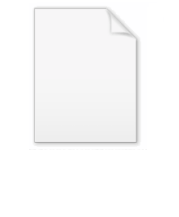
Fuxi Programming Language
Encyclopedia
Fuxi is a general-purpose, concurrent
, object-oriented, declarative programming language
. It was designed for use in application and systems programming, and implemented to meet the demands of network computing and mobility, and to provide a vehicle for rapid prototyping of software. It intends to make programming enjoyable, through the well-designed combination of the best ideas found in object-oriented, concurrent, functional, and logical programming paradigms, and the embodiment of a design philosophy: simplicity, efficiency, uniformity and consistency with conventions. So, Fuxi adopts a syntactical system and an object model strongly influenced by major current languages, such as C, C++
, C#, Java, though the language itself is more declarative.
, but differs from C++
and Java
in that it differentiates methods into functions, clauses, and triggers, and gives them a uniform syntax through pattern matching
. The notable language features include:
s:
import fuxi.*
public active Fibonacci : Applet
{
Fib(0) = 1
Fib(1) = 1
Fib( int n ) = Fib(n - 2) + Fib(n - 1)
public Activate =
let
{
int n = System.Console.Readln.ToInteger
}
in
{
System.Console.Print( "Please Input a Number:" )
System.Console.Println( "Fib(" + n + ")=" + Fib(n) )
}
}
Concurrency (computer science)
In computer science, concurrency is a property of systems in which several computations are executing simultaneously, and potentially interacting with each other...
, object-oriented, declarative programming language
Programming language
A programming language is an artificial language designed to communicate instructions to a machine, particularly a computer. Programming languages can be used to create programs that control the behavior of a machine and/or to express algorithms precisely....
. It was designed for use in application and systems programming, and implemented to meet the demands of network computing and mobility, and to provide a vehicle for rapid prototyping of software. It intends to make programming enjoyable, through the well-designed combination of the best ideas found in object-oriented, concurrent, functional, and logical programming paradigms, and the embodiment of a design philosophy: simplicity, efficiency, uniformity and consistency with conventions. So, Fuxi adopts a syntactical system and an object model strongly influenced by major current languages, such as C, C++
C++
C++ is a statically typed, free-form, multi-paradigm, compiled, general-purpose programming language. It is regarded as an intermediate-level language, as it comprises a combination of both high-level and low-level language features. It was developed by Bjarne Stroustrup starting in 1979 at Bell...
, C#, Java, though the language itself is more declarative.
Philosophy
Fuxi reflects the major aspects of Object-oriented programmingObject-oriented programming
Object-oriented programming is a programming paradigm using "objects" – data structures consisting of data fields and methods together with their interactions – to design applications and computer programs. Programming techniques may include features such as data abstraction,...
, but differs from C++
C++
C++ is a statically typed, free-form, multi-paradigm, compiled, general-purpose programming language. It is regarded as an intermediate-level language, as it comprises a combination of both high-level and low-level language features. It was developed by Bjarne Stroustrup starting in 1979 at Bell...
and Java
Java (programming language)
Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities...
in that it differentiates methods into functions, clauses, and triggers, and gives them a uniform syntax through pattern matching
Pattern matching
In computer science, pattern matching is the act of checking some sequence of tokens for the presence of the constituents of some pattern. In contrast to pattern recognition, the match usually has to be exact. The patterns generally have the form of either sequences or tree structures...
. The notable language features include:
- Orthogonal stylization of objects;
- Guarded fields;
- Pattern extending and overriding in inheritance;
- Scripted object definition.
Examples
The following is a simple example of Fuxi, which calculates the Fibonacci numberFibonacci number
In mathematics, the Fibonacci numbers are the numbers in the following integer sequence:0,\;1,\;1,\;2,\;3,\;5,\;8,\;13,\;21,\;34,\;55,\;89,\;144,\; \ldots\; ....
s:
import fuxi.*
public active Fibonacci : Applet
{
Fib(0) = 1
Fib(1) = 1
Fib( int n ) = Fib(n - 2) + Fib(n - 1)
public Activate =
let
{
int n = System.Console.Readln.ToInteger
}
in
{
System.Console.Print( "Please Input a Number:" )
System.Console.Println( "Fib(" + n + ")=" + Fib(n) )
}
}