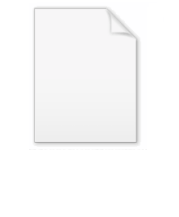
PCASTL
Encyclopedia
The PCASTL is an interpreted high-level programming language
. It was created in 2008 by Philippe Choquette. The PCASTL is designed to ease the writing of self-modifying code
. The language has reserved word
s parent and childset to access the nodes of the syntax tree
of the currently written code.
" is quite simple:
or
will do the same.
and R
. The source of R
version 2.5.1 has been studied to write the grammar
and the lexer used in the PCASTL interpreter.
, statements can, but do not have to, be separated by semicolon
s. Like in R
, a variable
can change type in a session
. Like in C
and R
, PCASTL uses balanced bracket
s ({ and }) to make blocks.
Operators
found in PCASTL have the same precedence
and associativity
as their counterparts in C
. for loops are defined like in C
.
are used like in C
to increment
or decrement
a variable before or after it is used in its expression.
An example of PCASTL using the for reserved word
and the
:
Functions
and comments in PCASTL are defined like in R
:
s can only be written lowercase and will not be recognized otherwise. The parent reserved word gives a reference
to the parent node in the syntax tree
of the code where the word is placed. In the following code, the parent node is the operator
The variable
"a" will hold a reference
to the
to the two child nodes of the operator
.
To display the value of "a", some ways are given in this example:
In the following code: we assign a code segment to the right child of the
High-level programming language
A high-level programming language is a programming language with strong abstraction from the details of the computer. In comparison to low-level programming languages, it may use natural language elements, be easier to use, or be from the specification of the program, making the process of...
. It was created in 2008 by Philippe Choquette. The PCASTL is designed to ease the writing of self-modifying code
Self-modifying code
In computer science, self-modifying code is code that alters its own instructions while it is executing - usually to reduce the instruction path length and improve performance or simply to reduce otherwise repetitively similar code, thus simplifying maintenance...
. The language has reserved word
Reserved word
Reserved words are one type of grammatical construct in programming languages. These words have special meaning within the language and are predefined in the language’s formal specifications...
s parent and childset to access the nodes of the syntax tree
Abstract syntax tree
In computer science, an abstract syntax tree , or just syntax tree, is a tree representation of the abstract syntactic structure of source code written in a programming language. Each node of the tree denotes a construct occurring in the source code. The syntax is 'abstract' in the sense that it...
of the currently written code.
Hello world
The "Hello world programHello world program
A "Hello world" program is a computer program that outputs "Hello world" on a display device. Because it is typically one of the simplest programs possible in most programming languages, it is by tradition often used to illustrate to beginners the most basic syntax of a programming language, or to...
" is quite simple:
"Hello, world!"
or
print("Hello, world!")
will do the same.
Syntax
The syntax of PCASTL is derived from programming languages CC (programming language)
C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system....
and R
R (programming language)
R is a programming language and software environment for statistical computing and graphics. The R language is widely used among statisticians for developing statistical software, and R is widely used for statistical software development and data analysis....
. The source of R
R (programming language)
R is a programming language and software environment for statistical computing and graphics. The R language is widely used among statisticians for developing statistical software, and R is widely used for statistical software development and data analysis....
version 2.5.1 has been studied to write the grammar
Formal grammar
A formal grammar is a set of formation rules for strings in a formal language. The rules describe how to form strings from the language's alphabet that are valid according to the language's syntax...
and the lexer used in the PCASTL interpreter.
Influences
Like in RR (programming language)
R is a programming language and software environment for statistical computing and graphics. The R language is widely used among statisticians for developing statistical software, and R is widely used for statistical software development and data analysis....
, statements can, but do not have to, be separated by semicolon
Semicolon
The semicolon is a punctuation mark with several uses. The Italian printer Aldus Manutius the Elder established the practice of using the semicolon to separate words of opposed meaning and to indicate interdependent statements. "The first printed semicolon was the work of ... Aldus Manutius"...
s. Like in R
R (programming language)
R is a programming language and software environment for statistical computing and graphics. The R language is widely used among statisticians for developing statistical software, and R is widely used for statistical software development and data analysis....
, a variable
Variable (programming)
In computer programming, a variable is a symbolic name given to some known or unknown quantity or information, for the purpose of allowing the name to be used independently of the information it represents...
can change type in a session
Session (computer science)
In computer science, in particular networking, a session is a semi-permanent interactive information interchange, also known as a dialogue, a conversation or a meeting, between two or more communicating devices, or between a computer and user . A session is set up or established at a certain point...
. Like in C
C (programming language)
C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system....
and R
R (programming language)
R is a programming language and software environment for statistical computing and graphics. The R language is widely used among statisticians for developing statistical software, and R is widely used for statistical software development and data analysis....
, PCASTL uses balanced bracket
Bracket
Brackets are tall punctuation marks used in matched pairs within text, to set apart or interject other text. In the United States, "bracket" usually refers specifically to the "square" or "box" type.-List of types:...
s ({ and }) to make blocks.
Operators
Operator (programming)
Programming languages typically support a set of operators: operations which differ from the language's functions in calling syntax and/or argument passing mode. Common examples that differ by syntax are mathematical arithmetic operations, e.g...
found in PCASTL have the same precedence
Order of operations
In mathematics and computer programming, the order of operations is a rule used to clarify unambiguously which procedures should be performed first in a given mathematical expression....
and associativity
Operator associativity
In programming languages and mathematical notation, the associativity of an operator is a property that determines how operators of the same precedence are grouped in the absence of parentheses...
as their counterparts in C
C (programming language)
C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system....
. for loops are defined like in C
C (programming language)
C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system....
.
++
and --
operatorsOperator (programming)
Programming languages typically support a set of operators: operations which differ from the language's functions in calling syntax and/or argument passing mode. Common examples that differ by syntax are mathematical arithmetic operations, e.g...
are used like in C
C (programming language)
C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system....
to increment
Increment
An increment is an increase of some amount, either fixed or variable. For example one's salary may have a fixed annual increment or one based on a percentage of its current value...
or decrement
Increment
An increment is an increase of some amount, either fixed or variable. For example one's salary may have a fixed annual increment or one based on a percentage of its current value...
a variable before or after it is used in its expression.
An example of PCASTL using the for reserved word
Reserved word
Reserved words are one type of grammatical construct in programming languages. These words have special meaning within the language and are predefined in the language’s formal specifications...
and the
++
operatorOperator (programming)
Programming languages typically support a set of operators: operations which differ from the language's functions in calling syntax and/or argument passing mode. Common examples that differ by syntax are mathematical arithmetic operations, e.g...
:
for (i = 1; i < 4; i++) print(i)
Functions
Subroutine
In computer science, a subroutine is a portion of code within a larger program that performs a specific task and is relatively independent of the remaining code....
and comments in PCASTL are defined like in R
R (programming language)
R is a programming language and software environment for statistical computing and graphics. The R language is widely used among statisticians for developing statistical software, and R is widely used for statistical software development and data analysis....
:
- function definition (comment)
a = function
{
print("Hello, world!")
}
- function call
a
parent and childset reserved words
Those reserved wordReserved word
Reserved words are one type of grammatical construct in programming languages. These words have special meaning within the language and are predefined in the language’s formal specifications...
s can only be written lowercase and will not be recognized otherwise. The parent reserved word gives a reference
Reference (computer science)
In computer science, a reference is a value that enables a program to indirectly access a particular data item, such as a variable or a record, in the computer's memory or in some other storage device. The reference is said to refer to the data item, and accessing those data is called...
to the parent node in the syntax tree
Abstract syntax tree
In computer science, an abstract syntax tree , or just syntax tree, is a tree representation of the abstract syntactic structure of source code written in a programming language. Each node of the tree denotes a construct occurring in the source code. The syntax is 'abstract' in the sense that it...
of the code where the word is placed. In the following code, the parent node is the operator
Operator (programming)
Programming languages typically support a set of operators: operations which differ from the language's functions in calling syntax and/or argument passing mode. Common examples that differ by syntax are mathematical arithmetic operations, e.g...
=
.
a = parent
The variable
Variable (programming)
In computer programming, a variable is a symbolic name given to some known or unknown quantity or information, for the purpose of allowing the name to be used independently of the information it represents...
"a" will hold a reference
Reference (computer science)
In computer science, a reference is a value that enables a program to indirectly access a particular data item, such as a variable or a record, in the computer's memory or in some other storage device. The reference is said to refer to the data item, and accessing those data is called...
to the
=
node. The following code shows how to get referencesReference (computer science)
In computer science, a reference is a value that enables a program to indirectly access a particular data item, such as a variable or a record, in the computer's memory or in some other storage device. The reference is said to refer to the data item, and accessing those data is called...
to the two child nodes of the operator
Operator (programming)
Programming languages typically support a set of operators: operations which differ from the language's functions in calling syntax and/or argument passing mode. Common examples that differ by syntax are mathematical arithmetic operations, e.g...
=
with the childset reserved wordReserved word
Reserved words are one type of grammatical construct in programming languages. These words have special meaning within the language and are predefined in the language’s formal specifications...
.
a.childset[0]
a.childset[1]
To display the value of "a", some ways are given in this example:
a
a.childset[0].parent
a.childset[1].parent
a.childset[0].parent.childset[0].parent # and so on...
In the following code: we assign a code segment to the right child of the
=
node, we execute the =
node a second time and we call the newly defined function.
a.childset[1] = `function print("hello")'
execute(a)
a