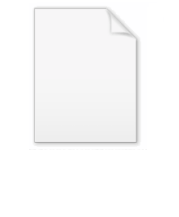
One-pass compiler
Encyclopedia
In computer programming
, a one-pass compiler is a compiler
that passes through the source code
of each compilation unit only once. In other words, a one-pass compiler does not "look back" at code it previously processed. Another term sometimes used is narrow compiler, which emphasizes the limited scope a one-pass compiler is obliged to use. This is in contrast to a multi-pass compiler
which traverses the source code and/or the abstract syntax tree
several times, building one or more intermediate representation
s that can be arbitrarily refined.
While one-pass compilers may be faster than multi-pass compilers, they are unable to generate as efficient programs, due to the limited scope available. (Many optimization
s require multiple passes over a program, subroutine, or basic block
.) In addition, some programming language
s simply cannot be compiled in a single pass, as a result of their design.
In contrast, some programming languages have been designed specifically to be compiled with one-pass compilers, and include special constructs to allow one-pass compilation. An example of such a construct is the forward declaration in Pascal
. Normally Pascal requires that procedures
be fully defined before use. This helps a one-pass compiler with its type checking: calling a procedure that hasn't been defined is a clear error. However, this requirement makes mutually recursive
procedures impossible to implement:
By adding a forward declaration for the function
Computer programming
Computer programming is the process of designing, writing, testing, debugging, and maintaining the source code of computer programs. This source code is written in one or more programming languages. The purpose of programming is to create a program that performs specific operations or exhibits a...
, a one-pass compiler is a compiler
Compiler
A compiler is a computer program that transforms source code written in a programming language into another computer language...
that passes through the source code
Source code
In computer science, source code is text written using the format and syntax of the programming language that it is being written in. Such a language is specially designed to facilitate the work of computer programmers, who specify the actions to be performed by a computer mostly by writing source...
of each compilation unit only once. In other words, a one-pass compiler does not "look back" at code it previously processed. Another term sometimes used is narrow compiler, which emphasizes the limited scope a one-pass compiler is obliged to use. This is in contrast to a multi-pass compiler
Multi-pass compiler
A multi-pass compiler is a type of compiler that processes the source code or abstract syntax tree of a program several times. This is in contrast to a one-pass compiler, which traverses the program only once. Each pass takes the result of the previous pass as the input, and creates an intermediate...
which traverses the source code and/or the abstract syntax tree
Abstract syntax tree
In computer science, an abstract syntax tree , or just syntax tree, is a tree representation of the abstract syntactic structure of source code written in a programming language. Each node of the tree denotes a construct occurring in the source code. The syntax is 'abstract' in the sense that it...
several times, building one or more intermediate representation
Intermediate language
In computer science, an intermediate language is the language of an abstract machine designed to aid in the analysis of computer programs. The term comes from their use in compilers, where a compiler first translates the source code of a program into a form more suitable for code-improving...
s that can be arbitrarily refined.
While one-pass compilers may be faster than multi-pass compilers, they are unable to generate as efficient programs, due to the limited scope available. (Many optimization
Compiler optimization
Compiler optimization is the process of tuning the output of a compiler to minimize or maximize some attributes of an executable computer program. The most common requirement is to minimize the time taken to execute a program; a less common one is to minimize the amount of memory occupied...
s require multiple passes over a program, subroutine, or basic block
Basic block
In computing, a basic block is a portion of the code within a program with certain desirable properties that make it highly amenable to analysis. Compilers usually decompose programs into their basic blocks as a first step in the analysis process...
.) In addition, some programming language
Programming language
A programming language is an artificial language designed to communicate instructions to a machine, particularly a computer. Programming languages can be used to create programs that control the behavior of a machine and/or to express algorithms precisely....
s simply cannot be compiled in a single pass, as a result of their design.
In contrast, some programming languages have been designed specifically to be compiled with one-pass compilers, and include special constructs to allow one-pass compilation. An example of such a construct is the forward declaration in Pascal
Pascal (programming language)
Pascal is an influential imperative and procedural programming language, designed in 1968/9 and published in 1970 by Niklaus Wirth as a small and efficient language intended to encourage good programming practices using structured programming and data structuring.A derivative known as Object Pascal...
. Normally Pascal requires that procedures
Subroutine
In computer science, a subroutine is a portion of code within a larger program that performs a specific task and is relatively independent of the remaining code....
be fully defined before use. This helps a one-pass compiler with its type checking: calling a procedure that hasn't been defined is a clear error. However, this requirement makes mutually recursive
Mutual recursion
Mutual recursion is a form of recursion where two mathematical or computational functions are defined in terms of each other.For instance, consider two functions even? and odd? defined as follows: function even?...
procedures impossible to implement:
function odd(n : integer) : boolean;
begin
if n = 0 then
odd := false
else if n < 0 then
odd := even(n + 1) { Compiler error: 'even' is not defined }
else
odd := even(n - 1)
end;
function even(n : integer) : boolean;
begin
if n = 0 then
even := true
else if n < 0 then
even := odd(n + 1)
else
even := odd(n - 1)
end;
By adding a forward declaration for the function
even
before the function odd
, the one-pass compiler is told that there will be a definition of even
later on in the program.
function even(n : integer) : boolean; forward;
function odd(n : integer) : boolean;
{ Et cetera }