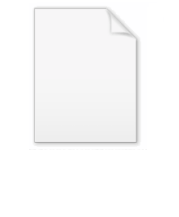
Name resolution
Encyclopedia
In computer languages
ExpressionsExpression (programming)
An expression in a programming language is a combination of explicit values, constants, variables, operators, and functions that are interpreted according to the particular rules of precedence and of association for a particular programming language, which computes and then produces another value...
in computer languages can contain identifiers. The semantics
Semantics
Semantics is the study of meaning. It focuses on the relation between signifiers, such as words, phrases, signs and symbols, and what they stand for, their denotata....
of such expressions depend on the entities that the identifiers refer to. The algorithm
Algorithm
In mathematics and computer science, an algorithm is an effective method expressed as a finite list of well-defined instructions for calculating a function. Algorithms are used for calculation, data processing, and automated reasoning...
that determines what an identifier in a given context refers to is part of the language definition.
The complexity of these algorithms is influenced by the sophistication of the language. For example, name resolution in assembly language
Assembly language
An assembly language is a low-level programming language for computers, microprocessors, microcontrollers, and other programmable devices. It implements a symbolic representation of the machine codes and other constants needed to program a given CPU architecture...
usually involves only a single simple table lookup
Associative array
In computer science, an associative array is an abstract data type composed of a collection of pairs, such that each possible key appears at most once in the collection....
, while name resolution in C++
C++
C++ is a statically typed, free-form, multi-paradigm, compiled, general-purpose programming language. It is regarded as an intermediate-level language, as it comprises a combination of both high-level and low-level language features. It was developed by Bjarne Stroustrup starting in 1979 at Bell...
is extremely complicated as it involves:
- namespaces, which make it possible for an identifier to have different meanings depending on its associated namespace;
- scopeScope (programming)In computer programming, scope is an enclosing context where values and expressions are associated. Various programming languages have various types of scopes. The type of scope determines what kind of entities it can contain and how it affects them—or semantics...
s, which make it possible for an identifier to have different meanings at different scope levels, and which involves various scope overriding and hiding rules. At the most basic level name resolution usually attempts to find the bindingName bindingIn programming languages, name binding is the association of objects with identifiers. An identifier bound to an object is said to reference that object. Machine languages have no built-in notion of identifiers, but name-object bindings as a service and notation for the programmer is implemented...
in the smallest enclosing scope, so that for example local variables supersede global variables; this is called shadowing. - visibility rules, which determine whether identifiers from specific namespaces or scopes are visible from the current context;
- overloadingMethod overloadingFunction overloading or method overloading is a feature found in various programming languages such as Ada, C#, VB.NET, C++, D and Java that allows the creation of several methods with the same name which differ from each other in terms of the type of the input and the type of the output of the...
, which makes it possible for an identifier to have different meanings depending on how it is used, even in a single namespace or scope; - accessibility, which determines whether identifiers from an otherwise visible scope are actually accessible and participate in the name resolution process.
Static versus dynamic
In programming languageProgramming language
A programming language is an artificial language designed to communicate instructions to a machine, particularly a computer. Programming languages can be used to create programs that control the behavior of a machine and/or to express algorithms precisely....
s, name resolution can be performed either at compile time
Compile time
In computer science, compile time refers to either the operations performed by a compiler , programming language requirements that must be met by source code for it to be successfully compiled , or properties of the program that can be reasoned about at compile time.The operations performed at...
or at runtime. The former is called static name resolution, the latter is called dynamic name resolution.
A somewhat common misconception is that dynamic typing implies dynamic name resolution. However, static typing does imply static name resolution. For example, Erlang is dynamically typed but has static name resolution.
Static name resolution catches, at compile time, use of variables that are not in scope; preventing programmer errors. Languages with dynamic scope resolution sacrifice this safety for more flexibility; they can typically set and get variables in the some scope at runtime.
For example, in Python
Python (programming language)
Python is a general-purpose, high-level programming language whose design philosophy emphasizes code readability. Python claims to "[combine] remarkable power with very clear syntax", and its standard library is large and comprehensive...
:
locals['num'] = 999 # equivalent to: num = 999
noun = "troubles"
noun2 = "hound"
- which variables to use are decided at runtime
print("{num} {noun} and a {noun2} ain't one".format(**locals))
- outputs: 999 troubles and a hound ain't one
However, relying on dynamic name resolution in code is discouraged by the Python community. The feature also may be removed in a later version of Python.
Examples of languages that use static name resolution include C
C (programming language)
C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system....
, C++
C++
C++ is a statically typed, free-form, multi-paradigm, compiled, general-purpose programming language. It is regarded as an intermediate-level language, as it comprises a combination of both high-level and low-level language features. It was developed by Bjarne Stroustrup starting in 1979 at Bell...
, E
E (programming language)
E is an object-oriented programming language for secure distributed computing, created by Mark S. Miller, Dan Bornstein, and others at Electric Communities in 1997. E is mainly descended from the concurrent language Joule and from Original-E, a set of extensions to Java for secure distributed...
, Erlang
Erlang
Erlang may refer to:* Agner Krarup Erlang , a mathematician and engineer after whom several concepts are named** Erlang , a unit to measure traffic in telecommunications or other domains...
, Haskell
Haskell (programming language)
Haskell is a standardized, general-purpose purely functional programming language, with non-strict semantics and strong static typing. It is named after logician Haskell Curry. In Haskell, "a function is a first-class citizen" of the programming language. As a functional programming language, the...
, Java
Java (programming language)
Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities...
, Pascal
Pascal (programming language)
Pascal is an influential imperative and procedural programming language, designed in 1968/9 and published in 1970 by Niklaus Wirth as a small and efficient language intended to encourage good programming practices using structured programming and data structuring.A derivative known as Object Pascal...
, Scheme, and Smalltalk
Smalltalk
Smalltalk is an object-oriented, dynamically typed, reflective programming language. Smalltalk was created as the language to underpin the "new world" of computing exemplified by "human–computer symbiosis." It was designed and created in part for educational use, more so for constructionist...
. Examples of languages that use dynamic name resolution include some Lisp dialects, Perl
Perl
Perl is a high-level, general-purpose, interpreted, dynamic programming language. Perl was originally developed by Larry Wall in 1987 as a general-purpose Unix scripting language to make report processing easier. Since then, it has undergone many changes and revisions and become widely popular...
, PHP
PHP
PHP is a general-purpose server-side scripting language originally designed for web development to produce dynamic web pages. For this purpose, PHP code is embedded into the HTML source document and interpreted by a web server with a PHP processor module, which generates the web page document...
, Python
Python (programming language)
Python is a general-purpose, high-level programming language whose design philosophy emphasizes code readability. Python claims to "[combine] remarkable power with very clear syntax", and its standard library is large and comprehensive...
, REBOL
REBOL
REBOL is a cross-platform data exchange language and a multi-paradigm dynamic programming language originally designed by Carl Sassenrath for network communications and distributed computing. The language and its official implementation, which is a proprietary freely redistributable software are...
, and Tcl
Tcl
Tcl is a scripting language created by John Ousterhout. Originally "born out of frustration", according to the author, with programmers devising their own languages intended to be embedded into applications, Tcl gained acceptance on its own...
.
Name masking
Masking occurs when the same identifier is used for different entities in overlapping lexical scopes. An identifier I' masks an identifier I when two conditions are met- I' has the same name as I
- I' is defined in a scope which is a subset of the scope of I
For example, the parameter x masks the local variable in this common pattern:
private int foo; // A declaration with name "foo" in an outer scope
public void setFoo(int foo) { // A declaration with the same name in the inner scope
// "foo" is resolved by looking in the innermost scope first,
// so the author uses a different syntax, this.foo, to refer to the name "foo"
// in the outer scope.
this.foo = foo;
}
// "foo" here means the same as this.foo below,
// since setFoo's parameter is no longer in scope.
public void getFoo { return foo; }
Alpha renaming to make name resolution trivial
In programming languages with lexical scopingScope (programming)
In computer programming, scope is an enclosing context where values and expressions are associated. Various programming languages have various types of scopes. The type of scope determines what kind of entities it can contain and how it affects them—or semantics...
that do not reflect
Reflection (computer science)
In computer science, reflection is the process by which a computer program can observe and modify its own structure and behavior at runtime....
over variable names, α-conversion (or α-renaming) can be used to make name resolution easy by finding a substitution that makes sure that no variable name masks
Variable shadowing
In computer programming, variable shadowing occurs when a variable declared within a certain scope has the same name as a variable declared in an outer scope. This outer variable is said to be shadowed...
another name in a containing scope.
Alpha-renaming can make static code analysis
Static code analysis
Static program analysis is the analysis of computer software that is performed without actually executing programs built from that software In most cases the analysis is performed on some version of the source code and in the other cases some form of the object code...
easier since only the alpha renamer needs to understand the language's scoping rules.
For example, in this code:
class Point {
private:
double x, y;
public:
Point(double x, double y) { // x and y declared here mask the privates
setX(x);
setY(y);
}
void setX(double newx) { x = newx; }
void setY(double newy) { y = newy; }
}
within the Point constructor, the class variables x and y are shadowed
Variable shadowing
In computer programming, variable shadowing occurs when a variable declared within a certain scope has the same name as a variable declared in an outer scope. This outer variable is said to be shadowed...
by local variables of the same name. This might be alpha-renamed to:
class Point {
private:
double x, y;
public:
Point(double a, double b) {
setX(a);
setY(b);
}
void setX(double newx) { x = newx; }
void setY(double newy) { y = newy; }
}
In the new version, there is no masking, so it is immediately obvious which uses correspond to which declarations.
In computer networks
In computer networks, name resolution is used to find a lower level address (such as an IP addressIP address
An Internet Protocol address is a numerical label assigned to each device participating in a computer network that uses the Internet Protocol for communication. An IP address serves two principal functions: host or network interface identification and location addressing...
) that corresponds to a given higher level address (such as a hostname
Hostname
A hostname is a label that is assigned to a device connected to a computer network and that is used to identify the device in various forms of electronic communication such as the World Wide Web, e-mail or Usenet...
). Commands that allow name resolution are: nslookup and host. See Domain Name System
Domain name system
The Domain Name System is a hierarchical distributed naming system for computers, services, or any resource connected to the Internet or a private network. It associates various information with domain names assigned to each of the participating entities...
, OSI Model
OSI model
The Open Systems Interconnection model is a product of the Open Systems Interconnection effort at the International Organization for Standardization. It is a prescription of characterizing and standardizing the functions of a communications system in terms of abstraction layers. Similar...
.
In semantics and text extraction
Also referred to as entity resolution, in this context name resolution refers to the ability of text miningText mining
Text mining, sometimes alternately referred to as text data mining, roughly equivalent to text analytics, refers to the process of deriving high-quality information from text. High-quality information is typically derived through the devising of patterns and trends through means such as...
software to determine which actual person, actor, or object a particular references refers to, by looking at natural language text.
Name resolution in simple text
For example, in the text miningText mining
Text mining, sometimes alternately referred to as text data mining, roughly equivalent to text analytics, refers to the process of deriving high-quality information from text. High-quality information is typically derived through the devising of patterns and trends through means such as...
field, software frequently needs to interpret the following text:
John gave Edward the book. He then stood up and called to John to come back into the room.
In these sentences, the software must determine whether the pronoun "he" refers to "John", or "Edward" from the first sentence. The software must also determine whether the "John" referred to in the second sentence is the same as the "John" in the first sentence, or a third person whose name also happens to be "John". Such examples apply to almost all languages, and not only English.
Name resolution across documents
Frequently, this type of name resolution is also used across documents, for example to determine whether the "George Bush" referenced in an old newspaper article as President of the United States (George H. W. BushGeorge H. W. Bush
George Herbert Walker Bush is an American politician who served as the 41st President of the United States . He had previously served as the 43rd Vice President of the United States , a congressman, an ambassador, and Director of Central Intelligence.Bush was born in Milton, Massachusetts, to...
) is the same person as the "George Bush" mentioned in a separate news article years later about a man who is running for President (George W. Bush
George W. Bush
George Walker Bush is an American politician who served as the 43rd President of the United States, from 2001 to 2009. Before that, he was the 46th Governor of Texas, having served from 1995 to 2000....
.) Because many people may have the same name, analysts and software must take into account substantially more information than only a name to determine whether two identical references ("George Bush") actually refer to the same specific entity or person.
Name/entity resolution in text extraction and semantics is a notoriously difficult problem, in part because in many cases there is not sufficient information to make an accurate determination. Numerous partial solutions exist that rely on specific contextual clues found in the data, but there is no currently known general solution.
For examples of software that might provide name resolution benefits, see also:
- AeroTextAeroTextAeroText is a suite of text mining applications that are used for content analysis. Content used can be in multiple languages.AeroText is a solution developed at the Integrated Systems and Solutions division of Lockheed Martin Corporation, a leading U.S. Defense contractor...
- AlchemyAPI
- AttensityAttensityAttensity provides text analytics software for Customer Experience Management . Attensity's software applications extract facts, relationships and sentiment from unstructured data, which comprise approximately 85% of the information companies store electronically.The software uses natural language...
- AutonomyAutonomy CorporationAutonomy is a multinational enterprise software company with joint headquarters in Cambridge, United Kingdom, and San Francisco, USA and a subsidiary of Hewlett-Packard. The company uses a combination of technologies born out of research at the University of Cambridge...
- DBpedia SpotlightDBpedia SpotlightDBpedia Spotlight is a tool for annotating mentions of DBpedia resources in text, providing a solution for linking unstructured information sources to the Linked Open Data cloud through DBpedia. DBpedia Spotlight performs named entity extraction, including entity detection and Name Resolution...
, providing a simple approach for name resolution using DBpedia and Wikipedia.
See also
- Identity resolutionIdentity resolutionIdentity resolution is an operational intelligence process, typically powered by an identity resolution engine or middleware stack, whereby organizations can connect disparate data sources with a view to understanding possible identity matches and non-obvious relationships across multiple data silos...
- namespace (programming)
- Scope (programming)Scope (programming)In computer programming, scope is an enclosing context where values and expressions are associated. Various programming languages have various types of scopes. The type of scope determines what kind of entities it can contain and how it affects them—or semantics...
- Named entity recognitionNamed entity recognitionNamed-entity recognition is a subtask of information extraction that seeks to locate and classify atomic elements in text into predefined categories such as the names of persons, organizations, locations, expressions of times, quantities, monetary values, percentages, etc.Most research on NER...
- Naming collisionNaming collisionA naming collision is a circumstance where two or more identifiers in a given namespace or a given scope cannot be unambiguously resolved, and such unambiguous resolution is a requirement of the underlying system.- Example: XML element names :...
- Anaphor resolution