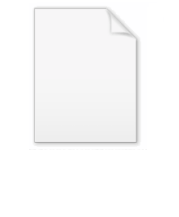
Multiple inheritance
Encyclopedia
Multiple inheritance is a feature of some object-oriented
computer programming language
s in which a class
can inherit
behaviors and features from more than one superclass.
Languages that support multiple inheritance include: C++
, Common Lisp
(via CLOS), EuLisp
(via The EuLisp Object System TELOS), Curl, Dylan, Eiffel
, Logtalk
, Object REXX
, Scala (via the use of mixin classes
), OCaml, Perl
, Perl 6
, Python
, and Tcl
(via Incremental Tcl
).
Other object-oriented languages, such as Java
and Ruby
implement single inheritance, although protocols
, or "interfaces," provide some of the functionality of true multiple inheritance.
describes a relationship between two types, or classes, of objects in which one is said to be a "subtype" or "child" of the other. The child inherits features of the parent, allowing for shared functionality. For example, one might create a variable class "Mammal" with features such as eating, reproducing, etc.; then define a subtype "Cat" that inherits those features without having to explicitly program them, while adding new features like "chasing mice".
If, however, one wants to use more than one totally orthogonal hierarchy simultaneously, such as allowing "Cat" to inherit from "Cartoon character" and "Pet" as well as "Mammal", lack of multiple inheritance often results in a very awkwardly mixed hierarchy, or forces functionality to be rewritten in more than one place (with the attendant maintenance problems).
Multiple inheritance has been a touchy issue for many years, with opponents pointing to its increased complexity and ambiguity in situations such as the diamond problem
.
Languages have different ways of dealing with these problems of repeated inheritance.
, called interfaces
in Java. These protocols define methods but do not provide concrete implementations. This strategy has been used by
C#, D
, Object Pascal / Delphi
, Java
, ActionScript
, Nemerle, Objective-C
, Ruby
, Smalltalk
and PHP
. All but Smalltalk allow classes to implement multiple protocols.
, for example has no multiple inheritance, as its designers felt that it would add unnecessary complexity.
Multiple inheritance in languages with C++-/Java-style constructors exacerbates the inheritance problem of constructors and constructor chaining, thereby creating maintenance and extensibility problems in these languages. Objects in inheritance relationship with greatly varying construction methods are hard to implement under the constructor-chaining paradigm.
Criticisms for the problems that it causes in certain languages, in particular C++, are:
Object-oriented programming
Object-oriented programming is a programming paradigm using "objects" – data structures consisting of data fields and methods together with their interactions – to design applications and computer programs. Programming techniques may include features such as data abstraction,...
computer programming language
Programming language
A programming language is an artificial language designed to communicate instructions to a machine, particularly a computer. Programming languages can be used to create programs that control the behavior of a machine and/or to express algorithms precisely....
s in which a class
Class (computer science)
In object-oriented programming, a class is a construct that is used as a blueprint to create instances of itself – referred to as class instances, class objects, instance objects or simply objects. A class defines constituent members which enable these class instances to have state and behavior...
can inherit
Inheritance (computer science)
In object-oriented programming , inheritance is a way to reuse code of existing objects, establish a subtype from an existing object, or both, depending upon programming language support...
behaviors and features from more than one superclass.
Languages that support multiple inheritance include: C++
C++
C++ is a statically typed, free-form, multi-paradigm, compiled, general-purpose programming language. It is regarded as an intermediate-level language, as it comprises a combination of both high-level and low-level language features. It was developed by Bjarne Stroustrup starting in 1979 at Bell...
, Common Lisp
Common Lisp
Common Lisp, commonly abbreviated CL, is a dialect of the Lisp programming language, published in ANSI standard document ANSI INCITS 226-1994 , . From the ANSI Common Lisp standard the Common Lisp HyperSpec has been derived for use with web browsers...
(via CLOS), EuLisp
EuLisp
EuLisp is a statically and dynamically scoped Lisp dialect developed by a loose formation of industrial and academic Lisp users and developers from around Europe. The standardizers intended to create a new Lisp "less encumbered by the past" , and not so minimalistic as Scheme...
(via The EuLisp Object System TELOS), Curl, Dylan, Eiffel
Eiffel (programming language)
Eiffel is an ISO-standardized, object-oriented programming language designed by Bertrand Meyer and Eiffel Software. The design of the language is closely connected with the Eiffel programming method...
, Logtalk
Logtalk
Logtalk is an object-oriented logic programming language that extends the Prolog language with a feature set suitable for programming in the large. It provides support for encapsulation and data hiding, separation of concerns and enhanced code reuse...
, Object REXX
Object REXX
The Object REXX programming language is an object-oriented scripting language initially produced by IBM for OS/2. It is a follow-on to and a significant extension of the "Classic Rexx" language originally created for the CMS component of VM/SP and later ported to MVS, OS/2 and PC DOS.On October 12,...
, Scala (via the use of mixin classes
Mixin
In object-oriented programming languages, a mixin is a class that provides a certain functionality to be inherited or just reused by a subclass, while not meant for instantiation , Mixins are synonymous functionally with abstract base classes...
), OCaml, Perl
Perl
Perl is a high-level, general-purpose, interpreted, dynamic programming language. Perl was originally developed by Larry Wall in 1987 as a general-purpose Unix scripting language to make report processing easier. Since then, it has undergone many changes and revisions and become widely popular...
, Perl 6
Perl 6
Perl 6 is a major revision to the Perl programming language. It is still in development, as a specification from which several interpreter and compiler implementations are being written. It is introducing elements of many modern and historical languages. Perl 6 is intended to have many...
, Python
Python (programming language)
Python is a general-purpose, high-level programming language whose design philosophy emphasizes code readability. Python claims to "[combine] remarkable power with very clear syntax", and its standard library is large and comprehensive...
, and Tcl
Tcl
Tcl is a scripting language created by John Ousterhout. Originally "born out of frustration", according to the author, with programmers devising their own languages intended to be embedded into applications, Tcl gained acceptance on its own...
(via Incremental Tcl
Itcl
incr Tcl is a set of object-oriented extensions for the Tcl programming language. It is widely used among the Tcl community, and is generally regarded as industrial strength...
).
Other object-oriented languages, such as Java
Java (programming language)
Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities...
and Ruby
Ruby (programming language)
Ruby is a dynamic, reflective, general-purpose object-oriented programming language that combines syntax inspired by Perl with Smalltalk-like features. Ruby originated in Japan during the mid-1990s and was first developed and designed by Yukihiro "Matz" Matsumoto...
implement single inheritance, although protocols
Protocol (object-oriented programming)
In object-oriented programming, a protocol or interface is a common means for unrelated objects to communicate with each other. These are definitions of methods and values which the objects agree upon in order to cooperate....
, or "interfaces," provide some of the functionality of true multiple inheritance.
Overview
In object-oriented programming (OOP), inheritanceInheritance (object-oriented programming)
In object-oriented programming , inheritance is a way to reuse code of existing objects, establish a subtype from an existing object, or both, depending upon programming language support...
describes a relationship between two types, or classes, of objects in which one is said to be a "subtype" or "child" of the other. The child inherits features of the parent, allowing for shared functionality. For example, one might create a variable class "Mammal" with features such as eating, reproducing, etc.; then define a subtype "Cat" that inherits those features without having to explicitly program them, while adding new features like "chasing mice".
If, however, one wants to use more than one totally orthogonal hierarchy simultaneously, such as allowing "Cat" to inherit from "Cartoon character" and "Pet" as well as "Mammal", lack of multiple inheritance often results in a very awkwardly mixed hierarchy, or forces functionality to be rewritten in more than one place (with the attendant maintenance problems).
Multiple inheritance has been a touchy issue for many years, with opponents pointing to its increased complexity and ambiguity in situations such as the diamond problem
Diamond problem
In object-oriented programming languages with multiple inheritance and knowledge organization, the diamond problem is an ambiguity that arises when two classes B and C inherit from A, and class D inherits from both B and C...
.
Languages have different ways of dealing with these problems of repeated inheritance.
- C++C++C++ is a statically typed, free-form, multi-paradigm, compiled, general-purpose programming language. It is regarded as an intermediate-level language, as it comprises a combination of both high-level and low-level language features. It was developed by Bjarne Stroustrup starting in 1979 at Bell...
requires the programmer to state which parent class the feature to be used is invoked from i.e. "Worker::Human.Age". C++ does not support explicit repeated inheritance since there would be no way to qualify which superclass to use (see criticisms). C++ also allows a single instance of the multiple class to be created via the virtual inheritance mechanism (i.e. "Worker::Human" and "Musician::Human" will reference the same object). - The Common Lisp Object System allows full programmer control of method combination, and if this is not enough, the Metaobject ProtocolMetaobjectIn computer science, a metaobject or meta-object is any entity that manipulates, creates, describes, or implements other objects. The object that the metaobject is about is called the base object...
gives the programmer a means to modify the inheritance, method dispatch, class instantiation, and other internal mechanisms without affecting the stability of the system. - Curl allows only classes that are explicitly marked as shared to be inherited repeatedly. Shared classes must define a secondary constructor for each regular constructorConstructor (computer science)In object-oriented programming, a constructor in a class is a special type of subroutine called at the creation of an object. It prepares the new object for use, often accepting parameters which the constructor uses to set any member variables required when the object is first created...
in the class. The regular constructor is called the first time the state for the shared class is initialized through a subclass constructor, and the secondary constructor will be invoked for all other subclasses. - EiffelEiffel (programming language)Eiffel is an ISO-standardized, object-oriented programming language designed by Bertrand Meyer and Eiffel Software. The design of the language is closely connected with the Eiffel programming method...
allows the programmer to explicitly join or separate features that are being inherited from superclasses. Eiffel will automatically join features together, if they have the same name and implementation. The class writer has the option to rename the inherited features to separate them. Eiffel also allows explicit repeated inheritance such as A: B, B. - LogtalkLogtalkLogtalk is an object-oriented logic programming language that extends the Prolog language with a feature set suitable for programming in the large. It provides support for encapsulation and data hiding, separation of concerns and enhanced code reuse...
supports both interface and implementation multi-inheritance, allowing the declaration of method aliases that provide both renaming and access to methods that would be masked out by the default conflict resolution mechanism. - Ocaml chooses the last matching definition of a class inheritance list to resolve which method implementation to use under ambiguities. To override the default behavior, one simply qualifies a method call with the desired class definition.
- PerlPerlPerl is a high-level, general-purpose, interpreted, dynamic programming language. Perl was originally developed by Larry Wall in 1987 as a general-purpose Unix scripting language to make report processing easier. Since then, it has undergone many changes and revisions and become widely popular...
uses the list of classes to inherit from as an ordered list. The compiler uses the first method it finds by depth-first searchDepth-first searchDepth-first search is an algorithm for traversing or searching a tree, tree structure, or graph. One starts at the root and explores as far as possible along each branch before backtracking....
ing of the superclass list or using the C3 linearizationC3 linearizationIn computing, the C3 superclass linearization is an algorithm used primarily to obtain a consistent linearization of a multiple inheritance hierarchy in object-oriented programming. This linearization is used to resolve the order in which methods should be inherited, and is often termed "MRO" for...
of the class hierarchy. Various extensions provide alternative class composition schemes. The order of inheritance affects the class semantics (see criticisms). - PythonPython (programming language)Python is a general-purpose, high-level programming language whose design philosophy emphasizes code readability. Python claims to "[combine] remarkable power with very clear syntax", and its standard library is large and comprehensive...
has the same structure as Perl, but unlike Perl includes it in the syntax of the language. The order of inheritance affects the class semantics (see criticisms). - Scala allows multiple instantiation of traits, which allows for multiple inheritance by adding a distinction between the class hierarchy and the trait hierarchy. A class can only inherit from a single class, but can mix-in as many traits as desired.
- TclTclTcl is a scripting language created by John Ousterhout. Originally "born out of frustration", according to the author, with programmers devising their own languages intended to be embedded into applications, Tcl gained acceptance on its own...
allows multiple parent classes- their serial affects the name resolution for class members.
Single inheritance
One way of resolving these problems has been to enforce single inheritance where a class can only derive from one base class. Typically these languages allow classes to implement multiple protocolsProtocol (object-oriented programming)
In object-oriented programming, a protocol or interface is a common means for unrelated objects to communicate with each other. These are definitions of methods and values which the objects agree upon in order to cooperate....
, called interfaces
Interface (Java)
An interface in the Java programming language is an abstract type that is used to specify an interface that classes must implement. Interfaces are declared using the interface keyword, and may only contain method signature and constant declarations...
in Java. These protocols define methods but do not provide concrete implementations. This strategy has been used by
C#, D
D (programming language)
The D programming language is an object-oriented, imperative, multi-paradigm, system programming language created by Walter Bright of Digital Mars. It originated as a re-engineering of C++, but even though it is mainly influenced by that language, it is not a variant of C++...
, Object Pascal / Delphi
Object Pascal
Object Pascal refers to a branch of object-oriented derivatives of Pascal, mostly known as the primary programming language of Embarcadero Delphi.-Early history at Apple:...
, Java
Java (programming language)
Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities...
, ActionScript
ActionScript
ActionScript is an object-oriented language originally developed by Macromedia Inc. . It is a dialect of ECMAScript , and is used primarily for the development of websites and software targeting the Adobe Flash Player platform, used on Web pages in the form of...
, Nemerle, Objective-C
Objective-C
Objective-C is a reflective, object-oriented programming language that adds Smalltalk-style messaging to the C programming language.Today, it is used primarily on Apple's Mac OS X and iOS: two environments derived from the OpenStep standard, though not compliant with it...
, Ruby
Ruby (programming language)
Ruby is a dynamic, reflective, general-purpose object-oriented programming language that combines syntax inspired by Perl with Smalltalk-like features. Ruby originated in Japan during the mid-1990s and was first developed and designed by Yukihiro "Matz" Matsumoto...
, Smalltalk
Smalltalk
Smalltalk is an object-oriented, dynamically typed, reflective programming language. Smalltalk was created as the language to underpin the "new world" of computing exemplified by "human–computer symbiosis." It was designed and created in part for educational use, more so for constructionist...
and PHP
PHP
PHP is a general-purpose server-side scripting language originally designed for web development to produce dynamic web pages. For this purpose, PHP code is embedded into the HTML source document and interpreted by a web server with a PHP processor module, which generates the web page document...
. All but Smalltalk allow classes to implement multiple protocols.
Criticisms
Multiple inheritance has been criticized by some for its unnecessary complexity and being difficult to implement efficiently, though some projects have certainly benefited from its use. JavaJava (programming language)
Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities...
, for example has no multiple inheritance, as its designers felt that it would add unnecessary complexity.
Multiple inheritance in languages with C++-/Java-style constructors exacerbates the inheritance problem of constructors and constructor chaining, thereby creating maintenance and extensibility problems in these languages. Objects in inheritance relationship with greatly varying construction methods are hard to implement under the constructor-chaining paradigm.
Criticisms for the problems that it causes in certain languages, in particular C++, are:
- Semantic ambiguity often summarized as the diamond problemDiamond problemIn object-oriented programming languages with multiple inheritance and knowledge organization, the diamond problem is an ambiguity that arises when two classes B and C inherit from A, and class D inherits from both B and C...
(although solvable by using virtual inheritanceVirtual inheritanceVirtual inheritance is a topic of object-oriented programming. It is a kind of inheritance in which the part of the object that belongs to the virtual base class becomes common direct base for the derived class and any next class that derives from it...
or 'using' declarations). - Not being able to explicitly inherit multiple times from a single class (on the other hand this feature is criticised as non-object-oriented).
- Order of inheritance changing class semantics (although it's the same with order of field declarations).
See also
- Implementation inheritance
- Virtual inheritanceVirtual inheritanceVirtual inheritance is a topic of object-oriented programming. It is a kind of inheritance in which the part of the object that belongs to the virtual base class becomes common direct base for the derived class and any next class that derives from it...
- MixinMixinIn object-oriented programming languages, a mixin is a class that provides a certain functionality to be inherited or just reused by a subclass, while not meant for instantiation , Mixins are synonymous functionally with abstract base classes...
- C3 linearizationC3 linearizationIn computing, the C3 superclass linearization is an algorithm used primarily to obtain a consistent linearization of a multiple inheritance hierarchy in object-oriented programming. This linearization is used to resolve the order in which methods should be inherited, and is often termed "MRO" for...
Further reading
- Stroustrup, Bjarne (1999). Multiple Inheritance for C++. Proceedings of the Spring 1987 European Unix Users Group Conference.
- Object-Oriented Software Construction, Second Edition, by Bertrand MeyerBertrand MeyerBertrand Meyer is an academic, author, and consultant in the field of computer languages. He created the Eiffel programming language.-Education and academic career:...
, Prentice Hall, 1997, ISBN 0-13-629155-4