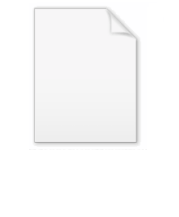
Inner class
Encyclopedia
In object-oriented programming
(OOP), an inner class or nested class is a class
declared entirely within the body of another class or interface. It is distinguished from a subclass.
Let us take the abstract notion of a
We have the top-level class
Inner classes provide us with a mechanism to accurately model this connection. We say that our
Inner classes therefore allow for the object orientation of certain parts of the program that would otherwise not be encapsulated into a class.
Larger segments of code within a class might be better modeled or refactored as a separate top-level class, rather than an inner class. This would make the code more general in its application and therefore more re-usable but potentially might be premature generalization. This may prove more effective, if code has many inner classes with the shared functionality.
there are four types of nested class:
Static
Non-Static / Inner Classes
Inner class - The following categories are called inner classes. Each instance of these classes has a reference to an enclosing instance (i.e. an instance of the enclosing class), except for local and anonymous classes declared in static context. Hence, they can implicitly refer to instance variables and methods of the enclosing class. The enclosing instance reference can be explicitly obtained via
Anonymous inner classes are also used where the event handling code is only used by one component and therefore does not need a named reference.
This avoids a large monolithic
method with multiple if-else branches to identify the source of the event. This type of code is often considered messy and the inner class variations are considered to be better in all regards.
Object-oriented programming
Object-oriented programming is a programming paradigm using "objects" – data structures consisting of data fields and methods together with their interactions – to design applications and computer programs. Programming techniques may include features such as data abstraction,...
(OOP), an inner class or nested class is a class
Class (computer science)
In object-oriented programming, a class is a construct that is used as a blueprint to create instances of itself – referred to as class instances, class objects, instance objects or simply objects. A class defines constituent members which enable these class instances to have state and behavior...
declared entirely within the body of another class or interface. It is distinguished from a subclass.
Overview
An instance of a normal or top-level class can exist on its own. By contrast, an instance of an inner class cannot be instantiated without being bound to a top-level class.Let us take the abstract notion of a
Car
with four Wheel
s. Our Wheel
s have a specific feature that relies on being part of our Car
. This notion does not represent the Wheel
s as Wheel
s in a more general form that could be part of a vehicle. Instead, it represents them as specific to this one. We can model this notion using inner classes as follows:We have the top-level class
Car
. Instances of Class Car
are composed of four instances of the class Wheel
. This particular implementation of Wheel
is specific to the car, so the code does not model the general notion of a wheel that would be better represented as a top-level class. Therefore, it is semantically connected to the class Car
and the code of Wheel
is in some way coupled to its outer class.Inner classes provide us with a mechanism to accurately model this connection. We say that our
Wheel
class is Car.Wheel
, Car
being the top-level class and Wheel
being the inner class.Inner classes therefore allow for the object orientation of certain parts of the program that would otherwise not be encapsulated into a class.
Larger segments of code within a class might be better modeled or refactored as a separate top-level class, rather than an inner class. This would make the code more general in its application and therefore more re-usable but potentially might be premature generalization. This may prove more effective, if code has many inner classes with the shared functionality.
Types of inner classes
In JavaJava (programming language)
Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities...
there are four types of nested class:
Static
- Static member class, also called static nested classes - They are declared
static
. Like other things in static scope (i.e. static methods), they do not have an enclosing instance, and cannot access instance variableInstance variableIn object-oriented programming with classes, an instance variable is a variable defined in a class , for which each object of the class has a separate copy. They live in memory for the life of the object....
s and methods of the enclosing class. They are almost identical to non-nested classes except for scope details (they can refer to static variableStatic variableIn computer programming, a static variable is a variable that has been allocated statically — whose lifetime extends across the entire run of the program...
s and methods of the enclosing class without qualifying the name; other classes that are not one of its enclosing classes have to qualify its name with its enclosing class's name). Nested interfaceProtocol (object-oriented programming)In object-oriented programming, a protocol or interface is a common means for unrelated objects to communicate with each other. These are definitions of methods and values which the objects agree upon in order to cooperate....
s are implicitly static.
Non-Static / Inner Classes
Inner class - The following categories are called inner classes. Each instance of these classes has a reference to an enclosing instance (i.e. an instance of the enclosing class), except for local and anonymous classes declared in static context. Hence, they can implicitly refer to instance variables and methods of the enclosing class. The enclosing instance reference can be explicitly obtained via
EnclosingClassName.this
. Inner classes may not have static variables or methods, except for compile-time constant variables. When they are created, they must have a reference to an instance of the enclosing class; which means they must either be created within an instance method or constructor of the enclosing class, or (for member and anonymous classes) be created using the syntax enclosingInstance.new InnerClass
.
- Member class - They are declared outside a function (hence a "member") and not declared "static".
- Local class - These are classes that are declared in the body of a function. They can only be referred to in the rest of the function. They can use local variables and parameters of the function, but only ones that are declared "final". (This is because the local class instance must maintain a separate copy of the variable, as it may out-live the function; so as not to have the confusion of two modifiable variables with the same name in the same scope, the variable is forced to be non-modifiable.)
- Anonymous class - These are local classes that are automatically declared and instantiated in the middle of an expression. They can only directly extend one class or implement one interface. They can specify arguments to the constructor of the superclass, but cannot otherwise have a constructor (however, this is not a limitation, since it can have an instance initializer block to perform any initialization).
Programming Languages
- Inner classes became a feature of the Java programming languageJava (programming language)Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities...
starting with version 1.1. - Nested classes are also a feature of the D programming languageD (programming language)The D programming language is an object-oriented, imperative, multi-paradigm, system programming language created by Walter Bright of Digital Mars. It originated as a re-engineering of C++, but even though it is mainly influenced by that language, it is not a variant of C++...
, Visual Basic .NETVisual Basic .NETVisual Basic .NET , is an object-oriented computer programming language that can be viewed as an evolution of the classic Visual Basic , which is implemented on the .NET Framework...
, C++C++C++ is a statically typed, free-form, multi-paradigm, compiled, general-purpose programming language. It is regarded as an intermediate-level language, as it comprises a combination of both high-level and low-level language features. It was developed by Bjarne Stroustrup starting in 1979 at Bell...
and C#. - In PythonPython (programming language)Python is a general-purpose, high-level programming language whose design philosophy emphasizes code readability. Python claims to "[combine] remarkable power with very clear syntax", and its standard library is large and comprehensive...
, it is possible to nest a class within another class, method or function. - C++C++C++ is a statically typed, free-form, multi-paradigm, compiled, general-purpose programming language. It is regarded as an intermediate-level language, as it comprises a combination of both high-level and low-level language features. It was developed by Bjarne Stroustrup starting in 1979 at Bell...
has nested classes that are like Java's static member classes, except that they are not declared with "static".
GUI code
Local inner classes are often used in Java to define callbacks for GUI code. Components can then share an object that implements an event handling interface or extends an abstract adapter class, containing the code to be executed when a given event is triggered.Anonymous inner classes are also used where the event handling code is only used by one component and therefore does not need a named reference.
This avoids a large monolithic
method with multiple if-else branches to identify the source of the event. This type of code is often considered messy and the inner class variations are considered to be better in all regards.