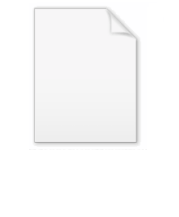
Procedural programming
Encyclopedia
Procedural programming can sometimes be used as a synonym for imperative programming
(specifying the steps the program must take to reach the desired state), but can also refer (as in this article) to a programming paradigm
, derived from structured programming
, based upon the concept of the procedure call. Procedures, also known as routines, subroutine
s, methods, or functions (not to be confused with mathematical functions, but similar to those used in functional programming
) simply contain a series of computational steps to be carried out. Any given procedure might be called at any point during a program's execution, including by other procedures or itself.
is generally desirable, especially in large, complicated programs. Inputs are usually specified syntactically in the form of arguments and the outputs delivered as return values.
Scoping is another technique that helps keep procedures strongly modular. It prevents the procedure from accessing the variables of other procedures (and vice-versa), including previous instances of itself, without explicit authorization.
Less modular procedures, often used in small or quickly written programs, tend to interact with a large number of variable
s in the execution environment, which other procedures might also modify.
Because of the ability to specify a simple interface, to be self-contained, and to be reused, procedures are a convenient vehicle for making pieces of code written by different people or different groups, including through programming libraries.
languages, because they make explicit references to the state of the execution environment. This could be anything from variables (which may correspond to processor register
s) to something like the position of the "turtle" in the Logo programming language.
, data structure
s, and subroutine
s, whereas in object-oriented programming
it is to break down a programming task into data types (classes
) that associate behavior (methods) with data (members or attributes). The most important distinction is whereas procedural programming uses procedures to operate on data structures, object-oriented programming bundles the two together so an "object", which is an instance of a class, operates on its "own" data structure.
Nomenclature varies between the two, although they have similar semantics:
See Algorithms + Data Structures = Programs
.
languages are fundamentally the same as in procedural languages, since they both stem from structured programming
. So for example:
The main difference between the styles is that functional programming languages remove or at least deemphasize the imperative elements of procedural programming. The feature set of functional languages is therefore designed to support writing programs as much as possible in terms of pure functions:
Many functional languages, however, are in fact impurely functional and offer imperative/procedural constructs that allow the programmer to write programs in procedural style, or in a combination of both styles. It is common for input/output
code in functional languages to be written in a procedural style.
There do exist a few esoteric
functional languages (like Unlambda
) that eschew structured programming
precepts for the sake of being difficult to program in (and therefore challenging). These languages are the exception to the common ground between procedural and functional languages.
, a program is a set of premises, and computation is performed by attempting to prove candidate theorems. From this point of view, logic programs are declarative
, focusing on what the problem is, rather than on how to solve it.
However, the backward reasoning technique, implemented by SLD resolution
, used to solve problems in logic programming languages such as Prolog
, treats programs as goal-reduction procedures. Thus clauses of the form:
have a dual interpretation, both as procedures
and as logical implications:
Experienced logic programmers use the procedural interpretation to write programs that are effective and efficient, and they use the declarative interpretation to help ensure that programs are correct.
Imperative programming
In computer science, imperative programming is a programming paradigm that describes computation in terms of statements that change a program state...
(specifying the steps the program must take to reach the desired state), but can also refer (as in this article) to a programming paradigm
Programming paradigm
A programming paradigm is a fundamental style of computer programming. Paradigms differ in the concepts and abstractions used to represent the elements of a program and the steps that compose a computation A programming paradigm is a fundamental style of computer programming. (Compare with a...
, derived from structured programming
Structured programming
Structured programming is a programming paradigm aimed on improving the clarity, quality, and development time of a computer program by making extensive use of subroutines, block structures and for and while loops - in contrast to using simple tests and jumps such as the goto statement which could...
, based upon the concept of the procedure call. Procedures, also known as routines, subroutine
Subroutine
In computer science, a subroutine is a portion of code within a larger program that performs a specific task and is relatively independent of the remaining code....
s, methods, or functions (not to be confused with mathematical functions, but similar to those used in functional programming
Functional programming
In computer science, functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids state and mutable data. It emphasizes the application of functions, in contrast to the imperative programming style, which emphasizes changes in state...
) simply contain a series of computational steps to be carried out. Any given procedure might be called at any point during a program's execution, including by other procedures or itself.
Procedures and modularity
ModularityModularity (programming)
Modular programming is a software design technique that increases the extent to which software is composed of separate, interchangeable components called modules by breaking down program functions into modules, each of which accomplishes one function and contains everything necessary to accomplish...
is generally desirable, especially in large, complicated programs. Inputs are usually specified syntactically in the form of arguments and the outputs delivered as return values.
Scoping is another technique that helps keep procedures strongly modular. It prevents the procedure from accessing the variables of other procedures (and vice-versa), including previous instances of itself, without explicit authorization.
Less modular procedures, often used in small or quickly written programs, tend to interact with a large number of variable
Variable (programming)
In computer programming, a variable is a symbolic name given to some known or unknown quantity or information, for the purpose of allowing the name to be used independently of the information it represents...
s in the execution environment, which other procedures might also modify.
Because of the ability to specify a simple interface, to be self-contained, and to be reused, procedures are a convenient vehicle for making pieces of code written by different people or different groups, including through programming libraries.
Comparison with imperative programming
Procedural programming languages are also imperativeImperative programming
In computer science, imperative programming is a programming paradigm that describes computation in terms of statements that change a program state...
languages, because they make explicit references to the state of the execution environment. This could be anything from variables (which may correspond to processor register
Processor register
In computer architecture, a processor register is a small amount of storage available as part of a CPU or other digital processor. Such registers are addressed by mechanisms other than main memory and can be accessed more quickly...
s) to something like the position of the "turtle" in the Logo programming language.
Comparison with object-oriented programming
The focus of procedural programming is to break down a programming task into a collection of variablesVariable (programming)
In computer programming, a variable is a symbolic name given to some known or unknown quantity or information, for the purpose of allowing the name to be used independently of the information it represents...
, data structure
Data structure
In computer science, a data structure is a particular way of storing and organizing data in a computer so that it can be used efficiently.Different kinds of data structures are suited to different kinds of applications, and some are highly specialized to specific tasks...
s, and subroutine
Subroutine
In computer science, a subroutine is a portion of code within a larger program that performs a specific task and is relatively independent of the remaining code....
s, whereas in object-oriented programming
Object-oriented programming
Object-oriented programming is a programming paradigm using "objects" – data structures consisting of data fields and methods together with their interactions – to design applications and computer programs. Programming techniques may include features such as data abstraction,...
it is to break down a programming task into data types (classes
Class (computer science)
In object-oriented programming, a class is a construct that is used as a blueprint to create instances of itself – referred to as class instances, class objects, instance objects or simply objects. A class defines constituent members which enable these class instances to have state and behavior...
) that associate behavior (methods) with data (members or attributes). The most important distinction is whereas procedural programming uses procedures to operate on data structures, object-oriented programming bundles the two together so an "object", which is an instance of a class, operates on its "own" data structure.
Nomenclature varies between the two, although they have similar semantics:
Procedural | Object-oriented |
---|---|
procedure | method |
record Record (computer science) In computer science, a record is an instance of a product of primitive data types called a tuple. In C it is the compound data in a struct. Records are among the simplest data structures. A record is a value that contains other values, typically in fixed number and sequence and typically indexed... |
object |
module | class |
procedure call | message |
See Algorithms + Data Structures = Programs
Algorithms + Data Structures = Programs
Algorithms + Data Structures = Programsis a 1976 book written by Niklaus Wirth covering some of the fundamental topics of computer programming, particularly that algorithms and data structures are inherently related...
.
Comparison with functional programming
The principles of modularity and code reuse in practical functionalFunctional programming
In computer science, functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids state and mutable data. It emphasizes the application of functions, in contrast to the imperative programming style, which emphasizes changes in state...
languages are fundamentally the same as in procedural languages, since they both stem from structured programming
Structured programming
Structured programming is a programming paradigm aimed on improving the clarity, quality, and development time of a computer program by making extensive use of subroutines, block structures and for and while loops - in contrast to using simple tests and jumps such as the goto statement which could...
. So for example:
- Procedures correspond to functions. Both allow the reuse of the same code in various parts of the programs, and at various points of its execution.
- By the same token, procedure calls correspond to function application.
- Functions and their invocations are modularly separated from each other in the same manner, by the use of function arguments, return values and variable scopes.
The main difference between the styles is that functional programming languages remove or at least deemphasize the imperative elements of procedural programming. The feature set of functional languages is therefore designed to support writing programs as much as possible in terms of pure functions:
- Whereas procedural languages model execution of the program as a sequence of imperative commands that may implicitly alter shared state, functional programming languages model execution as the evaluation of complex expressions that only depend on each other in terms of arguments and return values. For this reason, functional programs can have a freer order of code execution, and the languages may offer little control over the order in which various parts of the program are executed. (For example, the arguments to a procedure invocation in Scheme are executed in an arbitrary order.)
- Functional programming languages support (and heavily use) first-class functions, anonymous functions and closuresClosure (computer science)In computer science, a closure is a function together with a referencing environment for the non-local variables of that function. A closure allows a function to access variables outside its typical scope. Such a function is said to be "closed over" its free variables...
. - Functional programming languages tend to rely on tail call optimization and higher-order functions instead of imperative looping constructs.
Many functional languages, however, are in fact impurely functional and offer imperative/procedural constructs that allow the programmer to write programs in procedural style, or in a combination of both styles. It is common for input/output
Input/output
In computing, input/output, or I/O, refers to the communication between an information processing system , and the outside world, possibly a human, or another information processing system. Inputs are the signals or data received by the system, and outputs are the signals or data sent from it...
code in functional languages to be written in a procedural style.
There do exist a few esoteric
Esoteric programming language
An esoteric programming language is a programming language designed as a test of the boundaries of computer programming language design, as a proof of concept, or as a joke...
functional languages (like Unlambda
Unlambda
Unlambda is a minimal, "nearly pure" functional programming language invented by David Madore. It is based on combinatory logic, a version of the lambda calculus that omits the lambda operator. It relies mainly on two built-in functions and an "apply" operator...
) that eschew structured programming
Structured programming
Structured programming is a programming paradigm aimed on improving the clarity, quality, and development time of a computer program by making extensive use of subroutines, block structures and for and while loops - in contrast to using simple tests and jumps such as the goto statement which could...
precepts for the sake of being difficult to program in (and therefore challenging). These languages are the exception to the common ground between procedural and functional languages.
Comparison with logic programming
In logic programmingLogic programming
Logic programming is, in its broadest sense, the use of mathematical logic for computer programming. In this view of logic programming, which can be traced at least as far back as John McCarthy's [1958] advice-taker proposal, logic is used as a purely declarative representation language, and a...
, a program is a set of premises, and computation is performed by attempting to prove candidate theorems. From this point of view, logic programs are declarative
Declarative programming
In computer science, declarative programming is a programming paradigm that expresses the logic of a computation without describing its control flow. Many languages applying this style attempt to minimize or eliminate side effects by describing what the program should accomplish, rather than...
, focusing on what the problem is, rather than on how to solve it.
However, the backward reasoning technique, implemented by SLD resolution
SLD resolution
SLD resolution is the basic inference rule used in logic programming. It is a refinement of resolution, which is both sound and refutation complete for Horn clauses.-The SLD inference rule:...
, used to solve problems in logic programming languages such as Prolog
Prolog
Prolog is a general purpose logic programming language associated with artificial intelligence and computational linguistics.Prolog has its roots in first-order logic, a formal logic, and unlike many other programming languages, Prolog is declarative: the program logic is expressed in terms of...
, treats programs as goal-reduction procedures. Thus clauses of the form:
- H :- B1, …, Bn.
have a dual interpretation, both as procedures
- to show/solve H, show/solve B1 and … and Bn
and as logical implications:
- B1 and … and Bn implies H.
Experienced logic programmers use the procedural interpretation to write programs that are effective and efficient, and they use the declarative interpretation to help ensure that programs are correct.
See also
- Comparison of programming paradigmsComparison of programming paradigmsThis article attempts to set out the various similarities and differences between the various programming paradigms as a summary in both graphical and tabular format with links to the separate discussions concerning these similarities and differences in existing Wikipedia articles- Main paradigm...
- Declarative programmingDeclarative programmingIn computer science, declarative programming is a programming paradigm that expresses the logic of a computation without describing its control flow. Many languages applying this style attempt to minimize or eliminate side effects by describing what the program should accomplish, rather than...
- Functional programmingFunctional programmingIn computer science, functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids state and mutable data. It emphasizes the application of functions, in contrast to the imperative programming style, which emphasizes changes in state...
(contrast) - Imperative programmingImperative programmingIn computer science, imperative programming is a programming paradigm that describes computation in terms of statements that change a program state...
- Logic programmingLogic programmingLogic programming is, in its broadest sense, the use of mathematical logic for computer programming. In this view of logic programming, which can be traced at least as far back as John McCarthy's [1958] advice-taker proposal, logic is used as a purely declarative representation language, and a...
- Object-oriented programmingObject-oriented programmingObject-oriented programming is a programming paradigm using "objects" – data structures consisting of data fields and methods together with their interactions – to design applications and computer programs. Programming techniques may include features such as data abstraction,...
- Programming paradigmProgramming paradigmA programming paradigm is a fundamental style of computer programming. Paradigms differ in the concepts and abstractions used to represent the elements of a program and the steps that compose a computation A programming paradigm is a fundamental style of computer programming. (Compare with a...
s - Programming languageProgramming languageA programming language is an artificial language designed to communicate instructions to a machine, particularly a computer. Programming languages can be used to create programs that control the behavior of a machine and/or to express algorithms precisely....
- Structured programmingStructured programmingStructured programming is a programming paradigm aimed on improving the clarity, quality, and development time of a computer program by making extensive use of subroutines, block structures and for and while loops - in contrast to using simple tests and jumps such as the goto statement which could...