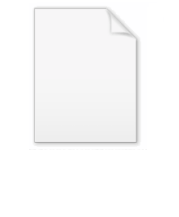
Model-view-controller
Encyclopedia
Model–view–controller (MVC) is a software architecture, currently considered an architectural pattern
used in software engineering
. The pattern isolates "domain logic" (the application logic for the user) from the user interface
(input and presentation), permitting independent development, testing and maintenance of each (separation of concerns
).
Model View Controller (MVC) pattern creates applications that separate the different aspects of the application (input logic, business logic, and UI logic), while providing a loose coupling between these elements.
, then working on Smalltalk
at Xerox PARC
. The original implementation is described in depth in the influential paper "Applications Programming in Smalltalk-80: How to use Model–View–Controller".
Some implementations such as the W3C XForms
also use the concept of a dependency graph
to automate the updating of views when data in the model changes.
The goal of MVC is, by decoupling models and views, to reduce the complexity in architectural design and to increase flexibility and maintainability of code
. MVC(Model-View-Controller) has also been used to simplify the design of Autonomic and Self-Managed systems
The view renders the model into a form suitable for interaction, typically a user interface element. Multiple views can exist for a single model for different purposes. A view port typically has a one to one correspondence with a display surface and knows how to render to it.
The controller receives user input and initiates a response by making calls on model objects. A controller accepts input from the user and instructs the model and a view port to perform actions based on that input.
MVC is often seen in web applications where the view is the HTML or XHTML generated by the app. The controller receives GET or POST input and decides what to do with it, handing over to domain objects (i.e. the model) that contain the business rule
s and know how to carry out specific tasks such as processing a new subscription, and which hand control to (X)HTML-generating components such as templating engines, XML pipeline
s, Ajax callbacks, etc.
The model is not necessarily merely a database; the 'model' in MVC is both the data and the business/domain logic needed to manipulate the data in the application. Many applications use a persistent storage mechanism such as a database to store data. MVC does not specifically mention the data access layer because it is understood to be underneath or encapsulated by the model. Models are not data access object
s; however, in very simple apps that have little domain logic there is no real distinction to be made. Active Record
is an accepted design pattern that merges domain logic and data access code — a model which knows how to persist itself.
, it is essentially an architecture. This means that it can be implemented even without an object-oriented language or a specific class hierarchy. For example, using as little as jQuery
's
. The key is simply to divide up the responsibilities of the MVC components into clearly defined sections of code. As stated in the overview, the code that embodies the model takes care of state, business logic, persistence, and notifications. The persistence can be implemented via cookies or AJAX
. The notifications can be taken care of by the
styles. The code that embodies the controller takes care of initialization of the model and wiring up the events between the view's HTML DOM elements and controller and between the model and the view code, using
s and Java Server Pages from Java EE:
Model
View
Controller
The Servlet is a Java class, and it communicates and interacts with the model, but does not need to generate HTML
or XHTML
output; the JSPs do not have to communicate with the model because the Servlet provides them with the information—they can concentrate on creating output.
's MVC implementation inspired many other GUI
frameworks, such as the following:
s as a "view for web" component.
MVC is typically implemented as a "Model 2
" architecture in Sun
parlance. Model 2 focuses on efficiently handling and dispatching full page form posts and reconstructing the full page via a front controller
. Complex web applications continue to be more difficult to design than traditional applications because of this "full page" effect. More recently "view for web" Ajax
driven frameworks that focus on firing focused UI events at specific UI Components on the page are emerging. This is causing MVC to be revisited for web application development using traditional desktop programming techniques.
Java Stand-alone Application Toolkit:
Architectural pattern (computer science)
An architectural pattern in software is a standard design in the field of software architecture. The concept of a software architectural pattern has a broader scope than the concept of a software design pattern...
used in software engineering
Software engineering
Software Engineering is the application of a systematic, disciplined, quantifiable approach to the development, operation, and maintenance of software, and the study of these approaches; that is, the application of engineering to software...
. The pattern isolates "domain logic" (the application logic for the user) from the user interface
User interface
The user interface, in the industrial design field of human–machine interaction, is the space where interaction between humans and machines occurs. The goal of interaction between a human and a machine at the user interface is effective operation and control of the machine, and feedback from the...
(input and presentation), permitting independent development, testing and maintenance of each (separation of concerns
Separation of concerns
In computer science, separation of concerns is the process of separating a computer program into distinct features that overlap in functionality as little as possible. A concern is any piece of interest or focus in a program. Typically, concerns are synonymous with features or behaviors...
).
Model View Controller (MVC) pattern creates applications that separate the different aspects of the application (input logic, business logic, and UI logic), while providing a loose coupling between these elements.
History
MVC was first described in 1979 by Trygve ReenskaugTrygve Reenskaug
Trygve Mikkjel Heyerdahl Reenskaug is a Norwegian computer scientist and professor emeritus of the University of Oslo. He formulated the model-view-controller pattern for Graphic User Interface software design in 1979 while visiting the Xerox Palo Alto Research Center...
, then working on Smalltalk
Smalltalk
Smalltalk is an object-oriented, dynamically typed, reflective programming language. Smalltalk was created as the language to underpin the "new world" of computing exemplified by "human–computer symbiosis." It was designed and created in part for educational use, more so for constructionist...
at Xerox PARC
Xerox PARC
PARC , formerly Xerox PARC, is a research and co-development company in Palo Alto, California, with a distinguished reputation for its contributions to information technology and hardware systems....
. The original implementation is described in depth in the influential paper "Applications Programming in Smalltalk-80: How to use Model–View–Controller".
Overview
Though MVC comes in different flavors, control flow is generally as follows:- The user interacts with the user interface in some way (for example, by pressing a mouse button).
- The controller handles the input event from the user interface, often via a registered handler or callbackCallback (computer science)In computer programming, a callback is a reference to executable code, or a piece of executable code, that is passed as an argument to other code. This allows a lower-level software layer to call a subroutine defined in a higher-level layer....
, and converts the event into an appropriate user action, understandable for the model. - The controller notifies the model of the user action, possibly resulting in a change in the model's state. (For example, the controller updates the user's shopping cartShopping cart softwareShopping cart software is software used in e-commerce to assist people making purchases online, analogous to the American English term 'shopping cart'...
.) - A view queries the model in order to generate an appropriate user interface (for example the view lists the shopping cart's contents). The view gets its own data from the model.. In some implementations, the controller may issue a general instruction to the view to render itself. In others, the view is automatically notified by the model of changes in state (ObserverObserver patternThe observer pattern is a software design pattern in which an object, called the subject, maintains a list of its dependents, called observers, and notifies them automatically of any state changes, usually by calling one of their methods...
) that require a screen update. - The user interface waits for further user interactions, which restarts the control flow cycle.
Some implementations such as the W3C XForms
XForms
XForms is an XML format for the specification of a data processing model for XML data and user interface for the XML data, such as web forms...
also use the concept of a dependency graph
Dependency graph
In mathematics, computer science and digital electronics, a dependency graph is a directed graph representing dependencies of several objects towards each other...
to automate the updating of views when data in the model changes.
The goal of MVC is, by decoupling models and views, to reduce the complexity in architectural design and to increase flexibility and maintainability of code
Source code
In computer science, source code is text written using the format and syntax of the programming language that it is being written in. Such a language is specially designed to facilitate the work of computer programmers, who specify the actions to be performed by a computer mostly by writing source...
. MVC(Model-View-Controller) has also been used to simplify the design of Autonomic and Self-Managed systems
Concepts
The model manages the behaviour and data of the application domain, responds to requests for information about its state (usually from the view), and responds to instructions to change state (usually from the controller). In event-driven systems, the model notifies observers (usually views) when the information changes so that they can react.The view renders the model into a form suitable for interaction, typically a user interface element. Multiple views can exist for a single model for different purposes. A view port typically has a one to one correspondence with a display surface and knows how to render to it.
The controller receives user input and initiates a response by making calls on model objects. A controller accepts input from the user and instructs the model and a view port to perform actions based on that input.
- An MVC application may be a collection of model/view/controller triads, each responsible for a different UI element. The SwingSwing (Java)Swing is the primary Java GUI widget toolkit. It is part of Oracle's Java Foundation Classes — an API for providing a graphical user interface for Java programs....
GUI system, for example, models almost all interface components as individual MVC systems.
MVC is often seen in web applications where the view is the HTML or XHTML generated by the app. The controller receives GET or POST input and decides what to do with it, handing over to domain objects (i.e. the model) that contain the business rule
Business rule
A Business rule is a statement that defines or constrains some aspect of the business and always resolves to either true or false. Business rules are intended to assert business structure or to control or influence the behavior of the business. Business rules describe the operations, definitions...
s and know how to carry out specific tasks such as processing a new subscription, and which hand control to (X)HTML-generating components such as templating engines, XML pipeline
XML pipeline
In software, an XML Pipeline is formed when XML processes, especially XML transformations and XML validations, are connected together....
s, Ajax callbacks, etc.
The model is not necessarily merely a database; the 'model' in MVC is both the data and the business/domain logic needed to manipulate the data in the application. Many applications use a persistent storage mechanism such as a database to store data. MVC does not specifically mention the data access layer because it is understood to be underneath or encapsulated by the model. Models are not data access object
Data Access Object
In computer software, a data access object is an object that provides an abstract interface to some type of database or persistence mechanism, providing some specific operations without exposing details of the database. It provides a mapping from application calls to the persistence layer...
s; however, in very simple apps that have little domain logic there is no real distinction to be made. Active Record
Active record pattern
In software engineering, the active record pattern is an architectural pattern found in software that stores its data in relational databases. It was named by Martin Fowler in his 2003 book Patterns of Enterprise Application Architecture...
is an accepted design pattern that merges domain logic and data access code — a model which knows how to persist itself.
Architecture vs. frameworks
Although MVC is typically associated with frameworksSoftware framework
In computer programming, a software framework is an abstraction in which software providing generic functionality can be selectively changed by user code, thus providing application specific software...
, it is essentially an architecture. This means that it can be implemented even without an object-oriented language or a specific class hierarchy. For example, using as little as jQuery
JQuery
jQuery is a cross-browser JavaScript library designed to simplify the client-side scripting of HTML. It was released in January 2006 at BarCamp NYC by John Resig...
's
trigger
and bind
, it is possible to build robust MVC applications in a browser using JavaScriptJavaScript
JavaScript is a prototype-based scripting language that is dynamic, weakly typed and has first-class functions. It is a multi-paradigm language, supporting object-oriented, imperative, and functional programming styles....
. The key is simply to divide up the responsibilities of the MVC components into clearly defined sections of code. As stated in the overview, the code that embodies the model takes care of state, business logic, persistence, and notifications. The persistence can be implemented via cookies or AJAX
Ajax
- Mythology :* Ajax , son of Telamon, ruler of Salamis and a hero in the Trojan War, also known as "Ajax the Great"* Ajax the Lesser, son of Oileus, ruler of Locris and the leader of the Locrian contingent during the Trojan War.- People :...
. The notifications can be taken care of by the
jQuery.trigger
. The code that embodies the view takes care of querying the model and rendering the view. The view code can be implemented in a variety of ways, including inserting HTML DOM nodes or changing CSSCSS
-Computing:*Cascading Style Sheets, a language used to describe the style of document presentations in web development*Central Structure Store in the PHIGS 3D API*Closed source software, software that is not distributed with source code...
styles. The code that embodies the controller takes care of initialization of the model and wiring up the events between the view's HTML DOM elements and controller and between the model and the view code, using
jQuery.bind
.Example
Here is a simple application of the pattern, implementing Java ServletJava Servlet
A servlet is a Java programming language class used to extend the capabilities of servers that host applications accessed via a request-response programming model. Although servlets can respond to any type of request, they are commonly used to extend the applications hosted by Web servers...
s and Java Server Pages from Java EE:
Model
- The model is a collection of Java classClass (computer science)In object-oriented programming, a class is a construct that is used as a blueprint to create instances of itself – referred to as class instances, class objects, instance objects or simply objects. A class defines constituent members which enable these class instances to have state and behavior...
es that form a software application intended to store, and optionally separate, data. A single front end class that can communicate with any user interface (for example: a console, a graphical user interfaceGraphical user interfaceIn computing, a graphical user interface is a type of user interface that allows users to interact with electronic devices with images rather than text commands. GUIs can be used in computers, hand-held devices such as MP3 players, portable media players or gaming devices, household appliances and...
, or a web applicationWeb applicationA web application is an application that is accessed over a network such as the Internet or an intranet. The term may also mean a computer software application that is coded in a browser-supported language and reliant on a common web browser to render the application executable.Web applications are...
).
View
- The view is represented by a Java Server Page, with data being transported to the page in the HttpServletRequest or HttpSession.
Controller
- The Controller servletJava ServletA servlet is a Java programming language class used to extend the capabilities of servers that host applications accessed via a request-response programming model. Although servlets can respond to any type of request, they are commonly used to extend the applications hosted by Web servers...
communicates with the front end of the model and loads the HttpServletRequest or HttpSession with appropriate data, before forwarding the HttpServletRequest and Response to the JSP using a RequestDispatcher.
The Servlet is a Java class, and it communicates and interacts with the model, but does not need to generate HTML
HTML
HyperText Markup Language is the predominant markup language for web pages. HTML elements are the basic building-blocks of webpages....
or XHTML
XHTML
XHTML is a family of XML markup languages that mirror or extend versions of the widely-used Hypertext Markup Language , the language in which web pages are written....
output; the JSPs do not have to communicate with the model because the Servlet provides them with the information—they can concentrate on creating output.
Implementations of MVC as GUI frameworks
SmalltalkSmalltalk
Smalltalk is an object-oriented, dynamically typed, reflective programming language. Smalltalk was created as the language to underpin the "new world" of computing exemplified by "human–computer symbiosis." It was designed and created in part for educational use, more so for constructionist...
's MVC implementation inspired many other GUI
Graphical user interface
In computing, a graphical user interface is a type of user interface that allows users to interact with electronic devices with images rather than text commands. GUIs can be used in computers, hand-held devices such as MP3 players, portable media players or gaming devices, household appliances and...
frameworks, such as the following:
- AppFlowerAppFlowerAppFlower is an open source application builder for use in building enterprise ready web-based applications. With the aim to make development doable by everyone, doing so with automatic code generations. AppFlower provides a visual designer to easily develop application without prior knowledge of...
open source application builder with visual designer. - XPagesXPagesXPages is a rapid web and mobile application development platform. It allows IBM Lotus Notes data to be displayed to browser clients on all platforms....
- for IBM Lotus Notes/Domino - CocoaCocoa (API)Cocoa is Apple's native object-oriented application programming interface for the Mac OS X operating system and—along with the Cocoa Touch extension for gesture recognition and animation—for applications for the iOS operating system, used on Apple devices such as the iPhone, the iPod Touch, and...
framework and its GUI part AppKitApplication KitThe Application Kit is a collection of classes within the OpenStep specification and provided by such operating systems as OPENSTEP, GNUstep, and Mac OS X under Cocoa, providing classes oriented around graphical user interface capabilities...
, as a direct descendant of OpenStepOpenStepOpenStep was an object-oriented application programming interface specification for an object-oriented operating system that used a non-NeXTSTEP operating system as its core, principally developed by NeXT with Sun Microsystems. OPENSTEP was a specific implementation of the OpenStep API developed...
, encourage the use of MVC. Interface BuilderInterface BuilderInterface Builder is a software development application for Apple's Mac OS X operating system. It is part of Xcode , the Apple Developer Connection developer's toolset. Interface Builder allows Cocoa and Carbon developers to create interfaces for applications using a graphical user...
constructs views, and connects them to Controllers via Outlets and Actions. - GNUstepGNUstepGNUstep is a free software implementation of Cocoa Objective-C libraries , widget toolkit, and application development tools not only for Unix-like operating systems, but also for Microsoft Windows. It is part of the GNU Project.GNUstep features a cross-platform, object-oriented development...
, also based on OpenStepOpenStepOpenStep was an object-oriented application programming interface specification for an object-oriented operating system that used a non-NeXTSTEP operating system as its core, principally developed by NeXT with Sun Microsystems. OPENSTEP was a specific implementation of the OpenStep API developed...
, encourages MVC as well. - GTK+GTK+GTK+ is a cross-platform widget toolkit for creating graphical user interfaces. It is licensed under the terms of the GNU LGPL, allowing both free and proprietary software to use it. It is one of the most popular toolkits for the X Window System, along with Qt.The name GTK+ originates from GTK;...
provides models (as both interfaces and as concrete implementations) and views, while clients implement the controllers through signals. - JFaceJFaceJFace is defined by the Eclipse project as "a UI toolkit that provides helper classes for developing UI features that can be tedious to implement." It is a layer that sits on top of the raw widget system, and provides classes for handling common UI programming tasks...
. - Oracle Application Development FrameworkOracle Application Development FrameworkIn computing, Oracle Application Development Framework, usually called Oracle ADF, provides a commercial Java framework for building enterprise applications. It provides visual and declarative approaches to Java EE development...
- Oracle ADF - Microsoft Foundation Class LibraryMicrosoft Foundation Class LibraryThe Microsoft Foundation Class Library is a library that wraps portions of the Windows API in C++ classes, including functionality that enables them to use a default application framework...
(MFC) - called the document/view architecture. - ASP.NET MVC FrameworkASP.NET MVC FrameworkThe ASP.NET MVC Framework is a web application framework that implements the model-view-controller pattern. Based on ASP.NET, it allows software developers to build a Web application as a composition of three roles: Model, View and Controller. A model represents the state of a particular aspect of...
- reusing jQuery libraries and proprietary Microsoft Ajax libraries. - Microsoft Composite UI Application BlockComposite UI Application BlockThe Composite UI Application Block is an addition to Microsoft's .NET Framework for creating complex user interfaces made of loosely coupled components. Developed by Microsoft's patterns & practices team, CAB is used exclusively for developing Windows Forms. A derivative version of CAB exists in...
, part of the Microsoft Enterprise LibraryMicrosoft Enterprise LibraryThe Microsoft Enterprise Library is a set of tools and programming libraries for the Microsoft .NET Framework. It provides an API to facilitate proven practices in core areas of programming including data access, security, logging, exception handling and others...
. - QtQt (toolkit)Qt is a cross-platform application framework that is widely used for developing application software with a graphical user interface , and also used for developing non-GUI programs such as command-line tools and consoles for servers...
since Qt4 release. - Java SwingSwing (Java)Swing is the primary Java GUI widget toolkit. It is part of Oracle's Java Foundation Classes — an API for providing a graphical user interface for Java programs....
. - Apache PivotApache PivotApache Pivot is an open-source platform for building rich web applications in Java or any JVM-compatible language. It is released under the Apache License version 2.0.-Architecture:Its classes are divided in the following categories:...
. - Adobe FlexAdobe FlexAdobe Flex is a software development kit released by Adobe Systems for the development and deployment of cross-platform rich Internet applications based on the Adobe Flash platform...
with the Cairngorm FrameworkCairngorm (Flex framework)Cairngorm was one of the primary open source frameworks for application architecture in Adobe Flex. It was developed by iteration::two, who was acquired by Macromedia in 2005. It is part of the Adobe Engagement Platform....
. - WavemakerWavemakerWaveMaker is an open source software development platform that automates much of the process for creating Java web and cloud applications. WaveMaker provides a visual rapid application development platform and is available as a free open source software download...
open source, browser-based development tool based on MVC. - The Model–view–viewmodel pattern, similar to MVC, is often used to develop Windows Presentation FoundationWindows Presentation FoundationDeveloped by Microsoft, the Windows Presentation Foundation is a computer-software graphical subsystem for rendering user interfaces in Windows-based applications. WPF, previously known as "Avalon", was initially released as part of .NET Framework 3.0. Rather than relying on the older GDI...
(WPF) applications. - Visual FoxExpress is a Visual FoxProVisual FoxProVisual FoxPro is a data-centric object-oriented and procedural programming language produced by Microsoft. It is derived from FoxPro which was developed by Fox Software beginning in 1984. Fox Technologies merged with Microsoft in 1992, after which the software acquired further features and the...
MVC framework. - Crank Storyboard SuiteCrank Storyboard SuiteCrank Storyboard Suite is an Eclipse-based graphical user interface builder called Storyboard Designer and a target runtime called Storyboard Engine.- History and development :...
- Eiffel (programming language)Eiffel (programming language)Eiffel is an ISO-standardized, object-oriented programming language designed by Bertrand Meyer and Eiffel Software. The design of the language is closely connected with the Eiffel programming method...
Agents (See Touch of Class, chapter 17).
Implementations of MVC as web-based frameworks
In the design of web applications, MVC is implemented by web template systemWeb template system
A Web template system describes the software and methodologies used to produce web pages and for deployment on websites and delivery over the Internet. Such systems process web templates, using a template engine...
s as a "view for web" component.
MVC is typically implemented as a "Model 2
Model 2
In the design of Java Web applications, there are two commonly used design models, referred to as Model 1 and Model 2.Model 1 is a simple pattern whereby the code responsible for the display of content is intermixed with logic. Model 1 is only recommended for small applications and is mostly...
" architecture in Sun
Sun Microsystems
Sun Microsystems, Inc. was a company that sold :computers, computer components, :computer software, and :information technology services. Sun was founded on February 24, 1982...
parlance. Model 2 focuses on efficiently handling and dispatching full page form posts and reconstructing the full page via a front controller
Front Controller pattern
The Front Controller Pattern is a software design pattern listed in several pattern catalogs. The pattern relates to the design of web applications. It "provides a centralized entry point for handling requests."...
. Complex web applications continue to be more difficult to design than traditional applications because of this "full page" effect. More recently "view for web" Ajax
Ajax (programming)
Ajax is a group of interrelated web development methods used on the client-side to create asynchronous web applications...
driven frameworks that focus on firing focused UI events at specific UI Components on the page are emerging. This is causing MVC to be revisited for web application development using traditional desktop programming techniques.
ABAP Objects
- Business Server Pages (BSP)
- Web Dynpro ABAP
ActionScript
- PureMVCPureMVCPureMVC is a framework for creating applications based upon the well-established Model, View and Controller design pattern. The free, open source framework was originally implemented in the ActionScript 3 language for use with Adobe Flex, Flash and AIR, and it has since been ported to nearly all...
Framework for ActionScript. - CairngormCairngorm (Flex framework)Cairngorm was one of the primary open source frameworks for application architecture in Adobe Flex. It was developed by iteration::two, who was acquired by Macromedia in 2005. It is part of the Adobe Engagement Platform....
is a Flex Framework part of the Adobe Engagement PlatformAdobe Engagement PlatformAt Adobe MAX 2008, Adobe announced the new Flash Platform concept, and this has replaced the branding formerly known as Adobe Engagement Platform.In 2005, Adobe Systems bought Macromedia. The Adobe Engagement Platform was the announced name of the product line resulting from the merger of...
. - Fabrication Framework for ActionScript.
- Robotlegs MVCS Framework for ActionScript with Dependency Injection.
C++
- Wt - Web toolkitWt - Web toolkitWt is an open source widget-centric web application framework for the C++ programming language developed by Emweb. It has an API that resembles the C++ desktop application library Qt, using also a widget tree and event-driven signal/slot programming model....
A library and application server for web applications using a desktop-like event-driven MVC pattern. - CppCMSCppCMSCppCMS is an open source web application framework for the C++ programming language developed by Artyom Beilis. It is one of very few web frameworks for C++. The primary goal of CppCMS is building performance-demanding web applications...
- A C++ MVC framework that has taken many ideas from Django. - PureMVCPureMVCPureMVC is a framework for creating applications based upon the well-established Model, View and Controller design pattern. The free, open source framework was originally implemented in the ActionScript 3 language for use with Adobe Flex, Flash and AIR, and it has since been ported to nearly all...
A framework for C++.
CFML - Adobe ColdFusion, Railo, and Open BlueDragon
- ColdBox PlatformColdBox PlatformColdBox is a professional open source enterprise, conventions based web application framework designed for applications written in ColdFusion Markup Language...
The first conventions over configuration framework and development platform for ColdFusion - ColdFusion on WheelsColdFusion on WheelsColdFusion on Wheels is an open source web application framework designed for applications written in ColdFusion Markup Language. Its name is often shortened to CFWheels or Wheels....
A convention over configuration framework similar to Ruby on Rails. - Framework OneFramework OneFramework One is a lightweight, convention-over-configuration MVC framework for CFML .-History:FW/1 was created in the summer of 2009 by Sean Corfield and the latest stable version is 1.2, released in October 2010....
Framework/1 is a new framework developed by Sean Corfield and provides a good stepping stone for users new to the MVC pattern. It consists of a single CFC and favors convention over configuration. - FuseboxFusebox (programming)Fusebox is a web application framework for ColdFusion and PHP. Originally released in 1997, the current version, 5.5, was released in December 2007....
Fusebox does not force the model–view–controller (MVC) pattern or object-oriented programming (OOP) on the developer. However, either or both of these development approaches can be used with Fusebox. - Mach-IIMach-IIMach-II is a web-application framework focused on easing software development and maintenance. It was the first Object-Oriented framework for CFML. It is maintained by a group of dedicated open source programmers-References:* * * *...
A framework that focuses on trying to ease software development and maintenance. - Model-GlueModel-GlueModel-Glue is an OO web application framework based on the MVC design pattern. Its goal is to simplify development of OO ColdFusion applications...
Through a simple implementation of implicit invocation and model–view–controller, they allow applications to be well organized without sacrificing flexibility. - PureMVCPureMVCPureMVC is a framework for creating applications based upon the well-established Model, View and Controller design pattern. The free, open source framework was originally implemented in the ActionScript 3 language for use with Adobe Flex, Flash and AIR, and it has since been ported to nearly all...
Framework for ColdFusion.
Flex
- CairngormCairngorm (Flex framework)Cairngorm was one of the primary open source frameworks for application architecture in Adobe Flex. It was developed by iteration::two, who was acquired by Macromedia in 2005. It is part of the Adobe Engagement Platform....
one of the primary open source frameworks for application architecture in Adobe Flex. - PureMVCPureMVCPureMVC is a framework for creating applications based upon the well-established Model, View and Controller design pattern. The free, open source framework was originally implemented in the ActionScript 3 language for use with Adobe Flex, Flash and AIR, and it has since been ported to nearly all...
ActionScript 3 MVC framework for Flex, Flash and AIR
Java
MVC web application frameworks:- AraneaAranea frameworkAranea framework is an Open Source Java Hierarchical Model-View-Controller web framework that was developed by an Estonian company Webmedia. Aranea provides a simple common approach to building web application components, reusing custom or general GUI logic and extending the framework...
- CocoonApache CocoonApache Cocoon, usually just called Cocoon, is a web application framework built around the concepts of pipeline, separation of concerns and component-based web development. The framework focuses on XML and XSLT publishing and is built using the Java programming language...
- CodeCharge StudioCodeCharge StudioCodeCharge Studio is a rapid application development and integrated development environment for creating database-driven web application...
- JSFJavaServer FacesJavaServer Faces is a Java-based Web application framework intended to simplify development integration of web-based user interfaces....
- MakumbaMakumba (framework)Makumba is a query-centric application framework using the model-view-controller pattern and designed to develop data driven web applications. It provides a custom JSP tag-library as a main interface, but leaves API open for advanced access...
Web development framework in the form of JSP Tag Library and Java API that is based on MVC, but willingly breaks it - Oracle Application Development FrameworkOracle Application Development FrameworkIn computing, Oracle Application Development Framework, usually called Oracle ADF, provides a commercial Java framework for building enterprise applications. It provides visual and declarative approaches to Java EE development...
- Oracle Application FrameworkOracle Application Framework-Introduction:Oracle Application Framework is a proprietary framework developed by Oracle Corporation for application development within the Oracle E-Business Suite...
- Play FrameworkPlay FrameworkPlay is an open source web application framework, written in Java, which follows the model-view-controller architectural pattern. It aims to optimize developer productivity by using convention over configuration, hot code reloading and display of errors in the browser.Although Play is written in...
- PureMVCPureMVCPureMVC is a framework for creating applications based upon the well-established Model, View and Controller design pattern. The free, open source framework was originally implemented in the ActionScript 3 language for use with Adobe Flex, Flash and AIR, and it has since been ported to nearly all...
, a framework for Java - SlingApache SlingApache Sling is an open source Web framework for the Java platform designed to create content-centric applications on top of a JSR-170-compliant content repository such as Apache Jackrabbit. Apache Sling allows developers to deploy their application components as OSGi bundles or as scripts and...
, used to create content based applications on top of JCR. Supported scripting languages are JSPJavaServer PagesJavaServer Pages is a Java technology that helps software developers serve dynamically generated web pages based on HTML, XML, or other document types...
, server-side JavaScriptJavaScriptJavaScript is a prototype-based scripting language that is dynamic, weakly typed and has first-class functions. It is a multi-paradigm language, supporting object-oriented, imperative, and functional programming styles....
, RubyRuby (programming language)Ruby is a dynamic, reflective, general-purpose object-oriented programming language that combines syntax inspired by Perl with Smalltalk-like features. Ruby originated in Japan during the mid-1990s and was first developed and designed by Yukihiro "Matz" Matsumoto...
, Velocity - Spring MVC Framework
- StrutsApache StrutsApache Struts is an open-source web application framework for developing Java EE web applications. It uses and extends the Java Servlet API to encourage developers to adopt a model-view-controller architecture. It was originally created by Craig McClanahan and donated to the Apache Foundation in...
- Struts2
- StripesStripes (framework)-External links:********...
- TapestryTapestry (programming)Apache Tapestry is an open-source component-oriented Java web application framework to implement applications in accordance with the model-view-controller architectural pattern. Tapestry was created by Howard Lewis Ship independently, and was adopted by the Apache Software Foundation as a top-level...
- WavemakerWavemakerWaveMaker is an open source software development platform that automates much of the process for creating Java web and cloud applications. WaveMaker provides a visual rapid application development platform and is available as a free open source software download...
, a WYSIWYG development platform for Ajax web applications. - WebObjectsWebObjectsWebObjects was a Java web application server from Apple Inc., and a web application framework that ran on the server. It was available at no additional cost. Its hallmark features were its object-orientation, database connectivity, and prototyping tools...
- WebWork
- WicketWicket frameworkApache Wicket, commonly referred to as Wicket, is a lightweight component-based web application framework for the Java programming language conceptually similar to JavaServer Faces and Tapestry. It was originally written by Jonathan Locke in April 2004. Version 1.0 was released in June 2005...
- Web DynproWeb DynproWeb Dynpro is a proprietary web application user interface technology developed by SAP AG and exists in a Java and an ABAP flavor. Both have in general the same functionality, but usually one flavor is improved after the other, so temporary one flavor is more advanced than the other...
Java
Java Stand-alone Application Toolkit:
- SwingSwing (Java)Swing is the primary Java GUI widget toolkit. It is part of Oracle's Java Foundation Classes — an API for providing a graphical user interface for Java programs....
, which uses a Model-Delegator pattern, where the view and controller are combined, but the model is separate. - ZKZK (framework)ZK is an open-source Ajax Web application framework, written in Java, that enables creation of rich graphical user interfaces for Web applications with no JavaScript and little programming knowledge....
- an Ajax framework that enables the Ajax-level MVC pattern.
JavaScript
MVC web application frameworks:- Knockout.js An MVC Framework based on MVVM for JavaScript.
- SproutCoreSproutCoreSproutCore is an open-source JavaScript framework. Its goal is to allow developers to create web applications with advanced capabilities and a user experience comparable to that of desktop applications. When developing a SproutCore application, all code is written in JavaScript...
- PureMVCPureMVCPureMVC is a framework for creating applications based upon the well-established Model, View and Controller design pattern. The free, open source framework was originally implemented in the ActionScript 3 language for use with Adobe Flex, Flash and AIR, and it has since been ported to nearly all...
Framework for JavaScript - JavascriptMVCJavascriptMVCJavaScriptMVC is an open-source Rich Internet Application framework based on jQuery and OpenAjax. It extends those libraries with a model–view–controller architecture and tools for testing and deployment...
JavaScript MVC framework based upon jQueryJQueryjQuery is a cross-browser JavaScript library designed to simplify the client-side scripting of HTML. It was released in January 2006 at BarCamp NYC by John Resig...
core. - eMVCEMVCe|MVC is a JavaScript-based open source Model–View–Controller framework, which includes an extensive JavaScript object library and a collection of widgets in the free download. The core e|MVC object bootstraps a model/controller combination for corresponding configured view objects...
is an MVC framework based upon Dojo Toolkit. - Dojo toolkitDojo ToolkitDojo Toolkit is an open source modular JavaScript library designed to ease the rapid development of cross-platform, JavaScript/Ajax-based applications and web sites. It was started by Alex Russell, Dylan Schiemann, David Schontzler, and others in 2004 and is dual-licensed under the modified BSD...
MVC used in stores + widgets. - Backbone.js A light weight MVC Framework for JavaScript.
- ExtJS uses MVC as of version 4
- YUI 3 has an MVC module
- AngularJS
- eyeballs.js
.NET
- ASP.NET MVC FrameworkASP.NET MVC FrameworkThe ASP.NET MVC Framework is a web application framework that implements the model-view-controller pattern. Based on ASP.NET, it allows software developers to build a Web application as a composition of three roles: Model, View and Controller. A model represents the state of a particular aspect of...
- Bistro FrameworkBistro FrameworkBistro Framework is a compositional .NET MVC framework with concepts from REST and AOP. Bistro modifies the traditional concepts of MVC by breaking down a single HTTP request into a series of aspects, each processed by a separate controller. Individual controllers are bound to aspects of a URI, and...
- CodeCharge StudioCodeCharge StudioCodeCharge Studio is a rapid application development and integrated development environment for creating database-driven web application...
- Maverick.NETMaverick.NETMaverick.NET is a Maverick port from Java to C# for its integration in the .NET platform. As many other similar tools targeted to this development platform, Maverick.NET also works with Mono....
- MonoCrossMonoCrossMonoCross is a C# .NET Model-view-controller framework where the Model and Controller are shared across platforms and the View is implemented for every specific platform and/or target architecture. It allows the development of both native and HTML5 web apps that share business logic and data code...
- MonoRail An ActionPack inspired MVC framework from the Castle Project
- Naked Objects MVCNaked Objects MVCNaked Objects MVC is a software framework that builds upon the Microsoft ASP.NET MVC Framework.As the name suggests, the framework synthesises two architectural patterns: naked objects and Model-view-controller . These two patterns have been considered as antithetical...
- PureMVCPureMVCPureMVC is a framework for creating applications based upon the well-established Model, View and Controller design pattern. The free, open source framework was originally implemented in the ActionScript 3 language for use with Adobe Flex, Flash and AIR, and it has since been ported to nearly all...
Framework for C# - Sharp-Architecture
- Spring Framework.NET
- Fubu MVC
Perl
- CatalystCatalyst (software)Catalyst is an open source web application framework written in Perl, that closely follows the model–view–controller architecture, and supports a number of experimental web patterns. It is written using Moose, a modern object system for Perl...
- Mature, stable and fast MVC framework. - CodeCharge StudioCodeCharge StudioCodeCharge Studio is a rapid application development and integrated development environment for creating database-driven web application...
- Dancer - micro web application framework for Perl
- MaypoleMaypole frameworkMaypole is a Perl web application framework for MVC-oriented applications. Maypole is designed to minimize coding requirements for creating simple web interfaces to databases, while remaining flexible enough to support enterprise web applications...
- An MVC Web framework, superseded by CatalystCatalyst (software)Catalyst is an open source web application framework written in Perl, that closely follows the model–view–controller architecture, and supports a number of experimental web patterns. It is written using Moose, a modern object system for Perl...
. - MojoliciousMojoliciousMojolicious is a real-time web application framework, written by Sebastian Riedel, creator of the web application framework Catalyst. Licensed as free software under the Artistic License v 2.0, It is written in Perl, and is designed for use in both simple and complex web applications, based on...
- A web application framework - PureMVCPureMVCPureMVC is a framework for creating applications based upon the well-established Model, View and Controller design pattern. The free, open source framework was originally implemented in the ActionScript 3 language for use with Adobe Flex, Flash and AIR, and it has since been ported to nearly all...
A framework for Perl.
PHP
- AgaviAgaviAgavi is a PHP5 web application framework that follows the Model-view-controller design pattern. It is not using the convention over configuration paradigm, but focuses on design decisions, which allow for better scalability.-External links:**...
is a PHP5 application framework that focuses on sustained quality and correctness. - AlloyAlloy (PHP framework)Alloy is a Hierarchical-Model-View-Controller web application framework for producing web applications with PHP 5.3 and above.-History:Alloy was created by Vance Lucas, and began in October 2009 as the core foundation for Cont-xt CMS, the winning entry in php|architect's PHP on Windows Contest.-See...
A lightweight REST-focused modular Hierarchical MVC PHP 5.3 framework. - AppFlowerAppFlowerAppFlower is an open source application builder for use in building enterprise ready web-based applications. With the aim to make development doable by everyone, doing so with automatic code generations. AppFlower provides a visual designer to easily develop application without prior knowledge of...
is a Rapid Application Development framework based on MVC, PHP5 and Symfony. - https://github.com/auraphp Aura PHP is a component based MVC framework.
- CakePHPCakePHPCakePHP is an open source web application framework for producing web applications. It is written in PHP, modeled after the concepts of Ruby on Rails, and distributed under the MIT License.-History:...
A web application framework modeled after the concepts of Ruby on RailsRuby on RailsRuby on Rails, often shortened to Rails or RoR, is an open source web application framework for the Ruby programming language.-History:...
. - CodeCharge StudioCodeCharge StudioCodeCharge Studio is a rapid application development and integrated development environment for creating database-driven web application...
is a visual rapid application development environment for web-based database driven application development. Code Charge Studio places emphasis on code generation technology to provide ASP.NETASP.NETASP.NET is a Web application framework developed and marketed by Microsoft to allow programmers to build dynamic Web sites, Web applications and Web services. It was first released in January 2002 with version 1.0 of the .NET Framework, and is the successor to Microsoft's Active Server Pages ...
, PHPPHPPHP is a general-purpose server-side scripting language originally designed for web development to produce dynamic web pages. For this purpose, PHP code is embedded into the HTML source document and interpreted by a web server with a PHP processor module, which generates the web page document...
, JSPJavaServer PagesJavaServer Pages is a Java technology that helps software developers serve dynamically generated web pages based on HTML, XML, or other document types...
, Servlets, ColdFusionColdFusionIn computing, ColdFusion is the name of a commercial rapid application development platform invented by Jeremy and JJ Allaire in 1995. ColdFusion was originally designed to make it easier to connect simple HTML pages to a database, by version 2 it had...
and PerlPerlPerl is a high-level, general-purpose, interpreted, dynamic programming language. Perl was originally developed by Larry Wall in 1987 as a general-purpose Unix scripting language to make report processing easier. Since then, it has undergone many changes and revisions and become widely popular...
language support. - AN Framework ANFire is a PHP framework that make it easy to develop build dynamic websites, web applications, and what you need in PHP, JS. (Website)
- CodeIgniter A simple, light, fast, open source MVC framework for building websites using PHP.
- DooPHPDooPHPDooPHP is designed to be a lightweight, low footprint and easy to learn PHP framework.-Key Features:*Built-in authentication support *Database replication*Code Generation*l18n internationalization*RESTfulAPI*Support for Ajax...
- Exponent CMSExponent CMSExponent CMS is a free, open source, open standards modular enterprise software framework and content management system written in the programming language PHP....
A Content Management SystemContent management systemA content management system is a system providing a collection of procedures used to manage work flow in a collaborative environment. These procedures can be manual or computer-based...
web application framework using its own MVC framework modeled after Rails. - eZ PublishEZ publish-External links:* * * * * *...
Based on eZ Components is an object-oriented web application framework written in PHP that separates its functionality into several abstraction layers, following a strict MVC approach. - Feng OfficeFeng OfficeFeng Office is a Software Development Company known for developing Feng Office Collaboration Platform and associated services:* Feng Sky is Feng Office on Demand provided as SaaS* Feng Onsite is Feng Office platform installed in External Servers...
is an open source MVC Framework Extranet that allows a group of a geographically distributed people to collaborate by sharing information over the Internet. - FLOW3 A modern PHP framework (Website)
- Fuel is a modern open source MVC framework, using PHP5.3, combining the best of CodeIgniter, Kohana, Rails & more.
- Joomla is a free and open source content management system (CMS) for publishing content on the World Wide Web and intranets and a model–view–controller (MVC) Web application framework that can also be used independently.
- Kohana FrameworkKohana FrameworkKohana PHP is an HMVC PHP5 framework that provides a rich set of components for quickly building robust and dynamic web applications. It is a relatively lesser known framework when compared to other, such as CakePHP or CodeIgniter, and has a small "strong but elite" community...
v2.x is an open source MVC framework, while v3.x is HMVC (both supported). - NetteNetteNette can refer to:* Nette , a river in Lower Saxony, tributary to the Innerste.* Nette , a river in Rhineland-Palatinate, tributary to the Rhine.* Nette , a river in North Rhine-Westphalia, tributary to the Niers....
Very powerful PHPPHPPHP is a general-purpose server-side scripting language originally designed for web development to produce dynamic web pages. For this purpose, PHP code is embedded into the HTML source document and interpreted by a web server with a PHP processor module, which generates the web page document...
framework with very good performance. - Odin Assemble A PHPPHPPHP is a general-purpose server-side scripting language originally designed for web development to produce dynamic web pages. For this purpose, PHP code is embedded into the HTML source document and interpreted by a web server with a PHP processor module, which generates the web page document...
-based MVC framework with a small footprint. - phpXCorePhpXCorephpXCore is a free open source content management framework for creating customized content management systems written in PHP. phpXCore framework bases on Model-view-controller design pattern and supports both PHP4 and PHP5.-Features:...
An MVC design pattern based PHP content management framework compatible with PHP4 and PHP5. - PureMVCPureMVCPureMVC is a framework for creating applications based upon the well-established Model, View and Controller design pattern. The free, open source framework was originally implemented in the ActionScript 3 language for use with Adobe Flex, Flash and AIR, and it has since been ported to nearly all...
A framework for PHP. - Horde FrameworkHorde (software)Horde is a PHP-based Web application framework.It offers applications such as the Horde IMP email client, a groupware package , a wiki and a time and task tracking software.-Horde Email Platform:...
A framework and application suite for PHP - QcodoQcodoThe Qcodo Development Framework is an open-source PHP web application framework which builds an Object Relational Model , CRUD UI pages, and AJAX hooks from an existing data model. It additionally includes a tightly-integrated HTML and JavaScript form toolkit which interfaces directly with the...
An open-source PHP 5 web application framework. - SleekMVC A fast and lightweight PHP 5 MVC framework, featuring similar syntax to KohanaKohanaKohana may refer to:*Kohana , a captive orca*Kohana, a Kamen Rider Den-O character*Kohana cat, a minor cat breed*Kohana Framework, a PHP 5 framework*Kohana , a local holiday in the Netherlands...
. - Scriptcase Generator A powerful tool to increase web development productivity.
- SilverStripeSilverStripeSilverStripe is a free and open source content management system for creating and maintaining websites. It provides an out of the box web-based administration panel that enables users to make modifications to parts of the website, which includes a WYSIWYG website editor...
A developer friendly CMS with it's own Framework. Use the framework with or without the CMS. (Website) - Switch board (framework)Switch board (framework)Switch Board is a MVC framework written for PHP. Its original concepts were taken from a preexisting ColdFusion/PHP framework called Fusebox. Its evolution through development has greatly changed its structure to have very little resemblance to Fusebox other than slight functionality of the switch,...
A PHP 5 MVC framework with routing. - SIFOSIFOSIFO may refer to:*Sifo, a Swedish company in the area of opinion and social research*National Institute for Consumer Research , a consumer affairs research institute based in Oslo, Norway...
MVC PHP Framework with multiple DB support, SphinxSphinxA sphinx is a mythical creature with a lion's body and a human head or a cat head.The sphinx, in Greek tradition, has the haunches of a lion, the wings of a great bird, and the face of a woman. She is mythicised as treacherous and merciless...
, RedisRedisRedis is used to refer to Romani people.Redis may also refer to:* Redis , an advanced key-value store...
and other stuff. - Solar framework A PHP 5 MVC framework. It is fully name-spaced and uses enterprise application design patterns, with built-in support for localization and configuration at all levels. (Website)
- Symfony Framework A PHP 5 MVC framework modeled after the concepts of Ruby on RailsRuby on RailsRuby on Rails, often shortened to Rails or RoR, is an open source web application framework for the Ruby programming language.-History:...
. - YiiYiiYii is an open source, object-oriented, high-performance component-based PHP web application framework. Yii is pronounced as "Yee" or [ji:] and it's an acronym for "Yes It Is!".- History :...
An open source, object-oriented, high-performance component-based PHP web application framework. - ZanPHP ZanPHP is an agile Web application development framework written in PHP5 that uses different design patterns and best practices to create applications more quickly with good quality code.
- Zend FrameworkZend FrameworkZend Framework is an open source, object-oriented web application framework implemented in PHP 5 and licensed under the New BSD License.-Licensing:...
An open-source PHP 5-based framework featuring a MVC layer and a broad-spectrum of loosely coupled components. - Lightwieght_MVC LightWeight MVC is a Open-Source GPL/Lesser GPL PHP5 Framework that is built to teach users the basics of MVC and implementation of a MVC Powered Web Site.
- TinyMVC FrameworkTinyMVC FrameworkTinyMVC Framework is designed to target a simple and light-weight PHP development framework. It intends to fix drawbacks of current MVC framework and shorten the learning curve of a framework. The TinyMVC is implemented in PHP 5 and licensed under the New BSD License .-Requirement:The framework is...
It is also call TyMVC. It is a light-weight and simple Open-Source (BSD licensed) PHP 5 Model-View-Controller development framework.
Python
- DjangoDjango web frameworkDjango is an open source web application framework, written in Python, which follows the model-view-controller architectural pattern. It was originally developed to manage several news-oriented sites for The World Company of Lawrence, Kansas, and was released publicly under a BSD license in July...
A complete Python web application framework. Django prefers to call its MVC implementation MTV, for model-template-view. - EnthoughtEnthoughtEnthought, Inc. is a software company based in Austin, Texas, USA that develops scientific computing tools using primarily the Python programming language...
The Enthought Tool Suite brings the Model–view–controller mindset to scientific GUIs and visualization - Pylons Python Web Framework
- TurboGearsTurboGearsTurboGears is a Python web application framework consisting of several WSGI components such as Pylons, SQLAlchemy, Genshi and Repoze.TurboGears is designed around the model-view-controller architecture, much like Struts or Ruby on Rails, designed to make rapid web application development in Python...
for Python - web2pyWeb2pyWeb2py is an open source web application framework. Web2py is written in the Python language and is programmable in Python. Since web2py was originally designed as a teaching tool with emphasis on ease of use and deployment, it does not have any project-level configuration files. Web2py was...
An open-source web application framework focussed on rapid development - ZopeZopeZope is a free and open-source, object-oriented Web application server written in the Python programming language. Zope stands for "Z Object Publishing Environment", and was the first system using the now common object publishing methodology for the Web...
Web application server - Plone Content management system built on top of Zope
- PureMVCPureMVCPureMVC is a framework for creating applications based upon the well-established Model, View and Controller design pattern. The free, open source framework was originally implemented in the ActionScript 3 language for use with Adobe Flex, Flash and AIR, and it has since been ported to nearly all...
Framework for Python
Ruby
- Ruby on RailsRuby on RailsRuby on Rails, often shortened to Rails or RoR, is an open source web application framework for the Ruby programming language.-History:...
- SinatraSinatra (software)Sinatra is a free and open source web application library and domain-specific language written in Ruby. It is an alternative to other Ruby web application frameworks such as Ruby on Rails, Merb, Nitro, Camping, and Rango. It is dependent on the Rack web server interface.Designed and developed by...
- MerbMerbMerb, short for "Mongrel+Erb", is a model–view–controller web framework written in Ruby. Merb adopts an approach that focuses on essential core functionality, leaving most functionality to plugins. Merb was merged into Rails web framework on December 23, 2008 as part of the Ruby on Rails 3.0...
- RamazeRamazeRamaze is a web application framework created by Michael Fellinger for Ruby. The philosophy of Ramaze is a mix of KISS and POLS, trying to make complex things simple and impossible things possible....
- CampingCamping (microframework)Camping is a web application framework written in Ruby which consistently stays under 4kb - the complete source code can be viewed on a single page....
- NitroNitro (web framework)Nitro was a Ruby-based web application framework created by George Moschovitis. Nitro featured a powerful template system with a configurable pipeline of transformation steps. It was licensed under a 3-clause BSD license....
- MonkeybarsMonkeybars FrameworkMonkeybars is a library that provides a structured way of building Swing applications using JRuby. Although Monkeybars uses the ideas of models, views, and controllers, its usage of the terms is not the same as in traditional MVC systems...
- PureMVCPureMVCPureMVC is a framework for creating applications based upon the well-established Model, View and Controller design pattern. The free, open source framework was originally implemented in the ActionScript 3 language for use with Adobe Flex, Flash and AIR, and it has since been ported to nearly all...
Framework for Ruby.
XML
- XFormsXFormsXForms is an XML format for the specification of a data processing model for XML data and user interface for the XML data, such as web forms...
—XForms has an integrated Model–view–controller architecture with an integral dependency graph that frees the programmer from specifically having to perform either push or pull operations. - XRXXRX (web application architecture)In software development XRX is a web application architecture based on XForms, REST and XQuery. XRX applications store data on both the web client and on the web server in XML format and do not require a translation between data formats...
-XForms, ReST, XQuery-is a web application framework where server logic is written in XQuery which processes the submitted XForm, and (typically) returns another (often generated on-the-fly) XForm to the client.
See also
- Trygve ReenskaugTrygve ReenskaugTrygve Mikkjel Heyerdahl Reenskaug is a Norwegian computer scientist and professor emeritus of the University of Oslo. He formulated the model-view-controller pattern for Graphic User Interface software design in 1979 while visiting the Xerox Palo Alto Research Center...
- first formulated the MVC pattern - Architectural patternsArchitectural pattern (computer science)An architectural pattern in software is a standard design in the field of software architecture. The concept of a software architectural pattern has a broader scope than the concept of a software design pattern...
- Model-view-presenter
- Model 1Model 1In the design of Java Web applications, there are two commonly used design models, referred to as Model 1 and Model 2.In Model 1, a request is made to a JSP or servlet and then that JSP or servlet handles all responsibilities for the request, including processing the request, validating data,...
- Model 2Model 2In the design of Java Web applications, there are two commonly used design models, referred to as Model 1 and Model 2.Model 1 is a simple pattern whereby the code responsible for the display of content is intermixed with logic. Model 1 is only recommended for small applications and is mostly...
- Three-tier (computing)
- The Observer design patternObserver patternThe observer pattern is a software design pattern in which an object, called the subject, maintains a list of its dependents, called observers, and notifies them automatically of any state changes, usually by calling one of their methods...
- The Template Method design patternTemplate method patternIn software engineering, the template method pattern is a design pattern.It is a behavioral pattern, and is unrelated to C++ templates.-Introduction:A template method defines the program skeleton of an algorithm...
- The Presentation-abstraction-controlPresentation-abstraction-controlPresentation–abstraction–control is a software architectural pattern. It is an interaction-oriented software architecture, and is somewhat similar to model–view–controller in that it separates an interactive system into three types of components responsible for specific aspects of the...
(PAC) pattern - The naked objectsNaked objectsNaked objects is an architectural pattern used in software engineering.-Definition:The naked objects pattern is defined by three principles:...
pattern, often positioned as the antithesis of MVC, though Reenskaug suggests otherwise - Model–view–adapter
- Model View ViewModelModel View ViewModelThe Model View ViewModel is an architectural pattern used in software engineering that originated from Microsoft as a specialization of the Presentation Model design pattern introduced by Martin Fowler...
- Template processorTemplate processorA template processor is software or a software component that is designed to combine one or moretemplates with a data model to produceone or more result documents...
External links
- An overview of the MVC pattern in Java from the Sun website and details of MVC design in Java Swing
- Java SE Application Design With MVC by Robert Eckstein, March 2007
- Model View Presenter with ASP.NET CodeProject article.
- History of the evolution of MVC and derivatives by Martin Fowler.
- Model View Controller in PHP A simple introduction to MVC pattern in PHP.
- Model View Controller - overview page in the Portland Pattern RepositoryPortland Pattern RepositoryThe Portland Pattern Repository is a repository for computer programming design patterns. It was accompanied by a companion website, WikiWikiWeb, which was the world's first wiki....
- Backbone.js A simple introduction to Backbone.js
- Model - Display view - Picking view - Controller (MDPC), an architecture for graphical interactive software aiming at improving further modularity and usability of programming
- Is MVC a design pattern or architectural pattern?, Discussion around MVC as a design pattern.