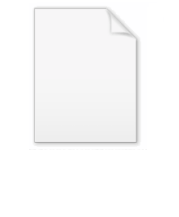
C standard library
Encyclopedia
The C Standard Library is the standard library
for the programming language C
, as specified in the ANSI C
standard.. It was developed at the same time as the C POSIX library
, which is basically a superset of it. Since ANSI C was adopted by the International Organization for Standardization
, the C standard library is also called the ISO C library.
Informally, the terms C standard library or C library or libc are also used to designate a particular implementation on a given system. In the Unix
environment, such an implementation is usually shipped with the operating system and its presence is assumed by many applications. For instance, GNU/Linux comes with the GNU
implementation glibc.
The C standard library provides macros, type definitions, and functions for tasks like string handling, mathematical computations, input/output processing, memory allocation and several other operating system
services.
(API) of the C standard library is declared in a number of header file
s. Each header file contains one or more function declarations, data type definitions and macros.
After a long period of stability,
three new header files (
, a revision to the C Standard published in 1999. In total, there are now 24 header files:
POSIX
standardized the use of several nonstandard C headers for Unix-specific functionality. Many have found their way to other architectures. Examples include
).
systems typically have a C library in shared library form, but the header files (and compiler toolchain) may be absent from an installation so C development may not be possible. The C library is considered part of the operating system on Unix-like systems. The C functions, including the ISO C standard ones, are widely used by programs, and are regarded as if they were not only an implementation of something in the C language, but also de facto part of the operating system interface. Unix-like operating systems generally cannot function if the C library is erased.
By contrast, on Microsoft Windows, the core system dynamic libraries (DLLs
) do not provide an implementation of the C standard library; this is provided by each compiler individually. Compiled applications written in C are either statically linked with a C library, or linked to a dynamic version of the library that is shipped with these applications, rather than relied upon to be present on the targeted systems. Functions in a compiler's C library are not regarded as interfaces to Microsoft Windows.
Many other implementations exist, provided with both various operating systems and C compilers.
Although there exist too many implementations to list, some popular implementations follow:
) provide built-in versions of many of the functions in the C standard library; that is, the implementations of the functions are written into the compiled object file
, and the program calls the built-in versions instead of the functions in the C library shared object file. This reduces function call overhead, especially if function calls are replaced with inline
variants, and allows other forms of optimization
(as the compiler knows the control-flow
characteristics of the built-in variants), but may cause confusion when debugging (for example, the built-in versions cannot be replaced with instrumented
variants).
However, the built-in functions must behave like ordinary functions in accordance with ISO C. The main implication is that the program must be able to create a pointer to these functions by taking their address, and invoke the function by means of that pointer. If two pointers to the same function are derived in two different translation unit in the program, these two pointers must compare equal; that is, the address comes by resolving the name of the function, which has external (program-wide) linkage.
vulnerabilities and generally encouraging buggy programming ever since their adoption. The most criticized items are:
Except the extreme case with
book by B. Kernighan and R. Pike where the authors commonly use wrappers that print error messages and quit the program if an error occurs.
s.
The current (version 2.8) situation under glibc is messy. Most (but not all)
functions raise exceptions on errors. Some also set errno. A few functions set
errno, but don't raise an exception. A very few functions do neither.
provided no built-in functions such as I/O operations, unlike traditional languages such as COBOL
and Fortran
. Over time, user communities of C, shared ideas and implementations, of what is now called C standard libraries. Many of these ideas were incorporated eventually into the definition of the standardized C language.
Both Unix
and C were created at AT&T's Bell Laboratories
in the late 1960s and early 1970s. During the 1970s the C language became increasingly popular. Many universities and organizations began creating their own variants of the language for their own projects. By the beginning of the 1980s compatibility problems between the various C implementations became apparent. In 1983 the American National Standards Institute
(ANSI) formed a committee to establish a standard specification of C known as "ANSI C
". This work culminated in the creation of the so-called C89 standard in 1989. Part of the resulting standard was a set of software libraries called the ANSI C standard library.
(and SUS
) specifies a number of routines that should be available over and above those in the C standard library proper; these are often implemented alongside the C standard library functionality, with varying degrees of closeness. For example, glibc implements functions such as fork
within
C library.
language, for example, includes the functionality of the C standard library in the namespace
and the main implementation of Python
known as CPython
. In the latter, for example, the built-in file objects are defined as "implemented using C's
), the standard library is minuscule. The library provides a basic set of mathematical functions, string manipulation, type conversions, and file and console-based I/O. It does not include a standard set of "container types
" like the C++
Standard Template Library
, let alone the complete graphical user interface
(GUI) toolkits, networking tools, and profusion of other functionality that Java provides as standard. The main advantage of the small standard library is that providing a working ISO C environment is much easier than it is with other languages, and consequently porting C to a new platform is relatively easy.
Standard library
A standard library for a programming language is the library that is conventionally made available in every implementation of that language. In some cases, the library is described directly in the programming language specification; in other cases, the contents of the standard library are...
for the programming language C
C (programming language)
C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system....
, as specified in the ANSI C
ANSI C
ANSI C refers to the family of successive standards published by the American National Standards Institute for the C programming language. Software developers writing in C are encouraged to conform to the standards, as doing so aids portability between compilers.-History and outlook:The first...
standard.. It was developed at the same time as the C POSIX library
C POSIX library
The C POSIX library is a specification of a C standard library for POSIX systems. It was developed at the same time as the ANSI C standard. Some effort was made to make POSIX compatible with standard C; POSIX includes additional functions to those introduced in standard C.- C POSIX library header...
, which is basically a superset of it. Since ANSI C was adopted by the International Organization for Standardization
International Organization for Standardization
The International Organization for Standardization , widely known as ISO, is an international standard-setting body composed of representatives from various national standards organizations. Founded on February 23, 1947, the organization promulgates worldwide proprietary, industrial and commercial...
, the C standard library is also called the ISO C library.
Informally, the terms C standard library or C library or libc are also used to designate a particular implementation on a given system. In the Unix
Unix
Unix is a multitasking, multi-user computer operating system originally developed in 1969 by a group of AT&T employees at Bell Labs, including Ken Thompson, Dennis Ritchie, Brian Kernighan, Douglas McIlroy, and Joe Ossanna...
environment, such an implementation is usually shipped with the operating system and its presence is assumed by many applications. For instance, GNU/Linux comes with the GNU
GNU
GNU is a Unix-like computer operating system developed by the GNU project, ultimately aiming to be a "complete Unix-compatible software system"...
implementation glibc.
The C standard library provides macros, type definitions, and functions for tasks like string handling, mathematical computations, input/output processing, memory allocation and several other operating system
Operating system
An operating system is a set of programs that manage computer hardware resources and provide common services for application software. The operating system is the most important type of system software in a computer system...
services.
Header files
The application programming interfaceApplication programming interface
An application programming interface is a source code based specification intended to be used as an interface by software components to communicate with each other...
(API) of the C standard library is declared in a number of header file
Header file
Some programming languages use header files. These files allow programmers to separate certain elements of a program's source code into reusable files. Header files commonly contain forward declarations of classes, subroutines, variables, and other identifiers...
s. Each header file contains one or more function declarations, data type definitions and macros.
After a long period of stability,
three new header files (
iso646.hIso646.hThe iso646.h header file is part of the C standard library. It was added to this library in a 1995 amendment to the C90 standard. It defines a number of macros which allow programmers to use C language bitwise and logical operators, which, without the header file, cannot be quickly or easily typed...
, wchar.h
, and wctype.h
) were added with Normative Addendum 1 (NA1), an addition to the C Standard ratified in 1995. Six more header files (complex.h
, fenv.h
, inttypes.h
, stdbool.h
, stdint.h
, and tgmath.h
) were added with C99C99
C99 is a modern dialect of the C programming language. It extends the previous version with new linguistic and library features, and helps implementations make better use of available computer hardware and compiler technology.-History:...
, a revision to the C Standard published in 1999. In total, there are now 24 header files:
Name | From | Description |
---|---|---|
|
Contains the assert Assertion (computing) In computer programming, an assertion is a predicate placed in a program to indicate that the developer thinks that the predicate is always true at that place.For example, the following code contains two assertions:... macro, used to assist with detecting logical errors and other types of bug in debugging versions of a program. |
|
|
C99 | A set of functions for manipulating complex number Complex number A complex number is a number consisting of a real part and an imaginary part. Complex numbers extend the idea of the one-dimensional number line to the two-dimensional complex plane by using the number line for the real part and adding a vertical axis to plot the imaginary part... s. |
|
Defines set of functions C character classification C character classification is an operation provided by a group of functions in the ANSI C Standard Library for the C programming language. These functions are used to test characters for membership in a particular class of characters, such as alphabetic characters, control characters, etc... used to classify characters by their types or to convert between upper and lower case in a way that is independent of the used character set (typically ASCII ASCII The American Standard Code for Information Interchange is a character-encoding scheme based on the ordering of the English alphabet. ASCII codes represent text in computers, communications equipment, and other devices that use text... or one of its extensions, although implementations utilizing EBCDIC EBCDIC Extended Binary Coded Decimal Interchange Code is an 8-bit character encoding used mainly on IBM mainframe and IBM midrange computer operating systems.... are also known). |
|
|
For testing error codes reported by library functions. | |
|
C99 | Defines a set of functions for controlling floating-point environment. |
|
Defines macro constants specifying the implementation-specific properties of the floating-point library. | |
|
C99 | Defines exact width integer types. |
|
NA1 | For programming in ISO 646 variant character sets. |
|
Defines macro constants specifying the implementation-specific properties of the integer types. | |
|
Defines localization functions. | |
|
Defines common mathematical functions. | |
|
Declares the macros setjmp and longjmp , which are used for non-local exits. |
|
|
For controlling various exceptional conditions. | |
|
For accessing a varying number of arguments passed to functions. | |
|
C99 | Defines a boolean data type. |
|
C99 | Defines exact width integer types. |
|
Defines several useful types and macros. | |
|
Defines core input and output functions C file input/output The C programming language provides many standard library functions for file input and output. These functions make up the bulk of the C standard library header... |
|
|
Defines numeric conversion functions, pseudo-random numbers generation functions, memory allocation, process control functions C process control C process control refers to a group of functions in the standard library of the C programming language implementing basic process control operations... |
|
|
Defines string handling functions C string handling C string handling refers to a group of functions implementing operations on strings in the C Standard Library. Various operations, such as copying, concatenation, tokenization and searching are supported.... . |
|
|
C99 | Defines type-generic mathematical functions. |
|
Defines date and time handling functions | |
|
NA1 | Defines wide string handling functions. |
|
NA1 | Defines set of functions C character classification C character classification is an operation provided by a group of functions in the ANSI C Standard Library for the C programming language. These functions are used to test characters for membership in a particular class of characters, such as alphabetic characters, control characters, etc... used to classify wide characters by their types or to convert between upper and lower case |
POSIX
POSIX
POSIX , an acronym for "Portable Operating System Interface", is a family of standards specified by the IEEE for maintaining compatibility between operating systems...
standardized the use of several nonstandard C headers for Unix-specific functionality. Many have found their way to other architectures. Examples include
unistd.h
and signal.h
. A number of other groups are using other nonstandard headers - most flavors of Linux have alloca.h
and HP OpenVMS has the va_count
function.Documentation
On Unix-like systems, the authorative documentation of the actually implemented API is provided in form of man pages. On most systems, man pages on standard library functions are in section 3; section 7 may contain some more generic pages on underlying concepts (e.g.man 7 math_error
in LinuxLinux
Linux is a Unix-like computer operating system assembled under the model of free and open source software development and distribution. The defining component of any Linux system is the Linux kernel, an operating system kernel first released October 5, 1991 by Linus Torvalds...
).
Implementations
Unix-likeUnix-like
A Unix-like operating system is one that behaves in a manner similar to a Unix system, while not necessarily conforming to or being certified to any version of the Single UNIX Specification....
systems typically have a C library in shared library form, but the header files (and compiler toolchain) may be absent from an installation so C development may not be possible. The C library is considered part of the operating system on Unix-like systems. The C functions, including the ISO C standard ones, are widely used by programs, and are regarded as if they were not only an implementation of something in the C language, but also de facto part of the operating system interface. Unix-like operating systems generally cannot function if the C library is erased.
By contrast, on Microsoft Windows, the core system dynamic libraries (DLLs
Dynamic-link library
Dynamic-link library , or DLL, is Microsoft's implementation of the shared library concept in the Microsoft Windows and OS/2 operating systems...
) do not provide an implementation of the C standard library; this is provided by each compiler individually. Compiled applications written in C are either statically linked with a C library, or linked to a dynamic version of the library that is shipped with these applications, rather than relied upon to be present on the targeted systems. Functions in a compiler's C library are not regarded as interfaces to Microsoft Windows.
Many other implementations exist, provided with both various operating systems and C compilers.
Although there exist too many implementations to list, some popular implementations follow:
- BSD libc, implementations distributed under BSDBerkeley Software DistributionBerkeley Software Distribution is a Unix operating system derivative developed and distributed by the Computer Systems Research Group of the University of California, Berkeley, from 1977 to 1995...
operating systems. - GNU C LibraryGNU C LibraryThe GNU C Library, commonly known as glibc, is the C standard library released by the GNU Project. Originally written by the Free Software Foundation for the GNU operating system, the library's development has been overseen by a committee since 2001, with Ulrich Drepper from Red Hat as the lead...
, used in GNUGNUGNU is a Unix-like computer operating system developed by the GNU project, ultimately aiming to be a "complete Unix-compatible software system"...
/LinuxLinuxLinux is a Unix-like computer operating system assembled under the model of free and open source software development and distribution. The defining component of any Linux system is the Linux kernel, an operating system kernel first released October 5, 1991 by Linus Torvalds...
and GNUGNUGNU is a Unix-like computer operating system developed by the GNU project, ultimately aiming to be a "complete Unix-compatible software system"...
/HURD. - Microsoft C Run-time Library, part of Microsoft Visual C++
- dietlibcDietlibcdietlibc is a C standard library released under the GNU General Public License Version 2. It was developed by Felix von Leitner with the goal to compile and link programs to the smallest possible size. dietlibc was developed from scratch and thus only implements the most important and commonly used...
, an alternative small implementation of the C standard library (MMU-less) - uClibcUClibcIn computing, uClibc is a small C standard library intended for embedded Linux systems. uClibc was created to support uClinux, a version of Linux not requiring a memory management unit and thus suited for microcontrollers .The project lead is Erik Andersen. The other main contributor is Manuel...
, a C standard library for embedded LinuxLinuxLinux is a Unix-like computer operating system assembled under the model of free and open source software development and distribution. The defining component of any Linux system is the Linux kernel, an operating system kernel first released October 5, 1991 by Linus Torvalds...
systems (MMU-less) - NewlibNewlibNewlib is a C standard library implementation intended for use on embedded systems. It is a conglomeration of several library parts, all under free software licenses that make them easily usable on embedded products....
, a C standard library for embedded systems (MMU-less) - klibcKlibcIn computing, klibc is a minimalistic subset of the standard C library developed by H. Peter Anvin. It was developed mainly to be used during the Linux startup process, and it is part of the early user space, i.e. components used during kernel startup, but which do not run in kernel mode...
, primarily for booting Linux systems. - EGLIBC, variant of glibc for embedded systems.
- musl, another lightweight C standard library implementation for Linux systems
Compiler built-in functions
Some compilers (for example, GCCGNU Compiler Collection
The GNU Compiler Collection is a compiler system produced by the GNU Project supporting various programming languages. GCC is a key component of the GNU toolchain...
) provide built-in versions of many of the functions in the C standard library; that is, the implementations of the functions are written into the compiled object file
Object file
An object file is a file containing relocatable format machine code that is usually not directly executable. Object files are produced by an assembler, compiler, or other language translator, and used as input to the linker....
, and the program calls the built-in versions instead of the functions in the C library shared object file. This reduces function call overhead, especially if function calls are replaced with inline
Inline function
In various versions of the C and C++ programming languages, an inline function is a function upon which the compiler has been requested to perform inline expansion...
variants, and allows other forms of optimization
Compiler optimization
Compiler optimization is the process of tuning the output of a compiler to minimize or maximize some attributes of an executable computer program. The most common requirement is to minimize the time taken to execute a program; a less common one is to minimize the amount of memory occupied...
(as the compiler knows the control-flow
Control flow
In computer science, control flow refers to the order in which the individual statements, instructions, or function calls of an imperative or a declarative program are executed or evaluated....
characteristics of the built-in variants), but may cause confusion when debugging (for example, the built-in versions cannot be replaced with instrumented
Instrumentation (computer programming)
In context of computer programming, instrumentation refers to an ability to monitor or measure the level of a product's performance, to diagnose errors and to write trace information. Programmers implement instrumentation in the form of code instructions that monitor specific components in a system...
variants).
However, the built-in functions must behave like ordinary functions in accordance with ISO C. The main implication is that the program must be able to create a pointer to these functions by taking their address, and invoke the function by means of that pointer. If two pointers to the same function are derived in two different translation unit in the program, these two pointers must compare equal; that is, the address comes by resolving the name of the function, which has external (program-wide) linkage.
Linking, libm
Under Linux, the mathematical functions (as declared inmath.h
) are bundled separately in the mathematical library libm. If any of them is used, the linker must be given the directive -lm
.Detection
According to the C standard the macro__STDC_HOSTED__
shall be defined to 1 if the implementation is hosted. A hosted implementation has all the headers specified by the C standard. An implementation can also be freestanding which means that these headers will not be present. If an implementation is freestanding, it shall define __STDC_HOSTED__
to 0.Buffer overflow vulnerabilities
A number of functions in the C standard library have been notorious for having buffer overflowBuffer overflow
In computer security and programming, a buffer overflow, or buffer overrun, is an anomaly where a program, while writing data to a buffer, overruns the buffer's boundary and overwrites adjacent memory. This is a special case of violation of memory safety....
vulnerabilities and generally encouraging buggy programming ever since their adoption. The most criticized items are:
- string-manipulation routines (
strcpy, strcat
, ...) - for lack of bounds checking and possible buffer overflows if the bounds aren't checked manually; there are now alternative routines (strncpy, strncat
, ...) - string routines in general - for side-effectsSide effect (computer science)In computer science, a function or expression is said to have a side effect if, in addition to returning a value, it also modifies some state or has an observable interaction with calling functions or the outside world...
, encouraging irresponsible buffer usage, not always guaranteeing output validity (i.e. null-termination), linear length calculation; -
printf
family routines - for spoiling the execution stackCall stackIn computer science, a call stack is a stack data structure that stores information about the active subroutines of a computer program. This kind of stack is also known as an execution stack, control stack, run-time stack, or machine stack, and is often shortened to just "the stack"...
when the format string doesn't match the arguments given. This fundamental flaw created an entire class of attacks: format string attackFormat string attackUncontrolled format string is a type of software vulnerability, discovered around 1999, that can be used in security exploits. Previously thought harmless, format string exploits can be used to crash a program or to execute harmful code...
s; - some I/O routines (
gets, scanf
) - for lack of (either any or easy) input length checking;
Except the extreme case with
gets
, all the security vulnerabilities can be avoided by introducing auxiliary code to perform memory management, bounds checking, input checking etc. This is often done in form of wrappers that make standard library functions safer and easier to use. This dates back to as early as The Practice of ProgrammingThe Practice of Programming
The Practice of Programming by Brian W. Kernighan and Rob Pike is a 1999 book about computer programming and software engineering, published by Addison-Wesley....
book by B. Kernighan and R. Pike where the authors commonly use wrappers that print error messages and quit the program if an error occurs.
Threading problems, vulnerability to race conditions
Some routines (mktemp, strerror
) are critized for being thread unsafe and otherwise vulnerable to race conditionRace condition
A race condition or race hazard is a flaw in an electronic system or process whereby the output or result of the process is unexpectedly and critically dependent on the sequence or timing of other events...
s.
Error handling
The Linux manual pagemath_error
says:The current (version 2.8) situation under glibc is messy. Most (but not all)
functions raise exceptions on errors. Some also set errno. A few functions set
errno, but don't raise an exception. A very few functions do neither.
Standardization
The original C languageC (programming language)
C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system....
provided no built-in functions such as I/O operations, unlike traditional languages such as COBOL
COBOL
COBOL is one of the oldest programming languages. Its name is an acronym for COmmon Business-Oriented Language, defining its primary domain in business, finance, and administrative systems for companies and governments....
and Fortran
Fortran
Fortran is a general-purpose, procedural, imperative programming language that is especially suited to numeric computation and scientific computing...
. Over time, user communities of C, shared ideas and implementations, of what is now called C standard libraries. Many of these ideas were incorporated eventually into the definition of the standardized C language.
Both Unix
Unix
Unix is a multitasking, multi-user computer operating system originally developed in 1969 by a group of AT&T employees at Bell Labs, including Ken Thompson, Dennis Ritchie, Brian Kernighan, Douglas McIlroy, and Joe Ossanna...
and C were created at AT&T's Bell Laboratories
Bell Labs
Bell Laboratories is the research and development subsidiary of the French-owned Alcatel-Lucent and previously of the American Telephone & Telegraph Company , half-owned through its Western Electric manufacturing subsidiary.Bell Laboratories operates its...
in the late 1960s and early 1970s. During the 1970s the C language became increasingly popular. Many universities and organizations began creating their own variants of the language for their own projects. By the beginning of the 1980s compatibility problems between the various C implementations became apparent. In 1983 the American National Standards Institute
American National Standards Institute
The American National Standards Institute is a private non-profit organization that oversees the development of voluntary consensus standards for products, services, processes, systems, and personnel in the United States. The organization also coordinates U.S. standards with international...
(ANSI) formed a committee to establish a standard specification of C known as "ANSI C
ANSI C
ANSI C refers to the family of successive standards published by the American National Standards Institute for the C programming language. Software developers writing in C are encouraged to conform to the standards, as doing so aids portability between compilers.-History and outlook:The first...
". This work culminated in the creation of the so-called C89 standard in 1989. Part of the resulting standard was a set of software libraries called the ANSI C standard library.
POSIX standard library
POSIXPOSIX
POSIX , an acronym for "Portable Operating System Interface", is a family of standards specified by the IEEE for maintaining compatibility between operating systems...
(and SUS
Single UNIX Specification
The Single UNIX Specification is the collective name of a family of standards for computer operating systems to qualify for the name "Unix"...
) specifies a number of routines that should be available over and above those in the C standard library proper; these are often implemented alongside the C standard library functionality, with varying degrees of closeness. For example, glibc implements functions such as fork
Fork (operating system)
In computing, when a process forks, it creates a copy of itself. More generally, a fork in a multithreading environment means that a thread of execution is duplicated, creating a child thread from the parent thread....
within
libc.so
, but before NPTL was merged into glibc it constituted a separate library with its own linker flag argument. Often, this POSIX-specified functionality will be regarded as part of the library; the C library proper may be identified as the ANSI or ISOInternational Organization for Standardization
The International Organization for Standardization , widely known as ISO, is an international standard-setting body composed of representatives from various national standards organizations. Founded on February 23, 1947, the organization promulgates worldwide proprietary, industrial and commercial...
C library.
Ongoing work
The ISO C committee published Technical reports TR 24731-1 and is working on TR 24731-2 to propose adoption of some functions with bounds checking and automatic buffer allocation, correspondingly. The former has met severe criticism with some praise, the latter received mixed responses. Despite this, TR 24731-1 has been implemented into Microsoft's C standard library and its compiler issues warnings when using old 'insecure' functions.The C standard library in other languages
Some languages include the functionality of the standard C library in their own libraries. The library may be adapted to better suit the language's structure, but the operation semantics are kept similar. The C++C++
C++ is a statically typed, free-form, multi-paradigm, compiled, general-purpose programming language. It is regarded as an intermediate-level language, as it comprises a combination of both high-level and low-level language features. It was developed by Bjarne Stroustrup starting in 1979 at Bell...
language, for example, includes the functionality of the C standard library in the namespace
std
(e.g., std::printf
, std::atoi
, std::feof
), in header files with similar names to the C ones (cstdio
, cmath
, cstdlib
, etc.). Other languages that take similar approaches are DD (programming language)
The D programming language is an object-oriented, imperative, multi-paradigm, system programming language created by Walter Bright of Digital Mars. It originated as a re-engineering of C++, but even though it is mainly influenced by that language, it is not a variant of C++...
and the main implementation of Python
Python (programming language)
Python is a general-purpose, high-level programming language whose design philosophy emphasizes code readability. Python claims to "[combine] remarkable power with very clear syntax", and its standard library is large and comprehensive...
known as CPython
CPython
CPython is the default, most-widely used implementation of the Python programming language. It is written in C. In addition to CPython, there are two other production-quality Python implementations: Jython, written in Java, and IronPython, which is written for the Common Language Runtime. There...
. In the latter, for example, the built-in file objects are defined as "implemented using C's
stdio
package", so that the available operations (open, read, write, etc.) are expected to have the same behavior as the corresponding C functions.Comparison to standard libraries of other languages
Compared to some other languages (for example JavaJava (programming language)
Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities...
), the standard library is minuscule. The library provides a basic set of mathematical functions, string manipulation, type conversions, and file and console-based I/O. It does not include a standard set of "container types
Container (data structure)
In computer science, a container is a class, a data structure, or an abstract data type whose instances are collections of other objects. In other words; they are used for storing objects in an organized way following specific access rules...
" like the C++
C++
C++ is a statically typed, free-form, multi-paradigm, compiled, general-purpose programming language. It is regarded as an intermediate-level language, as it comprises a combination of both high-level and low-level language features. It was developed by Bjarne Stroustrup starting in 1979 at Bell...
Standard Template Library
Standard Template Library
The Standard Template Library is a C++ software library which later evolved into the C++ Standard Library. It provides four components called algorithms, containers, functors, and iterators. More specifically, the C++ Standard Library is based on the STL published by SGI. Both include some...
, let alone the complete graphical user interface
Graphical user interface
In computing, a graphical user interface is a type of user interface that allows users to interact with electronic devices with images rather than text commands. GUIs can be used in computers, hand-held devices such as MP3 players, portable media players or gaming devices, household appliances and...
(GUI) toolkits, networking tools, and profusion of other functionality that Java provides as standard. The main advantage of the small standard library is that providing a working ISO C environment is much easier than it is with other languages, and consequently porting C to a new platform is relatively easy.
External links
- The C Standard Library: A detailed description of the header files
- The C Library Reference Guide
- Handy list of which headers are in which standard
- Microsoft C Run-Time Libraries on MSDN
- NetBSD C libraries manual and full C library source
- Manual pages for the original C standard libraries in Unix