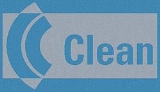
Clean programming language
Encyclopedia
In computer science
, Clean is a general-purpose
purely functional
computer
programming
language
.
:
referential transparency, list comprehension, guard
s, garbage collection
, higher order functions and currying
and lazy evaluation.
An integrated development environment
(IDE) is included in the Clean distribution.
Clean's method for dealing with mutable state and I/O is done through a uniqueness typing system
, in contrast to Haskell's use of monads
.
"The uniqueness type system also allows the Clean compiler to generate efficient code because uniquely
attributed data structures can be destructively updated."
:
module hello
Start :: {#Char}
Start = "Hello, world!"
Factorial
:
module factorial
fac 0 = 1
fac n = n * fac (n-1)
// find the factorial of 10
Start = fac 10
Factorial
:
module factorial2
import StdEnv
fac 0 = 1
fac n = prod [1..n]//Generate a list that goes from 1 to n and returns the product of the elements
// find the factorial of 6
Start = fac 6
Fibonacci sequence:
module fibonacci
fib 0 = 0
fib 1 = 1
fib n = fib (n - 2) + fib (n - 1)
Start = fib 7
Infix
operator:
(^) infixr 8 :: Int Int -> Int
(^) x 0 = 1
(^) x n = x * x ^ (n-1)
The type declaration states that the function is a right associative infix operator with priority 8: this states that
and reduction
. Constants such as numbers are graphs and functions are graph rewriting formulas. This, combined with compilation to native code, makes Clean programs relatively fast, even with high abstraction.
Earlier Clean system versions were written completely in C
, thus avoiding bootstrapping issues.
. It is also available with limited input/output
capabilities and without the "Dynamics" feature for Apple Macintosh, Solaris and Linux
.
d: it is available under the terms of the GNU LGPL
, and also under a proprietary license.
Computer science
Computer science or computing science is the study of the theoretical foundations of information and computation and of practical techniques for their implementation and application in computer systems...
, Clean is a general-purpose
General-purpose programming language
In computer software a general-purpose programming language is a programming language designed to be used for writing software in a wide variety of application domains...
purely functional
Purely functional
Purely functional is a term in computing used to describe algorithms, data structures or programming languages that exclude destructive modifications...
computer
Computer
A computer is a programmable machine designed to sequentially and automatically carry out a sequence of arithmetic or logical operations. The particular sequence of operations can be changed readily, allowing the computer to solve more than one kind of problem...
programming
Computer programming
Computer programming is the process of designing, writing, testing, debugging, and maintaining the source code of computer programs. This source code is written in one or more programming languages. The purpose of programming is to create a program that performs specific operations or exhibits a...
language
Programming language
A programming language is an artificial language designed to communicate instructions to a machine, particularly a computer. Programming languages can be used to create programs that control the behavior of a machine and/or to express algorithms precisely....
.
Features
The language Clean first appeared in 1987 and is still further developed; it shares many properties with HaskellHaskell (programming language)
Haskell is a standardized, general-purpose purely functional programming language, with non-strict semantics and strong static typing. It is named after logician Haskell Curry. In Haskell, "a function is a first-class citizen" of the programming language. As a functional programming language, the...
:
referential transparency, list comprehension, guard
Guard (computing)
In computer programming, a guard is a boolean expression that must evaluate to true if the program execution is to continue in the branch in question. The term is used at least in Haskell, Clean, Erlang, occam, Promela, OCaml and Scala programming languages. In Mathematica, guards are called...
s, garbage collection
Garbage collection (computer science)
In computer science, garbage collection is a form of automatic memory management. The garbage collector, or just collector, attempts to reclaim garbage, or memory occupied by objects that are no longer in use by the program...
, higher order functions and currying
Currying
In mathematics and computer science, currying is the technique of transforming a function that takes multiple arguments in such a way that it can be called as a chain of functions each with a single argument...
and lazy evaluation.
An integrated development environment
Integrated development environment
An integrated development environment is a software application that provides comprehensive facilities to computer programmers for software development...
(IDE) is included in the Clean distribution.
Clean's method for dealing with mutable state and I/O is done through a uniqueness typing system
Uniqueness type
In computing, a unique type guarantees that an object is used in a single-threaded way, with at most a single reference to it. If a value has a unique type, a function applied to it can be optimized to update the value in-place in the object code. In-place updates improve the efficiency of...
, in contrast to Haskell's use of monads
Monads in functional programming
In functional programming, a monad is a programming structure that represents computations. Monads are a kind of abstract data type constructor that encapsulate program logic instead of data in the domain model...
.
"The uniqueness type system also allows the Clean compiler to generate efficient code because uniquely
attributed data structures can be destructively updated."
Examples
Hello worldHello world program
A "Hello world" program is a computer program that outputs "Hello world" on a display device. Because it is typically one of the simplest programs possible in most programming languages, it is by tradition often used to illustrate to beginners the most basic syntax of a programming language, or to...
:
module hello
Start :: {#Char}
Start = "Hello, world!"
Factorial
Factorial
In mathematics, the factorial of a non-negative integer n, denoted by n!, is the product of all positive integers less than or equal to n...
:
module factorial
fac 0 = 1
fac n = n * fac (n-1)
// find the factorial of 10
Start = fac 10
Factorial
Factorial
In mathematics, the factorial of a non-negative integer n, denoted by n!, is the product of all positive integers less than or equal to n...
:
module factorial2
import StdEnv
fac 0 = 1
fac n = prod [1..n]//Generate a list that goes from 1 to n and returns the product of the elements
// find the factorial of 6
Start = fac 6
Fibonacci sequence:
module fibonacci
fib 0 = 0
fib 1 = 1
fib n = fib (n - 2) + fib (n - 1)
Start = fib 7
Infix
Infix notation
Infix notation is the common arithmetic and logical formula notation, in which operators are written infix-style between the operands they act on . It is not as simple to parse by computers as prefix notation or postfix notation Infix notation is the common arithmetic and logical formula notation,...
operator:
(^) infixr 8 :: Int Int -> Int
(^) x 0 = 1
(^) x n = x * x ^ (n-1)
The type declaration states that the function is a right associative infix operator with priority 8: this states that
x*x^(n-1)
is equivalent to x*(x^(n-1))
as opposed to (x*x)^(n-1)
; this operator is pre-defined in the Clean standard environment.How Clean works
Computation is based on graph rewritingGraph rewriting
Graph transformation, or Graph rewriting, concerns the technique of creating a new graph out of an original graph using some automatic machine. It has numerous applications, ranging from software verification to layout algorithms....
and reduction
Graph reduction
In computer science, graph reduction implements an efficient version of non-strict evaluation, an evaluation strategy where the arguments to a function are not immediately evaluated. This form of non-strict evaluation is also known as lazy evaluation and used in functional programming languages...
. Constants such as numbers are graphs and functions are graph rewriting formulas. This, combined with compilation to native code, makes Clean programs relatively fast, even with high abstraction.
Compiling
- Source files (.icl) and project files (.dcl) are converted into Clean's platform-independent bytecode (.abc), implemented in CC (programming language)C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system....
and Clean. - Bytecode is converted to object code (.obj) using CC (programming language)C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system....
. - object code is linked with other files in the module and the runtime system and converted into a normal executable in Clean.
Earlier Clean system versions were written completely in C
C (programming language)
C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system....
, thus avoiding bootstrapping issues.
Platforms
Clean is available for Microsoft WindowsMicrosoft Windows
Microsoft Windows is a series of operating systems produced by Microsoft.Microsoft introduced an operating environment named Windows on November 20, 1985 as an add-on to MS-DOS in response to the growing interest in graphical user interfaces . Microsoft Windows came to dominate the world's personal...
. It is also available with limited input/output
Input/output
In computing, input/output, or I/O, refers to the communication between an information processing system , and the outside world, possibly a human, or another information processing system. Inputs are the signals or data received by the system, and outputs are the signals or data sent from it...
capabilities and without the "Dynamics" feature for Apple Macintosh, Solaris and Linux
Linux
Linux is a Unix-like computer operating system assembled under the model of free and open source software development and distribution. The defining component of any Linux system is the Linux kernel, an operating system kernel first released October 5, 1991 by Linus Torvalds...
.
License
Clean is dual licenseDual license
Multi-licensing is the practice of distributing software under two or more different sets of terms and conditions. This may mean multiple different licenses or sets of licenses. Prefixes may be used to indicate the number of licenses used, e.g...
d: it is available under the terms of the GNU LGPL
GNU Lesser General Public License
The GNU Lesser General Public License or LGPL is a free software license published by the Free Software Foundation . It was designed as a compromise between the strong-copyleft GNU General Public License or GPL and permissive licenses such as the BSD licenses and the MIT License...
, and also under a proprietary license.
Speed
Some state that Clean is faster than Haskell, but other research show that this depends on the kind of program that is tested.Syntactic differences
The syntax of Clean is very similar to Haskell, with some notable differences:Haskell | Clean | Remarks |
---|---|---|
(a -> b) -> [a] -> [b] | (a -> b) [a] -> [b] | higher order function |
f . g | f o g | function composition Function composition (computer science) In computer science, function composition is an act or mechanism to combine simple functions to build more complicated ones... |
-5 | ~5 | unary minus |
[ x > x <- [1..10] , isOdd x] | [ x \\ x <- [1..10] > isOdd x] | list comprehension |
x:xs | |
cons Cons In computer programming, cons is a fundamental function in most dialects of the Lisp programming language. cons constructs memory objects which hold two values or pointers to values. These objects are referred to as cells, conses, non-atomic s-expressions , or pairs... operator |
See also
- Haskell programming languageHaskell (programming language)Haskell is a standardized, general-purpose purely functional programming language, with non-strict semantics and strong static typing. It is named after logician Haskell Curry. In Haskell, "a function is a first-class citizen" of the programming language. As a functional programming language, the...
- List of functional programming topics