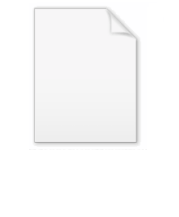
Field encapsulation
Encyclopedia
In computer programming
, field encapsulation, also called data hiding, involves providing method
s that can be used to read/write to/from the field
rather than accessing the field directly. Sometimes these accessor methods are called getX and setX (where X is the field's name), which are also known as mutator method
s. Usually the accessor methods have public visibility while the field being encapsulated is given private visibility - this allows a programmer to restrict what actions another user of the code can perform. Compare the following Java
class
in which the name field has not been encapsulated:
public class NormalFieldClass {
public String name;
public static void main(String[] args)
{
NormalFieldClass example1 = new NormalFieldClass;
example1.name = "myName";
System.out.println("My name is " + example1.name);
}
}
with the same example using encapsulation:
public class EncapsulatedFieldClass {
private String name;
public String getName
{
return name;
}
public void setName(String newName)
{
name = newName;
}
public static void main(String[] args)
{
EncapsulatedFieldClass example1 = new EncapsulatedFieldClass;
example1.setName("myName");
System.out.println("My name is " + example1.getName);
}
}
In the first example a user is free to use the public name variable however they see fit - in the second however the writer of the class retains control over how the private name variable is read and written by only permitting access to the field via its getName and setName methods.
Computer programming
Computer programming is the process of designing, writing, testing, debugging, and maintaining the source code of computer programs. This source code is written in one or more programming languages. The purpose of programming is to create a program that performs specific operations or exhibits a...
, field encapsulation, also called data hiding, involves providing method
Method (computer science)
In object-oriented programming, a method is a subroutine associated with a class. Methods define the behavior to be exhibited by instances of the associated class at program run time...
s that can be used to read/write to/from the field
Field (computer science)
In computer science, data that has several parts can be divided into fields. Relational databases arrange data as sets of database records, also called rows. Each record consists of several fields; the fields of all records form the columns....
rather than accessing the field directly. Sometimes these accessor methods are called getX and setX (where X is the field's name), which are also known as mutator method
Mutator method
In computer science, a mutator method is a method used to control changes to a variable.The mutator method, sometimes called a "setter", is most often used in object-oriented programming, in keeping with the principle of encapsulation...
s. Usually the accessor methods have public visibility while the field being encapsulated is given private visibility - this allows a programmer to restrict what actions another user of the code can perform. Compare the following Java
Java (programming language)
Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities...
class
Class (computer science)
In object-oriented programming, a class is a construct that is used as a blueprint to create instances of itself – referred to as class instances, class objects, instance objects or simply objects. A class defines constituent members which enable these class instances to have state and behavior...
in which the name field has not been encapsulated:
public class NormalFieldClass {
public String name;
public static void main(String[] args)
{
NormalFieldClass example1 = new NormalFieldClass;
example1.name = "myName";
System.out.println("My name is " + example1.name);
}
}
with the same example using encapsulation:
public class EncapsulatedFieldClass {
private String name;
public String getName
{
return name;
}
public void setName(String newName)
{
name = newName;
}
public static void main(String[] args)
{
EncapsulatedFieldClass example1 = new EncapsulatedFieldClass;
example1.setName("myName");
System.out.println("My name is " + example1.getName);
}
}
In the first example a user is free to use the public name variable however they see fit - in the second however the writer of the class retains control over how the private name variable is read and written by only permitting access to the field via its getName and setName methods.
Advantages
- The internal storage format of the data is hidden; in the example, an expectation of the use of restricted character sets could allow data compression through recoding (e.g., of eight bit characters to a six bit code). An attempt to encode characters out of the range of the expected data could then be handled by casting an error in the set routine.
- In general, the get and set methods may be produced in two versions - an efficient method that assumes that the caller is delivering appropriate data and that the data has been stored properly, and a debuggingDebuggingDebugging is a methodical process of finding and reducing the number of bugs, or defects, in a computer program or a piece of electronic hardware, thus making it behave as expected. Debugging tends to be harder when various subsystems are tightly coupled, as changes in one may cause bugs to emerge...
version that while slower, performs validity checks on data received and delivered. Such detection is useful when routines (calling or called) or internal storage formats are newly created or modified. - The location of the stored data within larger structures may be hidden and so enabling changes to be made to this storage without the necessity of changing the code that references the data. This also reduces the likelihood of unexpected side effectsSide effect (computer science)In computer science, a function or expression is said to have a side effect if, in addition to returning a value, it also modifies some state or has an observable interaction with calling functions or the outside world...
from such changes. This is especially advantageous when the accessors are part of an operating systemOperating systemAn operating system is a set of programs that manage computer hardware resources and provide common services for application software. The operating system is the most important type of system software in a computer system...
(OS), a case where the calling (application) code may not be available to the developers of the OS.
Disadvantages
- Access to a subroutine involves additional overhead not present when data is accessed directly. While this is becoming of less concern with the wide availability of fast general-purpose processors it may remain important in coding some real-time computingReal-time computingIn computer science, real-time computing , or reactive computing, is the study of hardware and software systems that are subject to a "real-time constraint"— e.g. operational deadlines from event to system response. Real-time programs must guarantee response within strict time constraints...
systems and systems using relatively slow and simple embedded processorEmbedded systemAn embedded system is a computer system designed for specific control functions within a larger system. often with real-time computing constraints. It is embedded as part of a complete device often including hardware and mechanical parts. By contrast, a general-purpose computer, such as a personal...
s.- In some languages, like C++, the getter / setter methods are usually inline functionInline functionIn various versions of the C and C++ programming languages, an inline function is a function upon which the compiler has been requested to perform inline expansion...
s, so that when inlining is performed, the code looks just like direct field accessing, so it does not have the overhead.
- In some languages, like C++, the getter / setter methods are usually inline function