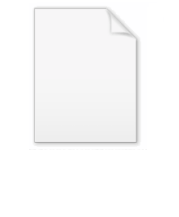
Play Framework
Encyclopedia
Play is an open source
web application framework
, written in Java
, which follows the model-view-controller
architectural pattern
. It aims to optimize developer productivity by using convention over configuration, hot code reloading and display of errors in the browser.
Although Play is written in Java, support for the Scala language has also been available since the 1.1 version. Starting from 2.0, the framework core will be completely rewritten in Scala. Build and deployment will be migrated to Simple Build Tool
and templates
will use Scala instead of Groovy.
and Django. A developer familiar with any of these frameworks will feel at home. Play leverages the power of Java to build web applications in an environment that is not Java Enterprise Edition
-centric. By lifting away the Java EE constraints, Play provides developers with an easy-to-develop and elegant stack aimed at productivity.
Although Play applications are designed to be run using the built-in JBoss Netty
web server, they can also be packaged as WAR files to be distributed to standard Java EE application servers
.
The following functionality is present in the core:
. Tests are run directly in the browser by going to the URL/@tests. By default all testing is done against the included H2
in-memory database.
Some notable public websites using Play:
In December 2010, the first e-book
for the Play framework was released. This was subsequently also published in hard copy
. In August 2011, a second book was released, covering more complex and modern features.
In August 2011, Heroku
announced native support for Play applications on its cloud computing
platform. This follows module-based support for Play on Google App Engine
, and documented support on Amazon Web Services
.
In May 2008 the first published code for 1.0 appeared on Launchpad
. This was followed by a full 1.0 release in October 2009.
Play 1.1 was released in November 2010 after a move from Launchpad to GitHub
. It included a migration from Apache MINA
to JBoss Netty, Scala support, native GlassFish
container, an asynchronous web services library, OAuth
support, HTTPS
support and other features.
Play 1.2 was released in April 2011. It included dependency management with Apache Ivy, support for WebSockets
, integrated database migration (reversion is not implemented yet), a switch to the H2 database and other features.
Open source
The term open source describes practices in production and development that promote access to the end product's source materials. Some consider open source a philosophy, others consider it a pragmatic methodology...
web application framework
Web application framework
A web application framework is a software framework that is designed to support the development of dynamic websites, web applications and web services. The framework aims to alleviate the overhead associated with common activities performed in Web development...
, written in Java
Java (programming language)
Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities...
, which follows the model-view-controller
Model-view-controller
Model–view–controller is a software architecture, currently considered an architectural pattern used in software engineering. The pattern isolates "domain logic" from the user interface , permitting independent development, testing and maintenance of each .Model View Controller...
architectural pattern
Architectural pattern (computer science)
An architectural pattern in software is a standard design in the field of software architecture. The concept of a software architectural pattern has a broader scope than the concept of a software design pattern...
. It aims to optimize developer productivity by using convention over configuration, hot code reloading and display of errors in the browser.
Although Play is written in Java, support for the Scala language has also been available since the 1.1 version. Starting from 2.0, the framework core will be completely rewritten in Scala. Build and deployment will be migrated to Simple Build Tool
Simple Build Tool
Simple Build Tool is an open source build tool for Scala projects written by Mark Harrah that aims to do the basics well.Its main features are:...
and templates
Web template
A web template is a tool used to separate content from presentation in web design, and for mass-production of web documents. It is a basic component of a web template system.Web templates can be used to set up any type of website...
will use Scala instead of Groovy.
Motivation
Play is heavily inspired by Ruby on RailsRuby on Rails
Ruby on Rails, often shortened to Rails or RoR, is an open source web application framework for the Ruby programming language.-History:...
and Django. A developer familiar with any of these frameworks will feel at home. Play leverages the power of Java to build web applications in an environment that is not Java Enterprise Edition
Java Platform, Enterprise Edition
Java Platform, Enterprise Edition or Java EE is widely used platform for server programming in the Java programming language. The Java platform differs from the Java Standard Edition Platform in that it adds libraries which provide functionality to deploy fault-tolerant, distributed, multi-tier...
-centric. By lifting away the Java EE constraints, Play provides developers with an easy-to-develop and elegant stack aimed at productivity.
Although Play applications are designed to be run using the built-in JBoss Netty
JBoss Netty
JBoss Netty is a New I/O client-server framework for the development of Java network applications such as protocol servers and clients. The asynchronous event-driven network application framework and tools is used to simplify network programming such as TCP and UDP socket servers. Netty includes...
web server, they can also be packaged as WAR files to be distributed to standard Java EE application servers
Application server
An application server is a software framework that provides an environment in which applications can run, no matter what the applications are or what they do...
.
Major differences
From other Java frameworks:- Stateless: Play is fully RESTfulRepresentational State TransferRepresentational state transfer is a style of software architecture for distributed hypermedia systems such as the World Wide Web. The term representational state transfer was introduced and defined in 2000 by Roy Fielding in his doctoral dissertation...
- there is no Java EE session per connection. This makes Play more outwardly-scalable than many other frameworks. - No configuration: download, unpack and develop.
- Easy round trips: no need to deploy to an application server, just edit the code and press the refresh button on the browser.
- Integrated unit testing: JUnitJUnitJUnit is a unit testing framework for the Java programming language. JUnit has been important in the development of test-driven development, and is one of a family of unit testing frameworks collectively known as xUnit that originated with SUnit....
and SeleniumSelenium (software)Selenium is a portable software testing framework for web applications. Selenium provides a record/playback tool for authoring tests without learning a test scripting language . It also provides a test domain-specific language to write tests in a number of popular programming languages, including...
support is included in the core. - Elegant APIApplication programming interfaceAn application programming interface is a source code based specification intended to be used as an interface by software components to communicate with each other...
: rarely will a developer need to import any third party library - Play comes with all the typical stuff built-in. - Static methods: all controller entry points and business logic methods are declared as static. This is very different from what can be seen in other Java frameworks.
- Asynchronous I/OAsynchronous I/OAsynchronous I/O, or non-blocking I/O, is a form of input/output processing that permits other processing to continue before the transmission has finished....
: due to using JBoss Netty as its web server, Play can service long requests asynchronously rather than tying up HTTP threads doing business logic like Java EE frameworks that don't use the asynchronous support offered by Servlet 3.0. - Modular architecture: like Rails and Django, Play comes with the concept of modules. These provide an elegant and simple way to expand the core.
- CRUD module: easily build administration UI with little code.
- Scala module: provides complete support with a Scala-specific database access layer (Anorm) and templating language.
Components
Play makes use of several popular Java libraries:- JBoss Netty for the web server
- HibernateHibernate (Java)Hibernate is an object-relational mapping library for the Java language, providing a framework for mapping an object-oriented domain model to a traditional relational database...
for the data layer - Groovy for the template engine
- The EclipseEclipse (software)Eclipse is a multi-language software development environment comprising an integrated development environment and an extensible plug-in system...
compiler for hot-reloading - Apache IvyApache IvyApache Ivy is a transitive relation dependency manager. It is a sub-project of the Apache Ant project, with which Ivy works to resolve project dependencies. An external XML file defines project dependencies and lists the resources necessary to build a project...
for dependency management
The following functionality is present in the core:
- a clean, RESTful framework
- CRUD: a module to simplify editing of model objects
- Secure: a module to enable simple user authentication
- a validation framework based on annotationsJava annotationAn annotation, in the Java computer programming language, is a special form of syntactic metadata that can be added to Java source code. Classes, methods, variables, parameters and packages may be annotated...
- a job scheduler
- a simple to use SMTPSimple Mail Transfer ProtocolSimple Mail Transfer Protocol is an Internet standard for electronic mail transmission across Internet Protocol networks. SMTP was first defined by RFC 821 , and last updated by RFC 5321 which includes the extended SMTP additions, and is the protocol in widespread use today...
mailer - JSONJSONJSON , or JavaScript Object Notation, is a lightweight text-based open standard designed for human-readable data interchange. It is derived from the JavaScript scripting language for representing simple data structures and associative arrays, called objects...
and XMLXMLExtensible Markup Language is a set of rules for encoding documents in machine-readable form. It is defined in the XML 1.0 Specification produced by the W3C, and several other related specifications, all gratis open standards....
parsers and marshalers - a persistence layer based on JPAJava Persistence APIThe Java Persistence API, sometimes referred to as JPA, is a Java programming language framework managing relational data in applications using Java Platform, Standard Edition and Java Platform, Enterprise Edition....
- an embedded database for quick deployment/testing purposes
- a full embedded testing framework
- an automatic file uploads functionality
- multi-environment configuration awareness
- a powerful template engine based on Groovy with templates, hierarchy and tags
- a modular architecture, which enables bringing new features in the core easily
- OpenIDOpenIDOpenID is an open standard that describes how users can be authenticated in a decentralized manner, eliminating the need for services to provide their own ad hoc systems and allowing users to consolidate their digital identities...
and web services clients
Testing framework
Play provides a built-in test framework for unit testing and functional testingFunctional testing
Functional testing is a type of black box testing that bases its test cases on the specifications of the software component under test. Functions are tested by feeding them input and examining the output, and internal program structure is rarely considered .Functional testing differs from system...
. Tests are run directly in the browser by going to the URL
H2 (DBMS)
H2 is a relational database management system written in Java. It can be embedded in Java applications or run in the client-server mode. The disk footprint is about 1 MB....
in-memory database.
Usage
The mailing list for the project has over 3,000 subscribers. It is used in various projects such as local governments, company intranets, mobile web sites and Open Source projects.Some notable public websites using Play:
- http://gendi.fr/, GENDI, a GS1GS1Founded in 1977, GS1 is an international not-for-profit association dedicated to the development and implementation of global standards and solutions to improve the efficiency and visibility of supply and demand chains globally and across multiple sectors...
member. - http://jobs.siliconsentier.org/, Jobs board of Silicon Sentier.
- http://www.seine-et-marne.fr/, General council website of Seine-et-MarneSeine-et-MarneSeine-et-Marne is a French department, named after the Seine and Marne rivers, and located in the Île-de-France region.- History:Seine-et-Marne is one of the original 83 departments, created on March 4, 1790 during the French Revolution in application of the law of December 22, 1789...
, France. - http://www.guardian.co.uk/, Guardian.co.ukGuardian.co.ukguardian.co.uk, formerly known as Guardian Unlimited, is a British website owned by the Guardian Media Group. Georgina Henry is the editor...
, online news resource. - http://typesafe.com/, corporate website for Scala company founded by Martin OderskyMartin OderskyMartin Odersky is a German computer scientist and professor of programming methods at the EPFL. He specialises in code analysis and programming languages.In 1989 Odersky received his Ph.D...
.
In December 2010, the first e-book
E-book
An electronic book is a book-length publication in digital form, consisting of text, images, or both, and produced on, published through, and readable on computers or other electronic devices. Sometimes the equivalent of a conventional printed book, e-books can also be born digital...
for the Play framework was released. This was subsequently also published in hard copy
Hard copy
In information handling, a hard copy is a permanent reproduction, or copy, in the form of a physical object, of any media suitable for direct use by a person , of displayed or transmitted data...
. In August 2011, a second book was released, covering more complex and modern features.
In August 2011, Heroku
Heroku
Heroku is a cloud Platform as a Service run by the San Francisco, California-based company with the same name. Heroku led the way for a multi-language PaaS, introducing the 'polyglot platform'. Heroku initially supported the Ruby programming language, with Rack and Ruby on Rails. Heroku PaaS now...
announced native support for Play applications on its cloud computing
Cloud computing
Cloud computing is the delivery of computing as a service rather than a product, whereby shared resources, software, and information are provided to computers and other devices as a utility over a network ....
platform. This follows module-based support for Play on Google App Engine
Google App Engine
Google App Engine is a platform as a service cloud computing platform for developing and hosting web applications in Google-managed data centers. It virtualizes applications across multiple servers,...
, and documented support on Amazon Web Services
Amazon Web Services
Amazon Web Services is a collection of remote computing services that together make up a cloud computing platform, offered over the Internet by Amazon.com...
.
History
Play was created by software developer Guillaume Bort, while working at Zenexity. Although the early releases are no longer available online, there is evidence of Play existing as far back as May 2007. In 2007 pre-release versions of the project were available to download from Zenexity's website.In May 2008 the first published code for 1.0 appeared on Launchpad
Launchpad (website)
Launchpad is a web application and website that allow users to develop and maintain software, particularly free software. Launchpad is developed and maintained by Canonical Ltd....
. This was followed by a full 1.0 release in October 2009.
Play 1.1 was released in November 2010 after a move from Launchpad to GitHub
Github
GitHub is a web-based hosting service for software development projects that use the Git revision control system. GitHub offers both commercial plans and free accounts for open source projects...
. It included a migration from Apache MINA
Apache MINA
Apache MINA is an open source Java network application framework. MINA can be used to create scalable, high performance network applications. MINA provides unified API's for various transports like TCP, UDP, serial communication. It also makes it easy to make an implementation of custom transport...
to JBoss Netty, Scala support, native GlassFish
GlassFish
GlassFish is an open source application server project started by Sun Microsystems for the Java EE platform and now sponsored by Oracle Corporation. The supported version is called Oracle GlassFish Server...
container, an asynchronous web services library, OAuth
OAuth
OAuth is an open standard for authorization. It allows users to share their private resources stored on one site with another site without having to hand out their credentials, typically username and password.OAuth allows users to hand out tokens instead of credentials to their data hosted by a...
support, HTTPS
Https
Hypertext Transfer Protocol Secure is a combination of the Hypertext Transfer Protocol with SSL/TLS protocol to provide encrypted communication and secure identification of a network web server...
support and other features.
Play 1.2 was released in April 2011. It included dependency management with Apache Ivy, support for WebSockets
WebSockets
WebSocket is a technology providing for bi-directional, full-duplex communications channels, over a single Transmission Control Protocol socket. It is designed to be implemented in web browsers and web servers, but it can be used by any client or server application...
, integrated database migration (reversion is not implemented yet), a switch to the H2 database and other features.
External links
- Play home page
- Source code
- Discussion group
- Bug tracker
- Stackoverflow
- Planet Play, aggregation of blogs from the community