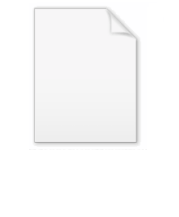
Factory method pattern
Encyclopedia
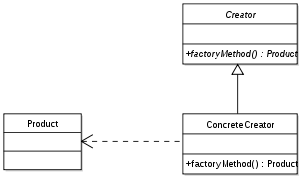
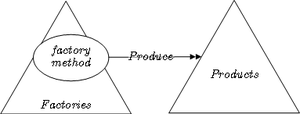
Design pattern (computer science)
In software engineering, a design pattern is a general reusable solution to a commonly occurring problem within a given context in software design. A design pattern is not a finished design that can be transformed directly into code. It is a description or template for how to solve a problem that...
to implement the concept of factories. Like other creational pattern
Creational pattern
In software engineering, creational design patterns are design patterns that deal with object creation mechanisms, trying to create objects in a manner suitable to the situation. The basic form of object creation could result in design problems or added complexity to the design. Creational design...
s, it deals with the problem of creating objects
Object (computer science)
In computer science, an object is any entity that can be manipulated by the commands of a programming language, such as a value, variable, function, or data structure...
(products) without specifying the exact class
Class (computer science)
In object-oriented programming, a class is a construct that is used as a blueprint to create instances of itself – referred to as class instances, class objects, instance objects or simply objects. A class defines constituent members which enable these class instances to have state and behavior...
of object that will be created.
The creation of an object often requires complex processes not appropriate to include within a composing object. The object's creation may lead to a significant duplication of code, may require information not accessible to the composing object, may not provide a sufficient level of abstraction, or may otherwise not be part of the composing object's concerns
Concern (computer science)
In computer science, a concern is a particular set of behaviors needed by a computer program, the conceptual sections. A concern can be as general as database interaction or as specific as performing a calculation, depending on the level of conversation between developers and the program being...
. The factory method design pattern handles these problems by defining a separate method
Method (computer science)
In object-oriented programming, a method is a subroutine associated with a class. Methods define the behavior to be exhibited by instances of the associated class at program run time...
for creating the objects, which subclasses can then override to specify the derived type
Subtype
In programming language theory, subtyping or subtype polymorphism is a form of type polymorphism in which a subtype is a datatype that is related to another datatype by some notion of substitutability, meaning that program constructs, typically subroutines or functions, written to operate on...
of product that will be created.
Some of the processes required in the creation of an object include determining which object to create, managing the lifetime of the object, and managing specialized build-up and tear-down concerns of the object. Outside the scope of design patterns, the term factory method can also refer to a method of a factory whose main purpose is creation of objects.
Definition
The essence of the Factory method Pattern is to "Define an interface for creating an object, but let the subclasses decide which class to instantiate. The Factory method lets a class defer instantiation to subclasses."Concept
In object-oriented computer programmingComputer programming
Computer programming is the process of designing, writing, testing, debugging, and maintaining the source code of computer programs. This source code is written in one or more programming languages. The purpose of programming is to create a program that performs specific operations or exhibits a...
, a factory is an object
Object (computer science)
In computer science, an object is any entity that can be manipulated by the commands of a programming language, such as a value, variable, function, or data structure...
for creating other objects. It is an abstraction
Abstraction (computer science)
In computer science, abstraction is the process by which data and programs are defined with a representation similar to its pictorial meaning as rooted in the more complex realm of human life and language with their higher need of summarization and categorization , while hiding away the...
of a constructor
Constructor (computer science)
In object-oriented programming, a constructor in a class is a special type of subroutine called at the creation of an object. It prepares the new object for use, often accepting parameters which the constructor uses to set any member variables required when the object is first created...
, and can be used to implement various allocation schemes. For example, using this definition, singletons implemented by the singleton pattern
Singleton pattern
In software engineering, the singleton pattern is a design pattern used to implement the mathematical concept of a singleton, by restricting the instantiation of a class to one object. This is useful when exactly one object is needed to coordinate actions across the system...
are formal factories.
A factory object typically has a method
Method (computer science)
In object-oriented programming, a method is a subroutine associated with a class. Methods define the behavior to be exhibited by instances of the associated class at program run time...
for every kind of object it is capable of creating. These methods optionally accept parameter
Parameter
Parameter from Ancient Greek παρά also “para” meaning “beside, subsidiary” and μέτρον also “metron” meaning “measure”, can be interpreted in mathematics, logic, linguistics, environmental science and other disciplines....
s defining how the object is created, and then return the created object.
Factory objects are used in situations where getting hold of an object of a particular kind is a more complex process than simply creating a new object. The factory object might decide to create the object's class
Class (computer science)
In object-oriented programming, a class is a construct that is used as a blueprint to create instances of itself – referred to as class instances, class objects, instance objects or simply objects. A class defines constituent members which enable these class instances to have state and behavior...
(if applicable) dynamically, return it from an object pool
Object pool
In computer programming, an object pool is a software design pattern. An object pool is a set of initialised objects that are kept ready to use, rather than allocated and destroyed on demand. A client of the pool will request an object from the pool and perform operations on the returned object...
, do complex configuration on the object, or other things.
These kinds of objects have proven useful and several design pattern
Design pattern (computer science)
In software engineering, a design pattern is a general reusable solution to a commonly occurring problem within a given context in software design. A design pattern is not a finished design that can be transformed directly into code. It is a description or template for how to solve a problem that...
s have been developed to implement them in many languages. For example, several "GoF patterns", like the "Factory method pattern", the "Builder
Builder pattern
The builder pattern is an object creation software design pattern. The intention is to abstract steps of construction of objects so that different implementations of these steps can construct different representations of objects...
" or even the "Singleton" are implementations of this concept. The "Abstract factory pattern
Abstract factory pattern
The abstract factory pattern is a software design pattern that provides a way to encapsulate a group of individual factories that have a common theme. In normal usage, the client software creates a concrete implementation of the abstract factory and then uses the generic interfaces to create the...
" instead is a method to build collections of factories.
Common usage
Factory methods are common in toolkitToolkit
A toolkit is an assembly of tools; set of basic building units for graphical user interfaces.Things called toolkits include:* Abstract Window Toolkit* Accessibility Toolkit* Adventure Game Toolkit* B-Toolkit* Battlefield Mod Development Toolkit...
s and frameworks
Software framework
In computer programming, a software framework is an abstraction in which software providing generic functionality can be selectively changed by user code, thus providing application specific software...
where library code needs to create objects of types which may be subclassed by applications using the framework.
Parallel class hierarchies often require objects from one hierarchy to be able to create appropriate objects from another.
Factory methods are used in test-driven development
Test-driven development
Test-driven development is a software development process that relies on the repetition of a very short development cycle: first the developer writes a failing automated test case that defines a desired improvement or new function, then produces code to pass that test and finally refactors the new...
to allow classes to be put under test. If such a class
Foo
creates another object Dangerous
that can't be put under automated unit testUnit test
In computer programming, unit testing is a method by which individual units of source code are tested to determine if they are fit for use.A unit is the smallest testable part of an application. In procedural programming a unit could be an entire module but is more commonly an individual function...
s (perhaps it communicates with a production database that isn't always available), then the creation of
Dangerous
objects is placed in the virtualVirtual function
In object-oriented programming, a virtual function or virtual method is a function or method whose behaviour can be overridden within an inheriting class by a function with the same signature...
factory method
createDangerous
in class Foo
. For testing, TestFoo
(a subclass of Foo
) is then created, with the virtual factory method createDangerous
overridden to create and return FakeDangerous
, a fake object. Unit tests then use TestFoo
to test the functionality of Foo
without incurring the side effect of using a real Dangerous
object.Applicability
Use the factory pattern when:- The creation of the object precludes reuse without significantly duplicating code.
- The creation of the object requires access to information or resources not appropriate to contain within the composing object.
- The lifetime management of created objects needs to be centralised to ensure consistent behavior.
Other benefits and variants
Although the motivation behind the factory method pattern is to allow subclasses to choose which type of object to create, there are other benefits to using factory methods, many of which do not depend on subclassing. Therefore, it is common to define "factory methods" that are not polymorphic to create objects in order to gain these other benefits. Such methods are often static.Descriptive names
A factory method has a distinct name. In many object-oriented languages, constructorsConstructor (computer science)
In object-oriented programming, a constructor in a class is a special type of subroutine called at the creation of an object. It prepares the new object for use, often accepting parameters which the constructor uses to set any member variables required when the object is first created...
must have the same name as the class they are in, which can lead to ambiguity if there is more than one way to create an object (see overloading). Factory methods have no such constraint and can have descriptive names. As an example, when complex number
Complex number
A complex number is a number consisting of a real part and an imaginary part. Complex numbers extend the idea of the one-dimensional number line to the two-dimensional complex plane by using the number line for the real part and adding a vertical axis to plot the imaginary part...
s are created from two real numbers the real numbers can be interpreted as Cartesian or polar coordinates, but using factory methods, the meaning is clear (the following examples are in Java
Java (programming language)
Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities...
and VB.NET):
class Complex {
public static Complex fromCartesian(double real, double imaginary) {
return new Complex(real, imaginary);
}
public static Complex fromPolar(double modulus, double angle) {
return new Complex(modulus * cos(angle), modulus * sin(angle));
}
private Complex(double a, double b) {
//...
}
}
Complex c = Complex.fromPolar(1, pi);
Public Class Complex
Public Shared Function fromCartesian(real As Double, imaginary As Double) As Complex
Return (New Complex(real, imaginary))
End Function
Public Shared Function fromPolar(modulus As Double, angle As Double) As Complex
Return (New Complex(modulus * Math.Cos(angle), modulus * Math.Sin(angle)))
End Function
Private Sub New(a As Double, b As Double)
'...
End Sub
End Class
When factory methods are used for disambiguation like this, the constructor is often made private to force clients to use the factory methods.
Encapsulation
Factory methods encapsulate the creation of objects.This can be useful if the creation process is very complex, for example if it depends on settings in configuration files or on user input.
Consider as an example a program to read image files and make thumbnail
Thumbnail
Thumbnails are reduced-size versions of pictures, used to help in recognizing and organizing them, serving the same role for images as a normal text index does for words...
s from them.
The program supports different image formats, represented by a reader class for each format:
public interface ImageReader
{
public DecodedImage getDecodedImage;
}
public class GifReader implements ImageReader
{
public DecodedImage getDecodedImage
{
// ...
return decodedImage;
}
}
public class JpegReader implements ImageReader
{
public DecodedImage getDecodedImage
{
// ...
return decodedImage;
}
}
Each time the program reads an image it needs to create a reader of the appropriate type based on some information in the file. This logic can be encapsulated in a factory method:
public class ImageReaderFactory
{
public static ImageReader getImageReader(InputStream is)
{
int imageType = determineImageType(is);
switch(imageType)
{
case ImageReaderFactory.GIF:
return new GifReader(is);
case ImageReaderFactory.JPEG:
return new JpegReader(is);
// etc.
}
}
}
The code fragment in the previous example uses a switch statement
Switch statement
In computer programming, a switch, case, select or inspect statement is a type of selection control mechanism that exists in most imperative programming languages such as Pascal, Ada, C/C++, C#, Java, and so on. It is also included in several other types of languages...
to associate an
imageType
with a specific factory objectFactory object
In object-oriented computer programming, a factory is an object for creating other objects. It is an abstraction of a constructor, and can be used to implement various allocation schemes. For example, using this definition, singletons implemented by the singleton pattern are formal factories.A...
. Alternatively, this association could also be implemented as a mapping. This would allow the switch statement to be replaced with an associative array
Associative array
In computer science, an associative array is an abstract data type composed of a collection of pairs, such that each possible key appears at most once in the collection....
lookup.
Java
A maze game may be played in two modes, one with regular rooms that are only connected with adjacent rooms, and one with magic rooms that allow players to be transported at random (this JavaJava (programming language)
Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities...
example is similar to one in the book Design Patterns
Design Patterns
Design Patterns: Elements of Reusable Object-Oriented Software is a software engineering book describing recurring solutions to common problems in software design. The book's authors are Erich Gamma, Richard Helm, Ralph Johnson and John Vlissides with a foreword by Grady Booch. The authors are...
). The regular game mode could use this template method:
public class MazeGame {
public MazeGame {
Room room1 = makeRoom;
Room room2 = makeRoom;
room1.connect(room2);
this.addRoom(room1);
this.addRoom(room2);
}
protected Room makeRoom {
return new OrdinaryRoom;
}
}
In the above snippet,
makeRoom
is a template method. It encapsulates the creation of rooms such that other rooms can be used in a subclass. To implement the other game mode that has magic rooms, it suffices to override the makeRoom
method:public class MagicMazeGame extends MazeGame {
@Override
protected Room makeRoom {
return new MagicRoom;
}
}
PHP
class Factory
{
public static function build($type)
{
$class = 'Format' . $type;
if (!class_exists($class)) {
throw new Exception('Missing format class.');
}
return new $class;
}
}
class FormatString {}
class FormatNumber {}
try {
$string = Factory::build('String');
}
catch (Exception $e) {
echo $e->getMessage;
}
try {
$number = Factory::build('Number');
}
catch (Exception $e) {
echo $e->getMessage;
}
Limitations
There are three limitations associated with the use of the factory method. The first relates to refactoring existing code; the other two relate to extending a class.- The first limitation is that refactoring an existing class to use factories breaks existing clients. For example, if class Complex was a standard class, it might have numerous clients with code like:
- Once we realize that two different factories are needed, we change the class (to the code shown earlier). But since the constructor is now private, the existing client code no longer compiles.
- The second limitation is that, since the pattern relies on using a private constructor, the class cannot be extended. Any subclass must invoke the inherited constructor, but this cannot be done if that constructor is private.
- The third limitation is that, if we do extend the class (e.g., by making the constructor protected—this is risky but feasible), the subclass must provide its own re-implementation of all factory methods with exactly the same signatures. For example, if class StrangeComplex extends Complex, then unless StrangeComplex provides its own version of all factory methods, the call StrangeComplex.fromPolar(1, pi) will yield an instance of Complex (the superclass) rather than the expected instance of the subclass.
All three problems could be alleviated by altering the underlying programming language to make factories first-class class members (see also Virtual class
Virtual class
A virtual class is an inner class that can be overridden by subclasses of the outer class.Virtual Classes are inner classes of another outer class, which behave like virtual functions. This means they can be overridden in a subclass of the outer class, and the run time type of a virtual class...
).
Uses
- In ADO.NETADO.NETADO.NET is a set of computer software components that programmers can use to access data and data services. It is a part of the base class library that is included with the Microsoft .NET Framework. It is commonly used by programmers to access and modify data stored in relational database systems,...
, IDbCommand.CreateParameter is an example of the use of factory method to connect parallel class hierarchies. - In QtQt (toolkit)Qt is a cross-platform application framework that is widely used for developing application software with a graphical user interface , and also used for developing non-GUI programs such as command-line tools and consoles for servers...
, QMainWindow::createPopupMenu is a factory method declared in a framework which can be overridden in application code. - In JavaJava (programming language)Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities...
, several factories are used in the javax.xml.parsers package. e.g. javax.xml.parsers.DocumentBuilderFactory or javax.xml.parsers.SAXParserFactory.
See also
- Design PatternsDesign PatternsDesign Patterns: Elements of Reusable Object-Oriented Software is a software engineering book describing recurring solutions to common problems in software design. The book's authors are Erich Gamma, Richard Helm, Ralph Johnson and John Vlissides with a foreword by Grady Booch. The authors are...
, the highly influential book - Design patternDesign patternA design pattern in architecture and computer science is a formal way of documenting a solution to a design problem in a particular field of expertise. The idea was introduced by the architect Christopher Alexander in the field of architecture and has been adapted for various other disciplines,...
, overview of design patterns in general - Abstract factory patternAbstract factory patternThe abstract factory pattern is a software design pattern that provides a way to encapsulate a group of individual factories that have a common theme. In normal usage, the client software creates a concrete implementation of the abstract factory and then uses the generic interfaces to create the...
, a pattern often implemented using factory methods - Builder patternBuilder patternThe builder pattern is an object creation software design pattern. The intention is to abstract steps of construction of objects so that different implementations of these steps can construct different representations of objects...
, another creational pattern - Template method patternTemplate method patternIn software engineering, the template method pattern is a design pattern.It is a behavioral pattern, and is unrelated to C++ templates.-Introduction:A template method defines the program skeleton of an algorithm...
, which may call factory methods
External links
- Factory method in UML and in LePUS3 (a Design Description Language)
- Consider static factory methods by Joshua Bloch