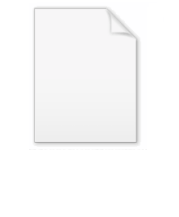
Augmented assignment
Encyclopedia
Augmented assignment is the name given to certain operator
s in certain programming languages (especially those derived from C
). An augmented assignment is generally used to replace a statement where an operator takes a variable
as one of its arguments and then assigns the result back to the same variable.
For example, the following statement or some variation of it can be found in many programs:
x = x + 1
This means "find the number stored in the variable x, add 1 to it, and store the result of the addition in the variable x." As simple as this seems, it may have an inefficiency, in that the location of variable x has to be looked up twice if the compiler does not recognize that two parts of the expression are identical: x might be a reference to some array element or other complexity. In comparison, here is the augmented assignment version:
x += 1
With this version, there is no excuse for a compiler failing to generate code that looks up the location of variable x just once, and modifies it in place, if of course the machine code supports such a sequence. For instance, if x is a simple variable, the machine code sequence might be something like
Load x
Add 1
Store x
and the same code would be generated for both forms. But if there is a special op code, it might be
MDM x,1
meaning "Modify Memory" by adding 1 to x, and a decent compiler would generate the same code for both forms. Some machine codes offer INC and DEC operations (to add or subtract one), others might allow constants other than one.
More generally, the form is
x ?= expression
where the ? stands for some operator (not always +), and there may be no special op codes to help. There is still the possibility that if x is a complicated entity the compiler will be encouraged to avoid duplication in accessing x, and of course, if x is a lengthy name, there will be less typing required. This last was the basis of the similar feature in the Algol compilers offered via the Burroughs B6700 systems, using the tilda symbol to stand for the variable being assigned to, so that
LongName:=x + sqrt(LongName)*7;
would become
LongName:=x + sqrt(~)*7;
and so forth. This is more general than just x:=~ + 1; Producing optimum code would remain the province of the compiler.
In general, in languages offering this feature, most operators that can take a variable as one of their arguments and return a result of the same type have an augmented assignment equivalent that assigns the result back to the variable in place, including arithmetic operators, bitshift operators, and bitwise operators
.
On the other hand, enthusiastic use of these features (especially with sub-expressions within larger expressions) soon produces sequences of symbols that are difficult to read or understand, and worse, a mistype can easily produce a different sequence of gibberish that although accepted by the compiler does not produce desired results.
The C++ assignment operator is = which is augmented as follows
Operator (programming)
Programming languages typically support a set of operators: operations which differ from the language's functions in calling syntax and/or argument passing mode. Common examples that differ by syntax are mathematical arithmetic operations, e.g...
s in certain programming languages (especially those derived from C
C (programming language)
C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system....
). An augmented assignment is generally used to replace a statement where an operator takes a variable
Variable (programming)
In computer programming, a variable is a symbolic name given to some known or unknown quantity or information, for the purpose of allowing the name to be used independently of the information it represents...
as one of its arguments and then assigns the result back to the same variable.
For example, the following statement or some variation of it can be found in many programs:
x = x + 1
This means "find the number stored in the variable x, add 1 to it, and store the result of the addition in the variable x." As simple as this seems, it may have an inefficiency, in that the location of variable x has to be looked up twice if the compiler does not recognize that two parts of the expression are identical: x might be a reference to some array element or other complexity. In comparison, here is the augmented assignment version:
x += 1
With this version, there is no excuse for a compiler failing to generate code that looks up the location of variable x just once, and modifies it in place, if of course the machine code supports such a sequence. For instance, if x is a simple variable, the machine code sequence might be something like
Load x
Add 1
Store x
and the same code would be generated for both forms. But if there is a special op code, it might be
MDM x,1
meaning "Modify Memory" by adding 1 to x, and a decent compiler would generate the same code for both forms. Some machine codes offer INC and DEC operations (to add or subtract one), others might allow constants other than one.
More generally, the form is
x ?= expression
where the ? stands for some operator (not always +), and there may be no special op codes to help. There is still the possibility that if x is a complicated entity the compiler will be encouraged to avoid duplication in accessing x, and of course, if x is a lengthy name, there will be less typing required. This last was the basis of the similar feature in the Algol compilers offered via the Burroughs B6700 systems, using the tilda symbol to stand for the variable being assigned to, so that
LongName:=x + sqrt(LongName)*7;
would become
LongName:=x + sqrt(~)*7;
and so forth. This is more general than just x:=~ + 1; Producing optimum code would remain the province of the compiler.
In general, in languages offering this feature, most operators that can take a variable as one of their arguments and return a result of the same type have an augmented assignment equivalent that assigns the result back to the variable in place, including arithmetic operators, bitshift operators, and bitwise operators
Bitwise operation
A bitwise operation operates on one or more bit patterns or binary numerals at the level of their individual bits. This is used directly at the digital hardware level as well as in microcode, machine code and certain kinds of high level languages...
.
On the other hand, enthusiastic use of these features (especially with sub-expressions within larger expressions) soon produces sequences of symbols that are difficult to read or understand, and worse, a mistype can easily produce a different sequence of gibberish that although accepted by the compiler does not produce desired results.
The C++ assignment operator is = which is augmented as follows
Operator | Description |
---|---|
+= |
Addition |
-= |
Subtraction |
*= |
Multiplication |
/= |
Division |
%= |
Modulus |
<<= |
Left bit shift |
>>= |
Right shift |
&= |
Bitwise AND |
^= |
Bitwise exclusive OR |
|= |
Bitwise inclusive OR |
Supporting languages
The following list, though not complete or all-inclusive, lists some of the major programming languages that support augmented assignment operators.- AWK
- CC (programming language)C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system....
- C++C++C++ is a statically typed, free-form, multi-paradigm, compiled, general-purpose programming language. It is regarded as an intermediate-level language, as it comprises a combination of both high-level and low-level language features. It was developed by Bjarne Stroustrup starting in 1979 at Bell...
- C#
- DD (programming language)The D programming language is an object-oriented, imperative, multi-paradigm, system programming language created by Walter Bright of Digital Mars. It originated as a re-engineering of C++, but even though it is mainly influenced by that language, it is not a variant of C++...
- GoGo (programming language)Go is a compiled, garbage-collected, concurrent programming language developed by Google Inc.The initial design of Go was started in September 2007 by Robert Griesemer, Rob Pike, and Ken Thompson. Go was officially announced in November 2009. In May 2010, Rob Pike publicly stated that Go was being...
- JavaJava (programming language)Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities...
- JavaScriptJavaScriptJavaScript is a prototype-based scripting language that is dynamic, weakly typed and has first-class functions. It is a multi-paradigm language, supporting object-oriented, imperative, and functional programming styles....
- PerlPerlPerl is a high-level, general-purpose, interpreted, dynamic programming language. Perl was originally developed by Larry Wall in 1987 as a general-purpose Unix scripting language to make report processing easier. Since then, it has undergone many changes and revisions and become widely popular...
- PHPPHPPHP is a general-purpose server-side scripting language originally designed for web development to produce dynamic web pages. For this purpose, PHP code is embedded into the HTML source document and interpreted by a web server with a PHP processor module, which generates the web page document...
- PythonPythonThe Pythonidae, commonly known simply as pythons, from the Greek word python-πυθων, are a family of non-venomous snakes found in Africa, Asia and Australia. Among its members are some of the largest snakes in the world...
- RubyRubyA ruby is a pink to blood-red colored gemstone, a variety of the mineral corundum . The red color is caused mainly by the presence of the element chromium. Its name comes from ruber, Latin for red. Other varieties of gem-quality corundum are called sapphires...
See also
- Increment and decrement operatorsIncrement and decrement operatorsIncrement and decrement operators are unary operators that add or subtract one from their operand, respectively. They are commonly implemented in imperative programming languages...
-- special case of augmented assignment, by 1