 2006, Nelson released Inform 7 , a completely new language based on principles of natural language and a new set of tools based around a book-publishing metaphor.- Z-Machine and...
and to some extent Python
Python (programming language)
Python is a general-purpose, high-level programming language whose design philosophy emphasizes code readability. Python claims to "[combine] remarkable power with very clear syntax", and its standard library is large and comprehensive...
. In these languages, parentheses and other special characters are replaced by prepositions, resulting in quite talkative code. These languages are typically dynamically typed, meaning that variable declarations and other boilerplate code can be omitted. Such languages may make it easier for a person without knowledge about the language to understand the code and perhaps also to learn the language. However, the similarity to natural language is usually more cosmetic than genuine. The syntax rules may be just as strict and formal as in conventional programming, and do not necessarily make development of the programs easier.
Mathematical programming languages
An alternative to using mathematical pseudocode (involving set theory notation or matrix operations) for documentation of algorithms is to use a formal mathematical programming language that is a mix of non-ASCII mathematical notation and program control structures. Then the code can be parsed and interpreted by a machine.Several formal specification language
Specification language
A specification language is a formal language used in computer science.Unlike most programming languages, which are directly executable formal languages used to implement a system, specification languages are used during systems analysis, requirements analysis and systems design.Specification...
s include set theory notation using special characters. Examples are:
- Z notationZ notationThe Z notation , named after Zermelo–Fraenkel set theory, is a formal specification language used for describing and modelling computing systems. It is targeted at the clear specification of computer programs and computer-based systems in general.-History:...
- Vienna Development MethodVienna Development MethodThe Vienna Development Method is one of the longest-established Formal Methods for the development of computer-based systems. Originating in work done at IBM's Vienna Laboratory in the 1970s, it has grown to include a group of techniques and tools based on a formal specification language - the VDM...
Specification Language (VDM-SL).
Some array programming languages include vectorized expressions and matrix operations as non-ASCII formulas, mixed with conventional control structures. Examples are:
- A programming language (APL), and its dialects APLXAPLXAPLX is a modern, second generation, cross-platform dialect of the APL programming language. APLX is targeted at applications such as financial planning, market research, statistics, management information, and various kinds of scientific and engineering work. APLX is based on IBM's APL2, but...
and A+A+ (programming language)A+ is an array programming language descendent from the programming language A, which in turn was created to replace APL in 1988. Arthur Whitney developed the "A" portion of A+, while other developers at Morgan Stanley extended it, adding a graphical user interface and other language features...
. - MathCADMathCadMathcad is computer software primarily intended for the verification, validation, documentation and re-use of engineering calculations. First introduced in 1986 on DOS, it was the first to introduce live editing of typeset mathematical notation, combined with its automatic computations...
.
Alternative forms of pseudocode
Since the usual aim of pseudocode is to present a simple form of some algorithm, you could use a language syntax closer to the problem domain. This would make the expression of ideas in the pseudocode simpler to convey in those domains.
See also
- Concept programmingConcept programmingConcept programming is a programming paradigm focusing on how concepts, that live in the programmer's head, translate into representations that are found in the code space. This approach was introduced in 2001 by Christophe de Dinechin with the XL Programming Language.- Pseudo-metrics :Concept...
- Dummy code
- Literate programmingLiterate programmingLiterate programming is an approach to programming introduced by Donald Knuth as an alternative to the structured programming paradigm of the 1970s....
- Pidgin code
- Program Design LanguageProgram Design LanguageProgram Design Language is a method for designing and documenting methods and procedures in software. It is related to pseudocode, but unlike pseudocode, it is written in plain language without any terms that could suggest the use of any programming language or library.PDL was originally developed...
(PDL) - Short CodeShort codeShort codes are special telephone numbers, significantly shorter than full telephone numbers, that can be used to address SMS and MMS messages from certain service provider's mobile phones or fixed phones...
- Skeleton program
- Structured EnglishStructured EnglishStructured English is the use of the English language with the syntax of structured programming. Thus structured English aims at getting the benefits of both the programming logic and natural language...
External links
- A pseudocode standard
- http://calgo.acm.org/Collected Algorithms of the ACMAssociation for Computing MachineryThe Association for Computing Machinery is a learned society for computing. It was founded in 1947 as the world's first scientific and educational computing society. Its membership is more than 92,000 as of 2009...
] - Pseudocode Guidelines, PDF file.
- Pseudocode Programming Process base on data from Code Complete book
- Pseudocode generation tool from a model tree learn how to generate pseudocode in a second