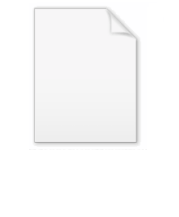
ECL programming language
Encyclopedia
The ECL programming language and system were an extensible
high-level programming language
and development environment
developed at Harvard University
in the 1970s. The name 'ECL' stood for 'Extensible Computer Language' or 'EClectic Language'. Some publications used the name 'ECL' for the entire system and 'EL/1' (Extensible Language) for the language itself.
ECL was an interactive system where programs were represented within the system; there was a compatible compiler
and interpreter. It had an ALGOL
-like syntax and an extensible data type
system, with data types as first-class citizens. Data objects were values, not references, and the calling conventions gave a choice between call by value and call by reference for each argument.
ECL was primarily used for research and teaching in programming language design, programming methodology (in particular programming by transformational refinement), and programming environment
s at Harvard, though it was said to be used at some government agencies as well. It was first implemented on the PDP-10
, with a later (interpreted-only) implementation on the PDP-11
written in BLISS
-11 and cross-compiled on the PDP-10.
of two integers according to the Euclidean algorithm
could be defined as follows:
gcd <-
EXPR(m:INT BYVAL, n: INT BYVAL; INT)
BEGIN
DECL r:INT;
REPEAT
r <- rem(m, n);
r = 0 => n;
m <- n;
n <- r;
END;
END
This is an assignment of a procedure constant to the variable
EXPR(m:INT BYVAL, n: INT BYVAL; INT)
indicates that the procedure takes two parameters, of type
r = 0 => n
when the form
In addition to the bind-class
for the actual parameter is to be passed to the formal parameter; this provides extraordinary flexibility for programmers to invent their own notations, with their own evaluation semantics, for certain procedure parameters. Bind-class
: the
representations, one for each remaining actual parameter. ECL has an
; alternatively, there are functions by which programmers can explore the nodes of the abstract syntax tree
and process them according to their own logic.
Extensible programming
Extensible programming is a term used in computer science to describe a style of computer programming that focuses on mechanisms to extend the programming language, compiler and runtime environment. Extensible programming languages, supporting this style of programming, were an active area of work...
high-level programming language
Programming language
A programming language is an artificial language designed to communicate instructions to a machine, particularly a computer. Programming languages can be used to create programs that control the behavior of a machine and/or to express algorithms precisely....
and development environment
Integrated development environment
An integrated development environment is a software application that provides comprehensive facilities to computer programmers for software development...
developed at Harvard University
Harvard University
Harvard University is a private Ivy League university located in Cambridge, Massachusetts, United States, established in 1636 by the Massachusetts legislature. Harvard is the oldest institution of higher learning in the United States and the first corporation chartered in the country...
in the 1970s. The name 'ECL' stood for 'Extensible Computer Language' or 'EClectic Language'. Some publications used the name 'ECL' for the entire system and 'EL/1' (Extensible Language) for the language itself.
ECL was an interactive system where programs were represented within the system; there was a compatible compiler
Compiler
A compiler is a computer program that transforms source code written in a programming language into another computer language...
and interpreter. It had an ALGOL
ALGOL
ALGOL is a family of imperative computer programming languages originally developed in the mid 1950s which greatly influenced many other languages and became the de facto way algorithms were described in textbooks and academic works for almost the next 30 years...
-like syntax and an extensible data type
Data type
In computer programming, a data type is a classification identifying one of various types of data, such as floating-point, integer, or Boolean, that determines the possible values for that type; the operations that can be done on values of that type; the meaning of the data; and the way values of...
system, with data types as first-class citizens. Data objects were values, not references, and the calling conventions gave a choice between call by value and call by reference for each argument.
ECL was primarily used for research and teaching in programming language design, programming methodology (in particular programming by transformational refinement), and programming environment
Integrated development environment
An integrated development environment is a software application that provides comprehensive facilities to computer programmers for software development...
s at Harvard, though it was said to be used at some government agencies as well. It was first implemented on the PDP-10
PDP-10
The PDP-10 was a mainframe computer family manufactured by Digital Equipment Corporation from the late 1960s on; the name stands for "Programmed Data Processor model 10". The first model was delivered in 1966...
, with a later (interpreted-only) implementation on the PDP-11
PDP-11
The PDP-11 was a series of 16-bit minicomputers sold by Digital Equipment Corporation from 1970 into the 1990s, one of a succession of products in the PDP series. The PDP-11 replaced the PDP-8 in many real-time applications, although both product lines lived in parallel for more than 10 years...
written in BLISS
BLISS
BLISS is a system programming language developed at Carnegie Mellon University by W. A. Wulf, D. B. Russell, and A. N. Habermann around 1970. It was perhaps the best known systems programming language right up until C made its debut a few years later. Since then, C took off and BLISS faded into...
-11 and cross-compiled on the PDP-10.
Procedures and bind-classes
An ECL procedure for computing the greatest common divisorGreatest common divisor
In mathematics, the greatest common divisor , also known as the greatest common factor , or highest common factor , of two or more non-zero integers, is the largest positive integer that divides the numbers without a remainder.For example, the GCD of 8 and 12 is 4.This notion can be extended to...
of two integers according to the Euclidean algorithm
Euclidean algorithm
In mathematics, the Euclidean algorithm is an efficient method for computing the greatest common divisor of two integers, also known as the greatest common factor or highest common factor...
could be defined as follows:
gcd <-
EXPR(m:INT BYVAL, n: INT BYVAL; INT)
BEGIN
DECL r:INT;
REPEAT
r <- rem(m, n);
r = 0 => n;
m <- n;
n <- r;
END;
END
This is an assignment of a procedure constant to the variable
gcd
. The lineEXPR(m:INT BYVAL, n: INT BYVAL; INT)
indicates that the procedure takes two parameters, of type
INT
, named m
and n
, and returns a result of type INT
. (Data types are called modes in ECL.) The bind-class BYVAL
in each parameter declaration indicates that that parameter is passed by value. The computational components of an ECL program are called forms. Some forms resemble the expressions of other programming languages and others resemble statements. The execution of a form always yields a value. The REPEAT
... END
construct is a loop form. Execution of the constructr = 0 => n
when the form
r = 0
evaluates to TRUE
causes execution of the loop to terminate with the value n
. The value of the last statement in a block (BEGIN
... END
) form becomes the value of the block form. The value of the form in a procedure declaration becomes the result of the procedure call.In addition to the bind-class
BYVAL
, ECL has bind-classes SHARED
, LIKE
, UNEVAL
, and LISTED
. Bind-class SHARED
indicates that a parameter is to be passed by reference. Bind-class LIKE
causes a parameter to be passed by reference if possible and by value if not (e.g., if the actual parameter is a pure value, or a variable to which a type conversion must be applied). Bind-class UNEVAL
specifies that an abstract syntax treeAbstract syntax tree
In computer science, an abstract syntax tree , or just syntax tree, is a tree representation of the abstract syntactic structure of source code written in a programming language. Each node of the tree denotes a construct occurring in the source code. The syntax is 'abstract' in the sense that it...
for the actual parameter is to be passed to the formal parameter; this provides extraordinary flexibility for programmers to invent their own notations, with their own evaluation semantics, for certain procedure parameters. Bind-class
LISTED
is similar to UNEVAL
, but provides a capability similar to that of varargs in CC (programming language)
C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system....
: the
LISTED
bind-class can only appear in the last formal parameter of the procedure, and that formal parameter is bound to a list of abstract syntax treeAbstract syntax tree
In computer science, an abstract syntax tree , or just syntax tree, is a tree representation of the abstract syntactic structure of source code written in a programming language. Each node of the tree denotes a construct occurring in the source code. The syntax is 'abstract' in the sense that it...
representations, one for each remaining actual parameter. ECL has an
EVAL
built-in function for evaluating an abstract syntax treeAbstract syntax tree
In computer science, an abstract syntax tree , or just syntax tree, is a tree representation of the abstract syntactic structure of source code written in a programming language. Each node of the tree denotes a construct occurring in the source code. The syntax is 'abstract' in the sense that it...
; alternatively, there are functions by which programmers can explore the nodes of the abstract syntax tree
Abstract syntax tree
In computer science, an abstract syntax tree , or just syntax tree, is a tree representation of the abstract syntactic structure of source code written in a programming language. Each node of the tree denotes a construct occurring in the source code. The syntax is 'abstract' in the sense that it...
and process them according to their own logic.