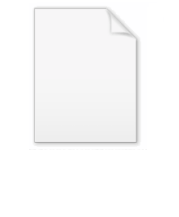
Wchar.h
Encyclopedia
C wide string handling refers to a group of functions implementing operations on wide strings in the C Standard Library
. Various operations, such as copying, concatenation, tokenization and searching are supported.
The only support in the C programming language itself for wide strings is that the compiler will translate a quoted wide string constant in the source into a null-terminated wide string
stored in static memory.
that was developed in an environment where the dominant character set was the 7-bit ASCII
code. Hence since then the 8-bit byte is the most common unit of encoding. However when a software is developed for an international purpose, it has to be able to represent different characters. For example character encoding schemes to represent the Indian, Chinese, Japanese writing systems should be available. The inconvenience of handling such varied multibyte characters can be eliminated by using characters that are simply a uniform number of bytes. ANSI C
provides a type that allows manipulation of variable width characters as uniform sized data objects called wide characters. The wide character set is a superset
of already existing character sets, including the 7-bit ASCII.
Wide string examination
Memory manipulation
C standard library
The C Standard Library is the standard library for the programming language C, as specified in the ANSI C standard.. It was developed at the same time as the C POSIX library, which is basically a superset of it...
. Various operations, such as copying, concatenation, tokenization and searching are supported.
The only support in the C programming language itself for wide strings is that the compiler will translate a quoted wide string constant in the source into a null-terminated wide string
Null-terminated string
In computer programming, a null-terminated string is a character string stored as an array containing the characters and terminated with a null character...
stored in static memory.
Wide Characters
C is a programming languageProgramming language
A programming language is an artificial language designed to communicate instructions to a machine, particularly a computer. Programming languages can be used to create programs that control the behavior of a machine and/or to express algorithms precisely....
that was developed in an environment where the dominant character set was the 7-bit ASCII
ASCII
The American Standard Code for Information Interchange is a character-encoding scheme based on the ordering of the English alphabet. ASCII codes represent text in computers, communications equipment, and other devices that use text...
code. Hence since then the 8-bit byte is the most common unit of encoding. However when a software is developed for an international purpose, it has to be able to represent different characters. For example character encoding schemes to represent the Indian, Chinese, Japanese writing systems should be available. The inconvenience of handling such varied multibyte characters can be eliminated by using characters that are simply a uniform number of bytes. ANSI C
ANSI C
ANSI C refers to the family of successive standards published by the American National Standards Institute for the C programming language. Software developers writing in C are encouraged to conform to the standards, as doing so aids portability between compilers.-History and outlook:The first...
provides a type that allows manipulation of variable width characters as uniform sized data objects called wide characters. The wide character set is a superset
SuperSet
SuperSet Software was a group founded by friends and former Eyring Research Institute co-workers Drew Major, Dale Neibaur, Kyle Powell and later joined by Mark Hurst...
of already existing character sets, including the 7-bit ASCII.
Macros
The standard header wchar.h contains the definitions or declarations of some constants.- NULL
- It is a Null pointer constant. It never points to a real object.
- WCHAR_MIN
- It indicates the lower limit or the minimum value for the type wchar_t.
- WCHAR_MAX
- It indicates the upper limit or the maximum value for the type wchar_t.
- WEOF
- It defines the return value of the type wint_t but the value does not correspond to any member of the extended character set. WEOF indicates the end of a character stream, the end of file(EOFEOFEOF may refer to:*End-of-file, the computing term for an end-of-file condition or its tangible indication*Empirical orthogonal functions, a statistical technique for simplifying a dataset*Enterprise Objects Framework, a product from Apple Computer...
) or an error case.
Data Types
- mbstate_t
- A variable of type mbstate_t contains all the information about the conversion state required from one call to a function to the other.
- size_t
- It is a size/count type, that stores the result or the returned value of the size of operator.
- wchar_t
- An object of type wchar_t can hold a wide character. It is also required for declaring or referencing wide characters and wide strings.
- wint_t
- This type is an integer type that can hold any value corresponding to the members of the extended character set. It can hold all values of the type wchar_t as well as the value of the macro WEOF. This type is unchanged by integral promotions.
Functions
Wide string manipulationwcscpy
- copies one wide string to anotherwcsncpy
- writes exactly n characters to a wide string, copying from given string or adding nullswcscat
- appends one wide string to anotherwcsncat
- appends no more than n characters from one wide string to anotherwcsxfrm
- transforms a wide string according to the current localeWide string examination
wcslen
- returns the length of a wide stringwcscmp
- compares two wide stringswcsncmp
- compares a specific number of characters in two wide stringswcscoll
- compares two wide strings according to the current localewcschr
- finds the first occurrence of a character in a wide stringwcsrchr
- finds the last occurrence of a character in a wide stringwcsspn
- finds in a wide string the first occurrence of a character not in a set of characterswcscspn
- finds in a wide string the last occurrence of a character not in a set of characterswcspbrk
- finds in a wide string the first occurrence of a character in a set of characterswcsstr
- finds in a wide string the first occurrence of a substringwcstok
- finds in a wide string the next occurrence of a tokenMemory manipulation
wmemset
- fills a buffer with a repeated wide characterwmemcpy
- copies one buffer to anotherwmemmove
- copies one buffer to another, possibly overlapping, bufferwmemcmp
- compares two bufferswmemchr
- finds the first occurrence of a wide character in a bufferConversion Functions
Name | Notes |
---|---|
wint_t btowc(int c); |
returns the result after converting c into its wide character equivalent and on error returns WEOF. |
int wctob(wint_t c); |
returns the one byte or multibyte equivalent of c and on error returns WEOF. |