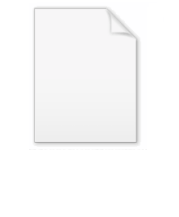
Coupling (computer science)
Encyclopedia
In computer science
, coupling or dependency is the degree to which each program module relies on each one of the other modules.
Coupling is usually contrasted with cohesion
. Low coupling often correlates with high cohesion, and vice versa. The software quality metrics
of coupling and cohesion were invented by Larry Constantine
, an original developer of Structured Design who was also an early proponent of these concepts (see also SSADM). Low coupling is often a sign of a well-structured computer system
and a good design, and when combined with high cohesion, supports the general goals of high readability and maintainability.
Content coupling (high): Content coupling is when one module modifies or relies on the internal workings of another module (e.g., accessing local data of another module).
Common coupling: Common coupling is when two modules share the same global data (e.g., a global variable).
External coupling: External coupling occurs when two modules share an externally imposed data format, communication protocol, or device interface.This is basically related to the communication to external tools and devices.
Control coupling: Control coupling is one module controlling the flow of another, by passing it information on what to do (e.g., passing a what-to-do flag).
Stamp coupling (Data-structured coupling): Stamp coupling is when modules share a composite data structure and use only a part of it, possibly a different part (e.g., passing a whole record to a function that only needs one field of it).
Data coupling: Data coupling is when modules share data through, for example, parameters. Each datum is an elementary piece, and these are the only data shared (e.g., passing an integer to a function that computes a square root).
Message coupling (low): This is the loosest type of coupling. It can be achieved by state decentralization (as in objects) and component communication is done via parameters or message passing (see Message passing
).
No coupling: Modules do not communicate at all with one another.
Temporal coupling: When two actions are bundled together into one module just because they happen to occur at the same time.
.
Message Creation Overhead and Performance: Since all messages and parameters must possess particular meanings to be consumed (i.e., result in intended logical flow within the receiver), they must be created with a particular meaning. Creating any sort of message requires overhead in either CPU or memory usage. Creating a single integer value message (which might be a reference to a string, array or data structure) requires less overhead than creating a complicated message such as a SOAP
message. Longer messages require more CPU and memory to produce. To optimize runtime performance, message length must be minimized and message meaning must be maximized.
Message Transmission Overhead and Performance: Since a message must be transmitted in full to retain its complete meaning, message transmission must be optimized. Longer messages require more CPU and memory to transmit and receive. Also, when necessary, receivers must reassemble a message into its original state to completely receive it. Hence, to optimize runtime performance, message length must be minimized and message meaning must be maximized.
Message Translation Overhead and Performance: Message protocols and messages themselves often contain extra information (i.e., packet, structure, definition and language information). Hence, the receiver often needs to translate a message into a more refined form by removing extra characters and structure information and/or by converting values from one type to another. Any sort of translation increases CPU and/or memory overhead. To optimize runtime performance, message form and content must be reduced and refined to maximize its meaning and reduce translation.
Message Interpretation Overhead and Performance: All messages must be interpreted by the receiver. Simple messages such as integers might not require additional processing to be interpreted. However, complex messages such as SOAP
messages require a parser and a string transformer for them to exhibit intended meanings. To optimize runtime performance, messages must be refined and reduced to minimize interpretation overhead.
, which seeks to limit the responsibilities of modules along functionality, coupling increases between two classes A and B if:
Low coupling refers to a relationship in which one module interacts with another module through a simple and stable interface and does not need to be concerned with the other module's internal implementation (see Information Hiding
).
Systems such as CORBA
or COM
allow objects to communicate with each other without having to know anything about the other object's implementation. Both of these systems even allow for objects to communicate with objects written in other languages.
are the two terms which very frequently occur together. Together they talk about the quality a module should have. Coupling talks about the interdependencies between the various modules while cohesion describes how related functions within a module are. Low cohesion implies that module performs tasks which are not very related to each other and hence can create problems as the module becomes large.
For data and control flow coupling:
For global coupling:
For environmental coupling:

For example, if a module has only a single input and output data parameter

If a module has 5 input and output data parameters, an equal number of control parameters, and accesses 10 items of global data, with a fan-in of 3 and a fan-out of 4,

Computer science
Computer science or computing science is the study of the theoretical foundations of information and computation and of practical techniques for their implementation and application in computer systems...
, coupling or dependency is the degree to which each program module relies on each one of the other modules.
Coupling is usually contrasted with cohesion
Cohesion (computer science)
In computer programming, cohesion is a measure of how strongly-related each piece of functionality expressed by the source code of a software module is...
. Low coupling often correlates with high cohesion, and vice versa. The software quality metrics
Software metric
A software metric is a measure of some property of a piece of software or its specifications. Since quantitative measurements are essential in all sciences, there is a continuous effort by computer science practitioners and theoreticians to bring similar approaches to software development...
of coupling and cohesion were invented by Larry Constantine
Larry Constantine
Larry LeRoy Constantine is an American software engineer and professor in the Mathematics and Engineering Department at the University of Madeira Portugal, who is considered one of the pioneers of computing...
, an original developer of Structured Design who was also an early proponent of these concepts (see also SSADM). Low coupling is often a sign of a well-structured computer system
Computer
A computer is a programmable machine designed to sequentially and automatically carry out a sequence of arithmetic or logical operations. The particular sequence of operations can be changed readily, allowing the computer to solve more than one kind of problem...
and a good design, and when combined with high cohesion, supports the general goals of high readability and maintainability.
Types of coupling
Coupling can be "low" (also "loose" and "weak") or "high" (also "tight" and "strong"). Some types of coupling, in order of highest to lowest coupling, are as follows:Content coupling (high): Content coupling is when one module modifies or relies on the internal workings of another module (e.g., accessing local data of another module).
- Therefore changing the way the second module produces data (location, type, timing) will lead to changing the dependent module.
Common coupling: Common coupling is when two modules share the same global data (e.g., a global variable).
- Changing the shared resource implies changing all the modules using it.
External coupling: External coupling occurs when two modules share an externally imposed data format, communication protocol, or device interface.This is basically related to the communication to external tools and devices.
Control coupling: Control coupling is one module controlling the flow of another, by passing it information on what to do (e.g., passing a what-to-do flag).
Stamp coupling (Data-structured coupling): Stamp coupling is when modules share a composite data structure and use only a part of it, possibly a different part (e.g., passing a whole record to a function that only needs one field of it).
- This may lead to changing the way a module reads a record because a field that the module doesn't need has been modified.
Data coupling: Data coupling is when modules share data through, for example, parameters. Each datum is an elementary piece, and these are the only data shared (e.g., passing an integer to a function that computes a square root).
Message coupling (low): This is the loosest type of coupling. It can be achieved by state decentralization (as in objects) and component communication is done via parameters or message passing (see Message passing
Message passing
Message passing in computer science is a form of communication used in parallel computing, object-oriented programming, and interprocess communication. In this model, processes or objects can send and receive messages to other processes...
).
No coupling: Modules do not communicate at all with one another.
Object-oriented programming
Subclass Coupling: Describes the relationship between a child and its parent. The child is connected to its parent, but the parent isn't connected to the child.Temporal coupling: When two actions are bundled together into one module just because they happen to occur at the same time.
Disadvantages
Tightly coupled systems tend to exhibit the following developmental characteristics, which are often seen as disadvantages:- A change in one module usually forces a ripple effectRipple effectThe ripple effect is a term used to describe a situation where, like the ever expanding ripples across water when an object is dropped into it, an effect from an initial state can be followed outwards incrementally....
of changes in other modules. - Assembly of modules might require more effort and/or time due to the increased inter-module dependency.
- A particular module might be harder to reuse and/or test because dependent modules must be included.
Performance issues
Whether loosely or tightly coupled, a system's performance is often reduced by message and parameter creation, transmission, translation (e.g. marshaling) and interpretation overhead. See event-driven programmingEvent-driven programming
In computer programming, event-driven programming or event-based programming is a programming paradigm in which the flow of the program is determined by events—i.e., sensor outputs or user actions or messages from other programs or threads.Event-driven programming can also be defined as an...
.
Message Creation Overhead and Performance: Since all messages and parameters must possess particular meanings to be consumed (i.e., result in intended logical flow within the receiver), they must be created with a particular meaning. Creating any sort of message requires overhead in either CPU or memory usage. Creating a single integer value message (which might be a reference to a string, array or data structure) requires less overhead than creating a complicated message such as a SOAP
SOAP
SOAP, originally defined as Simple Object Access Protocol, is a protocol specification for exchanging structured information in the implementation of Web Services in computer networks...
message. Longer messages require more CPU and memory to produce. To optimize runtime performance, message length must be minimized and message meaning must be maximized.
Message Transmission Overhead and Performance: Since a message must be transmitted in full to retain its complete meaning, message transmission must be optimized. Longer messages require more CPU and memory to transmit and receive. Also, when necessary, receivers must reassemble a message into its original state to completely receive it. Hence, to optimize runtime performance, message length must be minimized and message meaning must be maximized.
Message Translation Overhead and Performance: Message protocols and messages themselves often contain extra information (i.e., packet, structure, definition and language information). Hence, the receiver often needs to translate a message into a more refined form by removing extra characters and structure information and/or by converting values from one type to another. Any sort of translation increases CPU and/or memory overhead. To optimize runtime performance, message form and content must be reduced and refined to maximize its meaning and reduce translation.
Message Interpretation Overhead and Performance: All messages must be interpreted by the receiver. Simple messages such as integers might not require additional processing to be interpreted. However, complex messages such as SOAP
SOAP
SOAP, originally defined as Simple Object Access Protocol, is a protocol specification for exchanging structured information in the implementation of Web Services in computer networks...
messages require a parser and a string transformer for them to exhibit intended meanings. To optimize runtime performance, messages must be refined and reduced to minimize interpretation overhead.
Solutions
One approach to decreasing coupling is functional designFunctional design
Functional design is a paradigm used to simplify the design of hardware and software devices such as computer software and increasingly, 3D models. A functional design assures that each modular part of a device has only one responsibility and performs that responsibility with the minimum of side...
, which seeks to limit the responsibilities of modules along functionality, coupling increases between two classes A and B if:
- A has an attribute that refers to (is of type) B.
- A calls on services of an object B.
- A has a method that references B (via return type or parameter).
- A is a subclass of (or implements) class B.
Low coupling refers to a relationship in which one module interacts with another module through a simple and stable interface and does not need to be concerned with the other module's internal implementation (see Information Hiding
Information hiding
In computer science, information hiding is the principle of segregation of the design decisions in a computer program that are most likely to change, thus protecting other parts of the program from extensive modification if the design decision is changed...
).
Systems such as CORBA
Çorba
Chorba , ciorbă , shurpa , shorpo , or sorpa is one of various kinds of soup or stew found in national cuisines across Middle East...
or COM
Component Object Model
Component Object Model is a binary-interface standard for software componentry introduced by Microsoft in 1993. It is used to enable interprocess communication and dynamic object creation in a large range of programming languages...
allow objects to communicate with each other without having to know anything about the other object's implementation. Both of these systems even allow for objects to communicate with objects written in other languages.
Coupling versus Cohesion
Coupling and CohesionCohesion (computer science)
In computer programming, cohesion is a measure of how strongly-related each piece of functionality expressed by the source code of a software module is...
are the two terms which very frequently occur together. Together they talk about the quality a module should have. Coupling talks about the interdependencies between the various modules while cohesion describes how related functions within a module are. Low cohesion implies that module performs tasks which are not very related to each other and hence can create problems as the module becomes large.
Module coupling
Coupling in Software Engineering describes a version of metrics associated with this concept.For data and control flow coupling:
- di: number of input data parameters
- ci: number of input control parameters
- do: number of output data parameters
- co: number of output control parameters
For global coupling:
- gd: number of global variables used as data
- gc: number of global variables used as control
For environmental coupling:
- w: number of modules called (fan-out)
- r: number of modules calling the module under consideration (fan-in)

Coupling(C)
makes the value larger the more coupled the module is. This number ranges from approximately 0.67 (low coupling) to 1.0 (highly coupled)For example, if a module has only a single input and output data parameter

If a module has 5 input and output data parameters, an equal number of control parameters, and accesses 10 items of global data, with a fan-in of 3 and a fan-out of 4,

See also
- Cohesion (computer science)Cohesion (computer science)In computer programming, cohesion is a measure of how strongly-related each piece of functionality expressed by the source code of a software module is...
- Dependency hellDependency hellDependency hell is a colloquial term for the frustration of some software users who have installed software packages which have dependencies on specific versions of other software packages. This was mainly attributable to old Linux package managers...
- Efferent couplingEfferent CouplingEfferent Coupling is a metric in software development. It measures the number of data types a class knows about.This includes inheritance, interface implementation, parameter types, variable types, and exceptions....
- Inversion of controlInversion of ControlIn software engineering, Inversion of Control is an abstract principle describing an aspect of some software architecture designs in which the flow of control of a system is inverted in comparison to procedural programming....
- List of object-oriented programming terms
- Loose couplingLoose couplingIn computing and systems design a loosely coupled system is one where each of its components has, or makes use of, little or no knowledge of the definitions of other separate components. The notion was introduced into organizational studies by Karl Weick...
- Make (software)
- Static code analysisStatic code analysisStatic program analysis is the analysis of computer software that is performed without actually executing programs built from that software In most cases the analysis is performed on some version of the source code and in the other cases some form of the object code...