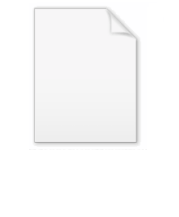
Little man computer
Encyclopedia
The Little Man Computer (LMC) is an instructional model of a computer
, created by Dr. Stuart Madnick
in 1965. The LMC is generally used to teach students, because it models a simple von Neumann architecture
computer - which has all of the basic features of a modern computer. It can be programmed in machine (albeit usually in decimal) or assembly code.
and a resettable counter known as the Program Counter. The Program Counter is similar to what a doorperson uses to keep track of how many people have entered a facility -- it can count up 1, or it can be reset to 0. As specified by the von Neumann architecture
, memory contains both instructions and data. The user loads data into the mailboxes and then signals the little man to begin execution.
processors, the simplicity of decimal
numbers was chosen to minimize the complexity for students who may not be comfortable working in binary/hexadecimal
.
INP
STA FIRST
INP
STA SECOND
LDA FIRST
SUB SECOND
OUT
HLT
FIRST DAT
SECOND DAT
To work around this difficulty, most assembly languages (including the LMC) allow the use of label
s. A label is simply a word provided as a name to the left of a particular line in the program text, which the assembler will convert to the appropriate address at the time of assembly. When seen to the right of the instruction, labels then take on the value of the address calculated.
Labels are commonly used to:
INP
LOOP SUB ONE // This address will be called LOOP, Subtract the value stored at ONE
OUT
BRZ QUIT // If we are at 0, then jump to the location QUIT
BRA LOOP // We are not at 0, go back to the start of LOOP
QUIT HLT
ONE DAT 1 // Put the value 1 in this mailbox, and call it ONE (variable declaration)
Computer
A computer is a programmable machine designed to sequentially and automatically carry out a sequence of arithmetic or logical operations. The particular sequence of operations can be changed readily, allowing the computer to solve more than one kind of problem...
, created by Dr. Stuart Madnick
Stuart Madnick
Stuart Madnick is a professor of information technology at the MIT Sloan School of Management and the Massachusetts Institute of Technology school of engineering.-Education:Madnick has degrees in Electrical Engineering Stuart Madnick is a professor of information technology at the MIT Sloan School...
in 1965. The LMC is generally used to teach students, because it models a simple von Neumann architecture
Von Neumann architecture
The term Von Neumann architecture, aka the Von Neumann model, derives from a computer architecture proposal by the mathematician and early computer scientist John von Neumann and others, dated June 30, 1945, entitled First Draft of a Report on the EDVAC...
computer - which has all of the basic features of a modern computer. It can be programmed in machine (albeit usually in decimal) or assembly code.
System architecture
The LMC model is based on the concept of a little man locked in a small room. At one end of the room, there are 100 mailboxes (memory), numbered 0 to 99, that can each contain a 3 digit instruction. Furthermore, there are two mailboxes at the other end labeled INBOX and OUTBOX which are used for receiving and outputting data. In the center of the room, there is a work area containing a simple two function (addition and subtraction) calculator known as the AccumulatorAccumulator (computing)
In a computer's central processing unit , an accumulator is a register in which intermediate arithmetic and logic results are stored. Without a register like an accumulator, it would be necessary to write the result of each calculation to main memory, perhaps only to be read right back again for...
and a resettable counter known as the Program Counter. The Program Counter is similar to what a doorperson uses to keep track of how many people have entered a facility -- it can count up 1, or it can be reset to 0. As specified by the von Neumann architecture
Von Neumann architecture
The term Von Neumann architecture, aka the Von Neumann model, derives from a computer architecture proposal by the mathematician and early computer scientist John von Neumann and others, dated June 30, 1945, entitled First Draft of a Report on the EDVAC...
, memory contains both instructions and data. The user loads data into the mailboxes and then signals the little man to begin execution.
Execution cycle
To execute a program, the little man performs these steps:- check the Program Counter for the mailbox number that contains a program instruction
- fetch the instruction from the mailbox with that number
- Increment the Program Counter (so that it contains the mailbox number of the next instruction)
- decode the instruction (includes finding the mailbox number for the data it will work on)
- fetch the data from the mailbox with the number found in the previous step
- execute the instruction
- store the new data in the mailbox from which the old data was retrieved
- Repeat the cycle or halt
Commands
While the LMC does reflect the actual workings of binaryBinary numeral system
The binary numeral system, or base-2 number system, represents numeric values using two symbols, 0 and 1. More specifically, the usual base-2 system is a positional notation with a radix of 2...
processors, the simplicity of decimal
Decimal
The decimal numeral system has ten as its base. It is the numerical base most widely used by modern civilizations....
numbers was chosen to minimize the complexity for students who may not be comfortable working in binary/hexadecimal
Hexadecimal
In mathematics and computer science, hexadecimal is a positional numeral system with a radix, or base, of 16. It uses sixteen distinct symbols, most often the symbols 0–9 to represent values zero to nine, and A, B, C, D, E, F to represent values ten to fifteen...
.
Instructions
Each LMC instruction is a 3 digit decimal number. The first digit represents the command to be performed and the final two digits represent the address of the mailbox affected by the command.Numeric code | Mnemonic code | Instruction | Description |
---|---|---|---|
1xx | ADD | ADD | Add the value stored in mailbox xx to whatever value is currently on the accumulator (calculator).
|
2xx | SUB | SUBTRACT | Subtract the value stored in mailbox xx from whatever value is currently on the accumulator (calculator).
|
3xx | STA | STORE | Store the contents of the accumulator in mailbox xx (destructive).
|
5xx | LDA | LOAD | Load the value from mailbox xx (non-destructive) and enter it in the accumulator (destructive). |
6xx | BRA | BRANCH Branch (computer science) A branch is sequence of code in a computer program which is conditionally executed depending on whether the flow of control is altered or not . The term can be used when referring to programs in high level languages as well as program written in machine code or assembly language... (unconditional) |
Set the program counter to the given address (value xx). That is, value xx will be the next instruction executed. |
7xx | BRZ | BRANCH IF ZERO (conditional) | If the accumulator (calculator) contains the value 000, set the program counter to the value xx. Otherwise, do nothing.
|
8xx | BRP | BRANCH IF POSITIVE (conditional) | If the accumulator (calculator) is 0 or positive, set the program counter to the value xx. Otherwise, do nothing.
|
901 | INP | INPUT | Go to the INBOX, fetch the value from the user, and put it in the accumulator (calculator)
|
902 | OUT | OUTPUT | Copy the value from the accumulator (calculator) to the OUTBOX.
|
000 | HLT HLT In the x86 computer architecture, HLT is an assembly language instruction which halts the CPU until the next external interrupt is fired. Such interrupts are used by devices in order to signal to the CPU that an event occurred which the CPU shall react on... |
HALT | Stop working. |
DAT | DATA | This is an assembler Assembly language An assembly language is a low-level programming language for computers, microprocessors, microcontrollers, and other programmable devices. It implements a symbolic representation of the machine codes and other constants needed to program a given CPU architecture... instruction which simply loads the value into the next available mailbox. DAT can also be used in conjunction with labels to declare variables. For example, DAT 984 will store the value 984 into a mailbox. |
Numeric
This program takes two numbers as input and outputs the difference. Notice that execution starts at Mailbox 00 and finishes at Mailbox 07.Mailbox | Numeric code |
Instruction | Comments |
---|---|---|---|
00 | 901 | INBOX --> ACCUMULATOR | INPUT the first number, enter into calculator (erasing whatever was there) |
01 | 308 | ACCUMULATOR --> MEMORY[08] | STORE the calculator's current value (to prepare for the next step...) |
02 | 901 | INBOX --> ACCUMULATOR | INPUT the second number, enter into calculator (erasing whatever was there) |
03 | 309 | ACCUMULATOR --> MEMORY[09] | STORE the calculator's current value (again, to prepare for the next step...) |
04 | 508 | MEMORY[08] --> ACCUMULATOR | (Now that both INPUT values are STORED in Mailboxes 08 and 09...) LOAD the first value back into the calculator (erasing whatever was there) |
05 | 209 | ACCUMULATOR = ACCUMULATOR - MEMORY[09] | SUBTRACT the second number from the calculator's current value (which was just set to the first number) |
06 | 902 | ACCUMULATOR --> OUTBOX | OUTPUT the calculator's result to the user |
07 | 000 | HALT | HALT (We're Done!) |
Mnemonic
Using the LMC mnemonic codes, an assembly language version of the program to subtract two numbers is given below.- This example program can be compiled and run on the LMC simulator available on the website of York UniversityYork UniversityYork University is a public research university in Toronto, Ontario, Canada. It is Canada's third-largest university, Ontario's second-largest graduate school, and Canada's leading interdisciplinary university....
(TorontoTorontoToronto is the provincial capital of Ontario and the largest city in Canada. It is located in Southern Ontario on the northwestern shore of Lake Ontario. A relatively modern city, Toronto's history dates back to the late-18th century, when its land was first purchased by the British monarchy from...
, CanadaCanadaCanada is a North American country consisting of ten provinces and three territories. Located in the northern part of the continent, it extends from the Atlantic Ocean in the east to the Pacific Ocean in the west, and northward into the Arctic Ocean...
) or on the desktop application written by Matthew Consterdine. Both simulators include full instructions and sample programs, compilers to convert the assembly into machine code, control interfaces to execute and monitor programs, and a step-by-step detailed description of each LMC instruction.
INP
STA FIRST
INP
STA SECOND
LDA FIRST
SUB SECOND
OUT
HLT
HLT
In the x86 computer architecture, HLT is an assembly language instruction which halts the CPU until the next external interrupt is fired. Such interrupts are used by devices in order to signal to the CPU that an event occurred which the CPU shall react on...
FIRST DAT
SECOND DAT
Labels
The convenience of assembled mnemonics is made apparent from this example — The programmer is no longer required to memorize a set of anonymous numeric codes, and can now program with a set of more memorable instructions. However, the programmer is still required to manually keep track of mailbox locations. Furthermore, if an instruction was to be inserted somewhere in the program, the final HLT instruction would move down to address 08. Suppose the user entered 600 as the first input. This value would overwrite the 000 (HLT) instruction. Since 600 means "Branch to mailbox 0" the program, instead of halting, would get stuck in an endless loop.To work around this difficulty, most assembly languages (including the LMC) allow the use of label
Label (programming language)
A label in a programming language is a sequence of characters that identifies a location within source code. In most languages labels take the form of an identifier, often followed by a punctuation character . In many high level programming languages the purpose of a label is to act as the...
s. A label is simply a word provided as a name to the left of a particular line in the program text, which the assembler will convert to the appropriate address at the time of assembly. When seen to the right of the instruction, labels then take on the value of the address calculated.
Labels are commonly used to:
- identify a particular instruction as a target for a BRANCH instruction
- identify space as a named variable (using DAT)
- load data into the program at assembly time for use by the program.
- This use is not obvious until one considers that there is no way of adding 1 to a counter. One could ask the user to input 1 at the beginning, but it would be better to have this loaded at the time of assembly
Example
This program will take a user input, and count down to zero.INP
LOOP SUB ONE // This address will be called LOOP, Subtract the value stored at ONE
OUT
BRZ QUIT // If we are at 0, then jump to the location QUIT
BRA LOOP // We are not at 0, go back to the start of LOOP
QUIT HLT
ONE DAT 1 // Put the value 1 in this mailbox, and call it ONE (variable declaration)
See also
- CARDboard Illustrative Aid to ComputationCARDboard Illustrative Aid to ComputationCardiac was a learning aid developed by David Hagelbarger and Saul Fingerman for Bell Telephone Laboratories in 1968 to teach high school students how computers work. The kit consisted of an instruction manual and a die-cut cardboard "computer".The computer "operated" by means of pencil and...
(CARDIAC) A similar instruction set - with simulator - from Bell Laboratories. - VIC, an extension of LMC from the Interdisciplinary Center Herzliya.
External links
- Richard J. Povinelli:Teaching:Introduction to Computer Hardware and Software:Little Man Computer
- The "Little Man" Computer