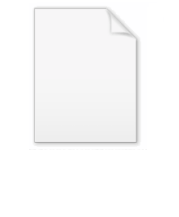
Hollywood Principle
Encyclopedia
In computer programming
, the Hollywood principle is stated as "don't call us, we'll call you." It has applications in software engineering
; see also implicit invocation
for a related architectural principle.
and low coupling
that is easier to debug, maintain and test.
Most beginners are first introduced to programming from a diametrically opposed viewpoint. Programs such as Hello World
take control of the running environment and make calls on the underlying system to do their work. A considerable amount of successful software has been developed using the same principles, and indeed many developers need never think there is any other approach. After all, programs with linear flow are generally easy to understand.
As systems increase in complexity, the linear model becomes less maintainable. Consider for example a simple program to bounce a square around a window in your favorite operating system or window manager. The linear approach may work, up to a point. You can keep the moving and drawing code in separate procedures, but soon the logic begins to branch.
It would be much more elegant if the programmer could concentrate on the application (in this case, updating the coordinates of the box) and leave the parts common to every application to "something else".
The key to making this possible is to sacrifice the element of control. Instead of your program running the system, the system runs your program. In our example, our program could register for timer events, and write a corresponding event handler that updates the coordinates. The program would include other callbacks
to respond to other events
, such as when the system requires part of a window to be redrawn. The system should provide suitable context information so the handler can perform the task and return. The user's program no longer includes an explicit control path
, aside from initialization and registration.
Event loop
programming, however, is merely the beginning of software development following the Hollywood principle. More advanced schemes such as event-driven object-orientation go further along the path, by software components sending messages
to each other and reacting to the messages they receive. Each message handler merely has to perform its own local processing. It becomes very easy to unit test individual components of the system in isolation, while integration of all the components typically does not have to concern itself excessively with the dependencies between them.
Software architecture
that encourages the Hollywood principle typically becomes more than "just" an API
– instead, it may take on more dominant roles such as a software framework
or container
. Examples:
All of these mechanisms require some cooperation from the developer. To integrate seamlessly with the framework, the developer must produce code that follows some conventions and requirements of the framework. This may be something as simple as implementing a specific interface, or, as in the case of EJB, a significant amount of wrapper code, often produced by code generation tools.
s go even further in pursuit of the Hollywood principle. Inversion of control
for instance takes even the integration and configuration of the system out of the application, and instead performs dependency injection
.
Again, this is most easily illustrated by an example. A more complex program such as a financial application is likely to depend on several external resources, such as database connections. Traditionally, the code to connect to the database ends up as a procedure somewhere in the program. It becomes difficult to change the database or test the code without one. The same is true for every other external resource that the application uses.
Various design patterns exist to try to reduce the coupling in such applications. In the Java world, the Service locator pattern
exists to look up resources in a directory, such as JNDI. This reduces the dependency – now, instead of every separate resource having its own initialization code, the program depends only on the service locator.
external from the code. The container is responsible for resolution of these dependencies, and delivers them to the other software components – for example by calling a setter method
. The code itself does not contain any configuration. Changing the database, or replacing it with a suitable mock object
for unit testing, becomes a relatively simple matter of changing the external configuration. Integration of software components is facilitated, and the individual components get even closer to the Hollywood principle.
Computer programming
Computer programming is the process of designing, writing, testing, debugging, and maintaining the source code of computer programs. This source code is written in one or more programming languages. The purpose of programming is to create a program that performs specific operations or exhibits a...
, the Hollywood principle is stated as "don't call us, we'll call you." It has applications in software engineering
Software engineering
Software Engineering is the application of a systematic, disciplined, quantifiable approach to the development, operation, and maintenance of software, and the study of these approaches; that is, the application of engineering to software...
; see also implicit invocation
Implicit invocation
Implicit invocation is a term used by some authors for a style of software architecture in which a system is structured around event handling, using a form of callback...
for a related architectural principle.
Overview
The Hollywood principle is a software design methodology that takes its name from the cliché response given to amateurs auditioning in Hollywood: "Don't call us, we'll call you". It is a useful paradigm that assists in the development of code with high cohesionCohesion (computer science)
In computer programming, cohesion is a measure of how strongly-related each piece of functionality expressed by the source code of a software module is...
and low coupling
Loose coupling
In computing and systems design a loosely coupled system is one where each of its components has, or makes use of, little or no knowledge of the definitions of other separate components. The notion was introduced into organizational studies by Karl Weick...
that is easier to debug, maintain and test.
Most beginners are first introduced to programming from a diametrically opposed viewpoint. Programs such as Hello World
Hello world program
A "Hello world" program is a computer program that outputs "Hello world" on a display device. Because it is typically one of the simplest programs possible in most programming languages, it is by tradition often used to illustrate to beginners the most basic syntax of a programming language, or to...
take control of the running environment and make calls on the underlying system to do their work. A considerable amount of successful software has been developed using the same principles, and indeed many developers need never think there is any other approach. After all, programs with linear flow are generally easy to understand.
As systems increase in complexity, the linear model becomes less maintainable. Consider for example a simple program to bounce a square around a window in your favorite operating system or window manager. The linear approach may work, up to a point. You can keep the moving and drawing code in separate procedures, but soon the logic begins to branch.
- What happens if the user resizes the window?
- Or if the square is partially off-screen?
- Are all those system callSystem callIn computing, a system call is how a program requests a service from an operating system's kernel. This may include hardware related services , creating and executing new processes, and communicating with integral kernel services...
s to get such resources as device contexts and interacting with the graphical user interface really part of the solution domain?
It would be much more elegant if the programmer could concentrate on the application (in this case, updating the coordinates of the box) and leave the parts common to every application to "something else".
The key to making this possible is to sacrifice the element of control. Instead of your program running the system, the system runs your program. In our example, our program could register for timer events, and write a corresponding event handler that updates the coordinates. The program would include other callbacks
Callback (computer science)
In computer programming, a callback is a reference to executable code, or a piece of executable code, that is passed as an argument to other code. This allows a lower-level software layer to call a subroutine defined in a higher-level layer....
to respond to other events
Event-driven programming
In computer programming, event-driven programming or event-based programming is a programming paradigm in which the flow of the program is determined by events—i.e., sensor outputs or user actions or messages from other programs or threads.Event-driven programming can also be defined as an...
, such as when the system requires part of a window to be redrawn. The system should provide suitable context information so the handler can perform the task and return. The user's program no longer includes an explicit control path
Control flow
In computer science, control flow refers to the order in which the individual statements, instructions, or function calls of an imperative or a declarative program are executed or evaluated....
, aside from initialization and registration.
Event loop
Event loop
In computer science, the event loop, message dispatcher, message loop, message pump, or run loop is a programming construct that waits for and dispatches events or messages in a program...
programming, however, is merely the beginning of software development following the Hollywood principle. More advanced schemes such as event-driven object-orientation go further along the path, by software components sending messages
Message passing
Message passing in computer science is a form of communication used in parallel computing, object-oriented programming, and interprocess communication. In this model, processes or objects can send and receive messages to other processes...
to each other and reacting to the messages they receive. Each message handler merely has to perform its own local processing. It becomes very easy to unit test individual components of the system in isolation, while integration of all the components typically does not have to concern itself excessively with the dependencies between them.
Software architecture
Software architecture
The software architecture of a system is the set of structures needed to reason about the system, which comprise software elements, relations among them, and properties of both...
that encourages the Hollywood principle typically becomes more than "just" an API
Application programming interface
An application programming interface is a source code based specification intended to be used as an interface by software components to communicate with each other...
– instead, it may take on more dominant roles such as a software framework
Software framework
In computer programming, a software framework is an abstraction in which software providing generic functionality can be selectively changed by user code, thus providing application specific software...
or container
Container (data structure)
In computer science, a container is a class, a data structure, or an abstract data type whose instances are collections of other objects. In other words; they are used for storing objects in an organized way following specific access rules...
. Examples:
- In the WindowsMicrosoft WindowsMicrosoft Windows is a series of operating systems produced by Microsoft.Microsoft introduced an operating environment named Windows on November 20, 1985 as an add-on to MS-DOS in response to the growing interest in graphical user interfaces . Microsoft Windows came to dominate the world's personal...
world:- MFCMicrosoft Foundation Class LibraryThe Microsoft Foundation Class Library is a library that wraps portions of the Windows API in C++ classes, including functionality that enables them to use a default application framework...
is an example of a framework for C++C++C++ is a statically typed, free-form, multi-paradigm, compiled, general-purpose programming language. It is regarded as an intermediate-level language, as it comprises a combination of both high-level and low-level language features. It was developed by Bjarne Stroustrup starting in 1979 at Bell...
developers to interact with the Windows environment. - .NET framework.NET FrameworkThe .NET Framework is a software framework that runs primarily on Microsoft Windows. It includes a large library and supports several programming languages which allows language interoperability...
is touted as a framework for scalable enterprise applicationsEnterprise softwareEnterprise software, also known as enterprise application software , is software used in organizations, such as in a business or government, contrary to software chosen by individuals...
.
- MFC
- On the JavaJava (programming language)Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities...
side:- Enterprise JavaBeanEnterprise JavaBeanEnterprise JavaBeans is a managed, server-side component architecture for modular construction of enterprise applications.The EJB specification is one of several Java APIs in the Java EE specification. EJB is a server-side model that encapsulates the business logic of an application...
s specification describes the responsibilities of an EJB container, which must support such enterprise features as remote procedure calls and transaction management.
- Enterprise JavaBean
All of these mechanisms require some cooperation from the developer. To integrate seamlessly with the framework, the developer must produce code that follows some conventions and requirements of the framework. This may be something as simple as implementing a specific interface, or, as in the case of EJB, a significant amount of wrapper code, often produced by code generation tools.
Recent paradigms
More recent paradigms and design patternDesign pattern
A design pattern in architecture and computer science is a formal way of documenting a solution to a design problem in a particular field of expertise. The idea was introduced by the architect Christopher Alexander in the field of architecture and has been adapted for various other disciplines,...
s go even further in pursuit of the Hollywood principle. Inversion of control
Inversion of Control
In software engineering, Inversion of Control is an abstract principle describing an aspect of some software architecture designs in which the flow of control of a system is inverted in comparison to procedural programming....
for instance takes even the integration and configuration of the system out of the application, and instead performs dependency injection
Dependency injection
Dependency injection is a design pattern in object-oriented computer programming whose purpose is to improve testability of, and simplify deployment of components in very large software systems....
.
Again, this is most easily illustrated by an example. A more complex program such as a financial application is likely to depend on several external resources, such as database connections. Traditionally, the code to connect to the database ends up as a procedure somewhere in the program. It becomes difficult to change the database or test the code without one. The same is true for every other external resource that the application uses.
Various design patterns exist to try to reduce the coupling in such applications. In the Java world, the Service locator pattern
Service locator pattern
The service locator pattern is a design pattern used in software development to encapsulate the processes involved in obtaining a service with a strong abstraction layer...
exists to look up resources in a directory, such as JNDI. This reduces the dependency – now, instead of every separate resource having its own initialization code, the program depends only on the service locator.
Inversion of control
Inversion of control containers take the next logical step. In this example, the configuration and location of the database (and all the other resources) is kept in a configuration fileConfiguration file
In computing, configuration files, or config files configure the initial settings for some computer programs. They are used for user applications, server processes and operating system settings. The files are often written in ASCII and line-oriented, with lines terminated by a newline or carriage...
external from the code. The container is responsible for resolution of these dependencies, and delivers them to the other software components – for example by calling a setter method
Mutator method
In computer science, a mutator method is a method used to control changes to a variable.The mutator method, sometimes called a "setter", is most often used in object-oriented programming, in keeping with the principle of encapsulation...
. The code itself does not contain any configuration. Changing the database, or replacing it with a suitable mock object
Mock object
In object-oriented programming, mock objects are simulated objects that mimic the behavior of real objects in controlled ways. A programmer typically creates a mock object to test the behavior of some other object, in much the same way that a car designer uses a crash test dummy to simulate the...
for unit testing, becomes a relatively simple matter of changing the external configuration. Integration of software components is facilitated, and the individual components get even closer to the Hollywood principle.