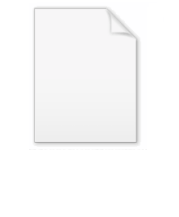
Method (computer science)
Encyclopedia
In object-oriented programming
, a method is a subroutine
(or procedure or function) associated with a class
. Methods define the behavior to be exhibited by instances of the associated class at program run time. Methods have the special property that at runtime, they have access to data stored in an instance of the class (or class instance or class object or object) they are associated with and are thereby able to control the state of the instance. The association between class and method is called binding. A method associated with a class is said to be bound to the class. Methods can be bound to a class at compile time (static binding) or to an object at runtime (dynamic binding
).
body. It is often used to specify that a subclass must provide an implementation of the method. Abstract methods are used to specify interfaces
in some computer languages.
of an object to be accessed (retrieved) from other parts of a program.
An update, modifier, or mutator
method, is an accessor method that changes the state of an object. Objects that provide such methods are considered mutable objects.
, is a class method that is called automatically at the beginning of an object's lifetime to initialize the object, a process called construction (or instantiation). Initialization may include acquisition of resources. A language may provide a means to control whether a constructor can be called implicitly (by the compiler) or only explicitly (by the programmer). Constructors may have parameters but usually do not return values in most languages.
is a class method that is called automatically at the end of an object's lifetime, a process called destruction
. Destructors in most languages do not allow destructor method arguments nor return values. (In some languages (ref required), a destructor can return a value which can then be used to obtain a public representation (transfer encoding) of an instance of a class and simultaneously destroy the copy of the instance stored in current thread's memory.) Destructors can be implemented such as to perform clean up chores and other tasks at object destruction.
Object-oriented programming
Object-oriented programming is a programming paradigm using "objects" – data structures consisting of data fields and methods together with their interactions – to design applications and computer programs. Programming techniques may include features such as data abstraction,...
, a method is a subroutine
Subroutine
In computer science, a subroutine is a portion of code within a larger program that performs a specific task and is relatively independent of the remaining code....
(or procedure or function) associated with a class
Class (computer science)
In object-oriented programming, a class is a construct that is used as a blueprint to create instances of itself – referred to as class instances, class objects, instance objects or simply objects. A class defines constituent members which enable these class instances to have state and behavior...
. Methods define the behavior to be exhibited by instances of the associated class at program run time. Methods have the special property that at runtime, they have access to data stored in an instance of the class (or class instance or class object or object) they are associated with and are thereby able to control the state of the instance. The association between class and method is called binding. A method associated with a class is said to be bound to the class. Methods can be bound to a class at compile time (static binding) or to an object at runtime (dynamic binding
Dynamic dispatch
In computer science, dynamic dispatch is the process of mapping a message to a specific sequence of code at runtime. This is done to support the cases where the appropriate method can't be determined at compile-time...
).
Abstract methods
An abstract method is one with only a signature and no implementationImplementation
Implementation is the realization of an application, or execution of a plan, idea, model, design, specification, standard, algorithm, or policy.-Computer Science:...
body. It is often used to specify that a subclass must provide an implementation of the method. Abstract methods are used to specify interfaces
Interface (computer science)
In the field of computer science, an interface is a tool and concept that refers to a point of interaction between components, and is applicable at the level of both hardware and software...
in some computer languages.
Accessor and mutator methods
An 'accessor' method is a method that is usually small, simple and provides the sole means for the stateState (computer science)
In computer science and automata theory, a state is a unique configuration of information in a program or machine. It is a concept that occasionally extends into some forms of systems programming such as lexers and parsers....
of an object to be accessed (retrieved) from other parts of a program.
- Although this introduces a new dependency, as stated above, use of methods is preferred, in the object-oriented paradigm, to directly accessing state data - because those methods provide an abstraction layerAbstraction layerAn abstraction layer is a way of hiding the implementation details of a particular set of functionality...
. For example, if a bank-account class provides agetBalance
accessor method to retrieve the current balanceBalance (accounting)In banking and accountancy, the outstanding balance is the amount of money owed, , that remains in a deposit account at a given date, after all past remittances, payments and withdrawal have been accounted for. It can be positive or negative ....
(rather than directly accessing the balance data fields), then later revisionRevisionRevision is the process of revising.More specifically, it may refer to:* Update, a modification of software or a database* Revision control, the management of changes to sets of computer files* Belief revision...
s of the same code can implement a more complex mechanism for balance retrieval (say, a databaseDatabaseA database is an organized collection of data for one or more purposes, usually in digital form. The data are typically organized to model relevant aspects of reality , in a way that supports processes requiring this information...
fetch), without the dependent code needing to be changed (However, this often claimed advantage is not unique to object oriented programming, and was earlier implemented - when desirable in critical systems - through conventional modular programming with optional run-time, system-wide locking mechanisms, in the imperative/procedural paradigms)
- To compare the value of two data items, two accessor-method calls are normally required before a comparison can take place between the retrieved primitive data type values. Comparator methods are required to compare entire objects for equality. This contrasts with a direct comparison in non-OOP paradigms.
An update, modifier, or mutator
Mutator method
In computer science, a mutator method is a method used to control changes to a variable.The mutator method, sometimes called a "setter", is most often used in object-oriented programming, in keeping with the principle of encapsulation...
method, is an accessor method that changes the state of an object. Objects that provide such methods are considered mutable objects.
Class methods
Class methods are methods that are called on a class (compare this to class instance methods, or object methods). Its meaning may vary depending on the programming language:- In some languages (e.g. C++C++C++ is a statically typed, free-form, multi-paradigm, compiled, general-purpose programming language. It is regarded as an intermediate-level language, as it comprises a combination of both high-level and low-level language features. It was developed by Bjarne Stroustrup starting in 1979 at Bell...
, JavaJava (programming language)Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities...
), class methods are synonymous with static methods (see section below), which are called with a known class name at compile-time.this
cannot be used in static methods. - In some other languages (e.g. SmalltalkSmalltalkSmalltalk is an object-oriented, dynamically typed, reflective programming language. Smalltalk was created as the language to underpin the "new world" of computing exemplified by "human–computer symbiosis." It was designed and created in part for educational use, more so for constructionist...
, RubyRuby (programming language)Ruby is a dynamic, reflective, general-purpose object-oriented programming language that combines syntax inspired by Perl with Smalltalk-like features. Ruby originated in Japan during the mid-1990s and was first developed and designed by Yukihiro "Matz" Matsumoto...
, Objective-CObjective-CObjective-C is a reflective, object-oriented programming language that adds Smalltalk-style messaging to the C programming language.Today, it is used primarily on Apple's Mac OS X and iOS: two environments derived from the OpenStep standard, though not compliant with it...
), class methods are methods that are called on a class object, which can be computed at runtime, there being no difference between calling a method on a regular object or a class object; thus both instance and class methods are resolved dynamically, and there are no "static" methods. Notably, in these class methods,this
refers to the class object. - Some languages have both. For example, in PythonPython (programming language)Python is a general-purpose, high-level programming language whose design philosophy emphasizes code readability. Python claims to "[combine] remarkable power with very clear syntax", and its standard library is large and comprehensive...
, one can create class methods and static methods using theclassmethod
andstaticmethod
decorators, respectively. The former has access tothis
, while the latter does not.
Conversion operator methods
A conversion operator provides a means for the compiler to implicitly (performed by the compiler automatically when appropriate) provide an object of a type other than the type of the class object.Operator methods
Operator methods define or redefine operator symbols and define the operations to be performed with the symbol and the associated method parameters.Overloaded methods
Overloaded methods are those with the same name but different formal parameters or return value type, if the language supports overloading on return type.Overridden methods
Overridden methods are those that are redefined in a subclass and hide methods of a superclass.Special methods
Special methods are very language-specific and a language may support none, some, or all of the special methods defined here. A language's compiler may automatically generate default special methods or a programmer may be allowed to optionally define special methods. Most special methods cannot be directly called, but rather the compiler generates code to call them at appropriate times. The syntax for definition and calling (i.e., when a special method can be called) of special methods varies amongst programming languages.Constructors
A constructorConstructor (computer science)
In object-oriented programming, a constructor in a class is a special type of subroutine called at the creation of an object. It prepares the new object for use, often accepting parameters which the constructor uses to set any member variables required when the object is first created...
, is a class method that is called automatically at the beginning of an object's lifetime to initialize the object, a process called construction (or instantiation). Initialization may include acquisition of resources. A language may provide a means to control whether a constructor can be called implicitly (by the compiler) or only explicitly (by the programmer). Constructors may have parameters but usually do not return values in most languages.
Destructors
A destructorDestructor (computer science)
In object-oriented programming, a destructor is a method which is automatically invoked when the object is destroyed...
is a class method that is called automatically at the end of an object's lifetime, a process called destruction
Object lifetime
In computer science, the object lifetime of an object in object-oriented programming is the time between an object's creation till the object is no longer used, and is destructed or freed.In object-oriented programming , the meaning of creating objects is far more subtle than simple...
. Destructors in most languages do not allow destructor method arguments nor return values. (In some languages (ref required), a destructor can return a value which can then be used to obtain a public representation (transfer encoding) of an instance of a class and simultaneously destroy the copy of the instance stored in current thread's memory.) Destructors can be implemented such as to perform clean up chores and other tasks at object destruction.
Copy-assignment operators
Copy-assignment operators define actions to be performed by the compiler when a class object is assigned to a class object of the same type.Static methods
Static methods neither require an instance of the class nor can they implicitly access the data (orthis
, self
, Me
, etc.) of such an instance. A static method is distinguished in some programming languages with the static
keyword placed somewhere in the method's signature. Static methods are called "static" because they are resolved statically (i.e. at compile time) based on the class they are called on; and not dynamically, as in the case with instance methods which are resolved polymorphically based on the runtime type of the object. Therefore, static methods cannot be overridden.