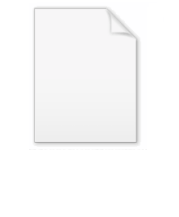
Event-driven programming
Encyclopedia
In computer programming
, event-driven programming or event-based programming is a programming paradigm
in which the flow of the program is determined by event
s—i.e., sensor
outputs or user actions (mouse clicks, key presses) or messages
from other programs or threads
.
Event-driven programming can also be defined as an application architecture technique in which the application has a main loop which is clearly divided down to two sections: the first is event selection (or event detection), and the second is event handling. In embedded systems the same may be achieved using interrupt
s instead of a constantly running main loop; in that case the former portion of the architecture resides completely in hardware.
Event-driven programs can be written in any language, although the task is easier in languages that provide high-level abstraction
s, such as closures
. Some integrated development environment
s provide code generation assistants that automate the most repetitive tasks required for event handling.
While keeping track of history is straightforward in a batch program, it requires special attention and planning in an event-driven program.
error, overflow
or "program checks" may occur that possibly prevents further processing. Exception handlers
may be provided by "ON statements" in (unseen) callers to provide housekeeping
routines to clean up afterwards before termination.
, called event-handler routines. These routines handle the events to which the main program will respond. For example, a single left-button mouse-click on a command button in a GUI
program may trigger a routine that will open another window, save data to a database
or exit the application. Many modern day programming environments provide the programmer with event templates so that the programmer only needs to supply the event code.
The second step is to bind event handlers to events so that the correct function is called when the event takes place. Graphical editors combine the first two steps: double-click on a button, and the editor creates an (empty) event handler associated with the user clicking the button and opens a text window so you can edit the event handler.
The third step in developing an event-driven program is to write the main loop. This is a function that checks for the occurrence of events, and then calls the matching event handler to process it. Most event-driven programming environments already provide this main loop, so it need not be specifically provided by the application programmer. RPG, an early programming language from IBM
, whose 1960s design concept was similar to event driven programming discussed above, provided a built-in main I/O
loop (known as the "program cycle") where the calculations responded in accordance to 'indicators' (flags
) that were set earlier in the cycle.
s as the model for interaction. The design of those toolkits has been criticized for promoting an over-simplified model of event-action, leading programmers to create error prone, difficult to extend and excessively complex application code:
. A thread context only needs a cpu stack while actively processing an event, once done the cpu can move on to process other event-driven threads, that allows an extremely large number of threads to be handled. This is essentially a Finite-state machine approach.
Computer programming
Computer programming is the process of designing, writing, testing, debugging, and maintaining the source code of computer programs. This source code is written in one or more programming languages. The purpose of programming is to create a program that performs specific operations or exhibits a...
, event-driven programming or event-based programming is a programming paradigm
Programming paradigm
A programming paradigm is a fundamental style of computer programming. Paradigms differ in the concepts and abstractions used to represent the elements of a program and the steps that compose a computation A programming paradigm is a fundamental style of computer programming. (Compare with a...
in which the flow of the program is determined by event
Event (computing)
In computing an event is an action that is usually initiated outside the scope of a program and that is handled by a piece of code inside the program. Typically events are handled synchronous with the program flow, that is, the program has one or more dedicated places where events are handled...
s—i.e., sensor
Sensor
A sensor is a device that measures a physical quantity and converts it into a signal which can be read by an observer or by an instrument. For example, a mercury-in-glass thermometer converts the measured temperature into expansion and contraction of a liquid which can be read on a calibrated...
outputs or user actions (mouse clicks, key presses) or messages
Message passing
Message passing in computer science is a form of communication used in parallel computing, object-oriented programming, and interprocess communication. In this model, processes or objects can send and receive messages to other processes...
from other programs or threads
Thread (computer science)
In computer science, a thread of execution is the smallest unit of processing that can be scheduled by an operating system. The implementation of threads and processes differs from one operating system to another, but in most cases, a thread is contained inside a process...
.
Event-driven programming can also be defined as an application architecture technique in which the application has a main loop which is clearly divided down to two sections: the first is event selection (or event detection), and the second is event handling. In embedded systems the same may be achieved using interrupt
Interrupt
In computing, an interrupt is an asynchronous signal indicating the need for attention or a synchronous event in software indicating the need for a change in execution....
s instead of a constantly running main loop; in that case the former portion of the architecture resides completely in hardware.
Event-driven programs can be written in any language, although the task is easier in languages that provide high-level abstraction
Abstraction (computer science)
In computer science, abstraction is the process by which data and programs are defined with a representation similar to its pictorial meaning as rooted in the more complex realm of human life and language with their higher need of summarization and categorization , while hiding away the...
s, such as closures
Closure (computer science)
In computer science, a closure is a function together with a referencing environment for the non-local variables of that function. A closure allows a function to access variables outside its typical scope. Such a function is said to be "closed over" its free variables...
. Some integrated development environment
Integrated development environment
An integrated development environment is a software application that provides comprehensive facilities to computer programmers for software development...
s provide code generation assistants that automate the most repetitive tasks required for event handling.
A trivial event handler
Because the code for checking for events and the main loop does not depend on the application, many programming frameworks take care of their implementation and expect the user to provide only the code for the event handlers. In this simple example there may be a call to an event handler called OnKeyEnter that includes an argument with a string of characters, corresponding to what the user typed before hitting the ENTER key. To add two numbers, storage outside the event handler must be used so the implementation might look like below.
globally declare the counter K and the integer T.
OnKeyEnter(character C)
{
convert C to a number N
if K is zero store N in T and increment K
otherwise add N to T, print the result and reset K to zero
}
While keeping track of history is straightforward in a batch program, it requires special attention and planning in an event-driven program.
Exception handlers
In some programming languages (e.g. PL/1), even though a program itself may not be predominantly event driven, certain abnormal events such as a hardwareHardware
Hardware is a general term for equipment such as keys, locks, hinges, latches, handles, wire, chains, plumbing supplies, tools, utensils, cutlery and machine parts. Household hardware is typically sold in hardware stores....
error, overflow
Overflow (software)
OVERFLOW - the OVERset grid FLOW solver - is a software package for simulating fluid flow around solid bodies using computational fluid dynamics...
or "program checks" may occur that possibly prevents further processing. Exception handlers
Exception handling
Exception handling is a programming language construct or computer hardware mechanism designed to handle the occurrence of exceptions, special conditions that change the normal flow of program execution....
may be provided by "ON statements" in (unseen) callers to provide housekeeping
Housekeeping (computing)
In computer programming, housekeeping can refer either to a standard entry or exit routine appended to a user written block of code at its entry and exit or, alternatively, to any other automated or manual software process whereby a computer is cleaned-up after usage In computer programming,...
routines to clean up afterwards before termination.
Creating event handlers
The first step in developing an event-driven program is to write a series of subroutines, or methodsMethod (computer science)
In object-oriented programming, a method is a subroutine associated with a class. Methods define the behavior to be exhibited by instances of the associated class at program run time...
, called event-handler routines. These routines handle the events to which the main program will respond. For example, a single left-button mouse-click on a command button in a GUI
Graphical user interface
In computing, a graphical user interface is a type of user interface that allows users to interact with electronic devices with images rather than text commands. GUIs can be used in computers, hand-held devices such as MP3 players, portable media players or gaming devices, household appliances and...
program may trigger a routine that will open another window, save data to a database
Database
A database is an organized collection of data for one or more purposes, usually in digital form. The data are typically organized to model relevant aspects of reality , in a way that supports processes requiring this information...
or exit the application. Many modern day programming environments provide the programmer with event templates so that the programmer only needs to supply the event code.
The second step is to bind event handlers to events so that the correct function is called when the event takes place. Graphical editors combine the first two steps: double-click on a button, and the editor creates an (empty) event handler associated with the user clicking the button and opens a text window so you can edit the event handler.
The third step in developing an event-driven program is to write the main loop. This is a function that checks for the occurrence of events, and then calls the matching event handler to process it. Most event-driven programming environments already provide this main loop, so it need not be specifically provided by the application programmer. RPG, an early programming language from IBM
IBM
International Business Machines Corporation or IBM is an American multinational technology and consulting corporation headquartered in Armonk, New York, United States. IBM manufactures and sells computer hardware and software, and it offers infrastructure, hosting and consulting services in areas...
, whose 1960s design concept was similar to event driven programming discussed above, provided a built-in main I/O
I/O
I/O may refer to:* Input/output, a system of communication for information processing systems* Input-output model, an economic model of flow prediction between sectors...
loop (known as the "program cycle") where the calculations responded in accordance to 'indicators' (flags
Flag (computing)
In computer programming, flag can refer to one or more bits that are used to store a binary value or code that has an assigned meaning, but can refer to uses of other data types...
) that were set earlier in the cycle.
Criticism and best practice
Event-driven programming is widely used in graphical user interfaces because it has been adopted by most commercial widget toolkitWidget toolkit
In computing, a widget toolkit, widget library, or GUI toolkit is a set of widgets for use in designing applications with graphical user interfaces...
s as the model for interaction. The design of those toolkits has been criticized for promoting an over-simplified model of event-action, leading programmers to create error prone, difficult to extend and excessively complex application code:
Stackless threading
An event driven approach is used in hardware description languagesHardware description language
In electronics, a hardware description language or HDL is any language from a class of computer languages, specification languages, or modeling languages for formal description and design of electronic circuits, and most-commonly, digital logic...
. A thread context only needs a cpu stack while actively processing an event, once done the cpu can move on to process other event-driven threads, that allows an extremely large number of threads to be handled. This is essentially a Finite-state machine approach.
See also
- InterruptInterruptIn computing, an interrupt is an asynchronous signal indicating the need for attention or a synchronous event in software indicating the need for a change in execution....
- Comparison of programming paradigmsComparison of programming paradigmsThis article attempts to set out the various similarities and differences between the various programming paradigms as a summary in both graphical and tabular format with links to the separate discussions concerning these similarities and differences in existing Wikipedia articles- Main paradigm...
- Dataflow programming (a similar concept)
- DOM eventsDOM EventsDOM events allow event-driven programming languages like JavaScript, JScript, ECMAScript, VBScript and Java to register various event handlers/listeners on the element nodes inside a DOM tree, e.g. HTML, XHTML, XUL and SVG documents....
- Event-driven architecture
- Event Stream ProcessingEvent Stream ProcessingEvent stream processing, or ESP, is a set of technologies designed to assist the construction of event-driven information systems. ESP technologies include event visualization, event databases, event-driven middleware, and event processing languages, or complex event processing...
(a similar concept) - Hardware Description LanguageHardware description languageIn electronics, a hardware description language or HDL is any language from a class of computer languages, specification languages, or modeling languages for formal description and design of electronic circuits, and most-commonly, digital logic...
- Inversion of controlInversion of ControlIn software engineering, Inversion of Control is an abstract principle describing an aspect of some software architecture designs in which the flow of control of a system is inverted in comparison to procedural programming....
- Message-oriented middlewareMessage-oriented middlewareMessage-oriented middleware is software or hardware infrastructure supporting sending and receiving messages between distributed systems. MOM allows application modules to be distributed over heterogeneous platforms and reduces the complexity of developing applications that span multiple...
- Programming paradigmProgramming paradigmA programming paradigm is a fundamental style of computer programming. Paradigms differ in the concepts and abstractions used to represent the elements of a program and the steps that compose a computation A programming paradigm is a fundamental style of computer programming. (Compare with a...
- Publish/subscribePublish/subscribePublish–subscribe is a messaging pattern where senders of messages, called publishers, do not program the messages to be sent directly to specific receivers, called subscribers. Published messages are characterized into classes, without knowledge of what, if any, subscribers there may be...
- Signal programmingSignal programmingSignal programming is used in the same sense as dataflow programming, and is similar to event-driven programming.The word signal is used instead of the word dataflow in documentation of such libraries as Qt, GTK+ and libsigc++...
(a similar concept) - SEDA (Staged Event-Driven Architecture)Staged event-driven architectureThe staged event-driven architecture refers to an approach to software design that decomposes a complex, event-driven application into a set of stages connected by queues. It avoids the high overhead associated with thread-based concurrency models, and decouples event and thread scheduling from...
- Virtual synchronyVirtual synchronyVirtual synchrony is an interprocess messaging passing technology. Virtual synchrony systems allow programs running in a network to organize themselves into process groups, and to send messages to groups...
, a distributed execution model for event-driven programming - QP event-driven framework for embedded systemsQP (framework)QP is a family of very lightweight, open-source, state machine frameworks for embedded processors, microcontrollers , and DSPs. The QP frameworks enable developing event-driven real-time embedded software consisting of concurrently executing UML state machines...
External links
- Description from Portland Pattern RepositoryPortland Pattern RepositoryThe Portland Pattern Repository is a repository for computer programming design patterns. It was accompanied by a companion website, WikiWikiWeb, which was the world's first wiki....
- Tutorial "Event-Driven Programming: Introduction, Tutorial, History" by Stephen Ferg
- Tutorial "Event Driven Programming" by Alan Gauld
- Article "Event Collaboration" by Martin FowlerMartin Fowler-Online presentations:* at RailsConf 2006* at JAOO 2006* at QCon London 2007 * at QCon London 2008 * at ThoughtWorks Quarterly Technology Briefing, October 2008...
- Article "Transitioning from Structured to Event-Driven Programming" by Ben WatsonBen WatsonBen Watson may refer to:*Ben Watson , , English footballer with Wigan Athletic*Ben Watson , English footballer with Eastbourne Borough...
- Article "Rethinking Swing Threading" by Jonathan SimonJonathan SimonJonathan Simon is the Associate Dean of the Jurisprudence and Social Policy Program at Boalt Hall School of Law at University of California, Berkeley, author of Governing Through Crime: How the War on Crime Transformed American Democracy and Created a Culture of Fear and Poor Discipline: Parole and...
- Article "The event driven programming style" by Chris McDonald
- Article "Event Driven Programming using Template Specialization" by Christopher Diggins
- Article "Concepts and Architecture of Vista - a Multiparadigm Programming Environment" by Stefan Schiffer and Joachim Hans Fröhlich
- Chapter "Event-Driven Programming and Agents"
- LabWindows/CVI Resources
- Comment by Tim Boudreau
- Complex Event Processing and Service Oriented Architecture http://news.tmcnet.com/news/2006/08/18/1816129.htm
- Event-driven programming and SOA: How EDA extends SOA and why it is important Jack van Hoof
- For an open source example which is in production on MSN.com and Microsoft.com, see Distributed Publish/Subscribe Event System
- For C-Code generation from UML State-Charts for Embedded Systems see Sinelabore.com
- StateWizard - A ClassWizard-like event-driven state machine framework and tool running in popular IDEs under open-source license.