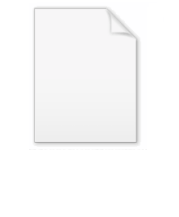
Classpath (Java)
Encyclopedia
Classpath is a parameter—set either on the command-line, or through an environment variable
—that tells the Java Virtual Machine
or the compiler where to look for user-defined classes
and packages
.
programs, the Java Virtual Machine
finds and loads classes lazily (it loads the bytecode
of a class only when this class is first used). The classpath tells Java where to look on the filesystem for files defining these classes.
The virtual machine searches for and loads classes in this order:
By default only the packages of the JDK standard API
and extension packages are accessible without needing to set where to find them. The path for all user-defined packages
and libraries must be set in the command-line (or in the Manifest
associated with the Jar file
containing the classes).
and the files defining this package are stored physically under the directory D:\myprogram (on Windows
) or /home/user/myprogram (on Linux
).
The file structure will look like this:
When we invoke Java, we specify the name of the application to run: org.mypackage.HelloWorld. However we must also tell Java where to look for the files and directories defining our package. So to launch the program, we use the following command:
where:
Note that if we ran Java in D:\myprogram\ (on Linux, /home/user/myprogram/) then we would not need to specify the classpath since Java implicitly looks in the current working directory
for files containing classes.
Windows example:
Linux example:
named CLASSPATH may be alternatively used to set the classpath. For the above example, we could also use on Windows:
called supportLib.jar, physically in the directory D:\myprogram\lib\.
The corresponding physical file structure is :
We should use the following command-line option:
java -classpath D:\myprogram;D:\myprogram\lib\supportLib.jar org.mypackage.HelloWorld
or alternatively:
set CLASSPATH=D:\myprogram;D:\myprogram\lib\supportLib.jar
java org.mypackage.HelloWorld
called helloWorld.jar, put directly in the D:\myprogram directory. We have the following file structure:
The manifest file
defined in this Jar file
has this definition:
Note: It's important that the manifest file
ends with either a new line or carriage return.
Also, note that the classpath string in this case describes the location of the supportLib.jar file relative to the location of the helloWorld.jar file, and not as an absolute file path (as it might be when setting the -classpath parameter on the command line, for example). Thus, the actual locations of the jar file and its support library are irrelevant so long as the relative directory structure between the two is preserved.
To launch the program, we can use the following command:
java -jar D:\myprogram\helloWorld.jar
It is not necessary to define the Main class at launch, the Classpath to the program classes, or the support library classes, because they are already defined in the manifest file
.
The syntax for specifying multiple library JAR files in the manifest file
is to separate the entries with a space:
This does not apply when the Classpath is defined in manifest file
s, where each file path must be separated by a space (" "), regardless of the operating system.
Environment variable
Environment variables are a set of dynamic named values that can affect the way running processes will behave on a computer.They can be said in some sense to create the operating environment in which a process runs...
—that tells the Java Virtual Machine
Java Virtual Machine
A Java virtual machine is a virtual machine capable of executing Java bytecode. It is the code execution component of the Java software platform. Sun Microsystems stated that there are over 4.5 billion JVM-enabled devices.-Overview:...
or the compiler where to look for user-defined classes
Class (computer science)
In object-oriented programming, a class is a construct that is used as a blueprint to create instances of itself – referred to as class instances, class objects, instance objects or simply objects. A class defines constituent members which enable these class instances to have state and behavior...
and packages
Java package
A Java package is a mechanism for organizing Java classes into namespaces similar to the modules of Modula. Java packages can be stored in compressed files called JAR files, allowing classes to download faster as a group rather than one at a time...
.
Overview and architecture
Similar to the classic dynamic loading behavior, when executing JavaJava (programming language)
Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities...
programs, the Java Virtual Machine
Java Virtual Machine
A Java virtual machine is a virtual machine capable of executing Java bytecode. It is the code execution component of the Java software platform. Sun Microsystems stated that there are over 4.5 billion JVM-enabled devices.-Overview:...
finds and loads classes lazily (it loads the bytecode
Java bytecode
Java bytecode is the form of instructions that the Java virtual machine executes. Each bytecode opcode is one byte in length, although some require parameters, resulting in some multi-byte instructions. Not all of the possible 256 opcodes are used. 51 are reserved for future use...
of a class only when this class is first used). The classpath tells Java where to look on the filesystem for files defining these classes.
The virtual machine searches for and loads classes in this order:
- bootstrap classes: the classes that are fundamental to the Java Platform (comprising the public classes of the Java Class LibraryJava Class LibraryThe Java Class Library is a set of dynamically loadable libraries that Java applications can call at run time. Because the Java Platform is not dependent on any specific operating system, applications cannot rely on any of the existing libraries...
, and the private classes that are necessary for this library to be functional). - extension classes: packagesJava packageA Java package is a mechanism for organizing Java classes into namespaces similar to the modules of Modula. Java packages can be stored in compressed files called JAR files, allowing classes to download faster as a group rather than one at a time...
that are in the extension directory of the JRE or JDK, jre/lib/ext/ - user-defined packages and libraries
By default only the packages of the JDK standard API
Java Platform, Standard Edition
Java Platform, Standard Edition or Java SE is a widely used platform for programming in the Java language. It is the Java Platform used to deploy portable applications for general use...
and extension packages are accessible without needing to set where to find them. The path for all user-defined packages
Java package
A Java package is a mechanism for organizing Java classes into namespaces similar to the modules of Modula. Java packages can be stored in compressed files called JAR files, allowing classes to download faster as a group rather than one at a time...
and libraries must be set in the command-line (or in the Manifest
Manifest file
On the Java platform, a Manifest file is a specific file contained within a JAR archive. It is used to define extension and package-related data. It is a metadata file that contains name-value pairs organized in different sections. If a JAR file is intended to be used as an executable file, the...
associated with the Jar file
JAR (file format)
In software, JAR is an archive file format typically used to aggregate many Java class files and associated metadata and resources into one file to distribute application software or libraries on the Java platform.JAR files are built on the ZIP file format and have the .jar file extension...
containing the classes).
Basic usage
Suppose we have a package called org.mypackage containing the classes:- HelloWorld (main class)
- SupportClass
- UtilClass
and the files defining this package are stored physically under the directory D:\myprogram (on Windows
Microsoft Windows
Microsoft Windows is a series of operating systems produced by Microsoft.Microsoft introduced an operating environment named Windows on November 20, 1985 as an add-on to MS-DOS in response to the growing interest in graphical user interfaces . Microsoft Windows came to dominate the world's personal...
) or /home/user/myprogram (on Linux
Linux
Linux is a Unix-like computer operating system assembled under the model of free and open source software development and distribution. The defining component of any Linux system is the Linux kernel, an operating system kernel first released October 5, 1991 by Linus Torvalds...
).
The file structure will look like this:
Microsoft Windows Microsoft Windows Microsoft Windows is a series of operating systems produced by Microsoft.Microsoft introduced an operating environment named Windows on November 20, 1985 as an add-on to MS-DOS in response to the growing interest in graphical user interfaces . Microsoft Windows came to dominate the world's personal... |
Linux Linux Linux is a Unix-like computer operating system assembled under the model of free and open source software development and distribution. The defining component of any Linux system is the Linux kernel, an operating system kernel first released October 5, 1991 by Linus Torvalds... |
---|---|
D:\myprogram\ > ---> org\ | ---> mypackage\ | ---> HelloWorld.class ---> SupportClass.class ---> UtilClass.class |
/home/user/myprogram/ > ---> org/ | ---> mypackage/ | ---> HelloWorld.class ---> SupportClass.class ---> UtilClass.class |
When we invoke Java, we specify the name of the application to run: org.mypackage.HelloWorld. However we must also tell Java where to look for the files and directories defining our package. So to launch the program, we use the following command:
Microsoft Windows Microsoft Windows Microsoft Windows is a series of operating systems produced by Microsoft.Microsoft introduced an operating environment named Windows on November 20, 1985 as an add-on to MS-DOS in response to the growing interest in graphical user interfaces . Microsoft Windows came to dominate the world's personal... |
Linux Linux Linux is a Unix-like computer operating system assembled under the model of free and open source software development and distribution. The defining component of any Linux system is the Linux kernel, an operating system kernel first released October 5, 1991 by Linus Torvalds... |
---|---|
|
|
where:
- -classpath D:\myprogram sets the path to the packages used in the program (on Linux, -classpath /home/user/myprogram)
- org.mypackage.HelloWorld is the name of the main class
Note that if we ran Java in D:\myprogram\ (on Linux, /home/user/myprogram/) then we would not need to specify the classpath since Java implicitly looks in the current working directory
Working directory
In computing, the working directory of a process is a directory of a hierarchical file system, if any, dynamically associated with each process. When the process refers to a file using a simple file name or relative path , the reference is interpreted relative to the current working directory of...
for files containing classes.
Adding all JAR files in a directory
In Java 6 and higher, one can add all jar-files in a specific directory to the classpath using wildcard notation.Windows example:
java -classpath ".;c:\mylib\*" MyApp
Linux example:
java -classpath '.:/mylib/*' MyApp
Setting the path through an environment variable
The environment variableEnvironment variable
Environment variables are a set of dynamic named values that can affect the way running processes will behave on a computer.They can be said in some sense to create the operating environment in which a process runs...
named CLASSPATH may be alternatively used to set the classpath. For the above example, we could also use on Windows:
set CLASSPATH=D:\myprogram
java org.mypackage.HelloWorld
Setting the path of a Jar file
Now, suppose the program uses a supporting library enclosed in a Jar fileJAR (file format)
In software, JAR is an archive file format typically used to aggregate many Java class files and associated metadata and resources into one file to distribute application software or libraries on the Java platform.JAR files are built on the ZIP file format and have the .jar file extension...
called supportLib.jar, physically in the directory D:\myprogram\lib\.
The corresponding physical file structure is :
D:\myprogram\
|
---> lib\
|
---> supportLib.jar
|
---> org\
|
--> mypackage\
|
---> HelloWorld.class
---> SupportClass.class
---> UtilClass.class
We should use the following command-line option:
java -classpath D:\myprogram;D:\myprogram\lib\supportLib.jar org.mypackage.HelloWorld
or alternatively:
set CLASSPATH=D:\myprogram;D:\myprogram\lib\supportLib.jar
java org.mypackage.HelloWorld
Setting the path in a Manifest file
Suppose that our program has been enclosed in a Jar fileJAR (file format)
In software, JAR is an archive file format typically used to aggregate many Java class files and associated metadata and resources into one file to distribute application software or libraries on the Java platform.JAR files are built on the ZIP file format and have the .jar file extension...
called helloWorld.jar, put directly in the D:\myprogram directory. We have the following file structure:
D:\myprogram\
|
---> helloWorld.jar
|
---> lib\
|
---> supportLib.jar
The manifest file
Manifest file
On the Java platform, a Manifest file is a specific file contained within a JAR archive. It is used to define extension and package-related data. It is a metadata file that contains name-value pairs organized in different sections. If a JAR file is intended to be used as an executable file, the...
defined in this Jar file
JAR (file format)
In software, JAR is an archive file format typically used to aggregate many Java class files and associated metadata and resources into one file to distribute application software or libraries on the Java platform.JAR files are built on the ZIP file format and have the .jar file extension...
has this definition:
Main-Class: org.mypackage.HelloWorld
Class-Path: lib/supportLib.jar
Note: It's important that the manifest file
Manifest file
On the Java platform, a Manifest file is a specific file contained within a JAR archive. It is used to define extension and package-related data. It is a metadata file that contains name-value pairs organized in different sections. If a JAR file is intended to be used as an executable file, the...
ends with either a new line or carriage return.
Also, note that the classpath string in this case describes the location of the supportLib.jar file relative to the location of the helloWorld.jar file, and not as an absolute file path (as it might be when setting the -classpath parameter on the command line, for example). Thus, the actual locations of the jar file and its support library are irrelevant so long as the relative directory structure between the two is preserved.
To launch the program, we can use the following command:
java -jar D:\myprogram\helloWorld.jar
It is not necessary to define the Main class at launch, the Classpath to the program classes, or the support library classes, because they are already defined in the manifest file
Manifest file
On the Java platform, a Manifest file is a specific file contained within a JAR archive. It is used to define extension and package-related data. It is a metadata file that contains name-value pairs organized in different sections. If a JAR file is intended to be used as an executable file, the...
.
The syntax for specifying multiple library JAR files in the manifest file
Manifest file
On the Java platform, a Manifest file is a specific file contained within a JAR archive. It is used to define extension and package-related data. It is a metadata file that contains name-value pairs organized in different sections. If a JAR file is intended to be used as an executable file, the...
is to separate the entries with a space:
Class-Path: lib/supportLib.jar lib/supportLib2.jar
OS specific notes
Being closely associated with the file system, the command-line Classpath syntax depends on the operating system. For example:- on all Unix-likeUnixUnix is a multitasking, multi-user computer operating system originally developed in 1969 by a group of AT&T employees at Bell Labs, including Ken Thompson, Dennis Ritchie, Brian Kernighan, Douglas McIlroy, and Joe Ossanna...
operating systems (such as LinuxLinuxLinux is a Unix-like computer operating system assembled under the model of free and open source software development and distribution. The defining component of any Linux system is the Linux kernel, an operating system kernel first released October 5, 1991 by Linus Torvalds...
and Mac OS XMac OS XMac OS X is a series of Unix-based operating systems and graphical user interfaces developed, marketed, and sold by Apple Inc. Since 2002, has been included with all new Macintosh computer systems...
), the directory structure has a Unix syntax, with separate file paths separated by a colonColon (punctuation)The colon is a punctuation mark consisting of two equally sized dots centered on the same vertical line.-Usage:A colon informs the reader that what follows the mark proves, explains, or lists elements of what preceded the mark....
(":"). - on WindowsMicrosoft WindowsMicrosoft Windows is a series of operating systems produced by Microsoft.Microsoft introduced an operating environment named Windows on November 20, 1985 as an add-on to MS-DOS in response to the growing interest in graphical user interfaces . Microsoft Windows came to dominate the world's personal...
, the directory structure has a Windows syntax, and each file path must be separated by a semicolonSemicolonThe semicolon is a punctuation mark with several uses. The Italian printer Aldus Manutius the Elder established the practice of using the semicolon to separate words of opposed meaning and to indicate interdependent statements. "The first printed semicolon was the work of ... Aldus Manutius"...
(";").
This does not apply when the Classpath is defined in manifest file
Manifest file
On the Java platform, a Manifest file is a specific file contained within a JAR archive. It is used to define extension and package-related data. It is a metadata file that contains name-value pairs organized in different sections. If a JAR file is intended to be used as an executable file, the...
s, where each file path must be separated by a space (" "), regardless of the operating system.
Diagnose
Application programmers may want to find out/debug the current settings under which the application is running:System.getProperty("java.class.path")JavaDoc
See also
- Java ClassloaderJava ClassloaderThe Java Classloader is a part of the Java Runtime Environment that dynamically loads Java classes into the Java Virtual Machine. Usually classes are only loaded on demand. The Java run time system does not need to know about files and file systems because of class loaders...
- Java packageJava packageA Java package is a mechanism for organizing Java classes into namespaces similar to the modules of Modula. Java packages can be stored in compressed files called JAR files, allowing classes to download faster as a group rather than one at a time...
- Jar file formatJAR (file format)In software, JAR is an archive file format typically used to aggregate many Java class files and associated metadata and resources into one file to distribute application software or libraries on the Java platform.JAR files are built on the ZIP file format and have the .jar file extension...
- Java programming languageJava (programming language)Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities...
- Manifest filesManifest fileOn the Java platform, a Manifest file is a specific file contained within a JAR archive. It is used to define extension and package-related data. It is a metadata file that contains name-value pairs organized in different sections. If a JAR file is intended to be used as an executable file, the...