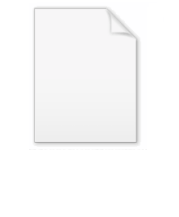
SIGFPE
Encyclopedia
On POSIX
compliant platforms, SIGFPE is the signal
sent to a process
when it performs an erroneous arithmetic operation. The symbolic constant
for SIGFPE is defined in the header file
for signal names; FPE is an acronym for floating-point exception. Although SIGFPE does not necessarily involve floating-point arithmetic, there is no way to change its name without breaking backward compatibility
.
SIGFPE can be handled. That is, programmers can specify the action they want to occur on receiving the signal, such as calling a subroutine
, ignoring the event, or restoring the default action.
Under certain circumstances, ignoring SIGFPE can result in undefined behaviour
. In particular, the program may hang as the offending operation is forever retried. However, it is safe to ignore SIGFPE signals not actually resulting from computation, such as those sent via the
.
A common oversight is to consider division by zero the only source of SIGFPE conditions. On some architectures (IA-32 includedhttp://www.hardtoc.com/archives/119), integer division of INT_MIN (the most negative representable integer value) by −1 triggers the signal because the quotient, a positive number, is not representable.
In contrast to that in C, SIGFPE (or any other signal) will never be issued for unsigned integer types. These are guaranteed by the C standard
to wrap around silently. Computation involving such types is always defined modulo M+1, where M is the maximum value representable in the type.
program that attempts to perform an erroneous arithmetic operation, namely integer
division by zero
, or FPE_INTDIV.
int main(void)
{
/* "volatile" needed to eliminate compile-time optimizations */
volatile int x = 42;
volatile int y = 0;
x=x/y;
return 0; /* Never reached */
}
Another example:
#include
int main(void)
{
volatile int x=INT_MIN;
volatile int y=-1;
x=x/y;
return 0;
}
Compiling and running either example on IA-32
with Linux
produces the following:
$ gcc -o sigfpe sigfpe.c
sigfpe.c: In function ‘main’:
sigfpe.c:3: warning: division by zero
$ ./sigfpe
Floating point exception (core dumped)
A backtrace from gdb shows that the SIGFPE signal occurred in the
Program received signal SIGFPE, Arithmetic exception.
0x08048373 in main
Compare the output from SIGFPE with that of a segmentation fault or the SIGILL signal for illegal instructions.
POSIX
POSIX , an acronym for "Portable Operating System Interface", is a family of standards specified by the IEEE for maintaining compatibility between operating systems...
compliant platforms, SIGFPE is the signal
Signal (computing)
A signal is a limited form of inter-process communication used in Unix, Unix-like, and other POSIX-compliant operating systems. Essentially it is an asynchronous notification sent to a process in order to notify it of an event that occurred. When a signal is sent to a process, the operating system...
sent to a process
Process (computing)
In computing, a process is an instance of a computer program that is being executed. It contains the program code and its current activity. Depending on the operating system , a process may be made up of multiple threads of execution that execute instructions concurrently.A computer program is a...
when it performs an erroneous arithmetic operation. The symbolic constant
C preprocessor
The C preprocessor is the preprocessor for the C and C++ computer programming languages. The preprocessor handles directives for source file inclusion , macro definitions , and conditional inclusion ....
for SIGFPE is defined in the header file
Header file
Some programming languages use header files. These files allow programmers to separate certain elements of a program's source code into reusable files. Header files commonly contain forward declarations of classes, subroutines, variables, and other identifiers...
signal.hSignal.hsignal.h is a header file defined in the C Standard Library to specify how a program handles signals while it executes. A signal can report some exceptional behavior within the program , or a signal can report some asynchronous event outside the program .A signal can be generated...
.Etymology
SIG is a common prefixPrefix
A prefix is an affix which is placed before the root of a word. Particularly in the study of languages,a prefix is also called a preformative, because it alters the form of the words to which it is affixed.Examples of prefixes:...
for signal names; FPE is an acronym for floating-point exception. Although SIGFPE does not necessarily involve floating-point arithmetic, there is no way to change its name without breaking backward compatibility
Backward compatibility
In the context of telecommunications and computing, a device or technology is said to be backward or downward compatible if it can work with input generated by an older device...
.
Description
SIGFPE is sent to processes for a variety of reasons. A common example might be an unexpected type overflow (e.g., signed integer) owing to exceptional input, or an error in a program construct.SIGFPE can be handled. That is, programmers can specify the action they want to occur on receiving the signal, such as calling a subroutine
Subroutine
In computer science, a subroutine is a portion of code within a larger program that performs a specific task and is relatively independent of the remaining code....
, ignoring the event, or restoring the default action.
Under certain circumstances, ignoring SIGFPE can result in undefined behaviour
Undefined behaviour
In computer programming, undefined behavior is a feature of some programming languages—most famously C. In these languages, to simplify the specification and allow some flexibility in implementation, the specification leaves the results of certain operations specifically undefined.For...
. In particular, the program may hang as the offending operation is forever retried. However, it is safe to ignore SIGFPE signals not actually resulting from computation, such as those sent via the
killKill (Unix)In computing, kill is a command that is used in several popular operating systems to send signals to running processes, for example to request the termination of this process.-Unix and Unix-like:...
system callSystem call
In computing, a system call is how a program requests a service from an operating system's kernel. This may include hardware related services , creating and executing new processes, and communicating with integral kernel services...
.
A common oversight is to consider division by zero the only source of SIGFPE conditions. On some architectures (IA-32 includedhttp://www.hardtoc.com/archives/119), integer division of INT_MIN (the most negative representable integer value) by −1 triggers the signal because the quotient, a positive number, is not representable.
In contrast to that in C, SIGFPE (or any other signal) will never be issued for unsigned integer types. These are guaranteed by the C standard
C99
C99 is a modern dialect of the C programming language. It extends the previous version with new linguistic and library features, and helps implementations make better use of available computer hardware and compiler technology.-History:...
to wrap around silently. Computation involving such types is always defined modulo M+1, where M is the maximum value representable in the type.
Example
Here is an example of an ANSI CANSI C
ANSI C refers to the family of successive standards published by the American National Standards Institute for the C programming language. Software developers writing in C are encouraged to conform to the standards, as doing so aids portability between compilers.-History and outlook:The first...
program that attempts to perform an erroneous arithmetic operation, namely integer
Integer
The integers are formed by the natural numbers together with the negatives of the non-zero natural numbers .They are known as Positive and Negative Integers respectively...
division by zero
Division by zero
In mathematics, division by zero is division where the divisor is zero. Such a division can be formally expressed as a / 0 where a is the dividend . Whether this expression can be assigned a well-defined value depends upon the mathematical setting...
, or FPE_INTDIV.
int main(void)
{
/* "volatile" needed to eliminate compile-time optimizations */
volatile int x = 42;
volatile int y = 0;
x=x/y;
return 0; /* Never reached */
}
Another example:
#include
int main(void)
{
volatile int x=INT_MIN;
volatile int y=-1;
x=x/y;
return 0;
}
Compiling and running either example on IA-32
IA-32
IA-32 , also known as x86-32, i386 or x86, is the CISC instruction-set architecture of Intel's most commercially successful microprocessors, and was first implemented in the Intel 80386 as a 32-bit extension of x86 architecture...
with Linux
Linux
Linux is a Unix-like computer operating system assembled under the model of free and open source software development and distribution. The defining component of any Linux system is the Linux kernel, an operating system kernel first released October 5, 1991 by Linus Torvalds...
produces the following:
$ gcc -o sigfpe sigfpe.c
sigfpe.c: In function ‘main’:
sigfpe.c:3: warning: division by zero
$ ./sigfpe
Floating point exception (core dumped)
A backtrace from gdb shows that the SIGFPE signal occurred in the
main
function:Program received signal SIGFPE, Arithmetic exception.
0x08048373 in main
Compare the output from SIGFPE with that of a segmentation fault or the SIGILL signal for illegal instructions.