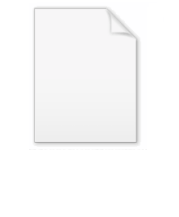
Go (programming language)
Encyclopedia
Go is a compiled
, garbage-collected
, concurrent programming language
developed by Google Inc.
The initial design of Go was started in September 2007 by Robert Griesemer, Rob Pike, and Ken Thompson
. Go was officially announced in November 2009. In May 2010, Rob Pike publicly stated that Go was being used "for real stuff" at Google. Go's "gc" compiler targets the Linux
, Mac OS X
, FreeBSD
, and Microsoft Windows
operating systems and the i386, amd64, and ARM
processor architectures.
: blocks of code are surrounded with curly braces; common control flow
structures include
Go is designed for exceptionally fast compiling times, even on modest hardware. The language requires garbage collection
. Certain concurrency-related structural conventions of Go (channel
s and alternative channel inputs) are borrowed from Tony Hoare's
CSP
. Unlike previous concurrent programming languages such as occam
or Limbo, Go does not provide any built-in notion of safe or verifiable concurrency.
Of features found in C++ or Java, Go does not include type inheritance
, generic programming
, assertions
, method overloading
, or pointer arithmetic. Of these, the Go authors express an openness to generic programming, explicitly argue against assertions and pointer arithmetic, while defending the choice to omit type inheritance as giving a more useful language, encouraging heavy use of interfaces
instead. Initially, the language did not include exception handling
, but in March 2010 a mechanism known as
Go interfaces do not participate in a type hierarchy like Java's. They are better described as a set of methods, identified by their name and signature. An interface can be declared to embed other interfaces, meaning the declared interface borrows the methods defined in the other interfaces, making them part of the set of methods of the declared interface . A type matches an interface if it defines the methods (same name and same signature) from this interface.
Visibility
of structures, structure fields, variables, constants, methods, top-level types and functions outside of their defining package is defined implicitly according to the capitalization of their identifier.
Both compilers work on Unix-like systems, and a port to Microsoft Windows of the gc compiler and runtime have been integrated in the main distribution. Most of the standard libraries also work on Windows.
There is also an unmaintained "tiny" runtime environment that allows Go programs to run on bare hardware.
in Go:
package main
import "fmt"
func main {
fmt.Println("Hello, World")
}
Go's automatic semicolon
insertion feature requires that opening braces not be placed on their own lines, and this is thus the preferred brace style; the examples shown comply with this style.
Example illustrating how to write a program like the Unix echo command in Go:
package main
import (
"os"
"flag" // command line option parser
)
var omitNewline = flag.Bool("n", false, "don't print final newline")
const (
Space = " "
Newline = "\n"
)
func main {
flag.Parse // Scans the arg list and sets up flags
var s string
for i := 0; i < flag.NArg; i++ {
if i > 0 {
s += Space
}
s += flag.Arg(i)
}
if !*omitNewline {
s += Newline
}
os.Stdout.WriteString(s)
}
David Given compared it unfavorably to another programming language he called "Brand X," which was finally revealed to be Algol 68
, commenting that this showed an overall lack of progress in procedural programming language design over the course of the intervening 41 years.
Michele Simionato wrote in an article for artima.com:
Dave Astels
at Engine Yard
wrote:
Blogger Michael Hoisie wrote:
Ars Technica
interviewed Rob Pike, one of the authors of Go, and asked why a new language was needed. He replied that:
Go entered the TIOBE Programming Community Index at fifteenth place in its first year, surpassing established languages like Pascal
. , it ranked 32nd in the index.
Bruce Eckel
stated:
(note the exclamation point), requested a name change of Google's language to prevent confusion with his language. While McCabe has not trademarked the name, some commenters on McCabe's request called for Google to adopt a new one. However, contradicting established practice of eliminating name clashes (frequently resorting to court
), the issue was closed on 12 October 2010 with the custom status "Unfortunate", the closing Google developer stating that "there are many computing products and services named Go. In the 11 months since our release, there has been minimal confusion of the two languages."
s. Goroutines are created with the
Goroutines are executed in parallel with other goroutines, including their caller. They do not necessarily run in separate threads, but a group of goroutines are multiplexed onto multiple threads — execution control is moved between them by blocking them when sending or receiving messages over channels.
Compiled language
A compiled language is a programming language whose implementations are typically compilers , and not interpreters ....
, garbage-collected
Garbage collection (computer science)
In computer science, garbage collection is a form of automatic memory management. The garbage collector, or just collector, attempts to reclaim garbage, or memory occupied by objects that are no longer in use by the program...
, concurrent programming language
Programming language
A programming language is an artificial language designed to communicate instructions to a machine, particularly a computer. Programming languages can be used to create programs that control the behavior of a machine and/or to express algorithms precisely....
developed by Google Inc.
Google
Google Inc. is an American multinational public corporation invested in Internet search, cloud computing, and advertising technologies. Google hosts and develops a number of Internet-based services and products, and generates profit primarily from advertising through its AdWords program...
The initial design of Go was started in September 2007 by Robert Griesemer, Rob Pike, and Ken Thompson
Ken Thompson
Kenneth Lane Thompson , commonly referred to as ken in hacker circles, is an American pioneer of computer science...
. Go was officially announced in November 2009. In May 2010, Rob Pike publicly stated that Go was being used "for real stuff" at Google. Go's "gc" compiler targets the Linux
Linux
Linux is a Unix-like computer operating system assembled under the model of free and open source software development and distribution. The defining component of any Linux system is the Linux kernel, an operating system kernel first released October 5, 1991 by Linus Torvalds...
, Mac OS X
Mac OS X
Mac OS X is a series of Unix-based operating systems and graphical user interfaces developed, marketed, and sold by Apple Inc. Since 2002, has been included with all new Macintosh computer systems...
, FreeBSD
FreeBSD
FreeBSD is a free Unix-like operating system descended from AT&T UNIX via BSD UNIX. Although for legal reasons FreeBSD cannot be called “UNIX”, as the direct descendant of BSD UNIX , FreeBSD’s internals and system APIs are UNIX-compliant...
, and Microsoft Windows
Microsoft Windows
Microsoft Windows is a series of operating systems produced by Microsoft.Microsoft introduced an operating environment named Windows on November 20, 1985 as an add-on to MS-DOS in response to the growing interest in graphical user interfaces . Microsoft Windows came to dominate the world's personal...
operating systems and the i386, amd64, and ARM
ARM
An arm is an upper limb of the body.Arm may also refer to:-Geography:* Arm , a narrow stretch of a larger body of water** Canal arm, a subsidiary branch of a canal or inland waterway** Distributary or arm, a subsidiary branch of a river...
processor architectures.
Goals
Go aims to provide the efficiency of a statically-typed compiled language with the ease of programming of a dynamic language. Other goals include:- Safety: type-safeType safetyIn computer science, type safety is the extent to which a programming language discourages or prevents type errors. A type error is erroneous or undesirable program behaviour caused by a discrepancy between differing data types...
and memory-safeMemory safetyMemory safety is a concern in software development that aims to avoid software bugs that cause security vulnerabilities dealing with random-access memory access, such as buffer overflows and dangling pointers....
. - Good support for concurrency and communication.
- Efficient, latency-free garbage collection.
- High-speed compilation.
Description
The syntax of Go is broadly similar to that of CC (programming language)
C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system....
: blocks of code are surrounded with curly braces; common control flow
Control flow
In computer science, control flow refers to the order in which the individual statements, instructions, or function calls of an imperative or a declarative program are executed or evaluated....
structures include
forFor loopIn computer science a for loop is a programming language statement which allows code to be repeatedly executed. A for loop is classified as an iteration statement....
, switchSwitch statementIn computer programming, a switch, case, select or inspect statement is a type of selection control mechanism that exists in most imperative programming languages such as Pascal, Ada, C/C++, C#, Java, and so on. It is also included in several other types of languages...
, and if
. Unlike C, line-ending semicolons are optional; variable declarations are written differently and are usually optional; type conversions must be made explicit; and new go
and select control keywords have been introduced to support concurrent programming. New built-in types include maps, Unicode strings, array slices, and channels for inter-thread communication.Go is designed for exceptionally fast compiling times, even on modest hardware. The language requires garbage collection
Garbage collection (computer science)
In computer science, garbage collection is a form of automatic memory management. The garbage collector, or just collector, attempts to reclaim garbage, or memory occupied by objects that are no longer in use by the program...
. Certain concurrency-related structural conventions of Go (channel
Channel (programming)
A Channel is a construct used in interprocess communication to represent some binding between concurrent processes. An object may be sent over a channel, and a process is able to receive any objects sent over a channel it has a reference to...
s and alternative channel inputs) are borrowed from Tony Hoare's
C. A. R. Hoare
Sir Charles Antony Richard Hoare , commonly known as Tony Hoare or C. A. R. Hoare, is a British computer scientist best known for the development of Quicksort, one of the world's most widely used sorting algorithms...
CSP
Communicating sequential processes
In computer science, Communicating Sequential Processes is a formal language for describing patterns of interaction in concurrent systems. It is a member of the family of mathematical theories of concurrency known as process algebras, or process calculi...
. Unlike previous concurrent programming languages such as occam
Occam (programming language)
occam is a concurrent programming language that builds on the Communicating Sequential Processes process algebra, and shares many of its features. It is named after William of Ockham of Occam's Razor fame....
or Limbo, Go does not provide any built-in notion of safe or verifiable concurrency.
Of features found in C++ or Java, Go does not include type inheritance
Inheritance (object-oriented programming)
In object-oriented programming , inheritance is a way to reuse code of existing objects, establish a subtype from an existing object, or both, depending upon programming language support...
, generic programming
Generic programming
In a broad definition, generic programming is a style of computer programming in which algorithms are written in terms of to-be-specified-later types that are then instantiated when needed for specific types provided as parameters...
, assertions
Assertion (computing)
In computer programming, an assertion is a predicate placed in a program to indicate that the developer thinks that the predicate is always true at that place.For example, the following code contains two assertions:...
, method overloading
Method overloading
Function overloading or method overloading is a feature found in various programming languages such as Ada, C#, VB.NET, C++, D and Java that allows the creation of several methods with the same name which differ from each other in terms of the type of the input and the type of the output of the...
, or pointer arithmetic. Of these, the Go authors express an openness to generic programming, explicitly argue against assertions and pointer arithmetic, while defending the choice to omit type inheritance as giving a more useful language, encouraging heavy use of interfaces
Protocol (object-oriented programming)
In object-oriented programming, a protocol or interface is a common means for unrelated objects to communicate with each other. These are definitions of methods and values which the objects agree upon in order to cooperate....
instead. Initially, the language did not include exception handling
Exception handling
Exception handling is a programming language construct or computer hardware mechanism designed to handle the occurrence of exceptions, special conditions that change the normal flow of program execution....
, but in March 2010 a mechanism known as
panic
/recover
was implemented to handle exceptional errors while avoiding some of the problems the Go authors find with exceptions.Go interfaces do not participate in a type hierarchy like Java's. They are better described as a set of methods, identified by their name and signature. An interface can be declared to embed other interfaces, meaning the declared interface borrows the methods defined in the other interfaces, making them part of the set of methods of the declared interface . A type matches an interface if it defines the methods (same name and same signature) from this interface.
Visibility
Linkage (software)
In programming languages, particularly C++, linkage describes how names can or can not refer to the same entity throughout the whole program or one single translation unit....
of structures, structure fields, variables, constants, methods, top-level types and functions outside of their defining package is defined implicitly according to the capitalization of their identifier.
Implementations
There are currently two Go compilers:- 6g/8g/5g (the compilers for AMD64, x86, and ARM respectively) with their supporting tools (collectively known as "gc") based on Ken's previous work on Plan 9Plan 9 from Bell LabsPlan 9 from Bell Labs is a distributed operating system. It was developed primarily for research purposes as the successor to Unix by the Computing Sciences Research Center at Bell Labs between the mid-1980s and 2002...
's C toolchain. - gccgo, a GCCGNU Compiler CollectionThe GNU Compiler Collection is a compiler system produced by the GNU Project supporting various programming languages. GCC is a key component of the GNU toolchain...
frontend written in C++, and now officially supported as of version 4.6, albeit not part of the standard binary for gcc.
Both compilers work on Unix-like systems, and a port to Microsoft Windows of the gc compiler and runtime have been integrated in the main distribution. Most of the standard libraries also work on Windows.
There is also an unmaintained "tiny" runtime environment that allows Go programs to run on bare hardware.
Examples
The following is a Hello world programHello world program
A "Hello world" program is a computer program that outputs "Hello world" on a display device. Because it is typically one of the simplest programs possible in most programming languages, it is by tradition often used to illustrate to beginners the most basic syntax of a programming language, or to...
in Go:
package main
import "fmt"
func main {
fmt.Println("Hello, World")
}
Go's automatic semicolon
Semicolon
The semicolon is a punctuation mark with several uses. The Italian printer Aldus Manutius the Elder established the practice of using the semicolon to separate words of opposed meaning and to indicate interdependent statements. "The first printed semicolon was the work of ... Aldus Manutius"...
insertion feature requires that opening braces not be placed on their own lines, and this is thus the preferred brace style; the examples shown comply with this style.
Example illustrating how to write a program like the Unix echo command in Go:
package main
import (
"os"
"flag" // command line option parser
)
var omitNewline = flag.Bool("n", false, "don't print final newline")
const (
Space = " "
Newline = "\n"
)
func main {
flag.Parse // Scans the arg list and sets up flags
var s string
for i := 0; i < flag.NArg; i++ {
if i > 0 {
s += Space
}
s += flag.Arg(i)
}
if !*omitNewline {
s += Newline
}
os.Stdout.WriteString(s)
}
Reception
Go's initial release led to much discussion.David Given compared it unfavorably to another programming language he called "Brand X," which was finally revealed to be Algol 68
ALGOL 68
ALGOL 68 isan imperative computerprogramming language that was conceived as a successor to theALGOL 60 programming language, designed with the goal of a...
, commenting that this showed an overall lack of progress in procedural programming language design over the course of the intervening 41 years.
Michele Simionato wrote in an article for artima.com:
Dave Astels
Dave Astels
Dave Astels is a software consultant and advocate of agile software development.Astels has written on extreme programming and test-driven development. Astels is a contributor to the RSpec behaviour-driven development....
at Engine Yard
Engine Yard
Engine Yard is a San Francisco, California based company focused on Ruby on Rails deployment and management-Software development:One of Engine Yard's four founders, Ezra Zygmuntowicz, was the creator of the Merb project, and the company continued supporting the project by hiring Yehuda Katz to work...
wrote:
Blogger Michael Hoisie wrote:
Ars Technica
Ars Technica
Ars Technica is a technology news and information website created by Ken Fisher and Jon Stokes in 1998. It publishes news, reviews and guides on issues such as computer hardware and software, science, technology policy, and video games. Ars Technica is known for its features, long articles that go...
interviewed Rob Pike, one of the authors of Go, and asked why a new language was needed. He replied that:
Go entered the TIOBE Programming Community Index at fifteenth place in its first year, surpassing established languages like Pascal
Pascal (programming language)
Pascal is an influential imperative and procedural programming language, designed in 1968/9 and published in 1970 by Niklaus Wirth as a small and efficient language intended to encourage good programming practices using structured programming and data structuring.A derivative known as Object Pascal...
. , it ranked 32nd in the index.
Bruce Eckel
Bruce Eckel
Bruce Eckel is the author of numerous books and articles about computer programming. He also gives frequent lectures and seminars for computer programmers...
stated:
Naming dispute
On the day of the general release of the language, Francis McCabe, developer of the Go! programming languageGo! (programming language)
Go! is an agent-based programming language in the tradition of logic-based programming languages like Prolog. It was introduced in a 2003 paper by Francis McCabe and Keith Clark....
(note the exclamation point), requested a name change of Google's language to prevent confusion with his language. While McCabe has not trademarked the name, some commenters on McCabe's request called for Google to adopt a new one. However, contradicting established practice of eliminating name clashes (frequently resorting to court
Court
A court is a form of tribunal, often a governmental institution, with the authority to adjudicate legal disputes between parties and carry out the administration of justice in civil, criminal, and administrative matters in accordance with the rule of law...
), the issue was closed on 12 October 2010 with the custom status "Unfortunate", the closing Google developer stating that "there are many computing products and services named Go. In the 11 months since our release, there has been minimal confusion of the two languages."
Concurrency
Go provides goroutines, small lightweight threads; the name alludes to coroutineCoroutine
Coroutines are computer program components that generalize subroutines to allow multiple entry points for suspending and resuming execution at certain locations...
s. Goroutines are created with the
go
statement from anonymous or named functions.Goroutines are executed in parallel with other goroutines, including their caller. They do not necessarily run in separate threads, but a group of goroutines are multiplexed onto multiple threads — execution control is moved between them by blocking them when sending or receiving messages over channels.
External links
(video) — A university lecture — Podcast interview with Rob Pike, Tech Lead for the Google Go team- Go Programming Language Resources (unofficial)
- irc://chat.freenode.net/#go-nuts – the IRC channel #go-nuts on freenodeFreenodefreenode, formerly known as Open Projects Network, is an IRC network used to discuss peer-directed projects. Their servers are all accessible from the domain name [irc://chat.freenode.net chat.freenode.net], which load balances connections by using the actual servers in rotation...
- Interview about Go with Andrew Gerrand on Coding By Numbers podcast