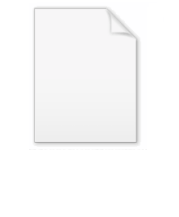
Uniform-cost search
Encyclopedia
In computer science
, uniform-cost search (UCS) is a tree search algorithm used for traversing or searching a weighted tree, tree structure
, or graph
. The search begins at the root node
. The search continues by visiting the next node which has the least total cost from the root. Nodes are visited in this manner until a goal state is reached.
Typically, the search algorithm involves expanding nodes by adding all unexpanded neighboring nodes that are connected by directed paths to a priority queue
. In the queue, each node is associated with its total path cost from the root, where the least-cost paths are given highest priority. The node at the head of the queue is subsequently expanded, adding the next set of connected nodes with the total path cost from the root to the respective node. The uniform-cost search is complete and optimal if the cost of each step exceeds some positive bound ε. The worst-case time and space complexity is O(b1 + C*/ε), where C* is the cost of the optimal solution. When all step costs are equal, this becomes O(bd + 1).
Start Node: A
Goal Node: G
Step 1
Frontier:
Explored: -
Step 2
Expand A
Frontier:
Explored: A
Step 3
Expand D
Frontier:
Explored: A D
Step 4
Expand B
Frontier:
Explored: A D B
Step 5
Expand E
Frontier:
Explored: A D B E
Step 6
Expand F
Frontier:
Explored: A D B E F
Step 7
Expand C
Frontier:
Explored: A D B E F C
Step 8
Expand G
Found the path: A to D to F to G
, which is perhaps better-known, can be regarded as a variant of uniform-cost search, where there is no goal state and processing continues until all nodes have been removed from the priority queue, i.e. until shortest paths to all nodes (not just a goal node) have been determined. As in Dijkstra's algorithm, UCS guarantees that (if all edge weights are non-negative) the shortest path to a particular node has been found once the node is extracted from the priority queue.
Uniform-cost search is a special case of the A* search algorithm if its heuristic is a constant function
. If A* is used with a monotonic heuristic, then it can be turned into a uniform cost search by subtracting from each edge cost the decrease in heuristic value along that edge. Breadth-first search
(BFS) is a special case of uniform-cost search when all edge costs are positive and identical. Where BFS first visits the node with the shortest path length (number of nodes) from the root node, UCS first visits the node with the shortest path costs (sum of edge weights) from the root node.
Uniform-cost search is a variant of best-first search
.
Computer science
Computer science or computing science is the study of the theoretical foundations of information and computation and of practical techniques for their implementation and application in computer systems...
, uniform-cost search (UCS) is a tree search algorithm used for traversing or searching a weighted tree, tree structure
Tree structure
A tree structure is a way of representing the hierarchical nature of a structure in a graphical form. It is named a "tree structure" because the classic representation resembles a tree, even though the chart is generally upside down compared to an actual tree, with the "root" at the top and the...
, or graph
Graph (data structure)
In computer science, a graph is an abstract data structure that is meant to implement the graph and hypergraph concepts from mathematics.A graph data structure consists of a finite set of ordered pairs, called edges or arcs, of certain entities called nodes or vertices...
. The search begins at the root node
Node (computer science)
A node is a record consisting of one or more fields that are links to other nodes, and a data field. The link and data fields are often implemented by pointers or references although it is also quite common for the data to be embedded directly in the node. Nodes are used to build linked, often...
. The search continues by visiting the next node which has the least total cost from the root. Nodes are visited in this manner until a goal state is reached.
Typically, the search algorithm involves expanding nodes by adding all unexpanded neighboring nodes that are connected by directed paths to a priority queue
Priority queue
A priority queue is an abstract data type in computer programming.It is exactly like a regular queue or stack data structure, but additionally, each element is associated with a "priority"....
. In the queue, each node is associated with its total path cost from the root, where the least-cost paths are given highest priority. The node at the head of the queue is subsequently expanded, adding the next set of connected nodes with the total path cost from the root to the respective node. The uniform-cost search is complete and optimal if the cost of each step exceeds some positive bound ε. The worst-case time and space complexity is O(b1 + C*/ε), where C* is the cost of the optimal solution. When all step costs are equal, this becomes O(bd + 1).
Pseudocode
procedure UniformCostSearch(Graph, root, goal)- node := root, cost = 0
- frontier := priority queue containing node only
- explored := empty set
- do
- if frontier is empty
- return failure
- node := frontier.pop
- if node is goal
- return solution
- explored.add(node)
- for each of node's neighbors n
- if n is not in explored
- if n is not in frontier
- frontier.add(n)
- if n is in frontier with higher cost
- replace existing node with n
- if n is not in frontier
- if n is not in explored
- if frontier is empty
Example
Expansion showing the explored set and the frontier (priority queue):Start Node: A
Goal Node: G
Step 1
Frontier:
Node | A |
Cost | 0 |
Explored: -
Step 2
Expand A
Frontier:
Node | D | B |
Cost | 3 | 5 |
Explored: A
Step 3
Expand D
Frontier:
Node | B | E | F |
Cost | 5 | 5 | 5 |
Explored: A D
Step 4
Expand B
Frontier:
Node | E | F | C |
Cost | 5 | 5 | 6 |
Explored: A D B
Step 5
Expand E
Frontier:
Node | F | C |
Cost | 5 | 6 |
Explored: A D B E
-
- Note: B was not added to the priority queue because it was already explored
Step 6
Expand F
Frontier:
Node | C | G |
Cost | 6 | 8 |
Explored: A D B E F
Step 7
Expand C
Frontier:
Node | G |
Cost | 8 |
Explored: A D B E F C
Step 8
Expand G
Found the path: A to D to F to G
Relationship to other algorithms
Dijkstra's algorithmDijkstra's algorithm
Dijkstra's algorithm, conceived by Dutch computer scientist Edsger Dijkstra in 1956 and published in 1959, is a graph search algorithm that solves the single-source shortest path problem for a graph with nonnegative edge path costs, producing a shortest path tree...
, which is perhaps better-known, can be regarded as a variant of uniform-cost search, where there is no goal state and processing continues until all nodes have been removed from the priority queue, i.e. until shortest paths to all nodes (not just a goal node) have been determined. As in Dijkstra's algorithm, UCS guarantees that (if all edge weights are non-negative) the shortest path to a particular node has been found once the node is extracted from the priority queue.
Uniform-cost search is a special case of the A* search algorithm if its heuristic is a constant function
Constant function
In mathematics, a constant function is a function whose values do not vary and thus are constant. For example the function f = 4 is constant since f maps any value to 4...
. If A* is used with a monotonic heuristic, then it can be turned into a uniform cost search by subtracting from each edge cost the decrease in heuristic value along that edge. Breadth-first search
Breadth-first search
In graph theory, breadth-first search is a graph search algorithm that begins at the root node and explores all the neighboring nodes...
(BFS) is a special case of uniform-cost search when all edge costs are positive and identical. Where BFS first visits the node with the shortest path length (number of nodes) from the root node, UCS first visits the node with the shortest path costs (sum of edge weights) from the root node.
Uniform-cost search is a variant of best-first search
Best-first search
Best-first search is a search algorithm which explores a graph by expanding the most promising node chosen according to a specified rule.Judea Pearl described best-first search as estimating the promise of node n by a "heuristic evaluation function f which, in general, may depend on the description...
.