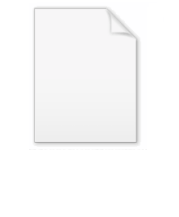
Wrapping (graphics)
Encyclopedia
In computer graphics, wrapping is the process of limiting a position to an area. A good example of wrapping is wallpaper
, a single pattern repeated indefinitely over a wall
. Wrapping is used in 3D computer graphics
to repeat a texture
over a polygon
, eliminating the need for large textures or multiple polygons.
To wrap a position x to an area of width w, calculate the value
.

where
is the highest value in the range, and
is the lowest value in the range.
Pseudocode
for wrapping of a value to a range other than 0-1 is
function wrap(X, Min, Max: Real): Real;
X := X - Int((X - Min) / (Max - Min)) * (Max - Min);
if X < 0 then //This corrects the problem caused by using Int instead of Floor
X := X + Max - Min;
return X;
Pseudocode
for wrapping of a value to a range of 0-1 is
function wrap(X: Real): Real;
X := X - Int(X);
if X < 0 then
X := X + 1;
return X;
Pseudocode
for wrapping of a value to a range of 0-1 without branching is,
function wrap(X: Real): Real;
return ((X mod 1.0) + 1.0) mod 1.0;
Wallpaper
Wallpaper is a kind of material used to cover and decorate the interior walls of homes, offices, and other buildings; it is one aspect of interior decoration. It is usually sold in rolls and is put onto a wall using wallpaper paste...
, a single pattern repeated indefinitely over a wall
Wall
A wall is a usually solid structure that defines and sometimes protects an area. Most commonly, a wall delineates a building and supports its superstructure, separates space in buildings into rooms, or protects or delineates a space in the open air...
. Wrapping is used in 3D computer graphics
3D computer graphics
3D computer graphics are graphics that use a three-dimensional representation of geometric data that is stored in the computer for the purposes of performing calculations and rendering 2D images...
to repeat a texture
Texture mapping
Texture mapping is a method for adding detail, surface texture , or color to a computer-generated graphic or 3D model. Its application to 3D graphics was pioneered by Dr Edwin Catmull in his Ph.D. thesis of 1974.-Texture mapping:...
over a polygon
Polygon
In geometry a polygon is a flat shape consisting of straight lines that are joined to form a closed chain orcircuit.A polygon is traditionally a plane figure that is bounded by a closed path, composed of a finite sequence of straight line segments...
, eliminating the need for large textures or multiple polygons.
To wrap a position x to an area of width w, calculate the value

Implementation
For computational purposes the wrapped value x of x can be expressed as
where


Pseudocode
Pseudocode
In computer science and numerical computation, pseudocode is a compact and informal high-level description of the operating principle of a computer program or other algorithm. It uses the structural conventions of a programming language, but is intended for human reading rather than machine reading...
for wrapping of a value to a range other than 0-1 is
function wrap(X, Min, Max: Real): Real;
X := X - Int((X - Min) / (Max - Min)) * (Max - Min);
if X < 0 then //This corrects the problem caused by using Int instead of Floor
X := X + Max - Min;
return X;
Pseudocode
Pseudocode
In computer science and numerical computation, pseudocode is a compact and informal high-level description of the operating principle of a computer program or other algorithm. It uses the structural conventions of a programming language, but is intended for human reading rather than machine reading...
for wrapping of a value to a range of 0-1 is
function wrap(X: Real): Real;
X := X - Int(X);
if X < 0 then
X := X + 1;
return X;
Pseudocode
Pseudocode
In computer science and numerical computation, pseudocode is a compact and informal high-level description of the operating principle of a computer program or other algorithm. It uses the structural conventions of a programming language, but is intended for human reading rather than machine reading...
for wrapping of a value to a range of 0-1 without branching is,
function wrap(X: Real): Real;
return ((X mod 1.0) + 1.0) mod 1.0;