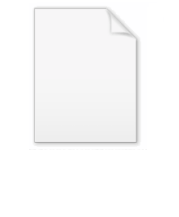
Interpreted language
Encyclopedia
Interpreted language is a programming language in which program
s are 'indirectly' executed ("interpreted") by an interpreter program
. This can be contrasted with a compiled language which is converted into machine code
and then 'directly' executed by the host CPU. Theoretically, any language may be compiled
or interpreted, so this designation is applied purely because of common implementation practice and not some essential property of a language. Indeed, for some programming languages, there is little performance difference between an interpretive- or compiled-based approach to their implementation.
Many languages have been implemented using both compilers and interpreters, including BASIC
, C
, Lisp, Pascal
, and Python
. While Java
is translated to a form that is intended to be interpreted, just-in-time compilation
is often used to generate machine code. The Microsoft
.NET Framework
languages always compile to Common Intermediate Language
(CIL) which is then just-in-time compiled into native machine code. Many Lisp implementations can freely mix interpreted and compiled code. These implementations also use a compiler that can translate arbitrary source code at runtime to machine code.
at the time it is declared or first used while some interpreted languages take advantage of the dynamic aspects of interpreting to make such declarations unnecessary. For example, Smalltalk
(1980), which was designed to be interpreted at run-time, allows generic objects to dynamically interact with each other.
Initially, interpreted languages were compiled line-by-line; that is, each line was compiled as it was about to be executed, and if a loop or subroutine caused certain lines to be executed multiple times, they would be recompiled every time. This has become much less common. Most so-called interpreted languages use an intermediate representation, which combines compiling and interpreting. In this case, a compiler may output some form of bytecode
or threaded code
, which is then executed by a bytecode interpreter.
Examples include:
The intermediate representation can be compiled once and for all (as in Java), each time before execution (as in Perl or Ruby), or each time a change in the source is detected before execution (as in Python).
execution on the host CPU. A technique used to improve performance is just-in-time compilation
which converts frequently executed sequences of interpreted instruction to host machine code.
code, which is then either interpreted or compiled at runtime to native code.
Computer program
A computer program is a sequence of instructions written to perform a specified task with a computer. A computer requires programs to function, typically executing the program's instructions in a central processor. The program has an executable form that the computer can use directly to execute...
s are 'indirectly' executed ("interpreted") by an interpreter program
Interpreter (computing)
In computer science, an interpreter normally means a computer program that executes, i.e. performs, instructions written in a programming language...
. This can be contrasted with a compiled language which is converted into machine code
Machine code
Machine code or machine language is a system of impartible instructions executed directly by a computer's central processing unit. Each instruction performs a very specific task, typically either an operation on a unit of data Machine code or machine language is a system of impartible instructions...
and then 'directly' executed by the host CPU. Theoretically, any language may be compiled
Compiler
A compiler is a computer program that transforms source code written in a programming language into another computer language...
or interpreted, so this designation is applied purely because of common implementation practice and not some essential property of a language. Indeed, for some programming languages, there is little performance difference between an interpretive- or compiled-based approach to their implementation.
Many languages have been implemented using both compilers and interpreters, including BASIC
BASIC
BASIC is a family of general-purpose, high-level programming languages whose design philosophy emphasizes ease of use - the name is an acronym from Beginner's All-purpose Symbolic Instruction Code....
, C
C (programming language)
C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system....
, Lisp, Pascal
Pascal (programming language)
Pascal is an influential imperative and procedural programming language, designed in 1968/9 and published in 1970 by Niklaus Wirth as a small and efficient language intended to encourage good programming practices using structured programming and data structuring.A derivative known as Object Pascal...
, and Python
Python (programming language)
Python is a general-purpose, high-level programming language whose design philosophy emphasizes code readability. Python claims to "[combine] remarkable power with very clear syntax", and its standard library is large and comprehensive...
. While Java
Java (programming language)
Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities...
is translated to a form that is intended to be interpreted, just-in-time compilation
Just-in-time compilation
In computing, just-in-time compilation , also known as dynamic translation, is a method to improve the runtime performance of computer programs. Historically, computer programs had two modes of runtime operation, either interpreted or static compilation...
is often used to generate machine code. The Microsoft
Microsoft
Microsoft Corporation is an American public multinational corporation headquartered in Redmond, Washington, USA that develops, manufactures, licenses, and supports a wide range of products and services predominantly related to computing through its various product divisions...
.NET Framework
.NET Framework
The .NET Framework is a software framework that runs primarily on Microsoft Windows. It includes a large library and supports several programming languages which allows language interoperability...
languages always compile to Common Intermediate Language
Common Intermediate Language
Common Intermediate Language is the lowest-level human-readable programming language defined by the Common Language Infrastructure specification and is used by the .NET Framework and Mono...
(CIL) which is then just-in-time compiled into native machine code. Many Lisp implementations can freely mix interpreted and compiled code. These implementations also use a compiler that can translate arbitrary source code at runtime to machine code.
Historical background of interpreted/compiled
In the early days of computing, language design was heavily influenced by the decision to use compiling or interpreting as a mode of execution. For example, some compiled languages require that programs must explicitly state the data-type of a variableVariable (programming)
In computer programming, a variable is a symbolic name given to some known or unknown quantity or information, for the purpose of allowing the name to be used independently of the information it represents...
at the time it is declared or first used while some interpreted languages take advantage of the dynamic aspects of interpreting to make such declarations unnecessary. For example, Smalltalk
Smalltalk
Smalltalk is an object-oriented, dynamically typed, reflective programming language. Smalltalk was created as the language to underpin the "new world" of computing exemplified by "human–computer symbiosis." It was designed and created in part for educational use, more so for constructionist...
(1980), which was designed to be interpreted at run-time, allows generic objects to dynamically interact with each other.
Initially, interpreted languages were compiled line-by-line; that is, each line was compiled as it was about to be executed, and if a loop or subroutine caused certain lines to be executed multiple times, they would be recompiled every time. This has become much less common. Most so-called interpreted languages use an intermediate representation, which combines compiling and interpreting. In this case, a compiler may output some form of bytecode
Bytecode
Bytecode, also known as p-code , is a term which has been used to denote various forms of instruction sets designed for efficient execution by a software interpreter as well as being suitable for further compilation into machine code...
or threaded code
Threaded code
In computer science, the term threaded code refers to a compiler implementation technique where the generated code has a form that essentially consists entirely of calls to subroutines...
, which is then executed by a bytecode interpreter.
Examples include:
- JavaJava (programming language)Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities...
- PythonPython (programming language)Python is a general-purpose, high-level programming language whose design philosophy emphasizes code readability. Python claims to "[combine] remarkable power with very clear syntax", and its standard library is large and comprehensive...
- RubyRuby (programming language)Ruby is a dynamic, reflective, general-purpose object-oriented programming language that combines syntax inspired by Perl with Smalltalk-like features. Ruby originated in Japan during the mid-1990s and was first developed and designed by Yukihiro "Matz" Matsumoto...
(similarly, it uses an abstract syntax treeAbstract syntax treeIn computer science, an abstract syntax tree , or just syntax tree, is a tree representation of the abstract syntactic structure of source code written in a programming language. Each node of the tree denotes a construct occurring in the source code. The syntax is 'abstract' in the sense that it...
as intermediate representation)
The intermediate representation can be compiled once and for all (as in Java), each time before execution (as in Perl or Ruby), or each time a change in the source is detected before execution (as in Python).
Advantages of interpreting a language
Interpreting a language gives implementations some additional flexibility over compiled implementations. Features that are often easier to implement in interpreters than in compilers include (but are not limited to):- platformPlatform (computing)A computing platform includes some sort of hardware architecture and a software framework , where the combination allows software, particularly application software, to run...
independence (Java's byte code, for example) - reflectionReflection (computer science)In computer science, reflection is the process by which a computer program can observe and modify its own structure and behavior at runtime....
and reflective use of the evaluator (e.g. a first-order evalEvalIn some programming languages, eval is a function which evaluates a string as though it were an expression and returns a result; in others, it executes multiple lines of code as though they had been included instead of the line including the eval...
function) - dynamic typing
- smaller executable program size (since implementations have flexibility to choose the instruction code)
- dynamic scoping
Disadvantages of interpreted languages
The main disadvantage of interpreting is a much slower speed of program execution compared to direct machine codeMachine code
Machine code or machine language is a system of impartible instructions executed directly by a computer's central processing unit. Each instruction performs a very specific task, typically either an operation on a unit of data Machine code or machine language is a system of impartible instructions...
execution on the host CPU. A technique used to improve performance is just-in-time compilation
Just-in-time compilation
In computing, just-in-time compilation , also known as dynamic translation, is a method to improve the runtime performance of computer programs. Historically, computer programs had two modes of runtime operation, either interpreted or static compilation...
which converts frequently executed sequences of interpreted instruction to host machine code.
List of frequently interpreted languages
- APL A vector oriented language using an unusual character set
- JJ (programming language)The J programming language, developed in the early 1990s by Kenneth E. Iverson and Roger Hui, is a synthesis of APL and the FP and FL function-level languages created by John Backus....
An APL variant in which tacit definition provides some of the benefits of compiling
- J
- ASPActive Server PagesActive Server Pages , also known as Classic ASP or ASP Classic, was Microsoft's first server-side script engine for dynamically-generated Web pages. Initially released as an add-on to Internet Information Services via the Windows NT 4.0 Option Pack Active Server Pages (ASP), also known as Classic...
Web page scripting language - BASICBASICBASIC is a family of general-purpose, high-level programming languages whose design philosophy emphasizes ease of use - the name is an acronym from Beginner's All-purpose Symbolic Instruction Code....
(although the original version, Dartmouth BASIC, was compiled, as are many modern BASICs)- thinBasicThinBasicthinBasic is a BASIC-like computer programming language interpreter with a central core engine architecture surrounded by many specialized modules...
- thinBasic
- COBOLCOBOLCOBOL is one of the oldest programming languages. Its name is an acronym for COmmon Business-Oriented Language, defining its primary domain in business, finance, and administrative systems for companies and governments....
- ECMAScriptECMAScriptECMAScript is the scripting language standardized by Ecma International in the ECMA-262 specification and ISO/IEC 16262. The language is widely used for client-side scripting on the web, in the form of several well-known dialects such as JavaScript, JScript, and ActionScript.- History :JavaScript...
- ActionScriptActionScriptActionScript is an object-oriented language originally developed by Macromedia Inc. . It is a dialect of ECMAScript , and is used primarily for the development of websites and software targeting the Adobe Flash Player platform, used on Web pages in the form of...
(version 3.0 is not interpreted, that's why eval function was removed) - E4XE4XECMAScript for XML is a programming language extension that adds native XML support to ECMAScript . The goal is to provide an alternative to DOM interfaces that uses a simpler syntax for accessing XML documents. It also offers a new way of making XML visible...
- JavaScriptJavaScriptJavaScript is a prototype-based scripting language that is dynamic, weakly typed and has first-class functions. It is a multi-paradigm language, supporting object-oriented, imperative, and functional programming styles....
(first named Mocha, then LiveScript) - JScriptJScriptJScript is a scripting language based on the ECMAScript standard that is used in Microsoft's Internet Explorer.JScript is implemented as a Windows Script engine. This means that it can be "plugged in" to any application that supports Windows Script, such as Internet Explorer, Active Server Pages,...
- ActionScript
- Equation manipulation and solving systems
- GNU OctaveGNU OctaveGNU Octave is a high-level language, primarily intended for numerical computations. It provides a convenient command-line interface for solving linear and nonlinear problems numerically, and for performing other numerical experiments using a language that is mostly compatible with MATLAB...
- Interactive Data Language (IDL)
- MathematicaMathematicaMathematica is a computational software program used in scientific, engineering, and mathematical fields and other areas of technical computing...
- MATLABMATLABMATLAB is a numerical computing environment and fourth-generation programming language. Developed by MathWorks, MATLAB allows matrix manipulations, plotting of functions and data, implementation of algorithms, creation of user interfaces, and interfacing with programs written in other languages,...
- GNU Octave
- Euphoria Interpreted or compiled.
- Forth (traditionally threadedThreaded codeIn computer science, the term threaded code refers to a compiler implementation technique where the generated code has a form that essentially consists entirely of calls to subroutines...
interpreted) - Game Maker LanguageGame Maker LanguageGame Maker Language is a scripting language developed for use with a computer game creation application called Game Maker. It was originally created by Mark Overmars to supplement the drag-and-drop action system used in Game Maker...
- Lava
- Madness Script
- PerlPerlPerl is a high-level, general-purpose, interpreted, dynamic programming language. Perl was originally developed by Larry Wall in 1987 as a general-purpose Unix scripting language to make report processing easier. Since then, it has undergone many changes and revisions and become widely popular...
- PHPPHPPHP is a general-purpose server-side scripting language originally designed for web development to produce dynamic web pages. For this purpose, PHP code is embedded into the HTML source document and interpreted by a web server with a PHP processor module, which generates the web page document...
- PostScriptPostScriptPostScript is a dynamically typed concatenative programming language created by John Warnock and Charles Geschke in 1982. It is best known for its use as a page description language in the electronic and desktop publishing areas. Adobe PostScript 3 is also the worldwide printing and imaging...
- PythonPython (programming language)Python is a general-purpose, high-level programming language whose design philosophy emphasizes code readability. Python claims to "[combine] remarkable power with very clear syntax", and its standard library is large and comprehensive...
- Lisp
- LogoLogo (programming language)Logo is a multi-paradigm computer programming language used in education. It is an adaptation and dialect of the Lisp language; some have called it Lisp without the parentheses. It was originally conceived and written as functional programming language, and drove a mechanical turtle as an output...
- Scheme
- Logo
- MUMPSMUMPSMUMPS , or alternatively M, is a programming language created in the late 1960s, originally for use in the healthcare industry. It was designed for the production of multi-user database-driven applications...
(traditionally interpreted, modern versions compiled) - REXXREXXREXX is an interpreted programming language that was developed at IBM. It is a structured high-level programming language that was designed to be both easy to learn and easy to read...
- RubyRuby (programming language)Ruby is a dynamic, reflective, general-purpose object-oriented programming language that combines syntax inspired by Perl with Smalltalk-like features. Ruby originated in Japan during the mid-1990s and was first developed and designed by Yukihiro "Matz" Matsumoto...
- JRubyJRubyJRuby is a Java implementation of the Ruby programming language, being developed by the JRuby team. It is free software released under a three-way CPL/GPL/LGPL license...
(A Java implementation of Ruby)
- JRuby
- SmalltalkSmalltalkSmalltalk is an object-oriented, dynamically typed, reflective programming language. Smalltalk was created as the language to underpin the "new world" of computing exemplified by "human–computer symbiosis." It was designed and created in part for educational use, more so for constructionist...
(pure object-orientation, originally from Xerox PARCXerox PARCPARC , formerly Xerox PARC, is a research and co-development company in Palo Alto, California, with a distinguished reputation for its contributions to information technology and hardware systems....
, often supports debugging across machines.)- BistroBistro (programming language)The Bistro programming language is object oriented, dynamically typed, and reflective. It is intended to integrate features of Smalltalk and Java, running as a variant of Smalltalk that runs atop any Java virtual machine conforming to Sun Microsystems' Java specification...
- Dolphin SmalltalkDolphin SmalltalkDolphin Smalltalk, or "Dolphin" for short , is an implementation of the Smalltalk programming language by Object Arts, targeted at the Microsoft Windows platform.The last major release was Dolphin Smalltalk X6, which comes in two versions:...
- F-Script
- Little SmalltalkLittle SmalltalkLittle Smalltalk is a non-standard dialect of the Smalltalk programming language invented by Timothy Budd. It was originally described in the book: "A Little Smalltalk", Timothy Budd, Addison-Wesley, 1987, ISBN 0-201-10698-1....
- SqueakSqueakThe Squeak programming language is a Smalltalk implementation. It is object-oriented, class-based and reflective.It was derived directly from Smalltalk-80 by a group at Apple Computer that included some of the original Smalltalk-80 developers...
- VisualAgeVisualAgeVisualAge was the name of a family of computer integrated development environments from IBM, which included support for multiple programming languages. VisualAge was first released in the 1980s and is still available in 2011...
- VisualWorksVisualWorksVisualWorks is a cross-platform implementation of the Smalltalk language. It is implemented as a development system based on "images", which are dynamic collections of software objects, each contained in a system image....
- Bistro
- Scripting languageScripting languageA scripting language, script language, or extension language is a programming language that allows control of one or more applications. "Scripts" are distinct from the core code of the application, as they are usually written in a different language and are often created or at least modified by the...
s- WebDNAWebDNAWebDNA is a server-side scripting, interpreted language containing an optional embedded, ram-resident, proprietary, searchable data structure. It has the ability to connect directly to other SQL servers if desired. Its primary use is in creating dynamic web page applications competing against the...
- WebDNA
- SpreadsheetSpreadsheetA spreadsheet is a computer application that simulates a paper accounting worksheet. It displays multiple cells usually in a two-dimensional matrix or grid consisting of rows and columns. Each cell contains alphanumeric text, numeric values or formulas...
s- ExcelMicrosoft ExcelMicrosoft Excel is a proprietary commercial spreadsheet application written and distributed by Microsoft for Microsoft Windows and Mac OS X. It features calculation, graphing tools, pivot tables, and a macro programming language called Visual Basic for Applications...
stores formulas, interprets them from a tokenized format
- Excel
- S
- RR (programming language)R is a programming language and software environment for statistical computing and graphics. The R language is widely used among statisticians for developing statistical software, and R is widely used for statistical software development and data analysis....
- R
- TclTclTcl is a scripting language created by John Ousterhout. Originally "born out of frustration", according to the author, with programmers devising their own languages intended to be embedded into applications, Tcl gained acceptance on its own...
- XOTclXOTclXOTcl is an object-oriented extension for the Tool Command Language created by Gustaf Neumann and Uwe Zdun. It is an extension of the MIT OTcl. XOTcl supports metaclasses. Class and method definitions are completely dynamic...
- XOTcl
- XMLmosaic An xml contained C# like programming language interpreted by a console application written in Visual Basic .NET
Languages usually compiled to a virtual machine code
Many interpreted languages are first compiled to some form of virtual machineVirtual machine
A virtual machine is a "completely isolated guest operating system installation within a normal host operating system". Modern virtual machines are implemented with either software emulation or hardware virtualization or both together.-VM Definitions:A virtual machine is a software...
code, which is then either interpreted or compiled at runtime to native code.
- JavaJava (programming language)Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities...
(frequently translated to bytecodeBytecodeBytecode, also known as p-code , is a term which has been used to denote various forms of instruction sets designed for efficient execution by a software interpreter as well as being suitable for further compilation into machine code...
, but can also be compiled to a native binary using an AOT compilerAOT compilerAn ahead-of-time compiler is a compiler that implements ahead-of-time compilation. This refers to the act of compiling an intermediate language, such as Java bytecode, .NET Common Intermediate Language , or IBM System/38 or IBM System i "Technology Independent Machine Interface" code, into a...
)- Groovy
- Join JavaJoin JavaJoin Java is a programming language that extends the standard Java programming language with the join semantics of the join-calculus. It was written at the University of South Australia within the Reconfigurable Computing Lab by Dr...
- ColdFusionColdFusionIn computing, ColdFusion is the name of a commercial rapid application development platform invented by Jeremy and JJ Allaire in 1995. ColdFusion was originally designed to make it easier to connect simple HTML pages to a database, by version 2 it had...
- Scala
- Lua
- .NET Framework.NET FrameworkThe .NET Framework is a software framework that runs primarily on Microsoft Windows. It includes a large library and supports several programming languages which allows language interoperability...
languages (translated to CILCommon Intermediate LanguageCommon Intermediate Language is the lowest-level human-readable programming language defined by the Common Language Infrastructure specification and is used by the .NET Framework and Mono...
code)- C#
- Visual Basic .NETVisual Basic .NETVisual Basic .NET , is an object-oriented computer programming language that can be viewed as an evolution of the classic Visual Basic , which is implemented on the .NET Framework...
- Pike
- PythonPython (programming language)Python is a general-purpose, high-level programming language whose design philosophy emphasizes code readability. Python claims to "[combine] remarkable power with very clear syntax", and its standard library is large and comprehensive...
- Squeak Smalltalk
- Visual FoxProVisual FoxProVisual FoxPro is a data-centric object-oriented and procedural programming language produced by Microsoft. It is derived from FoxPro which was developed by Fox Software beginning in 1984. Fox Technologies merged with Microsoft in 1992, after which the software acquired further features and the...