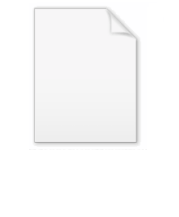
Integer (computer science)
Encyclopedia
In computer science, an integer is a datum
of integral data type, a data type
which represents some finite subset
of the mathematical integer
s. Integral data types may be of different sizes and may or may not be allowed to contain negative values.
An integer value is typically specified in the (source code
of the) program as a sequence of digits, without spaces or thousands separators, optionally prefixed with + or -. Some programming languages allow other notations, such as hexadecimal (base 16) or octal (base 8).
The internal representation of this datum is the way the value is stored in the computer’s memory.
Unlike mathematical integers, a typical datum in a computer has some minimal and maximum possible value. Typically all integers from the minimum through the maximum can be represented.
The maximum is sometimes called MAXINT or—as in the C standard library limits.h header—INT_MAX.
The most common representation of a positive integer is a string of bit
s, using the binary numeral system
. The order of the memory bytes storing the bits varies; see endianness
. The width or precision of an integral type is the number of bits in its representation. An integral type with n bits can encode 2n numbers; for example an unsigned type typically represents the non-negative values 0 through 2n−1.
There are four different ways to represent negative numbers in a binary numeral system. The most common is two’s complement
, which allows a signed integral type with n bits to represent numbers from −2(n−1) through 2(n−1)−1. Two’s complement arithmetic is convenient because there is a perfect one-to-one correspondence
between representations and values (in particular, no separate +0 and -0), and because addition
, subtraction
and multiplication
do not need to distinguish between signed and unsigned types. The other possibilities are offset binary
, sign-magnitude and ones' complement. See Signed number representations
for details.
Different CPUs
support different integral data types. Typically, hardware will support both signed and unsigned types but only a small, fixed set of widths.
The table above lists integral type widths that are supported in hardware by common processors. High level programming languages provide more possibilities. It is common to have a ‘double width’ integral type that has twice as many bits as the biggest hardware-supported type. Many languages also have bit-field types (a specified number of bits, usually constrained to be less than the maximum hardware-supported width) and range types (which can represent only the integers in a specified range).
Some languages, such as Lisp
, Smalltalk
, REXX
and Haskell
, support arbitrary precision integers (also known as infinite precision integers or bignums). Other languages which do not support this concept as a top-level construct may have libraries available to represent very large numbers using arrays of smaller variables, such as Java's
's "
A Boolean
or Flag
type is a type which can represent only two values: 0 and 1, usually identified with false and true respectively. This type can be stored in memory using a single bit, but is often given a full byte for convenience of addressing and speed of access.
A four-bit quantity is known as a nibble
(when eating, being smaller than a bite) or nybble (being a pun on the form of the word byte). One nibble corresponds to one digit in hexadecimal
and holds one digit or a sign code in binary-coded decimal.
The term octet always refers to an 8-bit quantity. It is mostly used in the field of computer network
ing, where computers with different byte widths might have to communicate.
In modern usage byte almost invariably means eight bits, since all other sizes have fallen into disuse; thus byte has come to be synonymous with octet.
. The size of a word is thus CPU-specific. Many different word sizes have been used, including 6-, 8-, 12-, 16-, 18-, 24-, 32-, 36-, 39-, 48-, 60-, and 64-bit. Since it is architectural, the size of a word is usually set by the first CPU in a family, rather than the characteristics of a later compatible CPU. The meanings of terms derived from word, such as longword, doubleword, quadword, and halfword, also vary with the CPU and OS.
Practically all new desktop processors are capable of using 64-bit words, though embedded processors
with 8- and 16-bit word size are still common. The 36-bit word length
was common in the early days of computers.
One important cause of non-portability of software is the incorrect assumption that all computers have the same word size as the computer used by the programmer. For example, if a programmer using the C language incorrectly declares as
A short integer in one programming language
may be a different size in a different language or on a different processor. In some languages this size is fixed across platforms, while in others it is machine-dependent. In some languages this datatype does not exist at all.
In C
, it is denoted by
, a
, the datatype
is greater than or equal to that of a standard integer on the same machine.
A long integer in one programming language
may be different in size from a long integer in a different language or processor. In some languages this size is fixed across platforms, while in others it is machine dependent. In some languages this data type
does not exist at all.
A long integer commonly requires double the storage capacity of a standard integer, although this is not always the case.
version of the C programming language
and the C++11 version of C++
, a
provides stdint.h; this was introduced in C99 and C++11.
† the term
Data
The term data refers to qualitative or quantitative attributes of a variable or set of variables. Data are typically the results of measurements and can be the basis of graphs, images, or observations of a set of variables. Data are often viewed as the lowest level of abstraction from which...
of integral data type, a data type
Data type
In computer programming, a data type is a classification identifying one of various types of data, such as floating-point, integer, or Boolean, that determines the possible values for that type; the operations that can be done on values of that type; the meaning of the data; and the way values of...
which represents some finite subset
Subset
In mathematics, especially in set theory, a set A is a subset of a set B if A is "contained" inside B. A and B may coincide. The relationship of one set being a subset of another is called inclusion or sometimes containment...
of the mathematical integer
Integer
The integers are formed by the natural numbers together with the negatives of the non-zero natural numbers .They are known as Positive and Negative Integers respectively...
s. Integral data types may be of different sizes and may or may not be allowed to contain negative values.
Value and representation
The value of an item with an integral type is the mathematical integer that it corresponds to. Integral types may be unsigned (capable of representing only non-negative integers) or signed (capable of representing negative integers as well).An integer value is typically specified in the (source code
Source code
In computer science, source code is text written using the format and syntax of the programming language that it is being written in. Such a language is specially designed to facilitate the work of computer programmers, who specify the actions to be performed by a computer mostly by writing source...
of the) program as a sequence of digits, without spaces or thousands separators, optionally prefixed with + or -. Some programming languages allow other notations, such as hexadecimal (base 16) or octal (base 8).
The internal representation of this datum is the way the value is stored in the computer’s memory.
Unlike mathematical integers, a typical datum in a computer has some minimal and maximum possible value. Typically all integers from the minimum through the maximum can be represented.
The maximum is sometimes called MAXINT or—as in the C standard library limits.h header—INT_MAX.
The most common representation of a positive integer is a string of bit
Bit
A bit is the basic unit of information in computing and telecommunications; it is the amount of information stored by a digital device or other physical system that exists in one of two possible distinct states...
s, using the binary numeral system
Binary numeral system
The binary numeral system, or base-2 number system, represents numeric values using two symbols, 0 and 1. More specifically, the usual base-2 system is a positional notation with a radix of 2...
. The order of the memory bytes storing the bits varies; see endianness
Endianness
In computing, the term endian or endianness refers to the ordering of individually addressable sub-components within the representation of a larger data item as stored in external memory . Each sub-component in the representation has a unique degree of significance, like the place value of digits...
. The width or precision of an integral type is the number of bits in its representation. An integral type with n bits can encode 2n numbers; for example an unsigned type typically represents the non-negative values 0 through 2n−1.
There are four different ways to represent negative numbers in a binary numeral system. The most common is two’s complement
Two's complement
The two's complement of a binary number is defined as the value obtained by subtracting the number from a large power of two...
, which allows a signed integral type with n bits to represent numbers from −2(n−1) through 2(n−1)−1. Two’s complement arithmetic is convenient because there is a perfect one-to-one correspondence
Bijection
A bijection is a function giving an exact pairing of the elements of two sets. A bijection from the set X to the set Y has an inverse function from Y to X. If X and Y are finite sets, then the existence of a bijection means they have the same number of elements...
between representations and values (in particular, no separate +0 and -0), and because addition
Addition
Addition is a mathematical operation that represents combining collections of objects together into a larger collection. It is signified by the plus sign . For example, in the picture on the right, there are 3 + 2 apples—meaning three apples and two other apples—which is the same as five apples....
, subtraction
Subtraction
In arithmetic, subtraction is one of the four basic binary operations; it is the inverse of addition, meaning that if we start with any number and add any number and then subtract the same number we added, we return to the number we started with...
and multiplication
Multiplication
Multiplication is the mathematical operation of scaling one number by another. It is one of the four basic operations in elementary arithmetic ....
do not need to distinguish between signed and unsigned types. The other possibilities are offset binary
Offset binary
Offset binary, also referred to as excess-K, is a digital coding scheme where all-zero corresponds to the minimal negative value and all-one to the maximal positive value. There is no standard for offset binary, but most often the offset K for an n-bit binary word is K=2^...
, sign-magnitude and ones' complement. See Signed number representations
Signed number representations
In computing, signed number representations are required to encode negative numbers in binary number systems.In mathematics, negative numbers in any base are represented by prefixing them with a − sign. However, in computer hardware, numbers are represented in binary only without extra...
for details.
Common integral data types
Bits | Name | Range (assuming two's complement Two's complement The two's complement of a binary number is defined as the value obtained by subtracting the number from a large power of two... for signed) |
Decimal Digits (approx.) | Uses | Implementations | ||||
---|---|---|---|---|---|---|---|---|---|
C C (programming language) C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system.... /C++ C++ C++ is a statically typed, free-form, multi-paradigm, compiled, general-purpose programming language. It is regarded as an intermediate-level language, as it comprises a combination of both high-level and low-level language features. It was developed by Bjarne Stroustrup starting in 1979 at Bell... | C# | Pascal and Delphi | Java | SQL SQL SQL is a programming language designed for managing data in relational database management systems .... |
|||||
4 | nibble Nibble In computing, a nibble is a four-bit aggregation, or half an octet... , semioctet |
Signed: From ![]() ![]() ![]() ![]() |
1 | Binary-coded decimal Binary-coded decimal In computing and electronic systems, binary-coded decimal is a digital encoding method for numbers using decimal notation, with each decimal digit represented by its own binary sequence. In BCD, a numeral is usually represented by four bits which, in general, represent the decimal range 0 through 9... , single decimal digit representation. |
|||||
Unsigned: From ![]() ![]() ![]() |
2 | ||||||||
8 | byte Byte The byte is a unit of digital information in computing and telecommunications that most commonly consists of eight bits. Historically, a byte was the number of bits used to encode a single character of text in a computer and for this reason it is the basic addressable element in many computer... , octet Octet (computing) An octet is a unit of digital information in computing and telecommunications that consists of eight bits. The term is often used when the term byte might be ambiguous, as there is no standard for the size of the byte.-Overview:... |
Signed: From ![]() ![]() 127 (number) 127 is the natural number following 126 and preceding 128.- In mathematics :*As a Mersenne prime, 127 is related to the perfect number 8128. 127 is also an exponent for the Mersenne prime 2127 - 1, making 127 a double Mersenne prime... , from ![]() ![]() |
3 | ASCII ASCII The American Standard Code for Information Interchange is a character-encoding scheme based on the ordering of the English alphabet. ASCII codes represent text in computers, communications equipment, and other devices that use text... characters |
int8_t, char | sbyte | Shortint | byte | tinyint |
Unsigned: From ![]() ![]() ![]() |
3 | uint8_t, char | byte | Byte | n/a | unsigned tinyint | |||
16 | halfword, word, short | Signed: From ![]() ![]() 30000 (number) 30,000 is the number that comes after 29,999 and before 30,001.-Selected numbers:* 30000 – round number* 30029 – primorial prime* 30030 – primorial* 30240 – harmonic divisor number... , from ![]() ![]() |
5 | UCS-2 characters | int16_t, short, int | short | Smallint | short | smallint |
Unsigned: From ![]() ![]() ![]() |
5 | uint16_t | ushort | Word | char | unsigned smallint | |||
32 | word, long, doubleword, longword, int | Signed: From ![]() ![]() ![]() ![]() |
10 | UCS-4 characters, Truecolor with alpha, FourCC FourCC A FourCC is a sequence of four bytes used to uniquely identify data formats.The concept originated in the OSType scheme used in the Macintosh system software and was adopted for the Amiga/Electronic Arts Interchange File Format and derivatives... , ActionScript int |
int32_t, int, long | int | LongInt; Integer | int | int |
Unsigned: From ![]() ![]() ![]() |
10 | uint32_t | uint | LongWord; Cardinal | n/a | unsigned int | |||
64 | word, doubleword, longword, long long, quad, quadword, int64 | Signed: From ![]() ![]() ![]() ![]() |
19 | Very large numbers | int64_t, long, long long | long | Int64 | long | bigint |
Unsigned: From ![]() ![]() ![]() |
20 | uint64_t | ulong | 'QWord | n/a | unsigned bigint | |||
128 | octaword, double quadword | Signed: From ![]() ![]() ![]() ![]() |
39 | C: only available as non-standard compiler-specific extension | |||||
Unsigned: From ![]() ![]() ![]() |
39 | ||||||||
n | n-bit integer (general case) |
Signed: ![]() ![]() |
![]() |
Ada Ada (programming language) Ada is a structured, statically typed, imperative, wide-spectrum, and object-oriented high-level computer programming language, extended from Pascal and other languages... range -2**(n-1)..2**(n-1)-1 |
|||||
Unsigned: 0 to ![]() |
![]() |
Ada range 0..2**n-1 , Ada mod 2**n |
Different CPUs
Central processing unit
The central processing unit is the portion of a computer system that carries out the instructions of a computer program, to perform the basic arithmetical, logical, and input/output operations of the system. The CPU plays a role somewhat analogous to the brain in the computer. The term has been in...
support different integral data types. Typically, hardware will support both signed and unsigned types but only a small, fixed set of widths.
The table above lists integral type widths that are supported in hardware by common processors. High level programming languages provide more possibilities. It is common to have a ‘double width’ integral type that has twice as many bits as the biggest hardware-supported type. Many languages also have bit-field types (a specified number of bits, usually constrained to be less than the maximum hardware-supported width) and range types (which can represent only the integers in a specified range).
Some languages, such as Lisp
Lisp programming language
Lisp is a family of computer programming languages with a long history and a distinctive, fully parenthesized syntax. Originally specified in 1958, Lisp is the second-oldest high-level programming language in widespread use today; only Fortran is older...
, Smalltalk
Smalltalk
Smalltalk is an object-oriented, dynamically typed, reflective programming language. Smalltalk was created as the language to underpin the "new world" of computing exemplified by "human–computer symbiosis." It was designed and created in part for educational use, more so for constructionist...
, REXX
REXX
REXX is an interpreted programming language that was developed at IBM. It is a structured high-level programming language that was designed to be both easy to learn and easy to read...
and Haskell
Haskell (programming language)
Haskell is a standardized, general-purpose purely functional programming language, with non-strict semantics and strong static typing. It is named after logician Haskell Curry. In Haskell, "a function is a first-class citizen" of the programming language. As a functional programming language, the...
, support arbitrary precision integers (also known as infinite precision integers or bignums). Other languages which do not support this concept as a top-level construct may have libraries available to represent very large numbers using arrays of smaller variables, such as Java's
BigInteger
class or PerlPerl
Perl is a high-level, general-purpose, interpreted, dynamic programming language. Perl was originally developed by Larry Wall in 1987 as a general-purpose Unix scripting language to make report processing easier. Since then, it has undergone many changes and revisions and become widely popular...
's "
bigint
" package. These use as much of the computer’s memory as is necessary to store the numbers; however, a computer has only a finite amount of storage, so they too can only represent a finite subset of the mathematical integers. These schemes support very large numbers, for example one kilobyte of memory could be used to store numbers up to 2466 decimal digits long.A Boolean
Boolean datatype
In computer science, the Boolean or logical data type is a data type, having two values , intended to represent the truth values of logic and Boolean algebra...
or Flag
Flag (computing)
In computer programming, flag can refer to one or more bits that are used to store a binary value or code that has an assigned meaning, but can refer to uses of other data types...
type is a type which can represent only two values: 0 and 1, usually identified with false and true respectively. This type can be stored in memory using a single bit, but is often given a full byte for convenience of addressing and speed of access.
A four-bit quantity is known as a nibble
Nibble
In computing, a nibble is a four-bit aggregation, or half an octet...
(when eating, being smaller than a bite) or nybble (being a pun on the form of the word byte). One nibble corresponds to one digit in hexadecimal
Hexadecimal
In mathematics and computer science, hexadecimal is a positional numeral system with a radix, or base, of 16. It uses sixteen distinct symbols, most often the symbols 0–9 to represent values zero to nine, and A, B, C, D, E, F to represent values ten to fifteen...
and holds one digit or a sign code in binary-coded decimal.
Bytes and octets
The term byte initially meant ‘the smallest addressable unit of memory’. In the past, 5-, 6-, 7-, 8-, and 9-bit bytes have all been used. There have also been computers that could address individual bits (‘bit-addressed machine’), or that could only address 16- or 32-bit quantities (‘word-addressed machine’). The term byte was usually not used at all in connection with bit- and word-addressed machines.The term octet always refers to an 8-bit quantity. It is mostly used in the field of computer network
Computer network
A computer network, often simply referred to as a network, is a collection of hardware components and computers interconnected by communication channels that allow sharing of resources and information....
ing, where computers with different byte widths might have to communicate.
In modern usage byte almost invariably means eight bits, since all other sizes have fallen into disuse; thus byte has come to be synonymous with octet.
Words
The term 'word' is used for a small group of bits which are handled simultaneously by processors of a particular architectureComputer architecture
In computer science and engineering, computer architecture is the practical art of selecting and interconnecting hardware components to create computers that meet functional, performance and cost goals and the formal modelling of those systems....
. The size of a word is thus CPU-specific. Many different word sizes have been used, including 6-, 8-, 12-, 16-, 18-, 24-, 32-, 36-, 39-, 48-, 60-, and 64-bit. Since it is architectural, the size of a word is usually set by the first CPU in a family, rather than the characteristics of a later compatible CPU. The meanings of terms derived from word, such as longword, doubleword, quadword, and halfword, also vary with the CPU and OS.
Practically all new desktop processors are capable of using 64-bit words, though embedded processors
Embedded system
An embedded system is a computer system designed for specific control functions within a larger system. often with real-time computing constraints. It is embedded as part of a complete device often including hardware and mechanical parts. By contrast, a general-purpose computer, such as a personal...
with 8- and 16-bit word size are still common. The 36-bit word length
36-bit word length
Many early computers aimed at the scientific market had a 36-bit word length. This word length was just long enough to represent positive and negative integers to an accuracy of ten decimal digits . It also allowed the storage of six alphanumeric characters encoded in a six-bit character encoding...
was common in the early days of computers.
One important cause of non-portability of software is the incorrect assumption that all computers have the same word size as the computer used by the programmer. For example, if a programmer using the C language incorrectly declares as
int
a variable that will be used to store values greater than 216-1, the program will fail on computers with 16-bit integers. That variable should have been declared as long
, which has at least 32 bits on any computer. Programmers may also incorrectly assume that a pointer can be converted to an integer without loss of information, which may work on (some) 32-bit computers, but fail on 64-bit computers with 64-bit pointers and 32-bit integers.Short integer
A short integer can represent a whole number which may take less storage, while having a smaller range, compared with a standard integer on the same machine.A short integer in one programming language
Programming language
A programming language is an artificial language designed to communicate instructions to a machine, particularly a computer. Programming languages can be used to create programs that control the behavior of a machine and/or to express algorithms precisely....
may be a different size in a different language or on a different processor. In some languages this size is fixed across platforms, while in others it is machine-dependent. In some languages this datatype does not exist at all.
In C
C (programming language)
C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system....
, it is denoted by
short
. It is required to be at least 16 bits, and is often smaller than a standard integer, but this is not required. A conforming program can assume that it can safely store values between −(215−1) and 215−1, but it may not assume that the range isn't larger. In JavaJava (programming language)
Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities...
, a
short
is always a 16-bit integer. In the Windows APIWindows API
The Windows API, informally WinAPI, is Microsoft's core set of application programming interfaces available in the Microsoft Windows operating systems. It was formerly called the Win32 API; however, the name "Windows API" more accurately reflects its roots in 16-bit Windows and its support on...
, the datatype
SHORT
is defined as a 16-bit signed integer on all machines.Common short integer sizes
Programming language Programming language A programming language is an artificial language designed to communicate instructions to a machine, particularly a computer. Programming languages can be used to create programs that control the behavior of a machine and/or to express algorithms precisely.... |
Platform Platform (computing) A computing platform includes some sort of hardware architecture and a software framework , where the combination allows software, particularly application software, to run... s |
Data type name | Signedness Signedness In computing, signedness is a property of data types representing numbers in computer programs. A numeric variable is signed if it can represent both positive and negative numbers, and unsigned if it can only represent non-negative numbers .As signed numbers can represent negative numbers, they... |
Storage in bytes | Minimum value | Maximum value |
---|---|---|---|---|---|---|
C C (programming language) C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system.... and C++ C++ C++ is a statically typed, free-form, multi-paradigm, compiled, general-purpose programming language. It is regarded as an intermediate-level language, as it comprises a combination of both high-level and low-level language features. It was developed by Bjarne Stroustrup starting in 1979 at Bell... |
common implementations | short |
signed | 2 | −32,768 | 32,767 |
unsigned short |
unsigned | 2 | 0 | 65,535 | ||
C# | .NET CLR/CTS .NET Framework The .NET Framework is a software framework that runs primarily on Microsoft Windows. It includes a large library and supports several programming languages which allows language interoperability... |
short |
signed | 2 | −32,768 | 32,767 |
ushort | unsigned | 2 | 0 | 65,535 | ||
Java Java (programming language) Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities... |
Java platform | short |
signed | 2 | −32,768 | 32,767 |
Long integer
A long integer can represent a whole integer number whose rangeRange (computer science)
In computer science, the term range may refer to one of three things:# The possible values that may be stored in a variable.# The upper and lower bounds of an array.# An alternative to iterator.-Range of a variable:...
is greater than or equal to that of a standard integer on the same machine.
A long integer in one programming language
Programming language
A programming language is an artificial language designed to communicate instructions to a machine, particularly a computer. Programming languages can be used to create programs that control the behavior of a machine and/or to express algorithms precisely....
may be different in size from a long integer in a different language or processor. In some languages this size is fixed across platforms, while in others it is machine dependent. In some languages this data type
Data type
In computer programming, a data type is a classification identifying one of various types of data, such as floating-point, integer, or Boolean, that determines the possible values for that type; the operations that can be done on values of that type; the meaning of the data; and the way values of...
does not exist at all.
A long integer commonly requires double the storage capacity of a standard integer, although this is not always the case.
C and C++
In the C99C99
C99 is a modern dialect of the C programming language. It extends the previous version with new linguistic and library features, and helps implementations make better use of available computer hardware and compiler technology.-History:...
version of the C programming language
C (programming language)
C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system....
and the C++11 version of C++
C++
C++ is a statically typed, free-form, multi-paradigm, compiled, general-purpose programming language. It is regarded as an intermediate-level language, as it comprises a combination of both high-level and low-level language features. It was developed by Bjarne Stroustrup starting in 1979 at Bell...
, a
long long
type is supported that doubles the minimum capacity of the standard long
to 64 bits. This type is not supported by compilers that require C code to be compliant with the previous C++ standard, C++03, because the long long
type did not exist in C++03. For an ANSI/ISO compliant compiler the minimum requirements for the specified ranges, that is −(231) to 231−1 for signed and 0 to 232−1 for unsigned, must be fulfilled; however, extending this range is permitted. This can be an issue when exchanging code and data between platforms, or doing direct hardware access. Thus, there are several sets of headers providing platform independent exact width types. The C standard libraryStandard library
A standard library for a programming language is the library that is conventionally made available in every implementation of that language. In some cases, the library is described directly in the programming language specification; in other cases, the contents of the standard library are...
provides stdint.h; this was introduced in C99 and C++11.
Common long integer sizes
Programming language Programming language A programming language is an artificial language designed to communicate instructions to a machine, particularly a computer. Programming languages can be used to create programs that control the behavior of a machine and/or to express algorithms precisely.... |
Approval Type | Platform Platform (computing) A computing platform includes some sort of hardware architecture and a software framework , where the combination allows software, particularly application software, to run... s |
Data type name | Storage in bytes | Signed Signedness In computing, signedness is a property of data types representing numbers in computer programs. A numeric variable is signed if it can represent both positive and negative numbers, and unsigned if it can only represent non-negative numbers .As signed numbers can represent negative numbers, they... range |
Unsigned Signedness In computing, signedness is a property of data types representing numbers in computer programs. A numeric variable is signed if it can represent both positive and negative numbers, and unsigned if it can only represent non-negative numbers .As signed numbers can represent negative numbers, they... range |
---|---|---|---|---|---|---|
C ISO/ANSI C99 | International Standard | Unix Unix Unix is a multitasking, multi-user computer operating system originally developed in 1969 by a group of AT&T employees at Bell Labs, including Ken Thompson, Dennis Ritchie, Brian Kernighan, Douglas McIlroy, and Joe Ossanna... ,16/32-bit systems Windows,16/32/64-bit systems |
long † |
4 (minimum requirement 4) |
−2,147,483,648 to 2,147,483,647 | 0 to 4,294,967,295 (minimum requirement) |
C ISO/ANSI C99 | International Standard | Unix Unix Unix is a multitasking, multi-user computer operating system originally developed in 1969 by a group of AT&T employees at Bell Labs, including Ken Thompson, Dennis Ritchie, Brian Kernighan, Douglas McIlroy, and Joe Ossanna... , 64-bit systems |
long † |
8 (minimum requirement 4) |
−9,223,372,036,854,775,808 to +9,223,372,036,854,775,807 | 0 to 18,446,744,073,709,551,615 |
C++ C++ C++ is a statically typed, free-form, multi-paradigm, compiled, general-purpose programming language. It is regarded as an intermediate-level language, as it comprises a combination of both high-level and low-level language features. It was developed by Bjarne Stroustrup starting in 1979 at Bell... ISO/ANSI |
International Standard | Unix Unix Unix is a multitasking, multi-user computer operating system originally developed in 1969 by a group of AT&T employees at Bell Labs, including Ken Thompson, Dennis Ritchie, Brian Kernighan, Douglas McIlroy, and Joe Ossanna... , Windows, 16/32-bit system |
long † |
4 (minimum requirement 4) |
−2,147,483,648 to 2,147,483,647 |
0 to 4,294,967,295 (minimum requirement) |
C++/CLI C++/CLI C++/CLI is Microsoft's language specification intended to supersede Managed Extensions for C++. It is a complete revision that aims to simplify the older Managed C++ syntax . C++/CLI is standardized by Ecma as ECMA-372... |
International Standard ECMA-372 |
Unix Unix Unix is a multitasking, multi-user computer operating system originally developed in 1969 by a group of AT&T employees at Bell Labs, including Ken Thompson, Dennis Ritchie, Brian Kernighan, Douglas McIlroy, and Joe Ossanna... , Windows, 16/32-bit systems |
long † |
4 (minimum requirement 4) |
−2,147,483,648 to 2,147,483,647 |
0 to 4,294,967,295 (minimum requirement) |
VB Visual Basic Visual Basic is the third-generation event-driven programming language and integrated development environment from Microsoft for its COM programming model... |
Company Standard | Windows Microsoft Windows Microsoft Windows is a series of operating systems produced by Microsoft.Microsoft introduced an operating environment named Windows on November 20, 1985 as an add-on to MS-DOS in response to the growing interest in graphical user interfaces . Microsoft Windows came to dominate the world's personal... |
Long |
4 | −2,147,483,648 to 2,147,483,647 | N/A |
VBA Visual Basic for Applications Visual Basic for Applications is an implementation of Microsoft's event-driven programming language Visual Basic 6 and its associated integrated development environment , which are built into most Microsoft Office applications... |
Company Standard | Windows Microsoft Windows Microsoft Windows is a series of operating systems produced by Microsoft.Microsoft introduced an operating environment named Windows on November 20, 1985 as an add-on to MS-DOS in response to the growing interest in graphical user interfaces . Microsoft Windows came to dominate the world's personal... , Mac OS Mac OS Mac OS is a series of graphical user interface-based operating systems developed by Apple Inc. for their Macintosh line of computer systems. The Macintosh user experience is credited with popularizing the graphical user interface... |
Long |
4 | −2,147,483,648 to 2,147,483,647 | N/A |
SQL Server Microsoft SQL Server Microsoft SQL Server is a relational database server, developed by Microsoft: It is a software product whose primary function is to store and retrieve data as requested by other software applications, be it those on the same computer or those running on another computer across a network... |
Company Standard | Windows Microsoft Windows Microsoft Windows is a series of operating systems produced by Microsoft.Microsoft introduced an operating environment named Windows on November 20, 1985 as an add-on to MS-DOS in response to the growing interest in graphical user interfaces . Microsoft Windows came to dominate the world's personal... |
BigInt |
8 | −9,223,372,036,854,775,808 to +9,223,372,036,854,775,807 | 0 to 18,446,744,073,709,551,615 |
C#/ VB.NET Visual Basic .NET Visual Basic .NET , is an object-oriented computer programming language that can be viewed as an evolution of the classic Visual Basic , which is implemented on the .NET Framework... |
ECMA International Standard | Microsoft .NET | long or Int64 |
8 | −9,223,372,036,854,775,808 to +9,223,372,036,854,775,807 | 0 to 18,446,744,073,709,551,615 |
Java Java (programming language) Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities... |
International/Company Standard | Java platform | long |
8 | −9,223,372,036,854,775,808 to +9,223,372,036,854,775,807 | N/A |
Pascal Pascal (programming language) Pascal is an influential imperative and procedural programming language, designed in 1968/9 and published in 1970 by Niklaus Wirth as a small and efficient language intended to encourage good programming practices using structured programming and data structuring.A derivative known as Object Pascal... |
? | Windows Microsoft Windows Microsoft Windows is a series of operating systems produced by Microsoft.Microsoft introduced an operating environment named Windows on November 20, 1985 as an add-on to MS-DOS in response to the growing interest in graphical user interfaces . Microsoft Windows came to dominate the world's personal... , UNIX Unix Unix is a multitasking, multi-user computer operating system originally developed in 1969 by a group of AT&T employees at Bell Labs, including Ken Thompson, Dennis Ritchie, Brian Kernighan, Douglas McIlroy, and Joe Ossanna... |
int64 |
8 | −9,223,372,036,854,775,808 to +9,223,372,036,854,775,807 | 0 to 18,446,744,073,709,551,615(Qword type) |
† the term
long int
is equivalent but it is used rarelySee also
- Signed number representationsSigned number representationsIn computing, signed number representations are required to encode negative numbers in binary number systems.In mathematics, negative numbers in any base are represented by prefixing them with a − sign. However, in computer hardware, numbers are represented in binary only without extra...
- Binary-coded decimalBinary-coded decimalIn computing and electronic systems, binary-coded decimal is a digital encoding method for numbers using decimal notation, with each decimal digit represented by its own binary sequence. In BCD, a numeral is usually represented by four bits which, in general, represent the decimal range 0 through 9...
, another, rather different, representation for integers which is still commonly used in mainframeMainframe computerMainframes are powerful computers used primarily by corporate and governmental organizations for critical applications, bulk data processing such as census, industry and consumer statistics, enterprise resource planning, and financial transaction processing.The term originally referred to the...
financial applications and in databases.