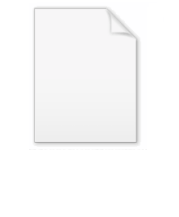
Java Classloader
Encyclopedia
The Java Classloader is a part of the Java Runtime Environment that dynamically load
s Java classes into the Java Virtual Machine
. Usually classes are only loaded on demand
. The Java run time system does not need to know about files and file systems because of class loaders. Delegation
is an important concept to understand when learning about class loaders.
A software library is a collection of related object code
.
In the Java language, libraries are typically packaged in Jar files. Libraries can contain objects of different types. The most important type of object contained in a Jar file is a Java class. A class can be thought of as a named unit of code. The class loader is responsible for locating libraries, reading their contents, and loading the classes contained within the libraries. This loading is typically done "on demand", in that it does not occur until the class is actually used by the program. A class with a given name can only be loaded once by a given classloader.
Each Java class must be loaded by a class loader. Furthermore, Java programs may make use of external libraries (that is, libraries written and provided by someone other than the author of the program) or they may be composed, at least in part, of a number of libraries.
When the JVM is started, three class loaders are used:
The bootstrap class loader loads the core Java libraries (
The extensions class loader loads the code in the extensions directories (
by the
The system class loader loads code found on
This makes it possible (for example):
(JEE) application servers typically load classes from a deployed WAR or EAR
archive by a tree of classloaders, isolating the application from other applications, but sharing classes between deployed modules. So-called "servlet containers" are typically implemented in terms of multiple classloaders.
used to describe all the various ways in which the classloading process can end up not working. Three ways JAR hell can occur are:
The OSGi
Alliance specified (starting as JSR 8 in 1998) a modularity framework that solved JAR hell for current and future VMs in ME, SE, and EE that is widely adopted. Using metadata in the JAR manifest
, JAR files (called bundles) are wired on a per-package basis. Bundles can export packages, import packages and keep packages private, providing the basic constructs of modularity and versioned dependency management.
To remedy the JAR hell problems a Java Community Process
— JSR 277 was initiated in 2005. The resolution — Java Module System
— intended to introduce a new distribution format, modules versioning scheme and a common modules repository (similar in purpose to Microsoft .NET's Global Assembly Cache
). In December 2008 Sun announced that JSR 277 was put on hold.
Loader (computing)
In computing, a loader is the part of an operating system that is responsible for loading programs. It is one of the essential stages in the process of starting a program, as it places programs into memory and prepares them for execution...
s Java classes into the Java Virtual Machine
Java Virtual Machine
A Java virtual machine is a virtual machine capable of executing Java bytecode. It is the code execution component of the Java software platform. Sun Microsystems stated that there are over 4.5 billion JVM-enabled devices.-Overview:...
. Usually classes are only loaded on demand
Lazy initialization
In computer programming, lazy initialization is the tactic of delaying the creation of an object, the calculation of a value, or some other expensive process until the first time it is needed....
. The Java run time system does not need to know about files and file systems because of class loaders. Delegation
Delegation (programming)
In object-oriented programming, there are two related notions of delegation.* Most commonly, it refers to a programming language feature making use of the method lookup rules for dispatching so-called self-calls as defined by Lieberman in his 1986 paper "Using Prototypical Objects to Implement...
is an important concept to understand when learning about class loaders.
A software library is a collection of related object code
Object code
Object code, or sometimes object module, is what a computer compiler produces. In a general sense object code is a sequence of statements in a computer language, usually a machine code language....
.
In the Java language, libraries are typically packaged in Jar files. Libraries can contain objects of different types. The most important type of object contained in a Jar file is a Java class. A class can be thought of as a named unit of code. The class loader is responsible for locating libraries, reading their contents, and loading the classes contained within the libraries. This loading is typically done "on demand", in that it does not occur until the class is actually used by the program. A class with a given name can only be loaded once by a given classloader.
Each Java class must be loaded by a class loader. Furthermore, Java programs may make use of external libraries (that is, libraries written and provided by someone other than the author of the program) or they may be composed, at least in part, of a number of libraries.
When the JVM is started, three class loaders are used:
- Bootstrap class loader
- Extensions class loader
- System class loader
The bootstrap class loader loads the core Java libraries (
/lib
directory). This class loader, which is part of the core JVM, is written in native code.The extensions class loader loads the code in the extensions directories (
/lib/ext
or any other directory specifiedby the
java.ext.dirs
system property). It is implemented by the sun.misc.Launcher$ExtClassLoader
class.The system class loader loads code found on
java.class.path
, which maps to the system CLASSPATHClasspath (Java)Classpath is a parameter—set either on the command-line, or through an environment variable—that tells the Java Virtual Machine or the compiler where to look for user-defined classes and packages.-Overview and architecture:...
variable. This is implemented by the sun.misc.Launcher$AppClassLoader
class.User-defined class loaders
By default, all user classes are loaded by the default system class loader, but it is possible to replace it by a user and even to chain class loaders as desired.This makes it possible (for example):
- to load or unload classes at runtime (for example to load libraries dynamically at runtime, even from a HTTPHypertext Transfer ProtocolThe Hypertext Transfer Protocol is a networking protocol for distributed, collaborative, hypermedia information systems. HTTP is the foundation of data communication for the World Wide Web....
resource). This is an important feature for:- implementing scripting languages,
- using bean builders,
- allowing user-defined extensibilityExtensibilityIn software engineering, extensibility is a system design principle where the implementation takes into consideration future growth. It is a systemic measure of the ability to extend a system and the level of effort required to implement the extension...
- allowing multiple namespaceNamespace (computer science)A namespace is an abstract container or environment created to hold a logical grouping of unique identifiers or symbols . An identifier defined in a namespace is associated only with that namespace. The same identifier can be independently defined in multiple namespaces...
s to communicate. This is one of the foundations of CORBACommon Object Request Broker ArchitectureThe Common Object Request Broker Architecture is a standard defined by the Object Management Group that enables software components written in multiple computer languages and running on multiple computers to work together .- Overview:CORBA enables separate pieces of software written in different...
/ RMIJava remote method invocationThe Java Remote Method Invocation Application Programming Interface , or Java RMI, is a Java application programming interface that performs the object-oriented equivalent of remote procedure calls ....
protocols, for example.
- to change the way the bytecodeJava bytecodeJava bytecode is the form of instructions that the Java virtual machine executes. Each bytecode opcode is one byte in length, although some require parameters, resulting in some multi-byte instructions. Not all of the possible 256 opcodes are used. 51 are reserved for future use...
is loaded (for example, it is possible to use encryptedEncryptionIn cryptography, encryption is the process of transforming information using an algorithm to make it unreadable to anyone except those possessing special knowledge, usually referred to as a key. The result of the process is encrypted information...
Java class bytecode). - to modify the loaded bytecode (for example, for load-time weavingAspect weaverAn aspect weaver is a metaprogramming utility for aspect-oriented languages designed to take instructions specified by aspects and generate the final implementation code. The weaver integrates aspects into the locations specified by the software as a pre-compilation step...
of aspects when using aspect-oriented programmingAspect-oriented programmingIn computing, aspect-oriented programming is a programming paradigm which aims to increase modularity by allowing the separation of cross-cutting concerns...
).
Class Loaders in JEE
Java Platform, Enterprise EditionJava Platform, Enterprise Edition
Java Platform, Enterprise Edition or Java EE is widely used platform for server programming in the Java programming language. The Java platform differs from the Java Standard Edition Platform in that it adds libraries which provide functionality to deploy fault-tolerant, distributed, multi-tier...
(JEE) application servers typically load classes from a deployed WAR or EAR
EAR (file format)
EAR is a file format used by Java EE for packaging one or more modules into a single archive so that the deployment of the various modules onto an application server happens simultaneously and coherently...
archive by a tree of classloaders, isolating the application from other applications, but sharing classes between deployed modules. So-called "servlet containers" are typically implemented in terms of multiple classloaders.
JAR hell
JAR hell is a term similar to DLL hellDLL hell
In computing, DLL Hell is a term for the complications that arise when working with dynamic link libraries used with Microsoft Windows operating systems, particularly legacy 16-bit editions which all run in a single memory space....
used to describe all the various ways in which the classloading process can end up not working. Three ways JAR hell can occur are:
- The first case is when a developer or deployer of a Java application has accidentally made two different versions of a library available to the system. This will not be considered an error by the system. Rather, the system will load classes from one or the other library. Adding the new library to the list of available libraries instead of replacing it, may see the application still behaving as though the old library is in use, which it may well be.
- Another version of the problem arises when two libraries (or a library and the application) require different versions of the same third library. If both versions of the third library use the same class names, there is no way to load both versions of the third library with the same classloader.
- The most complex JAR hell problems arise in circumstances that take advantage of the full complexity of the classloading system. A Java program is not required to use only a single "flat" classloader, but instead may be composed of several (potentially very many) nested, cooperating classloaders. Classes loaded by different classloaders may interact in complex ways not fully comprehended by a developer, leading to inexplicable errors or bugs.
The OSGi
OSGi
The Open Services Gateway initiative framework is a module system and service platform for the Java programming language that implements a complete and dynamic component model, something that does not exist in standalone Java/VM environments...
Alliance specified (starting as JSR 8 in 1998) a modularity framework that solved JAR hell for current and future VMs in ME, SE, and EE that is widely adopted. Using metadata in the JAR manifest
Manifest file
On the Java platform, a Manifest file is a specific file contained within a JAR archive. It is used to define extension and package-related data. It is a metadata file that contains name-value pairs organized in different sections. If a JAR file is intended to be used as an executable file, the...
, JAR files (called bundles) are wired on a per-package basis. Bundles can export packages, import packages and keep packages private, providing the basic constructs of modularity and versioned dependency management.
To remedy the JAR hell problems a Java Community Process
Java Community Process
The Java Community Process or JCP, established in 1998, is a formalized process that allows interested parties to get involved in the definition of future versions and features of the Java platform....
— JSR 277 was initiated in 2005. The resolution — Java Module System
Java Module System
The Java Module System specifies a distribution format for collections of Java code and associated resources. It also specifies a repository for storing these collections, or modules, and identifies how they can be discovered, loaded and checked for integrity...
— intended to introduce a new distribution format, modules versioning scheme and a common modules repository (similar in purpose to Microsoft .NET's Global Assembly Cache
Global Assembly Cache
The Global Assembly Cache or GAC is a machine-wide .NET assemblies cache for Microsoft's CLR platform. The approach of having a specially controlled central repository addresses the shared library concept and helps to avoid pitfalls of other solutions that led to drawbacks like DLL hell.-...
). In December 2008 Sun announced that JSR 277 was put on hold.
See also
- Loader (computing)Loader (computing)In computing, a loader is the part of an operating system that is responsible for loading programs. It is one of the essential stages in the process of starting a program, as it places programs into memory and prepares them for execution...
- Dynamic loadingDynamic loadingDynamic loading is a mechanism by which a computer program can, at run time, load a library into memory, retrieve the addresses of functions and variables contained in the library, execute those functions or access those variables, and unload the library from memory...
- DLL hellDLL hellIn computing, DLL Hell is a term for the complications that arise when working with dynamic link libraries used with Microsoft Windows operating systems, particularly legacy 16-bit editions which all run in a single memory space....
- OSGiOSGiThe Open Services Gateway initiative framework is a module system and service platform for the Java programming language that implements a complete and dynamic component model, something that does not exist in standalone Java/VM environments...
- Apache MavenApache MavenMaven is a build automation and software comprehension tool. While primarily used for Java programming, it can also be used to build and manage projects written in C#, Ruby, Scala, and other languages. Maven serves a similar purpose to the Apache Ant tool, but it is based on different concepts and...
, automated software build tool with dependency management
External links
- Chuck Mcmanis, "The basics of Java class loaders", 1996
- Brandon E. Taylor, "Java Class Loading: The Basics", 2003
- Jeff HansonJeff HansonJeff Hanson was a singer-songwriter, guitarist, and multi-instrumentalist whose voice was described in a 2005 Paste review as an "angelic falsetto, a cross between Alison Krauss and Art Garfunkel that is often mistaken for a female contralto."-Biography:Hanson was born in Milwaukee, Wisconsin...
, "Take Control of Class Loading in Java", 2006-06-01 - Andreas Schaefer, "Inside Class Loaders", 2003-11-12
- Sheng LiangSheng LiangSheng Liang was the lead developer on the original Java Virtual Machine team at Sun Microsystems, Inc.. He is currently the chief technology officer of the Cloud Platforms groups at Citrix Systems after their acquisition of Cloud.com, where he was co-founder and chief executive officer and founder...
and Gilad BrachaGilad BrachaGilad Bracha is a software engineer, a co-author of the second and third editions of the Java Language Specification, a major contributor to the second edition of the Java Virtual Machine Specification, and the creator of the Newspeak programming language....
, "Dynamic class loading in the Java virtual machine", In Proceedings of the 13th ACM Conference on Object-Oriented Programming, Systems, Languages, and Applications (OOPSLA'98), ACM SIGPLAN Notices, vol. 33, no. 10, ACM Press, 1998, pp. 36–44
- Dr. Christoph G. Jung, "Classloaders Revisited Hotdeploy", Java Specialist Newsletter, 2001-06-07
- Don Schwarz, "Managing Component Dependencies Using ClassLoaders", 2005-04-13