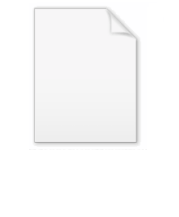
Software design
Encyclopedia
Software design is a process of problem solving and planning for a software solution. After the purpose and specifications of software are determined, software developer
s will design
or employ designer
s to develop a plan for a solution. It includes low-level component and algorithm
implementation issues as well as the architectural
view.
yields specifications that are used in software engineering
. If the software is "semiautomated" or user centered, software design may involve user experience design
yielding a story board to help determine those specifications. If the software is completely automated
(meaning no user
or user interface
), a software design may be as simple as a flow chart or text describing a planned sequence of events. There are also semi-standard methods like Unified Modeling Language
and Fundamental modeling concepts
. In either case some documentation
of the plan is usually the product of the design.
A software design may be platform-independent
or platform-specific
, depending on the availability of the technology called for by the design.
Software design can be considered as putting solution to the problem(s) in hand using the available capabilities. Hence the main difference between Software analysis and design is that the output of the analysis of a software problem will be smaller problems to solve and it should not deviate so much even if it is conducted by different team members or even by entirely different groups. But since design depends on the capabilities, we can have different designs for the same problem depending on the capabilities of the environment that will host the solution (whether it is some OS, web , mobile or even the new cloud computing paradigm). The solution will depend also on the used development environment (Whether you build a solution from scratch or using reliable frameworks or at least implement some suitable design patterns
)
is any artificial language that can be used to express information or knowledge or systems in a structure that is defined by a consistent set of rules. The rules are used for interpretation of the meaning of components in the structure. A modeling language can be graphical or textual. Examples of graphical modeling languages for software design are:
. The reuse of such patterns can speed up the software development process, having been tested and proven in the past.
or prototype
. It is possible to design software in the process of programming, without a plan or requirement analysis, but for more complex projects this would not be considered a professional approach. A separate design prior to programming allows for multidisciplinary designers and Subject Matter Expert
s (SMEs) to collaborate with highly-skilled programmers for software that is both useful and technically sound.
Software developer
A software developer is a person concerned with facets of the software development process. Their work includes researching, designing, developing, and testing software. A software developer may take part in design, computer programming, or software project management...
s will design
Design
Design as a noun informally refers to a plan or convention for the construction of an object or a system while “to design” refers to making this plan...
or employ designer
Designer
A designer is a person who designs. More formally, a designer is an agent that "specifies the structural properties of a design object". In practice, anyone who creates tangible or intangible objects, such as consumer products, processes, laws, games and graphics, is referred to as a...
s to develop a plan for a solution. It includes low-level component and algorithm
Algorithm
In mathematics and computer science, an algorithm is an effective method expressed as a finite list of well-defined instructions for calculating a function. Algorithms are used for calculation, data processing, and automated reasoning...
implementation issues as well as the architectural
Software architecture
The software architecture of a system is the set of structures needed to reason about the system, which comprise software elements, relations among them, and properties of both...
view.
Overview
The software requirements analysis (SRA) step of a software development processSoftware development process
A software development process, also known as a software development life cycle , is a structure imposed on the development of a software product. Similar terms include software life cycle and software process. It is often considered a subset of systems development life cycle...
yields specifications that are used in software engineering
Software engineering
Software Engineering is the application of a systematic, disciplined, quantifiable approach to the development, operation, and maintenance of software, and the study of these approaches; that is, the application of engineering to software...
. If the software is "semiautomated" or user centered, software design may involve user experience design
User experience design
User experience design is a subset of the field of experience design that pertains to the creation of the architecture and interaction models that affect user experience of a device or system...
yielding a story board to help determine those specifications. If the software is completely automated
Automation
Automation is the use of control systems and information technologies to reduce the need for human work in the production of goods and services. In the scope of industrialization, automation is a step beyond mechanization...
(meaning no user
User (computing)
A user is an agent, either a human agent or software agent, who uses a computer or network service. A user often has a user account and is identified by a username , screen name , nickname , or handle, which is derived from the identical Citizen's Band radio term.Users are...
or user interface
User interface
The user interface, in the industrial design field of human–machine interaction, is the space where interaction between humans and machines occurs. The goal of interaction between a human and a machine at the user interface is effective operation and control of the machine, and feedback from the...
), a software design may be as simple as a flow chart or text describing a planned sequence of events. There are also semi-standard methods like Unified Modeling Language
Unified Modeling Language
Unified Modeling Language is a standardized general-purpose modeling language in the field of object-oriented software engineering. The standard is managed, and was created, by the Object Management Group...
and Fundamental modeling concepts
Fundamental modeling concepts
Fundamental Modeling Concepts provide a framework to describe software-intensive systems. It strongly emphasizes the communication about software-intensive systems by using a semi-formal graphical notation that can easily be understood.- Introduction :...
. In either case some documentation
Documentation
Documentation is a term used in several different ways. Generally, documentation refers to the process of providing evidence.Modules of Documentation are Helpful...
of the plan is usually the product of the design.
A software design may be platform-independent
Platform-independent model
A Platform-Independent Model in software engineering is a model of a software system or business system, that is independent of the specific technological platform used to implement it...
or platform-specific
Platform-specific model
A platform-specific model is a model of a software or business system that is linked to a specific technological platform . Platform-specific models are indispensable for the actual implementation of a system.For example, a need to implement an online shop...
, depending on the availability of the technology called for by the design.
Software design can be considered as putting solution to the problem(s) in hand using the available capabilities. Hence the main difference between Software analysis and design is that the output of the analysis of a software problem will be smaller problems to solve and it should not deviate so much even if it is conducted by different team members or even by entirely different groups. But since design depends on the capabilities, we can have different designs for the same problem depending on the capabilities of the environment that will host the solution (whether it is some OS, web , mobile or even the new cloud computing paradigm). The solution will depend also on the used development environment (Whether you build a solution from scratch or using reliable frameworks or at least implement some suitable design patterns
Design Patterns
Design Patterns: Elements of Reusable Object-Oriented Software is a software engineering book describing recurring solutions to common problems in software design. The book's authors are Erich Gamma, Richard Helm, Ralph Johnson and John Vlissides with a foreword by Grady Booch. The authors are...
)
Design concepts
The design concepts provide the software designer with a foundation from which more sophisticated methods can be applied. A set of fundamental design concepts has evolved. They are:- AbstractionAbstractionAbstraction is a process by which higher concepts are derived from the usage and classification of literal concepts, first principles, or other methods....
- Abstraction is the process or result of generalization by reducing the information content of a concept or an observable phenomenon, typically in order to retain only information which is relevant for a particular purpose. - Refinement - It is the process of elaboration. A hierarchy is developed by decomposing a macroscopic statement of function in a stepwise fashion until programming language statements are reached. In each step, one or several instructions of a given program are decomposed into more detailed instructions. Abstraction and Refinement are complementary concepts.
- ModularityModularityModularity is a general systems concept, typically defined as a continuum describing the degree to which a system’s components may be separated and recombined. It refers to both the tightness of coupling between components, and the degree to which the “rules” of the system architecture enable the...
- Software architecture is divided into components called modules. - Software ArchitectureSoftware architectureThe software architecture of a system is the set of structures needed to reason about the system, which comprise software elements, relations among them, and properties of both...
- It refers to the overall structure of the software and the ways in which that structure provides conceptual integrity for a system. A good software architecture will yield a good return on investment with respect to the desired outcome of the project, e.g. in terms of performance, quality, schedule and cost. - Control Hierarchy - A program structure that represents the organization of a program component and implies a hierarchy of control.
- Structural Partitioning - The program structure can be divided both horizontally and vertically. Horizontal partitions define separate branches of modular hierarchy for each major program function. Vertical partitioning suggests that control and work should be distributed top down in the program structure.
- Data StructureData structureIn computer science, a data structure is a particular way of storing and organizing data in a computer so that it can be used efficiently.Different kinds of data structures are suited to different kinds of applications, and some are highly specialized to specific tasks...
- It is a representation of the logical relationship among individual elements of data. - Software Procedure - It focuses on the processing of each modules individually
- Information HidingInformation hidingIn computer science, information hiding is the principle of segregation of the design decisions in a computer program that are most likely to change, thus protecting other parts of the program from extensive modification if the design decision is changed...
- Modules should be specified and designed so that information contained within a module is inaccessible to other modules that have no need for such information.
Design considerations
There are many aspects to consider in the design of a piece of software. The importance of each should reflect the goals the software is trying to achieve. Some of these aspects are:- Compatibility - The software is able to operate with other products that are designed for interoperability with another product. For example, a piece of software may be backward-compatible with an older version of itself.
- ExtensibilityExtensibilityIn software engineering, extensibility is a system design principle where the implementation takes into consideration future growth. It is a systemic measure of the ability to extend a system and the level of effort required to implement the extension...
- New capabilities can be added to the software without major changes to the underlying architecture. - Fault-tolerance - The software is resistant to and able to recover from component failure.
- MaintainabilityMaintainabilityIn engineering, maintainability is the ease with which a product can be maintained in order to:* isolate defects or their cause* correct defects or their cause* meet new requirements* make future maintenance easier, or* cope with a changed environment...
- The software can be restored to a specified condition within a specified period of time. For example, antivirus software may include the ability to periodically receive virus definition updates in order to maintain the software's effectiveness. - ModularityModularityModularity is a general systems concept, typically defined as a continuum describing the degree to which a system’s components may be separated and recombined. It refers to both the tightness of coupling between components, and the degree to which the “rules” of the system architecture enable the...
- the resulting software comprises well defined, independent components. That leads to better maintainability. The components could be then implemented and tested in isolation before being integrated to form a desired software system. This allows division of work in a software development project. - Packaging - Printed material such as the box and manuals should match the style designated for the target market and should enhance usability. All compatibility information should be visible on the outside of the package. All components required for use should be included in the package or specified as a requirement on the outside of the package.
- Reliability - The software is able to perform a required function under stated conditions for a specified period of time.
- ReusabilityReusabilityIn computer science and software engineering, reusability is the likelihood a segment of source code that can be used again to add new functionalities with slight or no modification...
- the software is able to add further features and modification with slight or no modification. - RobustnessFault-tolerant systemFault-tolerance or graceful degradation is the property that enables a system to continue operating properly in the event of the failure of some of its components. A newer approach is progressive enhancement...
- The software is able to operate under stress or tolerate unpredictable or invalid input. For example, it can be designed with a resilience to low memory conditions. - SecurityComputer securityComputer security is a branch of computer technology known as information security as applied to computers and networks. The objective of computer security includes protection of information and property from theft, corruption, or natural disaster, while allowing the information and property to...
- The software is able to withstand hostile acts and influences. - UsabilityUsabilityUsability is the ease of use and learnability of a human-made object. The object of use can be a software application, website, book, tool, machine, process, or anything a human interacts with. A usability study may be conducted as a primary job function by a usability analyst or as a secondary job...
- The software user interfaceUser interfaceThe user interface, in the industrial design field of human–machine interaction, is the space where interaction between humans and machines occurs. The goal of interaction between a human and a machine at the user interface is effective operation and control of the machine, and feedback from the...
must be usable for its target user/audience. Default values for the parameters must be chosen so that they are a good choice for the majority of the users.
Modeling language
A modeling languageModeling language
A modeling language is any artificial language that can be used to express information or knowledge or systems in a structure that is defined by a consistent set of rules...
is any artificial language that can be used to express information or knowledge or systems in a structure that is defined by a consistent set of rules. The rules are used for interpretation of the meaning of components in the structure. A modeling language can be graphical or textual. Examples of graphical modeling languages for software design are:
- Business Process Modeling NotationBusiness Process Modeling NotationBusiness Process Model and Notation is a graphical representation for specifying business processes in a business process model. It was previously known as Business Process Modeling Notation....
(BPMN) is an example of a Process ModelingProcess modelingThe term process model is used in various contexts. For example, in business process modeling the enterprise process model is often referred to as the business process model. Process models are core concepts in the discipline of process engineering....
language. - EXPRESS and EXPRESS-G (ISO 10303-11) is an international standard general-purpose data modelingData modelingData modeling in software engineering is the process of creating a data model for an information system by applying formal data modeling techniques.- Overview :...
language. - Extended Enterprise Modeling LanguageExtended Enterprise Modeling LanguageExtended Enterprise Modeling Language in software engineering is a modelling language used for Enterprise modelling across a number of layers.-Overview:...
(EEML) is commonly used for business process modeling across a number of layers. - FlowchartFlowchartA flowchart is a type of diagram that represents an algorithm or process, showing the steps as boxes of various kinds, and their order by connecting these with arrows. This diagrammatic representation can give a step-by-step solution to a given problem. Process operations are represented in these...
is a schematic representation of an algorithm or a stepwise process, - Fundamental Modeling ConceptsFundamental modeling conceptsFundamental Modeling Concepts provide a framework to describe software-intensive systems. It strongly emphasizes the communication about software-intensive systems by using a semi-formal graphical notation that can easily be understood.- Introduction :...
(FMC) modeling language for software-intensive systems. - IDEFIDEFIDEF, an abbreviation of Integration Definition, refers to a family of modeling languages in the field of systems and software engineering. They cover a wide range of uses, from functional modeling to data, simulation, object-oriented analysis/design and knowledge acquisition. These "definition...
is a family of modeling languages, the most notable of which include IDEF0IDEF0IDEF0 is a function modeling methodology for describing manufacturing functions, which offers a functional modeling language for the analysis, development, reengineering, and integration of information systems; business processes; or software engineering analysis.IDEF0 is part of the IDEF family...
for functional modeling, IDEF1XIDEF1XIDEF1X is a data modeling language for the developing of semantic data models. IDEF1X is used to produce a graphical information model which represents the structure and semantics of information within an environment or system.IDEF1X permits the construction of semantic data models which may serve...
for information modeling, and IDEF5IDEF5IDEF5 is a software engineering method to develop and maintain usable, accurate, domain ontologies...
for modeling ontologies. - Jackson Structured ProgrammingJackson Structured ProgrammingJackson Structured Programming or JSP is a method for structured programming based on correspondences between data stream structure and program structure...
(JSP) is a method for structured programming based on correspondences between data stream structure and program structure - LePUS3Lepus3LePUS3 is a language for modelling and visualizing object-oriented programs and design patterns. It is defined as a formal specification language, formulated as an axiomatized subset of First-order predicate logic. A diagram in LePUS3 is also called a Codechart...
is an object-oriented visual Design Description Language and a formal specificationFormal specificationIn computer science, a formal specification is a mathematical description of software or hardware that may be used to develop an implementation. It describes what the system should do, not how the system should do it...
language that is suitable primarily for modelling large object-oriented (JavaJava (programming language)Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities...
, C++C++C++ is a statically typed, free-form, multi-paradigm, compiled, general-purpose programming language. It is regarded as an intermediate-level language, as it comprises a combination of both high-level and low-level language features. It was developed by Bjarne Stroustrup starting in 1979 at Bell...
, C#) programs and design patternsDesign PatternsDesign Patterns: Elements of Reusable Object-Oriented Software is a software engineering book describing recurring solutions to common problems in software design. The book's authors are Erich Gamma, Richard Helm, Ralph Johnson and John Vlissides with a foreword by Grady Booch. The authors are...
. - Unified Modeling LanguageUnified Modeling LanguageUnified Modeling Language is a standardized general-purpose modeling language in the field of object-oriented software engineering. The standard is managed, and was created, by the Object Management Group...
(UML) is a general modeling language to describe software both structurally and behaviorally. It has a graphical notation and allows for extension with a Profile (UML)Profile (UML)A profile in the Unified Modeling Language provides a generic extension mechanism for customizing UML models for particular domains and platforms...
. - Alloy (specification language)Alloy (specification language)In computer science and software engineering, Alloy is a declarative specification language for expressing complex structural constraints and behavior in a software system. Alloy provides a simple structural modeling tool based on first-order logic...
is a general purpose specification language for expressing complex structural constraints and behavior in a software system. It provides a concise language based on first-order relational logic. - Systems Modeling LanguageSystems Modeling LanguageThe Systems Modeling Language is a general-purpose modeling language for systems engineering applications. It supports the specification, analysis, design, verification and validation of a broad range of systems and systems-of-systems. SysML was originally developed by an open source specification...
(SysML) is a new general-purpose modelingGeneral-purpose modelingGeneral-purpose modeling is the systematic use of a general-purpose modeling language to represent the various facets of an object or a system...
language for systems engineering.
Design patterns
A software designer or architect may identify a design problem which has been solved by others before. A template or pattern describing a solution to a common problem is known as a design patternDesign pattern (computer science)
In software engineering, a design pattern is a general reusable solution to a commonly occurring problem within a given context in software design. A design pattern is not a finished design that can be transformed directly into code. It is a description or template for how to solve a problem that...
. The reuse of such patterns can speed up the software development process, having been tested and proven in the past.
Usage
Software design documentation may be reviewed or presented to allow constraints, specifications and even requirements to be adjusted prior to programming. Redesign may occur after review of a programmed simulationSimulation
Simulation is the imitation of some real thing available, state of affairs, or process. The act of simulating something generally entails representing certain key characteristics or behaviours of a selected physical or abstract system....
or prototype
Prototype
A prototype is an early sample or model built to test a concept or process or to act as a thing to be replicated or learned from.The word prototype derives from the Greek πρωτότυπον , "primitive form", neutral of πρωτότυπος , "original, primitive", from πρῶτος , "first" and τύπος ,...
. It is possible to design software in the process of programming, without a plan or requirement analysis, but for more complex projects this would not be considered a professional approach. A separate design prior to programming allows for multidisciplinary designers and Subject Matter Expert
Subject Matter Expert
A subject matter expert or domain expert is a person who is an expert in a particular area or topic. When spoken, sometimes the acronym "SME" is spelled out and other times voiced as a word ....
s (SMEs) to collaborate with highly-skilled programmers for software that is both useful and technically sound.
See also
- Aspect-oriented software developmentAspect-oriented software developmentIn computing, Aspect-oriented software development is an emerging software development technology that seeks new modularizations of software systems in order to isolate secondary or supporting functions from the main program's business logic...
- Bachelor of Science in Information TechnologyBachelor of Science in Information TechnologyA Bachelor of Science in Information Technology, , is a bachelor's degree awarded for the completion of an undergraduate course or program in information technology....
- Common Layers in an Information System Logical ArchitectureCommon layers in an information system logical architectureThe following four layers are the most common layers in a logical multilayered architecture for an information system with an object-oriented design:* User Interface Layer...
- Design rationaleDesign RationaleA Design Rationale is an explicit documentation of the reasons behind decisions made when designing a system or artifact. As initially developed by W.R...
- Interaction designInteraction designIn design, human–computer interaction, and software development, interaction design, often abbreviated IxD, is "the practice of designing interactive digital products, environments, systems, and services." Like many other design fields interaction design also has an interest in form but its main...
- Icon designIcon designIcon design is the process of designing a graphic symbol that represents some real, fantasy or abstract motive, entity or action. In the context of software applications, an icon often represents a program, a function, data or a collection of data on a computer system.Icon designs can be simple,...
- Search-based software engineeringSearch-based software engineeringSearch-based software engineering is an approach to apply metaheuristic search techniques like genetic algorithms, simulated annealing and tabu search to software engineering problems. It is inspired by the observation that many activities in software engineering can be formulated as optimization...
- Software architectureSoftware architectureThe software architecture of a system is the set of structures needed to reason about the system, which comprise software elements, relations among them, and properties of both...
- Software Design DescriptionSoftware Design DescriptionIEEE 1016-1998, also known as the Recommended Practice for Software Design Descriptions, is an IEEE standard that specifies an organizational structure for a software design description...
(IEEE 1016) - Software developmentSoftware developmentSoftware development is the development of a software product...
- User experienceUser experienceUser experience is the way a person feels about using a product, system or service. User experience highlights the experiential, affective, meaningful and valuable aspects of human-computer interaction and product ownership, but it also includes a person’s perceptions of the practical aspects such...
- User interface designUser interface designUser interface design or user interface engineering is the design of computers, appliances, machines, mobile communication devices, software applications, and websites with the focus on the user's experience and interaction...
- Zero One InfinityZero One InfinityThe Zero one or infinity rule is a rule of thumb in software design originated by early computing pioneer Willem van der Poel. It suggests that arbitrary limits on the number of instances of a particular entity should not be allowed...