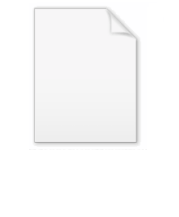
Nullable Types
Encyclopedia
In programming, nullable types are a feature of some statically-typed
programming languages which allows a data type
to be set to the special value NULL instead of their common range of possible values.
For value types or built-in data types like integer
s and boolean
s however, such behavior is mostly not possible. Nullable type support allows for the programmer to make also these value types NULL. This can be useful in general and also when working with databases. A field in a Relational database
like SQL
may have an entry that is NULL
(or empty) instead of containing a value. A language with nullable type support can then return a NULL value and correctly represent the behavior of the database.
( ..., -2, -1, 0, 1, 2, ...). The value 0 (zero) however is not the correct representation for this variable to contain nothing (it contains the value 0 and only the conception in the given context might suggest that 0 represents "nothing" instead of the actual representation: "nothing" of "something"). In many circumstances it is required to represent also the fact that a variable has not been given any value at all. This can be achieved with a Nullable Type. In programming languages like C# 2.0 a Nullable integer for example can be declared by a question mark (int? x). In programming languages like C# 1.0 Nullable Types can be defined by an external library as new types (e.g. NullableInteger, NullableBoolean).
A boolean variable makes the effect even more clear. Its values can be either "true" or "false", while a nullable boolean may also contain a representation for "undecided". However, the interpretation or treatment of a logical operation involving such a variable depends on the language.
Nullable references were invented by C.A.R. Hoare in 1965 as part of the Algol W
language. Hoare later described their invention as a "billion dollar mistake". This is because object pointers that can be NULL require the user to check the pointer before using it and require specific code to handle the case when the object pointer is NULL. In some languages, like Objective-C
, however, NULL object pointers can be used without problems.
.
There is a more general and formal concept that extend the nullable type concept, it comes from option type
s, which enforce explicit handling of the exceptional case.
Option type implementations usually adhere to the Special Case pattern
Type system
A type system associates a type with each computed value. By examining the flow of these values, a type system attempts to ensure or prove that no type errors can occur...
programming languages which allows a data type
Data type
In computer programming, a data type is a classification identifying one of various types of data, such as floating-point, integer, or Boolean, that determines the possible values for that type; the operations that can be done on values of that type; the meaning of the data; and the way values of...
to be set to the special value NULL instead of their common range of possible values.
For value types or built-in data types like integer
Integer
The integers are formed by the natural numbers together with the negatives of the non-zero natural numbers .They are known as Positive and Negative Integers respectively...
s and boolean
Boolean datatype
In computer science, the Boolean or logical data type is a data type, having two values , intended to represent the truth values of logic and Boolean algebra...
s however, such behavior is mostly not possible. Nullable type support allows for the programmer to make also these value types NULL. This can be useful in general and also when working with databases. A field in a Relational database
Relational database
A relational database is a database that conforms to relational model theory. The software used in a relational database is called a relational database management system . Colloquial use of the term "relational database" may refer to the RDBMS software, or the relational database itself...
like SQL
SQL
SQL is a programming language designed for managing data in relational database management systems ....
may have an entry that is NULL
Null (SQL)
Null is a special marker used in Structured Query Language to indicate that a data value does not exist in the database. Introduced by the creator of the relational database model, E. F. Codd, SQL Null serves to fulfill the requirement that all true relational database management systems support...
(or empty) instead of containing a value. A language with nullable type support can then return a NULL value and correctly represent the behavior of the database.
Example
An integer variable may contain a positive or negative natural numberNatural number
In mathematics, the natural numbers are the ordinary whole numbers used for counting and ordering . These purposes are related to the linguistic notions of cardinal and ordinal numbers, respectively...
( ..., -2, -1, 0, 1, 2, ...). The value 0 (zero) however is not the correct representation for this variable to contain nothing (it contains the value 0 and only the conception in the given context might suggest that 0 represents "nothing" instead of the actual representation: "nothing" of "something"). In many circumstances it is required to represent also the fact that a variable has not been given any value at all. This can be achieved with a Nullable Type. In programming languages like C# 2.0 a Nullable integer for example can be declared by a question mark (int? x). In programming languages like C# 1.0 Nullable Types can be defined by an external library as new types (e.g. NullableInteger, NullableBoolean).
A boolean variable makes the effect even more clear. Its values can be either "true" or "false", while a nullable boolean may also contain a representation for "undecided". However, the interpretation or treatment of a logical operation involving such a variable depends on the language.
Compared with null pointers
In contrast, object pointers can be set to NULL by default in most common languages, meaning that the pointer or reference points to nowhere, that no object is assigned (the variable does not point to any object).Nullable references were invented by C.A.R. Hoare in 1965 as part of the Algol W
ALGOL W
ALGOL W is a programming language. It was based on a proposal for ALGOL X by Niklaus Wirth and C. A. R. Hoare as a successor to ALGOL 60 in IFIP Working Group 2.1. When the committee decided that the proposal was not a sufficient advance over ALGOL 60, the proposal was published as A contribution...
language. Hoare later described their invention as a "billion dollar mistake". This is because object pointers that can be NULL require the user to check the pointer before using it and require specific code to handle the case when the object pointer is NULL. In some languages, like Objective-C
Objective-C
Objective-C is a reflective, object-oriented programming language that adds Smalltalk-style messaging to the C programming language.Today, it is used primarily on Apple's Mac OS X and iOS: two environments derived from the OpenStep standard, though not compliant with it...
, however, NULL object pointers can be used without problems.
Compared with option types
Nullable type implementations usually adhere to the null object patternNull Object pattern
In object-oriented computer programming, a Null Object is an object with defined neutral behavior. The Null Object design pattern describes the uses of such objects and their behavior . It was first published in the Pattern Languages of Program Design book series.-Motivation:In most...
.
There is a more general and formal concept that extend the nullable type concept, it comes from option type
Option type
In programming languages and type theory, an option type or maybe type is a polymorphic type that represents encapsulation of an optional value; e.g. it is used as the return type of functions which may or may not return a meaningful value when they're applied...
s, which enforce explicit handling of the exceptional case.
Option type implementations usually adhere to the Special Case pattern
Language support
The following programming languages support nullable types:- JavaJava (programming language)Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities...
- Oxygene
- .NET Framework.NET FrameworkThe .NET Framework is a software framework that runs primarily on Microsoft Windows. It includes a large library and supports several programming languages which allows language interoperability...
version 1.0 languages with the external NullableTypes library
This implementation adheres to the Null Object patternNull Object patternIn object-oriented computer programming, a Null Object is an object with defined neutral behavior. The Null Object design pattern describes the uses of such objects and their behavior . It was first published in the Pattern Languages of Program Design book series.-Motivation:In most...
, adheres to the NULL (SQL)Null (SQL)Null is a special marker used in Structured Query Language to indicate that a data value does not exist in the database. Introduced by the creator of the relational database model, E. F. Codd, SQL Null serves to fulfill the requirement that all true relational database management systems support...
Three-valued logic (3VL), includes all the .NET built-in types, requires programmers to implement the nullable behavior for a custom value-type. - .NET Framework.NET FrameworkThe .NET Framework is a software framework that runs primarily on Microsoft Windows. It includes a large library and supports several programming languages which allows language interoperability...
version 2.0 languages
This implementation violates the Null Object patternNull Object patternIn object-oriented computer programming, a Null Object is an object with defined neutral behavior. The Null Object design pattern describes the uses of such objects and their behavior . It was first published in the Pattern Languages of Program Design book series.-Motivation:In most...
, violates the NULL (SQL)Null (SQL)Null is a special marker used in Structured Query Language to indicate that a data value does not exist in the database. Introduced by the creator of the relational database model, E. F. Codd, SQL Null serves to fulfill the requirement that all true relational database management systems support...
Three-valued logic (3VL), makes use of the .NET 2.0 Generics so it includes all the .NET built-in types and can easily extends a custom value-type - PerlPerlPerl is a high-level, general-purpose, interpreted, dynamic programming language. Perl was originally developed by Larry Wall in 1987 as a general-purpose Unix scripting language to make report processing easier. Since then, it has undergone many changes and revisions and become widely popular...
scalar variables default toundef
and can be set toundef
. Similarly, list and hash variables can beor
{}
respectively.