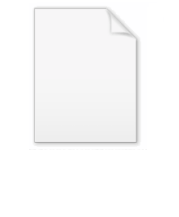
Iostream
Encyclopedia
iostream
is a header fileHeader file
Some programming languages use header files. These files allow programmers to separate certain elements of a program's source code into reusable files. Header files commonly contain forward declarations of classes, subroutines, variables, and other identifiers...
which is used for input/output
Input/output
In computing, input/output, or I/O, refers to the communication between an information processing system , and the outside world, possibly a human, or another information processing system. Inputs are the signals or data received by the system, and outputs are the signals or data sent from it...
in the C++
C++
C++ is a statically typed, free-form, multi-paradigm, compiled, general-purpose programming language. It is regarded as an intermediate-level language, as it comprises a combination of both high-level and low-level language features. It was developed by Bjarne Stroustrup starting in 1979 at Bell...
programming language. It is part of the C++ standard library
C++ standard library
In C++, the C++ Standard Library is a collection of classes and functions, which are written in the core language and part of the C++ ISO Standard itself...
. The name stands for Input/Output Stream. In C++ and its predecessor, the C programming language
C (programming language)
C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system....
, there is no special syntax for streaming data input or output. Instead, these are combined as a library
Library (computer science)
In computer science, a library is a collection of resources used to develop software. These may include pre-written code and subroutines, classes, values or type specifications....
of functions
Subroutine
In computer science, a subroutine is a portion of code within a larger program that performs a specific task and is relatively independent of the remaining code....
. Like the
cstdio
header inherited from C's stdio.h, iostream
provides basic input and output services for C++ programs. iostream uses the objectObject (computer science)
In computer science, an object is any entity that can be manipulated by the commands of a programming language, such as a value, variable, function, or data structure...
s
cin
, cout
, cerr
, and clog
for sending data to and from the standard streamsStandard streams
In Unix and Unix-like operating systems , as well as certain programming language interfaces, the standard streams are preconnected input and output channels between a computer program and its environment when it begins execution...
input, output, error (unbuffered), and error (buffered) respectively. As part of the C++ standard library
C++ standard library
In C++, the C++ Standard Library is a collection of classes and functions, which are written in the core language and part of the C++ ISO Standard itself...
, these objects are a part of the
std
namespaceNamespace
In general, a namespace is a container that provides context for the identifiers it holds, and allows the disambiguation of homonym identifiers residing in different namespaces....
.
Example usage
The canonical Hello world programHello world program
A "Hello world" program is a computer program that outputs "Hello world" on a display device. Because it is typically one of the simplest programs possible in most programming languages, it is by tradition often used to illustrate to beginners the most basic syntax of a programming language, or to...
can be expressed as follows:
- include
int main
{
std::cout << "Hello,world!\n";
return 0;
}
This program would output "Hello, world!" followed by a newline
Newline
In computing, a newline, also known as a line break or end-of-line marker, is a special character or sequence of characters signifying the end of a line of text. The name comes from the fact that the next character after the newline will appear on a new line—that is, on the next line below the...
and standard output stream buffer flush.
The
cout
object is of type ostream
, which overloadsOperator overloading
In object oriented computer programming, operator overloading—less commonly known as operator ad-hoc polymorphism—is a specific case of polymorphism, where different operators have different implementations depending on their arguments...
the left bit-shift operator
Operator (programming)
Programming languages typically support a set of operators: operations which differ from the language's functions in calling syntax and/or argument passing mode. Common examples that differ by syntax are mathematical arithmetic operations, e.g...
to make it perform an operation completely unrelated to bitwise operations. The
cerr
and clog
objects are also of type ostream
, so they overload that operator as well. The cin
object is of type istream
, which overloads the right bit-shift operator. The directions of the bit-shift operators make it seem as though data is flowing towards the output stream or flowing away from the input stream.An alternative to the newline character
\n
is endl
, which is used as follows:- include
int main
{
std::cout << "Hello, world!" << std::endl;
return 0;
}
endl
is an output manipulator that writes a newline and flushes the buffer, ensuring that the data is output immediately. Several other manipulators are listed below.Methods
width(int x) |
minimum number of characters for next output |
fill(char x) |
character used to fill with in the case that the width needs to be elongated to fill the minimum. |
precision(int x) |
sets the number of significant digits for floating-point numbers |
Example:
using namespace std;
cout.width(10);
cout << "ten" << "four" << "four";
Manipulators
Manipulators are objects that can modify a stream using the<<
or >>
operators.endl |
"end line": inserts a newline into the stream and calls flush. |
ends |
"end string": inserts a null character into the stream and calls flush. |
flush |
forces an output stream to write any buffered characters |
dec |
changes the output format of number to be in decimal format |
oct |
changes the output format of number to be in octal format |
hex |
changes the output format of number to be in hexadecimal format |
ws |
causes an inputstream to 'eat' whitespace |
showpoint |
tells the stream to show the decimal point and some zeros with whole numbers |
Other manipulators can be found using the header
iomanip
.Criticism
Some environments do not provide a shared implementation of the C++ library. These include embedded systemEmbedded system
An embedded system is a computer system designed for specific control functions within a larger system. often with real-time computing constraints. It is embedded as part of a complete device often including hardware and mechanical parts. By contrast, a general-purpose computer, such as a personal...
s and Windows systems running programs built with MinGW
MinGW
MinGW , formerly mingw32, is a native software port of the GNU Compiler Collection and GNU Binutils for use in the development of native Microsoft Windows applications; MinGW can function either as a cross compiler targeting Windows or as a native toolchain run on Windows itself...
. Under these systems, the C++ standard library must be statically linked
Static library
In computer science, a static library or statically-linked library is a set of routines, external functions and variables which are resolved in a caller at compile-time and copied into a target application by a compiler, linker, or binder, producing an object file and a stand-alone executable...
to a program, which increases the size of the program, or distributed as a shared library alongside the program.
Some implementations of the C++ standard library have significant amounts of dead code
Dead code
Dead code is a computer programming term for code in the source code of a program which is executed but whose result is never used in any other computation...
. For example, GNU libstdc++ automatically constructs
Constructor (computer science)
In object-oriented programming, a constructor in a class is a special type of subroutine called at the creation of an object. It prepares the new object for use, often accepting parameters which the constructor uses to set any member variables required when the object is first created...
a locale
Locale
In computing, locale is a set of parameters that defines the user's language, country and any special variant preferences that the user wants to see in their user interface...
when building an
ostream
even if a program never uses any types (date, time or money) that a locale affects,and a statically-linked hello world program
Hello world program
A "Hello world" program is a computer program that outputs "Hello world" on a display device. Because it is typically one of the simplest programs possible in most programming languages, it is by tradition often used to illustrate to beginners the most basic syntax of a programming language, or to...
that uses
<iostream>
of GNU libstdc++ produces an executable an order of magnitudeOrder of magnitude
An order of magnitude is the class of scale or magnitude of any amount, where each class contains values of a fixed ratio to the class preceding it. In its most common usage, the amount being scaled is 10 and the scale is the exponent being applied to this amount...
larger than an equivalent program that uses
<cstdio>
.There exist partial implementations of the C++ standard library designed for space-constrained environments; their
<iostream>
may leave out features that programs in such environments may not need, such as locale support.