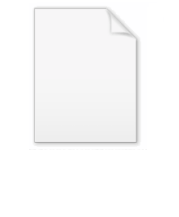
Thread pool pattern
Encyclopedia
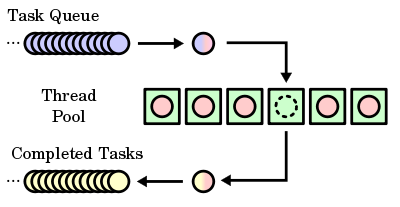
Computer programming
Computer programming is the process of designing, writing, testing, debugging, and maintaining the source code of computer programs. This source code is written in one or more programming languages. The purpose of programming is to create a program that performs specific operations or exhibits a...
, the thread pool pattern
Design pattern (computer science)
In software engineering, a design pattern is a general reusable solution to a commonly occurring problem within a given context in software design. A design pattern is not a finished design that can be transformed directly into code. It is a description or template for how to solve a problem that...
is where a number of threads
Thread (computer science)
In computer science, a thread of execution is the smallest unit of processing that can be scheduled by an operating system. The implementation of threads and processes differs from one operating system to another, but in most cases, a thread is contained inside a process...
are created to perform a number of tasks
Task (computers)
A task is an execution path through address space. In other words, a set of program instructions that are loaded in memory. The address registers have been loaded with the initial address of the program. At the next clock cycle, the CPU will start execution, in accord with the program. The sense is...
, which are usually organized in a queue. Typically, there are many more tasks than threads. As soon as a thread completes its task, it will request the next task from the queue until all tasks have been completed. The thread can then terminate, or sleep until there are new tasks available.
The number of threads used is a parameter that can be tuned to provide the best performance.
Additionally, the number of threads can be dynamic based on the number of waiting tasks.
For example, a web server
Web server
Web server can refer to either the hardware or the software that helps to deliver content that can be accessed through the Internet....
can add threads if numerous web page
Web page
A web page or webpage is a document or information resource that is suitable for the World Wide Web and can be accessed through a web browser and displayed on a monitor or mobile device. This information is usually in HTML or XHTML format, and may provide navigation to other web pages via hypertext...
requests come in and can remove threads when those requests taper down.
The cost of having a larger thread pool is increased resource usage.
The algorithm used to determine when to create or destroy threads will have an impact on the overall performance:
- create too many threads, and resources are wasted and time also wasted creating any unused threads
- destroy too many threads and more time will be spent later creating them again
- creating threads too slowly might result in poor client performance (long wait times)
- destroying threads too slowly may starve other processes of resources
The algorithm chosen will depend on the problem and the expected usage patterns.
If the number of tasks is very large, then creating a thread for each one may be impractical.
Another advantage of using a thread pool over creating a new thread for each task is thread creation and destruction overhead is negated, which may result in better performance
Performance tuning
Performance tuning is the improvement of system performance. This is typically a computer application, but the same methods can be applied to economic markets, bureaucracies or other complex systems. The motivation for such activity is called a performance problem, which can be real or anticipated....
and better system stability
Stability Model
Stability Model is a method of designing and modelling software. It is an extension of Object Oriented Software Design methodology, like UML, but adds its own set of rules, guidelines, procedures, and heuristics to achieve a more advanced Object Oriented software.The motivation is to achieve a...
. Creating and destroying a thread and its associated resources is an expensive process in terms of time. An excessive number of threads will also waste memory, and context-switching between the runnable threads also damages performance. For example, a socket connection to another machine—which might take thousands (or even millions) of cycles to drop and re-establish—can be avoided by associating it with a thread which lives over the course of more than one transaction.
When implementing this pattern, the programmer should ensure thread-safety
Thread-safe
Thread safety is a computer programming concept applicable in the context of multi-threaded programs. A piece of code is thread-safe if it only manipulates shared data structures in a thread-safe manner, which enables safe execution by multiple threads at the same time...
of the queue.
Typically, a thread pool executes on a single computer. However, thread pools are conceptually related to server farms
Server farm
A server farm or server cluster is a collection of computer servers usually maintained by an enterprise to accomplish server needs far beyond the capability of one machine. Server farms often have backup servers, which can take over the function of primary servers in the event of a primary server...
in which a master process distributes tasks to worker processes on different computers, in order to increase the overall throughput. Embarrassingly parallel
Embarrassingly parallel
In parallel computing, an embarrassingly parallel workload is one for which little or no effort is required to separate the problem into a number of parallel tasks...
problems are highly amenable to this approach.
See also
- Concurrency patternConcurrency patternIn software engineering, concurrency patterns are those types of design patterns that deal with multi-threaded programming paradigm.Examples of this class of patterns include:* Active Object* Balking pattern* Double checked locking pattern...
- Grand Central Dispatch
- Parallel Extensions for the .NET Framework.NET FrameworkThe .NET Framework is a software framework that runs primarily on Microsoft Windows. It includes a large library and supports several programming languages which allows language interoperability...
- Parallelization
- Server farmServer farmA server farm or server cluster is a collection of computer servers usually maintained by an enterprise to accomplish server needs far beyond the capability of one machine. Server farms often have backup servers, which can take over the function of primary servers in the event of a primary server...
External links
- Article "Query by Slice, Parallel Execute, and Join: A Thread Pool Pattern in Java" by Binildas C. A.
- Article "Thread pools and work queues" by Brian Goetz
- Article "A Method of Worker Thread Pooling" by Pradeep Kumar Sahu
- Article "Work Queue" by Uri Twig
- Article "Windows Thread Pooling and Execution Chaining"
- Article "Smart Thread Pool" by Ami Bar
- Article "Programming the Thread Pool in the .NET Framework" by David Carmona
- Article "The Thread Pool and Asynchronous Methods" by Jon Skeet
- Article "Creating a Notifying Blocking Thread Pool in Java" by Amir Kirsh
- Article "Practical Threaded Programming with Python: Thread Pools and Queues" by Noah Gift
- Paper "Optimizing Thread-Pool Strategies for Real-Time CORBA" by Irfan Pyarali, Marina Spivak, Douglas C. SchmidtDouglas C. SchmidtDouglas C. Schmidt is a computer scientist and author known for his works in the fields of object-oriented programming, distributed computing and design patterns. Currently he is working as Associate Chair of Computer Science and Engineering and Professor of Computer Science in Vanderbilt University...
and Ron Cytron - Conference Paper "Deferred cancellation. A behavioral pattern" by Philipp Bachmann